BluezQt::ObexTransfer
#include <BluezQt/ObexTransfer>
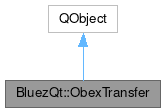
Public Types | |
enum | Status { Queued , Active , Suspended , Complete , Error , Unknown } |
Properties | |
QString | fileName |
QString | name |
quint64 | size |
Status | status |
bool | suspendable |
quint64 | time |
quint64 | transferred |
QString | type |
![]() | |
objectName | |
Signals | |
void | fileNameChanged (const QString &fileName) |
void | statusChanged (Status status) |
void | transferredChanged (quint64 transferred) |
Public Member Functions | |
~ObexTransfer () override | |
PendingCall * | cancel () |
QString | fileName () const |
bool | isSuspendable () const |
QString | name () const |
QDBusObjectPath | objectPath () const |
PendingCall * | resume () |
quint64 | size () const |
Status | status () const |
PendingCall * | suspend () |
quint64 | time () const |
ObexTransferPtr | toSharedPtr () const |
quint64 | transferred () const |
QString | type () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
OBEX transfer.
This class represents transfer of one file.
Definition at line 32 of file obextransfer.h.
Member Enumeration Documentation
◆ Status
Status of transfer.
Definition at line 49 of file obextransfer.h.
Property Documentation
◆ fileName
|
read |
Definition at line 42 of file obextransfer.h.
◆ name
|
read |
Definition at line 37 of file obextransfer.h.
◆ size
|
read |
Definition at line 40 of file obextransfer.h.
◆ status
|
read |
Definition at line 36 of file obextransfer.h.
◆ suspendable
|
read |
Definition at line 43 of file obextransfer.h.
◆ time
|
read |
Definition at line 39 of file obextransfer.h.
◆ transferred
|
read |
Definition at line 41 of file obextransfer.h.
◆ type
|
read |
Definition at line 38 of file obextransfer.h.
Constructor & Destructor Documentation
◆ ~ObexTransfer()
|
overridedefault |
Destroys an ObexTransfer object.
Member Function Documentation
◆ cancel()
PendingCall * BluezQt::ObexTransfer::cancel | ( | ) |
Stops the current transfer.
Possible errors: PendingCall::NotAuthorized, PendingCall::InProgress PendingCall::Failed
- Returns
- void pending call
Definition at line 163 of file obextransfer.cpp.
◆ fileName()
QString BluezQt::ObexTransfer::fileName | ( | ) | const |
Returns the full name of the transferred file.
- Returns
- full name of transferred file
Definition at line 153 of file obextransfer.cpp.
◆ fileNameChanged
|
signal |
Indicates that the name of transferred file have changed.
◆ isSuspendable()
bool BluezQt::ObexTransfer::isSuspendable | ( | ) | const |
Returns whether the transfer is suspendable.
- Returns
- true if transfer is suspendable
Definition at line 158 of file obextransfer.cpp.
◆ name()
QString BluezQt::ObexTransfer::name | ( | ) | const |
Returns the name of the transferred object.
- Returns
- name of transferred object
Definition at line 128 of file obextransfer.cpp.
◆ objectPath()
QDBusObjectPath BluezQt::ObexTransfer::objectPath | ( | ) | const |
D-Bus object path of the transfer.
- Returns
- object path of transfer
Definition at line 118 of file obextransfer.cpp.
◆ resume()
PendingCall * BluezQt::ObexTransfer::resume | ( | ) |
Resumes the current transfer.
Possible errors: PendingCall::NotAuthorized, PendingCall::NotInProgress
- Returns
- void pending call
Definition at line 173 of file obextransfer.cpp.
◆ size()
quint64 BluezQt::ObexTransfer::size | ( | ) | const |
Returns the total size of the transferred object.
- Returns
- size of transferred object
Definition at line 143 of file obextransfer.cpp.
◆ status()
ObexTransfer::Status BluezQt::ObexTransfer::status | ( | ) | const |
Returns the status of the transfer.
- Returns
- status of transfer
Definition at line 123 of file obextransfer.cpp.
◆ statusChanged
|
signal |
Indicates that the status of transfer have changed.
◆ suspend()
PendingCall * BluezQt::ObexTransfer::suspend | ( | ) |
Suspends the current transfer.
Only suspendable transfers can be suspended.
Possible errors: PendingCall::NotAuthorized, PendingCall::NotInProgress
- See also
- isSuspendable() const
- Returns
- void pending call
Definition at line 168 of file obextransfer.cpp.
◆ time()
quint64 BluezQt::ObexTransfer::time | ( | ) | const |
Returns the time of the transferred object.
- Returns
- time of transferred object
Definition at line 138 of file obextransfer.cpp.
◆ toSharedPtr()
ObexTransferPtr BluezQt::ObexTransfer::toSharedPtr | ( | ) | const |
Returns a shared pointer from this.
- Returns
- ObexTransferPtr
Definition at line 113 of file obextransfer.cpp.
◆ transferred()
quint64 BluezQt::ObexTransfer::transferred | ( | ) | const |
Returns the number of bytes transferred.
- Returns
- number of bytes transferred
Definition at line 148 of file obextransfer.cpp.
◆ transferredChanged
|
signal |
Indicates that the number of transferred bytes have changed.
◆ type()
QString BluezQt::ObexTransfer::type | ( | ) | const |
Returns the type of the transferred object.
- Returns
- type of transferred object
Definition at line 133 of file obextransfer.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:53:30 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.