BluezQt::Profile
#include <BluezQt/Profile>
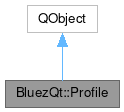
Public Types | |
enum | LocalRole { ClientRole , ServerRole } |
![]() | |
typedef | QObjectList |
Properties | |
QString | uuid |
![]() | |
objectName | |
Public Member Functions | |
Profile (QObject *parent=nullptr) | |
~Profile () override | |
QSharedPointer< QLocalSocket > | createSocket (const QDBusUnixFileDescriptor &fd) |
virtual void | newConnection (DevicePtr device, const QDBusUnixFileDescriptor &fd, const QVariantMap &properties, const Request<> &request) |
virtual QDBusObjectPath | objectPath () const =0 |
virtual void | release () |
virtual void | requestDisconnection (DevicePtr device, const Request<> &request) |
void | setAutoConnect (bool autoConnect) |
void | setChannel (quint16 channel) |
void | setFeatures (quint16 features) |
void | setLocalRole (LocalRole role) |
void | setName (const QString &name) |
void | setPsm (quint16 psm) |
void | setRequireAuthentication (bool require) |
void | setRequireAuthorization (bool require) |
void | setService (const QString &service) |
void | setServiceRecord (const QString &serviceRecord) |
void | setVersion (quint16 version) |
virtual QString | uuid () const =0 |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Bluetooth profile.
This class represents a Bluetooth profile.
It is only needed to reimplement pure virtual functions. You don't need to set any additional properties.
But you may need to specify at least channel number or PSM in case it couldn't be determined from UUID. It is also a good idea to provide name for the profile.
Setting the channel number with setChannel() will make the profile use RFCOMM, while setting the PSM with setPsm() will make the profile use L2CAP.
- Note
- The return value of requests will be sent asynchronously with Request class. It is also possible to cancel/reject all requests.
Member Enumeration Documentation
◆ LocalRole
Property Documentation
◆ uuid
Constructor & Destructor Documentation
◆ Profile()
|
explicit |
◆ ~Profile()
|
overridedefault |
Destroys a Profile object.
Member Function Documentation
◆ createSocket()
QSharedPointer< QLocalSocket > BluezQt::Profile::createSocket | ( | const QDBusUnixFileDescriptor & | fd | ) |
Creates a socket from file descriptor.
- Parameters
-
fd socket file descriptor
- Returns
- socket
- See also
- newConnection()
Definition at line 107 of file profile.cpp.
◆ newConnection()
|
virtual |
Requests the new connection.
Common properties:
To create socket from fd, you can use:
- Parameters
-
device device that requested connection fd socket file descriptor properties additional properties request request to be used for sending reply
Definition at line 115 of file profile.cpp.
◆ objectPath()
|
pure virtual |
D-Bus object path of the profile.
The path where the profile will be registered.
- Note
- You must provide valid object path!
- Returns
- object path of agent
◆ release()
|
virtual |
Indicates that the profile was unregistered.
This method gets called when the Bluetooth daemon unregisters the profile.
A profile can use it to do cleanup tasks. There is no need to unregister the profile, because when this method gets called it has already been unregistered.
Definition at line 131 of file profile.cpp.
◆ requestDisconnection()
Requests the disconnection of the profile.
This method gets called when a profile gets disconnected.
- Parameters
-
device device to be disconnected request request to be used for sending reply
Definition at line 124 of file profile.cpp.
◆ setAutoConnect()
void BluezQt::Profile::setAutoConnect | ( | bool | autoConnect | ) |
Sets whether the profile is automatically connected.
In case of a client UUID this will force connection of the RFCOMM or L2CAP channels when a remote device is connected.
- Parameters
-
autoConnect autoconnect the profile
Definition at line 87 of file profile.cpp.
◆ setChannel()
void BluezQt::Profile::setChannel | ( | quint16 | channel | ) |
Sets the RFCOMM channel number.
Available channel number range is 0-31. Setting channel number to 0 will automatically choose correct channel number for profile UUID.
- Parameters
-
channel channel number
Definition at line 62 of file profile.cpp.
◆ setFeatures()
void BluezQt::Profile::setFeatures | ( | quint16 | features | ) |
Sets the profile features.
- Parameters
-
features features of the profile
Definition at line 102 of file profile.cpp.
◆ setLocalRole()
void BluezQt::Profile::setLocalRole | ( | Profile::LocalRole | role | ) |
Sets the local role to identify side.
For asymmetric profiles that do not have UUIDs available to uniquely identify each side this parameter allows specifying the precise local role.
- Parameters
-
role local role
Definition at line 38 of file profile.cpp.
◆ setName()
void BluezQt::Profile::setName | ( | const QString & | name | ) |
Sets the human readable name of the profile.
- Parameters
-
name name of the profile
Definition at line 28 of file profile.cpp.
◆ setPsm()
void BluezQt::Profile::setPsm | ( | quint16 | psm | ) |
Sets the L2CAP port number.
PSM (Protocol Service Multiplexer) is a port number in L2CAP.
Setting PSM to 0 will automatically choose correct PSM for profile UUID.
- Parameters
-
psm PSM
Definition at line 72 of file profile.cpp.
◆ setRequireAuthentication()
void BluezQt::Profile::setRequireAuthentication | ( | bool | require | ) |
Sets whether the pairing is required to connect.
- Parameters
-
require require to pair
Definition at line 77 of file profile.cpp.
◆ setRequireAuthorization()
void BluezQt::Profile::setRequireAuthorization | ( | bool | require | ) |
Sets whether the authorization is required to connect.
- Parameters
-
require require to authorize
Definition at line 82 of file profile.cpp.
◆ setService()
void BluezQt::Profile::setService | ( | const QString & | service | ) |
Sets the primary service class UUID (if different from profile UUID).
- Parameters
-
service service UUID
Definition at line 33 of file profile.cpp.
◆ setServiceRecord()
void BluezQt::Profile::setServiceRecord | ( | const QString & | serviceRecord | ) |
Sets a SDP record.
This allows to provide a manual SDP record, otherwise it will be generated automatically.
- Parameters
-
serviceRecord SDP record
Definition at line 92 of file profile.cpp.
◆ setVersion()
void BluezQt::Profile::setVersion | ( | quint16 | version | ) |
Sets the profile version.
- Parameters
-
version version of the profile
Definition at line 97 of file profile.cpp.
◆ uuid()
|
pure virtual |
UUID of the profile.
- Returns
- UUID of the profile
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 3 2025 11:49:41 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.