KCompletionBase
#include <KCompletionBase>
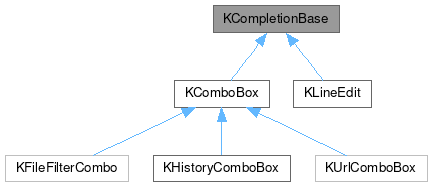
Public Types | |
typedef QMap< KeyBindingType, QList< QKeySequence > > | KeyBindingMap |
enum | KeyBindingType { TextCompletion , PrevCompletionMatch , NextCompletionMatch , SubstringCompletion } |
Public Member Functions | |
KCompletionBase () | |
virtual | ~KCompletionBase () |
KCompletion::CompletionMode | completionMode () const |
KCompletion * | completionObject (bool handleSignals=true) |
KCompletion * | compObj () const |
bool | emitSignals () const |
bool | handleSignals () const |
bool | isCompletionObjectAutoDeleted () const |
QList< QKeySequence > | keyBinding (KeyBindingType item) const |
void | setAutoDeleteCompletionObject (bool autoDelete) |
virtual void | setCompletedItems (const QStringList &items, bool autoSuggest=true)=0 |
virtual void | setCompletedText (const QString &text)=0 |
virtual void | setCompletionMode (KCompletion::CompletionMode mode) |
virtual void | setCompletionObject (KCompletion *completionObject, bool handleSignals=true) |
void | setEmitSignals (bool emitRotationSignals) |
void | setEnableSignals (bool enable) |
virtual void | setHandleSignals (bool handle) |
bool | setKeyBinding (KeyBindingType item, const QList< QKeySequence > &key) |
void | useGlobalKeyBindings () |
Protected Member Functions | |
KCompletionBase * | delegate () const |
KeyBindingMap | keyBindingMap () const |
void | setDelegate (KCompletionBase *delegate) |
void | setKeyBindingMap (KeyBindingMap keyBindingMap) |
virtual void | virtual_hook (int id, void *data) |
Detailed Description
An abstract base class for adding a completion feature into widgets.
This is a convenience class that provides the basic functions needed to add text completion support into widgets. All that is required is an implementation for the pure virtual function setCompletedText(). Refer to KLineEdit or KComboBox to see how easily such support can be added using this as a base class.
An abstract class for adding text completion support to widgets.
Definition at line 36 of file kcompletionbase.h.
Member Typedef Documentation
◆ KeyBindingMap
Definition at line 65 of file kcompletionbase.h.
Member Enumeration Documentation
◆ KeyBindingType
Constants that represent the items whose shortcut key binding is programmable.
The default key bindings for these items are defined in KStandardShortcut.
Definition at line 45 of file kcompletionbase.h.
Constructor & Destructor Documentation
◆ KCompletionBase()
KCompletionBase::KCompletionBase | ( | ) |
Default constructor.
Definition at line 59 of file kcompletionbase.cpp.
◆ ~KCompletionBase()
|
virtual |
Destructor.
Definition at line 66 of file kcompletionbase.cpp.
Member Function Documentation
◆ completionMode()
KCompletion::CompletionMode KCompletionBase::completionMode | ( | ) | const |
Returns the current completion mode.
- Returns
- the completion mode.
Definition at line 201 of file kcompletionbase.cpp.
◆ completionObject()
KCompletion * KCompletionBase::completionObject | ( | bool | handleSignals = true | ) |
Returns a pointer to the current completion object.
If the completion object does not exist, it is automatically created and by default handles all the completion signals internally unless handleSignals
is set to false. It is also automatically destroyed when the destructor is called. You can change this default behavior using the setAutoDeleteCompletionObject and setHandleSignals member functions.
See also compObj.
- Parameters
-
handleSignals if true, handles completion signals internally.
- Returns
- a pointer to the completion object.
Definition at line 90 of file kcompletionbase.cpp.
◆ compObj()
KCompletion * KCompletionBase::compObj | ( | ) | const |
Returns a pointer to the completion object.
This method is only different from completionObject() in that it does not create a new KCompletion object even if the internal pointer is NULL
. Use this method to get the pointer to a completion object when inheriting so that you will not inadvertently create it.
- Returns
- the completion object or
NULL
if one does not exist.
Definition at line 246 of file kcompletionbase.cpp.
◆ delegate()
|
protected |
Returns the delegation object.
- Returns
- the delegation object, or
nullptr
if there is none
- See also
- setDelegate()
Definition at line 84 of file kcompletionbase.cpp.
◆ emitSignals()
bool KCompletionBase::emitSignals | ( | ) | const |
Returns true if the object emits the signals.
- Returns
- true if signals are emitted
Definition at line 169 of file kcompletionbase.cpp.
◆ handleSignals()
bool KCompletionBase::handleSignals | ( | ) | const |
Returns true if the object handles the signals.
- Returns
- true if this signals are handled internally.
Definition at line 163 of file kcompletionbase.cpp.
◆ isCompletionObjectAutoDeleted()
bool KCompletionBase::isCompletionObjectAutoDeleted | ( | ) | const |
Returns true if the completion object is deleted upon this widget's destruction.
See setCompletionObject() and enableCompletion() for details.
- Returns
- true if the completion object will be deleted automatically
Definition at line 137 of file kcompletionbase.cpp.
◆ keyBinding()
QList< QKeySequence > KCompletionBase::keyBinding | ( | KeyBindingType | item | ) | const |
Returns the key binding used for the specified item.
This method returns the key binding used to activate the feature given by item
. If the binding contains modifier key(s), the sum of the modifier key and the actual key code is returned.
- Parameters
-
item the item to check
- Returns
- the key binding used for the feature given by
item
.
- See also
- setKeyBinding
- Since
- 5.0
Definition at line 225 of file kcompletionbase.cpp.
◆ keyBindingMap()
|
protected |
Returns a key binding map.
This method is the same as getKeyBinding(), except that it returns the whole keymap containing the key bindings.
- Returns
- the key binding used for the feature given by
item
.
- Since
- 5.0
Definition at line 252 of file kcompletionbase.cpp.
◆ setAutoDeleteCompletionObject()
void KCompletionBase::setAutoDeleteCompletionObject | ( | bool | autoDelete | ) |
Sets the completion object when this widget's destructor is called.
If the argument is set to true, the completion object is deleted when this widget's destructor is called.
- Parameters
-
autoDelete if true, delete completion object on destruction.
Definition at line 143 of file kcompletionbase.cpp.
◆ setCompletedItems()
|
pure virtual |
A pure virtual function that must be implemented by all inheriting classes.
- Parameters
-
items the list of completed items autoSuggest if true
, the first element ofitems
is automatically completed (i.e. preselected).
◆ setCompletedText()
|
pure virtual |
A pure virtual function that must be implemented by all inheriting classes.
This function is intended to allow external completion implementations to set completed text appropriately. It is mostly relevant when the completion mode is set to CompletionAuto and CompletionManual modes. See KCompletionBase::setCompletedText. Does nothing in CompletionPopup mode, as all available matches will be shown in the popup.
- Parameters
-
text the completed text to be set in the widget.
◆ setCompletionMode()
|
virtual |
Sets the type of completion to be used.
- Parameters
-
mode Completion type
- See also
- CompletionMode
Reimplemented in KLineEdit.
Definition at line 185 of file kcompletionbase.cpp.
◆ setCompletionObject()
|
virtual |
Sets up the completion object to be used.
This method assigns the completion object and sets it up to automatically handle the completion and rotation signals internally. You should use this function if you want to share one completion object among your widgets or need to use a customized completion object.
The object assigned through this method is not deleted when this object's destructor is invoked unless you explicitly call setAutoDeleteCompletionObject after calling this method. Be sure to set the bool argument to false, if you want to handle the completion signals yourself.
- Parameters
-
completionObject a KCompletion or a derived child object. handleCompletionSignals if true, handles completion signals internally.
Reimplemented in KLineEdit, and KUrlComboBox.
Definition at line 104 of file kcompletionbase.cpp.
◆ setDelegate()
|
protected |
Sets or removes the delegation object.
If a delegation object is set, all function calls will be forwarded to the delegation object.
- Parameters
-
delegate the delegation object, or nullptr
to remove it
Definition at line 70 of file kcompletionbase.cpp.
◆ setEmitSignals()
void KCompletionBase::setEmitSignals | ( | bool | emitRotationSignals | ) |
Sets whether the object emits rotation signals.
- Parameters
-
emitRotationSignals if false, disables the emission of rotation signals.
Definition at line 175 of file kcompletionbase.cpp.
◆ setEnableSignals()
void KCompletionBase::setEnableSignals | ( | bool | enable | ) |
Sets the widget's ability to emit text completion and rotation signals.
Invoking this function with enable
set to false
will cause the completion and rotation signals not to be emitted. However, unlike setting the completion object to nullptr
using setCompletionObject, disabling the emission of the signals through this method does not affect the current completion object.
There is no need to invoke this function by default. When a completion object is created through completionObject or setCompletionObject, these signals are set to emit automatically. Also note that disabling this signals will not necessarily interfere with the objects' ability to handle these events internally. See setHandleSignals.
- Parameters
-
enable if false, disables the emission of completion and rotation signals.
Definition at line 153 of file kcompletionbase.cpp.
◆ setHandleSignals()
|
virtual |
Enables this object to handle completion and rotation events internally.
This function simply assigns a boolean value that indicates whether it should handle rotation and completion events or not. Note that this does not stop the object from emitting signals when these events occur.
- Parameters
-
handle if true, it handles completion and rotation internally.
Definition at line 127 of file kcompletionbase.cpp.
◆ setKeyBinding()
bool KCompletionBase::setKeyBinding | ( | KeyBindingType | item, |
const QList< QKeySequence > & | key ) |
Sets the key binding to be used for manual text completion, text rotation in a history list as well as a completion list.
When the keys set by this function are pressed, a signal defined by the inheriting widget will be activated. If the default value or 0 is specified by the second parameter, then the key binding as defined in the global setting should be used. This method returns false when key
is negative or the supplied key binding conflicts with another one set for another feature.
NOTE: To use a modifier key (Shift, Ctrl, Alt) as part of the key binding simply sum
up the values of the modifier and the actual key. For example, to use CTRL+E, supply "Qt::CtrlButton | Qt::Key_E"
as the second argument to this function.
- Parameters
-
item the feature whose key binding needs to be set: - TextCompletion the manual completion key binding.
- PrevCompletionMatch the previous match key for multiple completion.
- NextCompletionMatch the next match key for for multiple completion.
- SubstringCompletion the key for substring completion
key key binding used to rotate down in a list.
- Returns
- true if key binding is successfully set.
- See also
- keyBinding
Definition at line 207 of file kcompletionbase.cpp.
◆ setKeyBindingMap()
|
protected |
◆ useGlobalKeyBindings()
void KCompletionBase::useGlobalKeyBindings | ( | ) |
Sets this object to use global values for key bindings.
This method changes the values of the key bindings for rotation and completion features to the default values provided in KGlobalSettings.
NOTE: By default, inheriting widgets should use the global key bindings so that there is no need to call this method.
Definition at line 231 of file kcompletionbase.cpp.
◆ virtual_hook()
|
protectedvirtual |
Virtual hook, used to add new "virtual" functions while maintaining binary compatibility.
Unused in this class.
Definition at line 269 of file kcompletionbase.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:57:19 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.