KConfigSkeletonItem
#include <KCoreConfigSkeleton>
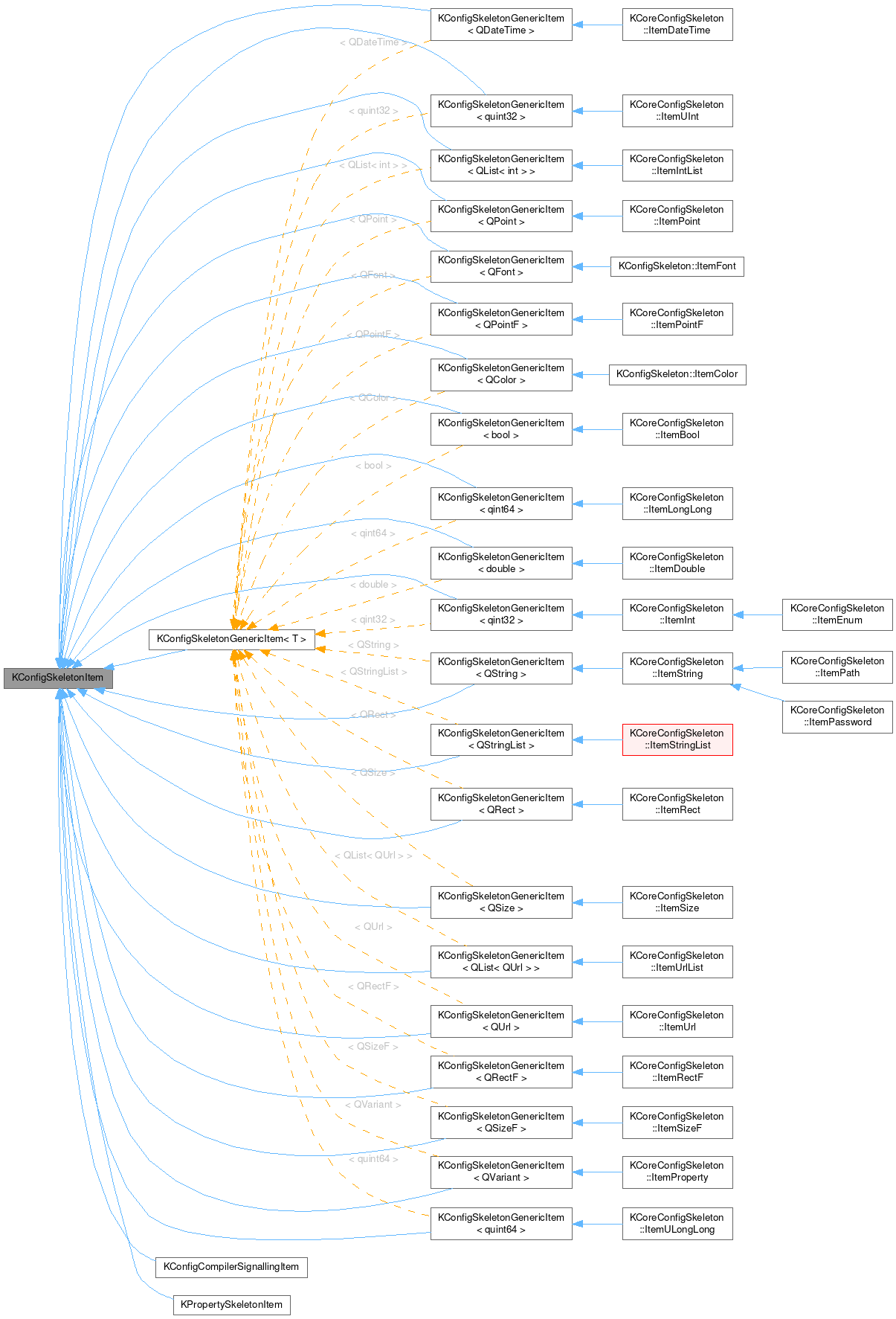
Public Types | |
typedef QHash< QString, KConfigSkeletonItem * > | Dict |
typedef QHash< QString, KConfigSkeletonItem * >::Iterator | DictIterator |
typedef QList< KConfigSkeletonItem * > | List |
Public Member Functions | |
KConfigSkeletonItem (const QString &_group, const QString &_key) | |
virtual | ~KConfigSkeletonItem () |
KConfigGroup | configGroup (KConfig *config) const |
QVariant | getDefault () const |
QString | group () const |
bool | isDefault () const |
virtual bool | isEqual (const QVariant &p) const =0 |
bool | isImmutable () const |
bool | isSaveNeeded () const |
QString | key () const |
QString | label () const |
virtual QVariant | maxValue () const |
virtual QVariant | minValue () const |
QString | name () const |
virtual QVariant | property () const =0 |
virtual void | readConfig (KConfig *)=0 |
virtual void | readDefault (KConfig *)=0 |
virtual void | setDefault ()=0 |
void | setGroup (const KConfigGroup &cg) |
void | setGroup (const QString &_group) |
void | setKey (const QString &_key) |
void | setLabel (const QString &l) |
void | setName (const QString &_name) |
virtual void | setProperty (const QVariant &p)=0 |
void | setToolTip (const QString &t) |
void | setWhatsThis (const QString &w) |
void | setWriteFlags (KConfigBase::WriteConfigFlags flags) |
virtual void | swapDefault ()=0 |
QString | toolTip () const |
QString | whatsThis () const |
virtual void | writeConfig (KConfig *)=0 |
KConfigBase::WriteConfigFlags | writeFlags () const |
Protected Member Functions | |
KCONFIGCORE_NO_EXPORT | KConfigSkeletonItem (KConfigSkeletonItemPrivate &dd, const QString &_group, const QString &_key) |
void | readImmutability (const KConfigGroup &group) |
void | setGetDefaultImpl (const std::function< QVariant()> &impl) |
void | setIsDefaultImpl (const std::function< bool()> &impl) |
void | setIsSaveNeededImpl (const std::function< bool()> &impl) |
Protected Attributes | |
KConfigSkeletonItemPrivate *const | d_ptr |
QString | mGroup |
QString | mKey |
QString | mName |
Detailed Description
Class for storing a preferences setting.
- See also
- KCoreConfigSkeleton
This class represents one preferences setting as used by KCoreConfigSkeleton. Subclasses of KConfigSkeletonItem implement storage functions for a certain type of setting. Normally you don't have to use this class directly. Use the special addItem() functions of KCoreConfigSkeleton instead. If you subclass this class you will have to register instances with the function KCoreConfigSkeleton::addItem().
Definition at line 41 of file kcoreconfigskeleton.h.
Member Typedef Documentation
◆ Dict
typedef QHash<QString, KConfigSkeletonItem *> KConfigSkeletonItem::Dict |
Definition at line 46 of file kcoreconfigskeleton.h.
◆ DictIterator
typedef QHash<QString,KConfigSkeletonItem*>::Iterator KConfigSkeletonItem::DictIterator |
Definition at line 47 of file kcoreconfigskeleton.h.
◆ List
typedef QList<KConfigSkeletonItem *> KConfigSkeletonItem::List |
Definition at line 45 of file kcoreconfigskeleton.h.
Constructor & Destructor Documentation
◆ KConfigSkeletonItem() [1/2]
Constructor.
- Parameters
-
_group Config file group. _key Config file key.
Definition at line 31 of file kcoreconfigskeleton.cpp.
◆ ~KConfigSkeletonItem()
|
virtual |
Destructor.
Definition at line 45 of file kcoreconfigskeleton.cpp.
◆ KConfigSkeletonItem() [2/2]
|
explicitprotected |
Definition at line 38 of file kcoreconfigskeleton.cpp.
Member Function Documentation
◆ configGroup()
KConfigGroup KConfigSkeletonItem::configGroup | ( | KConfig * | config | ) | const |
Return a KConfigGroup, the one provided by setGroup(const KConfigGroup&) if it's valid, or make one from config
and item's group.
- See also
- setGroup(const QString &_group)
- setGroup(KConfigGroup cg)
- Since
- 5.68
Definition at line 61 of file kcoreconfigskeleton.cpp.
◆ getDefault()
QVariant KConfigSkeletonItem::getDefault | ( | ) | const |
◆ group()
QString KConfigSkeletonItem::group | ( | ) | const |
Return name of config file group.
Definition at line 70 of file kcoreconfigskeleton.cpp.
◆ isDefault()
bool KConfigSkeletonItem::isDefault | ( | ) | const |
Indicates if the item is set to its default value.
- Since
- 5.64
Definition at line 159 of file kcoreconfigskeleton.cpp.
◆ isEqual()
|
pure virtual |
Check whether the item is equal to p
.
Use this function to compare items that use custom types, because QVariant::operator== will not work for those.
- Parameters
-
p QVariant to compare to
- Returns
true
if the item is equal top
,false
otherwise
Implemented in KPropertySkeletonItem, KConfigCompilerSignallingItem, KCoreConfigSkeleton::ItemString, KCoreConfigSkeleton::ItemUrl, KCoreConfigSkeleton::ItemProperty, KCoreConfigSkeleton::ItemBool, KCoreConfigSkeleton::ItemInt, KCoreConfigSkeleton::ItemLongLong, KCoreConfigSkeleton::ItemUInt, KCoreConfigSkeleton::ItemULongLong, KCoreConfigSkeleton::ItemDouble, KCoreConfigSkeleton::ItemRect, KCoreConfigSkeleton::ItemRectF, KCoreConfigSkeleton::ItemPoint, KCoreConfigSkeleton::ItemPointF, KCoreConfigSkeleton::ItemSize, KCoreConfigSkeleton::ItemSizeF, KCoreConfigSkeleton::ItemDateTime, KCoreConfigSkeleton::ItemStringList, KCoreConfigSkeleton::ItemUrlList, KCoreConfigSkeleton::ItemIntList, KConfigSkeleton::ItemColor, and KConfigSkeleton::ItemFont.
◆ isImmutable()
bool KConfigSkeletonItem::isImmutable | ( | ) | const |
Return if the entry can be modified.
Definition at line 153 of file kcoreconfigskeleton.cpp.
◆ isSaveNeeded()
bool KConfigSkeletonItem::isSaveNeeded | ( | ) | const |
Indicates if the item has a different value than the previously loaded value.
- Since
- 5.64
Definition at line 165 of file kcoreconfigskeleton.cpp.
◆ key()
QString KConfigSkeletonItem::key | ( | ) | const |
Return config file key.
Definition at line 80 of file kcoreconfigskeleton.cpp.
◆ label()
QString KConfigSkeletonItem::label | ( | ) | const |
Return the label of the item.
- See also
- setLabel()
Definition at line 101 of file kcoreconfigskeleton.cpp.
◆ maxValue()
|
virtual |
Return maximum value of item or invalid if not specified.
Reimplemented in KConfigCompilerSignallingItem, KCoreConfigSkeleton::ItemInt, KCoreConfigSkeleton::ItemLongLong, KCoreConfigSkeleton::ItemUInt, KCoreConfigSkeleton::ItemULongLong, and KCoreConfigSkeleton::ItemDouble.
Definition at line 148 of file kcoreconfigskeleton.cpp.
◆ minValue()
|
virtual |
Return minimum value of item or invalid if not specified.
Reimplemented in KConfigCompilerSignallingItem, KCoreConfigSkeleton::ItemInt, KCoreConfigSkeleton::ItemLongLong, KCoreConfigSkeleton::ItemUInt, KCoreConfigSkeleton::ItemULongLong, and KCoreConfigSkeleton::ItemDouble.
Definition at line 143 of file kcoreconfigskeleton.cpp.
◆ name()
QString KConfigSkeletonItem::name | ( | ) | const |
Return internal name of entry.
Definition at line 90 of file kcoreconfigskeleton.cpp.
◆ property()
|
pure virtual |
Return item as property.
Implemented in KPropertySkeletonItem, KConfigCompilerSignallingItem, KCoreConfigSkeleton::ItemString, KCoreConfigSkeleton::ItemUrl, KCoreConfigSkeleton::ItemProperty, KCoreConfigSkeleton::ItemBool, KCoreConfigSkeleton::ItemInt, KCoreConfigSkeleton::ItemLongLong, KCoreConfigSkeleton::ItemUInt, KCoreConfigSkeleton::ItemULongLong, KCoreConfigSkeleton::ItemDouble, KCoreConfigSkeleton::ItemRect, KCoreConfigSkeleton::ItemRectF, KCoreConfigSkeleton::ItemPoint, KCoreConfigSkeleton::ItemPointF, KCoreConfigSkeleton::ItemSize, KCoreConfigSkeleton::ItemSizeF, KCoreConfigSkeleton::ItemDateTime, KCoreConfigSkeleton::ItemStringList, KCoreConfigSkeleton::ItemUrlList, KCoreConfigSkeleton::ItemIntList, KConfigSkeleton::ItemColor, and KConfigSkeleton::ItemFont.
◆ readConfig()
|
pure virtual |
This function is called by KCoreConfigSkeleton to read the value for this setting from a config file.
Implemented in KPropertySkeletonItem, KConfigCompilerSignallingItem, KCoreConfigSkeleton::ItemString, KCoreConfigSkeleton::ItemUrl, KCoreConfigSkeleton::ItemProperty, KCoreConfigSkeleton::ItemBool, KCoreConfigSkeleton::ItemInt, KCoreConfigSkeleton::ItemLongLong, KCoreConfigSkeleton::ItemEnum, KCoreConfigSkeleton::ItemUInt, KCoreConfigSkeleton::ItemULongLong, KCoreConfigSkeleton::ItemDouble, KCoreConfigSkeleton::ItemRect, KCoreConfigSkeleton::ItemRectF, KCoreConfigSkeleton::ItemPoint, KCoreConfigSkeleton::ItemPointF, KCoreConfigSkeleton::ItemSize, KCoreConfigSkeleton::ItemSizeF, KCoreConfigSkeleton::ItemDateTime, KCoreConfigSkeleton::ItemStringList, KCoreConfigSkeleton::ItemPathList, KCoreConfigSkeleton::ItemUrlList, KCoreConfigSkeleton::ItemIntList, KConfigSkeleton::ItemColor, and KConfigSkeleton::ItemFont.
◆ readDefault()
|
pure virtual |
Read global default value.
Implemented in KPropertySkeletonItem, KConfigCompilerSignallingItem, KConfigSkeletonGenericItem< T >, KConfigSkeletonGenericItem< bool >, KConfigSkeletonGenericItem< double >, KConfigSkeletonGenericItem< QColor >, KConfigSkeletonGenericItem< QDateTime >, KConfigSkeletonGenericItem< QFont >, KConfigSkeletonGenericItem< qint32 >, KConfigSkeletonGenericItem< qint64 >, KConfigSkeletonGenericItem< QList< int > >, KConfigSkeletonGenericItem< QList< QUrl > >, KConfigSkeletonGenericItem< QPoint >, KConfigSkeletonGenericItem< QPointF >, KConfigSkeletonGenericItem< QRect >, KConfigSkeletonGenericItem< QRectF >, KConfigSkeletonGenericItem< QSize >, KConfigSkeletonGenericItem< QSizeF >, KConfigSkeletonGenericItem< QString >, KConfigSkeletonGenericItem< QStringList >, KConfigSkeletonGenericItem< quint32 >, KConfigSkeletonGenericItem< quint64 >, KConfigSkeletonGenericItem< QUrl >, and KConfigSkeletonGenericItem< QVariant >.
◆ readImmutability()
|
protected |
Sets mIsImmutable to true
if mKey in config is immutable.
- Parameters
-
group KConfigGroup to check if mKey is immutable in
Definition at line 177 of file kcoreconfigskeleton.cpp.
◆ setDefault()
|
pure virtual |
Sets the current value to the default value.
Implemented in KPropertySkeletonItem, KConfigSkeletonGenericItem< T >, KConfigSkeletonGenericItem< bool >, KConfigSkeletonGenericItem< double >, KConfigSkeletonGenericItem< QColor >, KConfigSkeletonGenericItem< QDateTime >, KConfigSkeletonGenericItem< QFont >, KConfigSkeletonGenericItem< qint32 >, KConfigSkeletonGenericItem< qint64 >, KConfigSkeletonGenericItem< QList< int > >, KConfigSkeletonGenericItem< QList< QUrl > >, KConfigSkeletonGenericItem< QPoint >, KConfigSkeletonGenericItem< QPointF >, KConfigSkeletonGenericItem< QRect >, KConfigSkeletonGenericItem< QRectF >, KConfigSkeletonGenericItem< QSize >, KConfigSkeletonGenericItem< QSizeF >, KConfigSkeletonGenericItem< QString >, KConfigSkeletonGenericItem< QStringList >, KConfigSkeletonGenericItem< quint32 >, KConfigSkeletonGenericItem< quint64 >, KConfigSkeletonGenericItem< QUrl >, KConfigSkeletonGenericItem< QVariant >, and KConfigCompilerSignallingItem.
◆ setGetDefaultImpl()
|
protected |
Definition at line 195 of file kcoreconfigskeleton.cpp.
◆ setGroup() [1/2]
void KConfigSkeletonItem::setGroup | ( | const KConfigGroup & | cg | ) |
Set config file group but giving the KConfigGroup.
Allow the item to be in nested groups.
- Since
- 5.68
Definition at line 55 of file kcoreconfigskeleton.cpp.
◆ setGroup() [2/2]
void KConfigSkeletonItem::setGroup | ( | const QString & | _group | ) |
Set config file group.
Definition at line 50 of file kcoreconfigskeleton.cpp.
◆ setIsDefaultImpl()
|
protected |
Definition at line 183 of file kcoreconfigskeleton.cpp.
◆ setIsSaveNeededImpl()
|
protected |
Definition at line 189 of file kcoreconfigskeleton.cpp.
◆ setKey()
void KConfigSkeletonItem::setKey | ( | const QString & | _key | ) |
Set config file key.
Definition at line 75 of file kcoreconfigskeleton.cpp.
◆ setLabel()
void KConfigSkeletonItem::setLabel | ( | const QString & | l | ) |
Set label providing a translated one-line description of the item.
Definition at line 95 of file kcoreconfigskeleton.cpp.
◆ setName()
void KConfigSkeletonItem::setName | ( | const QString & | _name | ) |
Set internal name of entry.
Definition at line 85 of file kcoreconfigskeleton.cpp.
◆ setProperty()
|
pure virtual |
Set item to p
.
Implemented in KPropertySkeletonItem, KConfigCompilerSignallingItem, KCoreConfigSkeleton::ItemString, KCoreConfigSkeleton::ItemUrl, KCoreConfigSkeleton::ItemProperty, KCoreConfigSkeleton::ItemBool, KCoreConfigSkeleton::ItemInt, KCoreConfigSkeleton::ItemLongLong, KCoreConfigSkeleton::ItemUInt, KCoreConfigSkeleton::ItemULongLong, KCoreConfigSkeleton::ItemDouble, KCoreConfigSkeleton::ItemRect, KCoreConfigSkeleton::ItemRectF, KCoreConfigSkeleton::ItemPoint, KCoreConfigSkeleton::ItemPointF, KCoreConfigSkeleton::ItemSize, KCoreConfigSkeleton::ItemSizeF, KCoreConfigSkeleton::ItemDateTime, KCoreConfigSkeleton::ItemStringList, KCoreConfigSkeleton::ItemUrlList, KCoreConfigSkeleton::ItemIntList, KConfigSkeleton::ItemColor, and KConfigSkeleton::ItemFont.
◆ setToolTip()
void KConfigSkeletonItem::setToolTip | ( | const QString & | t | ) |
◆ setWhatsThis()
void KConfigSkeletonItem::setWhatsThis | ( | const QString & | w | ) |
Set WhatsThis description of item.
Definition at line 119 of file kcoreconfigskeleton.cpp.
◆ setWriteFlags()
void KConfigSkeletonItem::setWriteFlags | ( | KConfigBase::WriteConfigFlags | flags | ) |
The write flags to be used when writing configuration.
- Since
- 5.58
Definition at line 131 of file kcoreconfigskeleton.cpp.
◆ swapDefault()
|
pure virtual |
Exchanges the current value with the default value Used by KCoreConfigSkeleton::useDefaults(bool);.
Implemented in KPropertySkeletonItem, KConfigSkeletonGenericItem< T >, KConfigSkeletonGenericItem< bool >, KConfigSkeletonGenericItem< double >, KConfigSkeletonGenericItem< QColor >, KConfigSkeletonGenericItem< QDateTime >, KConfigSkeletonGenericItem< QFont >, KConfigSkeletonGenericItem< qint32 >, KConfigSkeletonGenericItem< qint64 >, KConfigSkeletonGenericItem< QList< int > >, KConfigSkeletonGenericItem< QList< QUrl > >, KConfigSkeletonGenericItem< QPoint >, KConfigSkeletonGenericItem< QPointF >, KConfigSkeletonGenericItem< QRect >, KConfigSkeletonGenericItem< QRectF >, KConfigSkeletonGenericItem< QSize >, KConfigSkeletonGenericItem< QSizeF >, KConfigSkeletonGenericItem< QString >, KConfigSkeletonGenericItem< QStringList >, KConfigSkeletonGenericItem< quint32 >, KConfigSkeletonGenericItem< quint64 >, KConfigSkeletonGenericItem< QUrl >, KConfigSkeletonGenericItem< QVariant >, and KConfigCompilerSignallingItem.
◆ toolTip()
QString KConfigSkeletonItem::toolTip | ( | ) | const |
Return ToolTip description of item.
- See also
- setToolTip()
- Since
- 4.2
Definition at line 113 of file kcoreconfigskeleton.cpp.
◆ whatsThis()
QString KConfigSkeletonItem::whatsThis | ( | ) | const |
Return WhatsThis description of item.
- See also
- setWhatsThis()
Definition at line 125 of file kcoreconfigskeleton.cpp.
◆ writeConfig()
|
pure virtual |
This function is called by KCoreConfigSkeleton to write the value of this setting to a config file.
Make sure to pass writeFlags() to every call of KConfigGroup::writeEntry() and KConfigGroup::revertToDefault().
Implemented in KPropertySkeletonItem, KConfigCompilerSignallingItem, KConfigSkeletonGenericItem< T >, KConfigSkeletonGenericItem< bool >, KConfigSkeletonGenericItem< double >, KConfigSkeletonGenericItem< QColor >, KConfigSkeletonGenericItem< QDateTime >, KConfigSkeletonGenericItem< QFont >, KConfigSkeletonGenericItem< qint32 >, KConfigSkeletonGenericItem< qint64 >, KConfigSkeletonGenericItem< QList< int > >, KConfigSkeletonGenericItem< QList< QUrl > >, KConfigSkeletonGenericItem< QPoint >, KConfigSkeletonGenericItem< QPointF >, KConfigSkeletonGenericItem< QRect >, KConfigSkeletonGenericItem< QRectF >, KConfigSkeletonGenericItem< QSize >, KConfigSkeletonGenericItem< QSizeF >, KConfigSkeletonGenericItem< QString >, KConfigSkeletonGenericItem< QStringList >, KConfigSkeletonGenericItem< quint32 >, KConfigSkeletonGenericItem< quint64 >, KConfigSkeletonGenericItem< QUrl >, KConfigSkeletonGenericItem< QVariant >, KCoreConfigSkeleton::ItemString, KCoreConfigSkeleton::ItemUrl, KCoreConfigSkeleton::ItemEnum, KCoreConfigSkeleton::ItemPathList, and KCoreConfigSkeleton::ItemUrlList.
◆ writeFlags()
KConfigBase::WriteConfigFlags KConfigSkeletonItem::writeFlags | ( | ) | const |
Return write flags to be used when writing configuration.
They should be passed to every call of KConfigGroup::writeEntry() and KConfigGroup::revertToDefault().
- Since
- 5.58
Definition at line 137 of file kcoreconfigskeleton.cpp.
Member Data Documentation
◆ d_ptr
|
protected |
Definition at line 261 of file kcoreconfigskeleton.h.
◆ mGroup
|
protected |
The group name for this item.
Definition at line 251 of file kcoreconfigskeleton.h.
◆ mKey
|
protected |
The config key for this item.
Definition at line 252 of file kcoreconfigskeleton.h.
◆ mName
|
protected |
The name of this item.
Definition at line 253 of file kcoreconfigskeleton.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Tue Mar 26 2024 11:20:28 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.