NotificationManager::Settings
#include <settings.h>
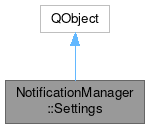
Public Types | |
enum | NotificationBehavior { ShowPopups = 1 << 1 , ShowPopupsInDoNotDisturbMode = 1 << 2 , ShowInHistory = 1 << 3 , ShowBadges = 1 << 4 } |
typedef QFlags< NotificationBehavior > | NotificationBehaviors |
enum | PopupPosition { CloseToWidget = 0 , TopLeft , TopCenter , TopRight , BottomLeft , BottomCenter , BottomRight } |
![]() | |
typedef | QObjectList |
Signals | |
void | dirtyChanged () |
void | knownApplicationsChanged () |
void | liveChanged () |
void | notificationInhibitionApplicationsChanged () |
void | notificationsInhibitedByApplicationChanged (bool notificationsInhibitedByApplication) |
void | screensMirroredChanged () |
void | settingsChanged () |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Notification settings and state.
This class encapsulates all global settings related to notifications as well as do not disturb mode and other state.
This class can be used by applications to alter their behavior depending on user's notification preferences.
Definition at line 34 of file settings.h.
Member Typedef Documentation
◆ NotificationBehaviors
QFlags< NotificationBehavior > NotificationManager::Settings::NotificationBehaviors |
Definition at line 228 of file settings.h.
Member Enumeration Documentation
◆ NotificationBehavior
enum NotificationManager::Settings::NotificationBehavior |
Definition at line 221 of file settings.h.
◆ PopupPosition
enum NotificationManager::Settings::PopupPosition |
Definition at line 210 of file settings.h.
Property Documentation
◆ badgeBlacklistedApplications
|
read |
A list of desktop entries of applications which shouldn't show badges in task manager.
Definition at line 117 of file settings.h.
◆ badgesInTaskManager
|
readwrite |
Whether to show notification badges (numbers in circles) in task manager.
Definition at line 80 of file settings.h.
◆ criticalPopupsInDoNotDisturbMode
|
readwrite |
Whether to show critical notification popups in do not disturb mode.
Definition at line 42 of file settings.h.
◆ dirty
|
read |
Whether the settings have changed and need to be saved.
- See also
- save()
Definition at line 200 of file settings.h.
◆ doNotDisturbPopupWhitelistedApplications
|
read |
A list of desktop entries of applications for which a popup should be shown even in do not disturb mode.
Definition at line 99 of file settings.h.
◆ doNotDisturbPopupWhitelistedServices
|
read |
A list of notifyrc names of services for which a popup should be shown even in do not disturb mode.
Definition at line 103 of file settings.h.
◆ historyBlacklistedApplications
|
read |
A list of desktop entries of applications which shouldn't be shown in the history.
Definition at line 108 of file settings.h.
◆ historyBlacklistedServices
|
read |
A list of notifyrc names of services which shouldn't be shown in the history.
Definition at line 112 of file settings.h.
◆ inhibitNotificationsWhenScreenSharing
|
readwrite |
Whether to enable do not disturb mode while screen sharing.
- Since
- 5.22
Definition at line 168 of file settings.h.
◆ inhibitNotificationsWhenScreensMirrored
|
readwrite |
Whether to enable do not disturb mode when screens are mirrored/overlapping.
- Since
- 5.17
Definition at line 148 of file settings.h.
◆ jobsInNotifications
|
readwrite |
Whether to show application jobs as notifications.
Definition at line 71 of file settings.h.
◆ keepNormalAlwaysOnTop
|
readwrite |
Whether to keep normal notifications always on top.
Definition at line 46 of file settings.h.
◆ knownApplications
|
read |
A list of desktop entries of applications that have been seen sending a notification.
Definition at line 85 of file settings.h.
◆ live
|
readwrite |
Whether to update the properties immediately when they are changed on disk.
This can be undesirable for a settings dialog where outside changes should not suddenly cause the UI to change.
Default is true.
Definition at line 193 of file settings.h.
◆ lowPriorityHistory
|
readwrite |
Whether to add low priority notifications to the history.
Definition at line 54 of file settings.h.
◆ lowPriorityPopups
|
readwrite |
Whether to show popups for low priority notifications.
Definition at line 50 of file settings.h.
◆ notificationInhibitionApplications
|
read |
Definition at line 139 of file settings.h.
◆ notificationInhibitionReasons
|
read |
Definition at line 141 of file settings.h.
◆ notificationsInhibitedByApplication
|
read |
Whether an application currently requested do not disturb mode.
Do not disturb mode is considered active when this property is true OR notificationsInhibitedUntil points to a date in the future.
- See also
- revokeApplicationInhibitions
Definition at line 137 of file settings.h.
◆ notificationsInhibitedUntil
|
readwrite |
The date until which do not disturb mode is enabled.
When invalid or in the past, do not disturb mode should be considered disabled. Do not disturb mode is considered active when this property points to a date in the future OR notificationsInhibitedByApplication is true.
Definition at line 126 of file settings.h.
◆ notificationSoundsInhibited
|
readwrite |
Whether notification sounds should be disabled.
This does not reflect the actual mute state of the Notification Sounds stream but only remembers what value was assigned to this property.
This way you can tell whether to unmute notification sounds or not, in case the user had them explicitly muted previously.
- Note
- This does not actually mute or unmute the actual sound stream, you need to do this yourself using e.g. PulseAudio.
Definition at line 183 of file settings.h.
◆ permanentJobPopups
|
readwrite |
Whether application jobs stay visible for the whole duration of the job.
Definition at line 75 of file settings.h.
◆ popupBlacklistedApplications
|
read |
A list of desktop entries of applications for which no popups should be shown.
Definition at line 90 of file settings.h.
◆ popupBlacklistedServices
|
read |
A list of notifyrc names of services for which no popups should be shown.
Definition at line 94 of file settings.h.
◆ popupPosition
|
readwrite |
The notification popup position on screen.
CloseToWidget means they should be positioned closely to where the plasmoid is located on screen.
Definition at line 60 of file settings.h.
◆ popupTimeout
|
readwrite |
The default timeout for notification popups that do not have an explicit timeout set, in milliseconds.
Default is 5000ms (5 seconds).
Definition at line 66 of file settings.h.
◆ screensMirrored
|
readwrite |
Whether there currently are mirrored/overlapping screens.
This property is only updated when inhibitNotificationsWhenScreensMirrored
is set to true, otherwise it is always false. You can assign false to this property if you want to temporarily revoke automatic do not disturb mode when screens are mirrored until the screen configuration changes.
- Since
- 5.17
Definition at line 161 of file settings.h.
Constructor & Destructor Documentation
◆ Settings() [1/2]
|
explicit |
Definition at line 148 of file settings.cpp.
◆ Settings() [2/2]
Settings::Settings | ( | const KSharedConfig::Ptr & | config, |
QObject * | parent = nullptr ) |
Definition at line 165 of file settings.cpp.
◆ ~Settings()
|
override |
Definition at line 171 of file settings.cpp.
Member Function Documentation
◆ applicationBehavior()
Settings::NotificationBehaviors Settings::applicationBehavior | ( | const QString & | desktopEntry | ) | const |
Definition at line 176 of file settings.cpp.
◆ badgeBlacklistedApplications()
QStringList Settings::badgeBlacklistedApplications | ( | ) | const |
Definition at line 497 of file settings.cpp.
◆ badgesInTaskManager()
bool Settings::badgesInTaskManager | ( | ) | const |
Definition at line 448 of file settings.cpp.
◆ criticalPopupsInDoNotDisturbMode()
bool Settings::criticalPopupsInDoNotDisturbMode | ( | ) | const |
Definition at line 332 of file settings.cpp.
◆ defaults()
void Settings::defaults | ( | ) |
Definition at line 260 of file settings.cpp.
◆ dirty()
bool Settings::dirty | ( | ) | const |
Definition at line 325 of file settings.cpp.
◆ doNotDisturbPopupWhitelistedApplications()
QStringList Settings::doNotDisturbPopupWhitelistedApplications | ( | ) | const |
Definition at line 477 of file settings.cpp.
◆ doNotDisturbPopupWhitelistedServices()
QStringList Settings::doNotDisturbPopupWhitelistedServices | ( | ) | const |
Definition at line 482 of file settings.cpp.
◆ forgetKnownApplication()
void Settings::forgetKnownApplication | ( | const QString & | desktopEntry | ) |
Definition at line 220 of file settings.cpp.
◆ historyBlacklistedApplications()
QStringList Settings::historyBlacklistedApplications | ( | ) | const |
Definition at line 487 of file settings.cpp.
◆ historyBlacklistedServices()
QStringList Settings::historyBlacklistedServices | ( | ) | const |
Definition at line 492 of file settings.cpp.
◆ inhibitNotificationsWhenScreenSharing()
bool Settings::inhibitNotificationsWhenScreenSharing | ( | ) | const |
Definition at line 565 of file settings.cpp.
◆ inhibitNotificationsWhenScreensMirrored()
bool Settings::inhibitNotificationsWhenScreensMirrored | ( | ) | const |
Definition at line 533 of file settings.cpp.
◆ jobsInNotifications()
bool Settings::jobsInNotifications | ( | ) | const |
Definition at line 421 of file settings.cpp.
◆ keepNormalAlwaysOnTop()
bool Settings::keepNormalAlwaysOnTop | ( | ) | const |
Definition at line 346 of file settings.cpp.
◆ knownApplications()
QStringList Settings::knownApplications | ( | ) | const |
Definition at line 462 of file settings.cpp.
◆ live()
bool Settings::live | ( | ) | const |
Definition at line 270 of file settings.cpp.
◆ load()
void Settings::load | ( | ) |
Definition at line 237 of file settings.cpp.
◆ lowPriorityHistory()
bool Settings::lowPriorityHistory | ( | ) | const |
Definition at line 374 of file settings.cpp.
◆ lowPriorityPopups()
bool Settings::lowPriorityPopups | ( | ) | const |
Definition at line 360 of file settings.cpp.
◆ notificationInhibitionApplications()
QStringList Settings::notificationInhibitionApplications | ( | ) | const |
Definition at line 523 of file settings.cpp.
◆ notificationInhibitionReasons()
QStringList Settings::notificationInhibitionReasons | ( | ) | const |
Definition at line 528 of file settings.cpp.
◆ notificationsInhibitedByApplication()
bool Settings::notificationsInhibitedByApplication | ( | ) | const |
Definition at line 518 of file settings.cpp.
◆ notificationsInhibitedUntil()
QDateTime Settings::notificationsInhibitedUntil | ( | ) | const |
Definition at line 502 of file settings.cpp.
◆ notificationSoundsInhibited()
bool Settings::notificationSoundsInhibited | ( | ) | const |
Definition at line 585 of file settings.cpp.
◆ permanentJobPopups()
bool Settings::permanentJobPopups | ( | ) | const |
Definition at line 434 of file settings.cpp.
◆ popupBlacklistedApplications()
QStringList Settings::popupBlacklistedApplications | ( | ) | const |
Definition at line 467 of file settings.cpp.
◆ popupBlacklistedServices()
QStringList Settings::popupBlacklistedServices | ( | ) | const |
Definition at line 472 of file settings.cpp.
◆ popupPosition()
Settings::PopupPosition Settings::popupPosition | ( | ) | const |
Definition at line 388 of file settings.cpp.
◆ popupTimeout()
int Settings::popupTimeout | ( | ) | const |
Definition at line 402 of file settings.cpp.
◆ registerKnownApplication()
void Settings::registerKnownApplication | ( | const QString & | desktopEntry | ) |
Definition at line 198 of file settings.cpp.
◆ resetNotificationsInhibitedUntil()
void Settings::resetNotificationsInhibitedUntil | ( | ) |
Definition at line 513 of file settings.cpp.
◆ resetPopupTimeout()
void Settings::resetPopupTimeout | ( | ) |
Definition at line 416 of file settings.cpp.
◆ revokeApplicationInhibitions()
void Settings::revokeApplicationInhibitions | ( | ) |
Revoke application notification inhibitions.
- Note
- Applications are not notified of the fact that their inhibition might have been taken away.
Definition at line 580 of file settings.cpp.
◆ save()
void Settings::save | ( | ) |
Definition at line 249 of file settings.cpp.
◆ screensMirrored()
bool Settings::screensMirrored | ( | ) | const |
Definition at line 548 of file settings.cpp.
◆ serviceBehavior()
Settings::NotificationBehaviors Settings::serviceBehavior | ( | const QString & | desktopEntry | ) | const |
Definition at line 187 of file settings.cpp.
◆ setApplicationBehavior()
void Settings::setApplicationBehavior | ( | const QString & | desktopEntry, |
NotificationBehaviors | behaviors ) |
Definition at line 181 of file settings.cpp.
◆ setBadgesInTaskManager()
void Settings::setBadgesInTaskManager | ( | bool | enable | ) |
Definition at line 453 of file settings.cpp.
◆ setCriticalPopupsInDoNotDisturbMode()
void Settings::setCriticalPopupsInDoNotDisturbMode | ( | bool | enable | ) |
Definition at line 337 of file settings.cpp.
◆ setInhibitNotificationsWhenScreenSharing()
void Settings::setInhibitNotificationsWhenScreenSharing | ( | bool | inhibit | ) |
Definition at line 570 of file settings.cpp.
◆ setInhibitNotificationsWhenScreensMirrored()
void Settings::setInhibitNotificationsWhenScreensMirrored | ( | bool | mirrored | ) |
Definition at line 538 of file settings.cpp.
◆ setJobsInNotifications()
void Settings::setJobsInNotifications | ( | bool | enable | ) |
Definition at line 425 of file settings.cpp.
◆ setKeepNormalAlwaysOnTop()
void Settings::setKeepNormalAlwaysOnTop | ( | bool | enable | ) |
Definition at line 351 of file settings.cpp.
◆ setLive()
void Settings::setLive | ( | bool | live | ) |
Definition at line 275 of file settings.cpp.
◆ setLowPriorityHistory()
void Settings::setLowPriorityHistory | ( | bool | enable | ) |
Definition at line 379 of file settings.cpp.
◆ setLowPriorityPopups()
void Settings::setLowPriorityPopups | ( | bool | enable | ) |
Definition at line 365 of file settings.cpp.
◆ setNotificationsInhibitedUntil()
void Settings::setNotificationsInhibitedUntil | ( | const QDateTime & | time | ) |
Definition at line 507 of file settings.cpp.
◆ setNotificationSoundsInhibited()
void Settings::setNotificationSoundsInhibited | ( | bool | inhibited | ) |
Definition at line 590 of file settings.cpp.
◆ setPermanentJobPopups()
void Settings::setPermanentJobPopups | ( | bool | enable | ) |
Definition at line 439 of file settings.cpp.
◆ setPopupPosition()
void Settings::setPopupPosition | ( | Settings::PopupPosition | position | ) |
Definition at line 393 of file settings.cpp.
◆ setPopupTimeout()
void Settings::setPopupTimeout | ( | int | popupTimeout | ) |
Definition at line 407 of file settings.cpp.
◆ setScreensMirrored()
void Settings::setScreensMirrored | ( | bool | enable | ) |
Definition at line 553 of file settings.cpp.
◆ setServiceBehavior()
void Settings::setServiceBehavior | ( | const QString & | desktopEntry, |
NotificationBehaviors | behaviors ) |
Definition at line 192 of file settings.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 3 2025 11:55:13 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.