TaskManager::VirtualDesktopInfo
#include <virtualdesktopinfo.h>
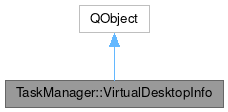
Properties | |
QML_ELEMENTQVariant | currentDesktop |
QVariantList | desktopIds |
int | desktopLayoutRows |
QStringList | desktopNames |
int | navigationWrappingAround |
int | numberOfDesktops |
![]() | |
objectName | |
Public Member Functions | |
VirtualDesktopInfo (QObject *parent=nullptr) | |
QVariant | currentDesktop () const |
QVariantList | desktopIds () const |
int | desktopLayoutRows () const |
QStringList | desktopNames () const |
bool | navigationWrappingAround () const |
int | numberOfDesktops () const |
quint32 | position (const QVariant &desktop) const |
void | requestActivate (const QVariant &desktop) |
void | requestCreateDesktop (quint32 position) |
void | requestRemoveDesktop (quint32 position) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Provides basic virtual desktop information.
The underlying windowing system is abstracted away.
This class provides basic information about the virtual desktops present in the session as a set of notifiable properties.
Definition at line 26 of file virtualdesktopinfo.h.
Property Documentation
◆ currentDesktop
|
read |
Definition at line 31 of file virtualdesktopinfo.h.
◆ desktopIds
|
read |
Definition at line 33 of file virtualdesktopinfo.h.
◆ desktopLayoutRows
|
read |
Definition at line 35 of file virtualdesktopinfo.h.
◆ desktopNames
|
read |
Definition at line 34 of file virtualdesktopinfo.h.
◆ navigationWrappingAround
|
read |
Definition at line 36 of file virtualdesktopinfo.h.
◆ numberOfDesktops
|
read |
Definition at line 32 of file virtualdesktopinfo.h.
Constructor & Destructor Documentation
◆ VirtualDesktopInfo()
|
explicit |
Definition at line 513 of file virtualdesktopinfo.cpp.
◆ ~VirtualDesktopInfo()
|
override |
Definition at line 536 of file virtualdesktopinfo.cpp.
Member Function Documentation
◆ currentDesktop()
QVariant TaskManager::VirtualDesktopInfo::currentDesktop | ( | ) | const |
The currently active virtual desktop.
- Returns
- the id of the currently active virtual desktop. QString on Wayland; uint >0 on X11.
Definition at line 546 of file virtualdesktopinfo.cpp.
◆ desktopIds()
QVariantList TaskManager::VirtualDesktopInfo::desktopIds | ( | ) | const |
The ids of all virtual desktops present in the session.
On Wayland, the ids are QString. On X11, they are uint >0.
- Returns
- a the list of ids of the virtual desktops present in the session.
Definition at line 556 of file virtualdesktopinfo.cpp.
◆ desktopLayoutRows()
int TaskManager::VirtualDesktopInfo::desktopLayoutRows | ( | ) | const |
The number of rows in the virtual desktop layout.
- Returns
- the number of rows in the virtual desktop layout.
Definition at line 571 of file virtualdesktopinfo.cpp.
◆ desktopNames()
QStringList TaskManager::VirtualDesktopInfo::desktopNames | ( | ) | const |
The names of all virtual desktops present in the session.
Note that on X11, virtual desktops are indexed starting at 1, so the name for virtual desktop 1 is at index 0 in this list.
- Returns
- the list of names of the virtual desktops present in the session.
Definition at line 561 of file virtualdesktopinfo.cpp.
◆ navigationWrappingAround()
bool TaskManager::VirtualDesktopInfo::navigationWrappingAround | ( | ) | const |
Whether or not to wrap navigation such that activating the next virtual desktop when at the last one will go to the first one, and activating the previous virtual desktop when at the first one will go to the last one.
Definition at line 591 of file virtualdesktopinfo.cpp.
◆ numberOfDesktops()
int TaskManager::VirtualDesktopInfo::numberOfDesktops | ( | ) | const |
The number of virtual desktops present in the session.
- Returns
- the number of virtual desktops present in the session.
Definition at line 551 of file virtualdesktopinfo.cpp.
◆ position()
quint32 TaskManager::VirtualDesktopInfo::position | ( | const QVariant & | desktop | ) | const |
Returns the position of the passed-in virtual desktop.
- Parameters
-
desktop A virtual desktop id (QString on Wayland; uint >0 on X11).
- Returns
- the position of the virtual desktop, or -1 if the desktop id is not valid.
Definition at line 566 of file virtualdesktopinfo.cpp.
◆ requestActivate()
void TaskManager::VirtualDesktopInfo::requestActivate | ( | const QVariant & | desktop | ) |
Request activating the passed-in virtual desktop.
- Parameters
-
desktop A virtual desktop id (QString on Wayland; uint >0 on X11).
Definition at line 576 of file virtualdesktopinfo.cpp.
◆ requestCreateDesktop()
void TaskManager::VirtualDesktopInfo::requestCreateDesktop | ( | quint32 | position | ) |
Request adding a new virtual desktop at the specified position.
On X11, the position parameter is ignored and the new desktop is always created at the end of the list.
- Parameters
-
position The position of the requested new virtual desktop (ignored on X11).
Definition at line 581 of file virtualdesktopinfo.cpp.
◆ requestRemoveDesktop()
void TaskManager::VirtualDesktopInfo::requestRemoveDesktop | ( | quint32 | position | ) |
Request removing the virtual desktop at the specified position.
On X11, the position parameter is ignored and the last desktop in the list is always the one removed.
- Parameters
-
position The position of the virtual desktop to remove (ignored on X11).
Definition at line 586 of file virtualdesktopinfo.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:59:37 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.