TaskManager::XWindowTasksModel
#include <xwindowtasksmodel.h>
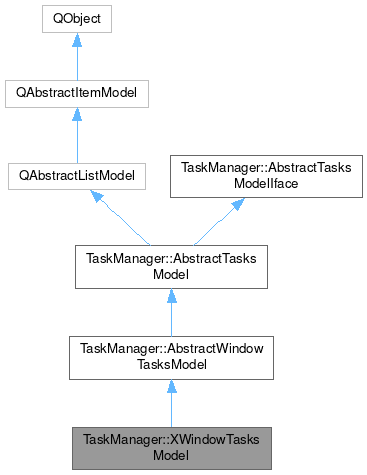
Public Member Functions | |
XWindowTasksModel (QObject *parent=nullptr) | |
QVariant | data (const QModelIndex &index, int role=Qt::DisplayRole) const override |
void | requestActivate (const QModelIndex &index) override |
void | requestActivities (const QModelIndex &index, const QStringList &activities) override |
void | requestClose (const QModelIndex &index) override |
void | requestMove (const QModelIndex &index) override |
void | requestNewInstance (const QModelIndex &index) override |
void | requestNewVirtualDesktop (const QModelIndex &index) override |
void | requestOpenUrls (const QModelIndex &index, const QList< QUrl > &urls) override |
void | requestPublishDelegateGeometry (const QModelIndex &index, const QRect &geometry, QObject *delegate=nullptr) override |
void | requestResize (const QModelIndex &index) override |
void | requestToggleFullScreen (const QModelIndex &index) override |
void | requestToggleKeepAbove (const QModelIndex &index) override |
void | requestToggleKeepBelow (const QModelIndex &index) override |
void | requestToggleMaximized (const QModelIndex &index) override |
void | requestToggleMinimized (const QModelIndex &index) override |
void | requestToggleNoBorder (const QModelIndex &index) override |
void | requestToggleShaded (const QModelIndex &index) override |
void | requestVirtualDesktops (const QModelIndex &index, const QVariantList &desktops) override |
int | rowCount (const QModelIndex &parent=QModelIndex()) const override |
![]() | |
AbstractWindowTasksModel (QObject *parent=nullptr) | |
![]() | |
AbstractTasksModel (QObject *parent=nullptr) | |
QModelIndex | index (int row, int column=0, const QModelIndex &parent=QModelIndex()) const override |
QHash< int, QByteArray > | roleNames () const override |
![]() | |
QAbstractListModel (QObject *parent) | |
virtual bool | dropMimeData (const QMimeData *data, Qt::DropAction action, int row, int column, const QModelIndex &parent) override |
virtual Qt::ItemFlags | flags (const QModelIndex &index) const const override |
virtual QModelIndex | sibling (int row, int column, const QModelIndex &idx) const const override |
![]() | |
QAbstractItemModel (QObject *parent) | |
virtual QModelIndex | buddy (const QModelIndex &index) const const |
virtual bool | canDropMimeData (const QMimeData *data, Qt::DropAction action, int row, int column, const QModelIndex &parent) const const |
virtual bool | canFetchMore (const QModelIndex &parent) const const |
bool | checkIndex (const QModelIndex &index, CheckIndexOptions options) const const |
virtual bool | clearItemData (const QModelIndex &index) |
virtual int | columnCount (const QModelIndex &parent) const const=0 |
void | columnsAboutToBeInserted (const QModelIndex &parent, int first, int last) |
void | columnsAboutToBeMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationColumn) |
void | columnsAboutToBeRemoved (const QModelIndex &parent, int first, int last) |
void | columnsInserted (const QModelIndex &parent, int first, int last) |
void | columnsMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationColumn) |
void | columnsRemoved (const QModelIndex &parent, int first, int last) |
void | dataChanged (const QModelIndex &topLeft, const QModelIndex &bottomRight, const QList< int > &roles) |
virtual void | fetchMore (const QModelIndex &parent) |
virtual bool | hasChildren (const QModelIndex &parent) const const |
bool | hasIndex (int row, int column, const QModelIndex &parent) const const |
virtual QVariant | headerData (int section, Qt::Orientation orientation, int role) const const |
void | headerDataChanged (Qt::Orientation orientation, int first, int last) |
bool | insertColumn (int column, const QModelIndex &parent) |
virtual bool | insertColumns (int column, int count, const QModelIndex &parent) |
bool | insertRow (int row, const QModelIndex &parent) |
virtual bool | insertRows (int row, int count, const QModelIndex &parent) |
virtual QMap< int, QVariant > | itemData (const QModelIndex &index) const const |
void | layoutAboutToBeChanged (const QList< QPersistentModelIndex > &parents, QAbstractItemModel::LayoutChangeHint hint) |
void | layoutChanged (const QList< QPersistentModelIndex > &parents, QAbstractItemModel::LayoutChangeHint hint) |
virtual QModelIndexList | match (const QModelIndex &start, int role, const QVariant &value, int hits, Qt::MatchFlags flags) const const |
virtual QMimeData * | mimeData (const QModelIndexList &indexes) const const |
virtual QStringList | mimeTypes () const const |
void | modelAboutToBeReset () |
void | modelReset () |
bool | moveColumn (const QModelIndex &sourceParent, int sourceColumn, const QModelIndex &destinationParent, int destinationChild) |
virtual bool | moveColumns (const QModelIndex &sourceParent, int sourceColumn, int count, const QModelIndex &destinationParent, int destinationChild) |
bool | moveRow (const QModelIndex &sourceParent, int sourceRow, const QModelIndex &destinationParent, int destinationChild) |
virtual bool | moveRows (const QModelIndex &sourceParent, int sourceRow, int count, const QModelIndex &destinationParent, int destinationChild) |
virtual void | multiData (const QModelIndex &index, QModelRoleDataSpan roleDataSpan) const const |
virtual QModelIndex | parent (const QModelIndex &index) const const=0 |
bool | removeColumn (int column, const QModelIndex &parent) |
virtual bool | removeColumns (int column, int count, const QModelIndex &parent) |
bool | removeRow (int row, const QModelIndex &parent) |
virtual bool | removeRows (int row, int count, const QModelIndex &parent) |
virtual void | revert () |
void | rowsAboutToBeInserted (const QModelIndex &parent, int start, int end) |
void | rowsAboutToBeMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationRow) |
void | rowsAboutToBeRemoved (const QModelIndex &parent, int first, int last) |
void | rowsInserted (const QModelIndex &parent, int first, int last) |
void | rowsMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationRow) |
void | rowsRemoved (const QModelIndex &parent, int first, int last) |
virtual bool | setData (const QModelIndex &index, const QVariant &value, int role) |
virtual bool | setHeaderData (int section, Qt::Orientation orientation, const QVariant &value, int role) |
virtual bool | setItemData (const QModelIndex &index, const QMap< int, QVariant > &roles) |
virtual void | sort (int column, Qt::SortOrder order) |
virtual QSize | span (const QModelIndex &index) const const |
virtual bool | submit () |
virtual Qt::DropActions | supportedDragActions () const const |
virtual Qt::DropActions | supportedDropActions () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static WId | winIdFromMimeData (const QMimeData *mimeData, bool *ok=nullptr) |
static QList< WId > | winIdsFromMimeData (const QMimeData *mimeData, bool *ok=nullptr) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Detailed Description
A tasks model for X Window System windows.
This model presents tasks sourced from window data on the X Windows server the host process is connected to.
For the purposes of presentation in a user interface and efficiency, certain types of windows (e.g. utility windows, or windows that are transients for an otherwise-included window) are omitted from the model.
- See also
- WindowTasksModel
Definition at line 38 of file xwindowtasksmodel.h.
Constructor & Destructor Documentation
◆ XWindowTasksModel()
|
explicit |
Definition at line 612 of file xwindowtasksmodel.cpp.
◆ ~XWindowTasksModel()
|
override |
Definition at line 619 of file xwindowtasksmodel.cpp.
Member Function Documentation
◆ data()
|
overridevirtual |
Reimplemented from TaskManager::AbstractTasksModel.
Definition at line 623 of file xwindowtasksmodel.cpp.
◆ requestActivate()
|
overridevirtual |
Request activation of the window at the given index.
If the window has a transient demanding attention, it will be activated instead.
If the window has a transient in shaded state, it will be activated instead.
- Parameters
-
index An index in this window tasks model.
Reimplemented from TaskManager::AbstractTasksModel.
Definition at line 732 of file xwindowtasksmodel.cpp.
◆ requestActivities()
|
overridevirtual |
Request moving the task at the given index to the specified activities.
This is meant for tasks that have an associated window, and may be a no-op when there is no window.
- Parameters
-
index An index in this tasks model. activities The new list of activities.
Reimplemented from TaskManager::AbstractTasksModel.
Definition at line 1046 of file xwindowtasksmodel.cpp.
◆ requestClose()
|
overridevirtual |
Request the window at the given index be closed.
- Parameters
-
index An index in this window tasks model.
Reimplemented from TaskManager::AbstractTasksModel.
Definition at line 783 of file xwindowtasksmodel.cpp.
◆ requestMove()
|
overridevirtual |
Request starting an interactive move for the window at the given index.
If the window is not currently the active window, it will be activated.
If the window is not on the current desktop, the current desktop will be set to the window's desktop. FIXME: Desktop logic should maybe move into proxy.
- Parameters
-
index An index in this window tasks model.
Reimplemented from TaskManager::AbstractTasksModel.
Definition at line 793 of file xwindowtasksmodel.cpp.
◆ requestNewInstance()
|
overridevirtual |
Request an additional instance of the application owning the window at the given index.
Success depends on whether a AbstractTasksModel::LauncherUrl could be derived from window metadata.
- Parameters
-
index An index in this window tasks model.
Reimplemented from TaskManager::AbstractTasksModel.
Definition at line 765 of file xwindowtasksmodel.cpp.
◆ requestNewVirtualDesktop()
|
overridevirtual |
Request entering the window at the given index on a new virtual desktop, which is created in response to this request.
- Parameters
-
index An index in this window tasks model.
Reimplemented from TaskManager::AbstractTasksModel.
Definition at line 1026 of file xwindowtasksmodel.cpp.
◆ requestOpenUrls()
|
overridevirtual |
Runs the application backing the launcher at the given index with the given URLs.
Success depends on whether a AbstractTasksModel::LauncherUrl could be derived from window metadata and a KService could be found from that.
- Parameters
-
index An index in this launcher tasks model urls The URLs to be passed to the application
Reimplemented from TaskManager::AbstractTasksModel.
Definition at line 774 of file xwindowtasksmodel.cpp.
◆ requestPublishDelegateGeometry()
|
overridevirtual |
Request informing the window manager of new geometry for a visual delegate for the window at the given index.
- Parameters
-
index An index in this window tasks model. geometry Visual delegate geometry in screen coordinates. delegate The delegate. Unused in this implementation.
Reimplemented from TaskManager::AbstractTasksModel.
Definition at line 1057 of file xwindowtasksmodel.cpp.
◆ requestResize()
|
overridevirtual |
Request starting an interactive resize for the window at the given index.
If the window is not currently the active window, it will be activated.
If the window is not on the current desktop, the current desktop will be set to the window's desktop. FIXME: Desktop logic should maybe move into proxy.
- Parameters
-
index An index in this window tasks model.
Reimplemented from TaskManager::AbstractTasksModel.
Definition at line 819 of file xwindowtasksmodel.cpp.
◆ requestToggleFullScreen()
|
overridevirtual |
Request toggling the fullscreen state of the task at the given index.
- Parameters
-
index An index in this window tasks model.
Reimplemented from TaskManager::AbstractTasksModel.
Definition at line 941 of file xwindowtasksmodel.cpp.
◆ requestToggleKeepAbove()
|
overridevirtual |
Request toggling the keep-above state of the task at the given index.
- Parameters
-
index An index in this window tasks model.
Reimplemented from TaskManager::AbstractTasksModel.
Definition at line 905 of file xwindowtasksmodel.cpp.
◆ requestToggleKeepBelow()
|
overridevirtual |
Request toggling the keep-below state of the task at the given index.
- Parameters
-
index An index in this window tasks model.
Reimplemented from TaskManager::AbstractTasksModel.
Definition at line 923 of file xwindowtasksmodel.cpp.
◆ requestToggleMaximized()
|
overridevirtual |
Request toggling the maximized state of the task at the given index.
If the window is not on the current desktop, the current desktop will be set to the window's desktop. FIXME: Desktop logic should maybe move into proxy.
- Parameters
-
index An index in this window tasks model.
Reimplemented from TaskManager::AbstractTasksModel.
Definition at line 872 of file xwindowtasksmodel.cpp.
◆ requestToggleMinimized()
|
overridevirtual |
Request toggling the minimized state of the window at the given index.
If the window is not on the current desktop, the current desktop will be set to the window's desktop. FIXME: Desktop logic should maybe move into proxy.
- Parameters
-
index An index in this window tasks model.
Reimplemented from TaskManager::AbstractTasksModel.
Definition at line 845 of file xwindowtasksmodel.cpp.
◆ requestToggleNoBorder()
|
overridevirtual |
Request toggling the no border state of the task at given index.
This X11 implementation does nothing.
- Parameters
-
index An index in this tasks model.
- Since
- 6.4
Reimplemented from TaskManager::AbstractTasksModel.
Definition at line 977 of file xwindowtasksmodel.cpp.
◆ requestToggleShaded()
|
overridevirtual |
Request toggling the shaded state of the task at the given index.
- Parameters
-
index An index in this window tasks model.
Reimplemented from TaskManager::AbstractTasksModel.
Definition at line 959 of file xwindowtasksmodel.cpp.
◆ requestVirtualDesktops()
|
overridevirtual |
Request entering the window at the given index on the specified virtual desktop.
For compatibility across windowing systems the library supports, the desktops parameter is a list; however, on X11 a window can only be on one or all virtual desktops. Therefore, only the first list entry is actually used.
An empty list has a special meaning: The window is entered on all virtual desktops in the session.
The id 0 has a special meaning: The window is entered on all virtual desktops in the session.
- Parameters
-
index An index in this window tasks model. desktops A list of virtual desktop ids (uint).
Reimplemented from TaskManager::AbstractTasksModel.
Definition at line 983 of file xwindowtasksmodel.cpp.
◆ rowCount()
|
overridevirtual |
Implements QAbstractItemModel.
Definition at line 727 of file xwindowtasksmodel.cpp.
◆ winIdFromMimeData()
|
static |
Tries to extract a X11 window id from supplied mime data.
- Parameters
-
mimeData Some mime data.
ok Set to true or false on success or failure.
Definition at line 1088 of file xwindowtasksmodel.cpp.
◆ winIdsFromMimeData()
|
static |
Tries to extract X11 window ids from supplied mime data.
- Parameters
-
mimeData Some mime data.
ok Set to true or false on success or failure.
Definition at line 1115 of file xwindowtasksmodel.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:59:37 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.