KCompressionDevice
#include <KCompressionDevice>
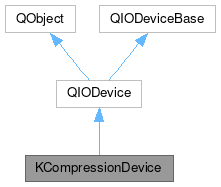
Public Types | |
enum | CompressionType { GZip , BZip2 , Xz , None , Zstd } |
![]() | |
enum | OpenModeFlag |
Public Member Functions | |
KCompressionDevice (const QString &fileName) | |
KCompressionDevice (const QString &fileName, CompressionType type) | |
KCompressionDevice (QIODevice *inputDevice, bool autoDeleteInputDevice, CompressionType type) | |
~KCompressionDevice () override | |
bool | atEnd () const override |
void | close () override |
CompressionType | compressionType () const |
QFileDevice::FileError | error () const |
bool | open (QIODevice::OpenMode mode) override |
bool | seek (qint64) override |
void | setOrigFileName (const QByteArray &fileName) |
void | setSkipHeaders () |
![]() | |
QIODevice (QObject *parent) | |
void | aboutToClose () |
virtual qint64 | bytesAvailable () const const |
virtual qint64 | bytesToWrite () const const |
void | bytesWritten (qint64 bytes) |
virtual bool | canReadLine () const const |
void | channelBytesWritten (int channel, qint64 bytes) |
void | channelReadyRead (int channel) |
void | commitTransaction () |
int | currentReadChannel () const const |
int | currentWriteChannel () const const |
QString | errorString () const const |
bool | getChar (char *c) |
bool | isOpen () const const |
bool | isReadable () const const |
virtual bool | isSequential () const const |
bool | isTextModeEnabled () const const |
bool | isTransactionStarted () const const |
bool | isWritable () const const |
virtual bool | open (QIODeviceBase::OpenMode mode) |
QIODeviceBase::OpenMode | openMode () const const |
qint64 | peek (char *data, qint64 maxSize) |
QByteArray | peek (qint64 maxSize) |
virtual qint64 | pos () const const |
bool | putChar (char c) |
qint64 | read (char *data, qint64 maxSize) |
QByteArray | read (qint64 maxSize) |
QByteArray | readAll () |
int | readChannelCount () const const |
void | readChannelFinished () |
qint64 | readLine (char *data, qint64 maxSize) |
QByteArray | readLine (qint64 maxSize) |
void | readyRead () |
virtual bool | reset () |
void | rollbackTransaction () |
void | setCurrentReadChannel (int channel) |
void | setCurrentWriteChannel (int channel) |
void | setTextModeEnabled (bool enabled) |
virtual qint64 | size () const const |
qint64 | skip (qint64 maxSize) |
void | startTransaction () |
void | ungetChar (char c) |
virtual bool | waitForBytesWritten (int msecs) |
virtual bool | waitForReadyRead (int msecs) |
qint64 | write (const char *data) |
qint64 | write (const char *data, qint64 maxSize) |
qint64 | write (const QByteArray &data) |
int | writeChannelCount () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static CompressionType | compressionTypeForMimeType (const QString &mimetype) |
static KFilterBase * | filterForCompressionType (CompressionType type) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
KFilterBase * | filterBase () |
qint64 | readData (char *data, qint64 maxlen) override |
qint64 | writeData (const char *data, qint64 len) override |
![]() | |
virtual qint64 | readLineData (char *data, qint64 maxSize) |
void | setErrorString (const QString &str) |
void | setOpenMode (QIODeviceBase::OpenMode openMode) |
virtual qint64 | skipData (qint64 maxSize) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
![]() | |
Append | |
ExistingOnly | |
NewOnly | |
NotOpen | |
typedef | OpenMode |
ReadOnly | |
ReadWrite | |
Text | |
Truncate | |
Unbuffered | |
WriteOnly | |
Detailed Description
A class for reading and writing compressed data onto a device (e.g.
file, but other usages are possible, like a buffer or a socket).
Use this class to read/write compressed files.
Definition at line 30 of file kcompressiondevice.h.
Member Enumeration Documentation
◆ CompressionType
Enumerator | |
---|---|
Zstd |
|
Definition at line 34 of file kcompressiondevice.h.
Constructor & Destructor Documentation
◆ KCompressionDevice() [1/3]
KCompressionDevice::KCompressionDevice | ( | QIODevice * | inputDevice, |
bool | autoDeleteInputDevice, | ||
CompressionType | type ) |
Constructs a KCompressionDevice for a given CompressionType (e.g.
GZip, BZip2 etc.).
- Parameters
-
inputDevice input device. autoDeleteInputDevice if true, inputDevice
will be deleted automaticallytype the CompressionType to use.
Definition at line 189 of file kcompressiondevice.cpp.
◆ KCompressionDevice() [2/3]
KCompressionDevice::KCompressionDevice | ( | const QString & | fileName, |
CompressionType | type ) |
Constructs a KCompressionDevice for a given CompressionType (e.g.
GZip, BZip2 etc.).
- Parameters
-
fileName the name of the file to filter. type the CompressionType to use.
Definition at line 200 of file kcompressiondevice.cpp.
◆ KCompressionDevice() [3/3]
|
explicit |
Constructs a KCompressionDevice for a given fileName
.
- Parameters
-
fileName the name of the file to filter.
- Since
- 5.85
Definition at line 213 of file kcompressiondevice.cpp.
◆ ~KCompressionDevice()
|
override |
Destructs the KCompressionDevice.
Calls close() if the filter device is still open.
Definition at line 218 of file kcompressiondevice.cpp.
Member Function Documentation
◆ atEnd()
|
overridevirtual |
Reimplemented from QIODevice.
Definition at line 350 of file kcompressiondevice.cpp.
◆ close()
|
overridevirtual |
Close after reading or writing.
Reimplemented from QIODevice.
Definition at line 267 of file kcompressiondevice.cpp.
◆ compressionType()
KCompressionDevice::CompressionType KCompressionDevice::compressionType | ( | ) | const |
The compression actually used by this device.
If the support for the compression requested in the constructor is not available, then the device will use None.
Definition at line 227 of file kcompressiondevice.cpp.
◆ compressionTypeForMimeType()
|
static |
Returns the compression type for the given MIME type, if possible.
Otherwise returns None. This handles simple cases like application/gzip, but also application/x-compressed-tar, and inheritance.
- Since
- 5.85
Definition at line 105 of file kcompressiondevice.cpp.
◆ error()
QFileDevice::FileError KCompressionDevice::error | ( | ) | const |
Returns the error code from the last failing operation.
This is especially useful after calling close(), which unfortunately returns void (see https://bugreports.qt.io/browse/QTBUG-70033), to see if the flushing done by close was able to write all the data to disk.
Definition at line 289 of file kcompressiondevice.cpp.
◆ filterBase()
|
protected |
Definition at line 514 of file kcompressiondevice.cpp.
◆ filterForCompressionType()
|
static |
Call this to create the appropriate filter for the CompressionType named type
.
- Parameters
-
type the type of the compression filter
- Returns
- the filter for the
type
, or 0 if not found
Definition at line 160 of file kcompressiondevice.cpp.
◆ open()
|
override |
Open for reading or writing.
Definition at line 232 of file kcompressiondevice.cpp.
◆ readData()
|
overrideprotectedvirtual |
Implements QIODevice.
Definition at line 357 of file kcompressiondevice.cpp.
◆ seek()
|
overridevirtual |
That one can be quite slow, when going back.
Use with care.
Reimplemented from QIODevice.
Definition at line 294 of file kcompressiondevice.cpp.
◆ setOrigFileName()
void KCompressionDevice::setOrigFileName | ( | const QByteArray & | fileName | ) |
For writing gzip compressed files only: set the name of the original file, to be used in the gzip header.
- Parameters
-
fileName the name of the original file
Definition at line 504 of file kcompressiondevice.cpp.
◆ setSkipHeaders()
void KCompressionDevice::setSkipHeaders | ( | ) |
Call this let this device skip the gzip headers when reading/writing.
This way KCompressionDevice (with gzip filter) can be used as a direct wrapper around zlib - this is used by KZip.
Definition at line 509 of file kcompressiondevice.cpp.
◆ writeData()
|
overrideprotectedvirtual |
Implements QIODevice.
Definition at line 431 of file kcompressiondevice.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:56:40 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.