KRecentFilesAction
#include <KRecentFilesAction>
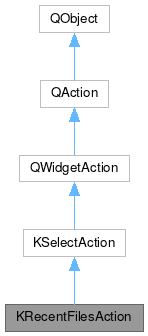
Properties | |
int | maxItems |
![]() | |
autoRepeat | |
checkable | |
checked | |
enabled | |
font | |
icon | |
iconText | |
iconVisibleInMenu | |
menuRole | |
priority | |
shortcut | |
shortcutContext | |
shortcutVisibleInContextMenu | |
statusTip | |
text | |
toolTip | |
visible | |
whatsThis | |
![]() | |
objectName | |
Signals | |
void | recentListCleared () |
void | urlSelected (const QUrl &url) |
![]() | |
void | actionTriggered (QAction *action) |
void | indexTriggered (int index) |
void | textTriggered (const QString &text) |
Public Slots | |
virtual void | clear () |
Additional Inherited Members | |
![]() | |
enum | ToolBarMode |
![]() | |
enum | ActionEvent |
enum | MenuRole |
enum | Priority |
![]() | |
typedef | QObjectList |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
ComboBoxMode | |
MenuMode | |
![]() | |
AboutQtRole | |
AboutRole | |
ApplicationSpecificRole | |
HighPriority | |
Hover | |
LowPriority | |
NormalPriority | |
NoRole | |
PreferencesRole | |
QuitRole | |
TextHeuristicRole | |
Trigger | |
![]() | |
virtual void | slotActionTriggered (QAction *action) |
void | slotToggled (bool) |
![]() | |
KWIDGETSADDONS_NO_EXPORT | KSelectAction (KSelectActionPrivate &dd, QObject *parent) |
QWidget * | createWidget (QWidget *parent) override |
void | deleteWidget (QWidget *widget) override |
![]() | |
QList< QWidget * > | createdWidgets () const const |
virtual bool | event (QEvent *event) override |
virtual bool | eventFilter (QObject *obj, QEvent *event) override |
![]() | |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Recent files action.
This class is an action to handle a recent files submenu. The best way to create the action is to use KStandardAction::openRecent. Then you simply need to call loadEntries on startup, saveEntries on shutdown, addURL when your application loads/saves a file.
Definition at line 40 of file krecentfilesaction.h.
Property Documentation
◆ maxItems
|
readwrite |
Definition at line 43 of file krecentfilesaction.h.
Constructor & Destructor Documentation
◆ KRecentFilesAction() [1/3]
|
explicit |
Constructs an action with the specified parent.
- Parameters
-
parent The parent of this action.
Definition at line 41 of file krecentfilesaction.cpp.
◆ KRecentFilesAction() [2/3]
Constructs an action with text; a shortcut may be specified by the ampersand character (e.g.
"&Option" creates a shortcut with key O )
This is the most common KAction used when you do not have a corresponding icon (note that it won't appear in the current version of the "Edit ToolBar" dialog, because an action needs an icon to be plugged in a toolbar...).
- Parameters
-
text The text that will be displayed. parent The parent of this action.
Definition at line 49 of file krecentfilesaction.cpp.
◆ KRecentFilesAction() [3/3]
KRecentFilesAction::KRecentFilesAction | ( | const QIcon & | icon, |
const QString & | text, | ||
QObject * | parent ) |
Constructs an action with text and an icon; a shortcut may be specified by the ampersand character (e.g.
"&Option" creates a shortcut with key O )
This is the other common KAction used. Use it when you do have a corresponding icon.
- Parameters
-
icon The icon to display. text The text that will be displayed. parent The parent of this action.
Definition at line 60 of file krecentfilesaction.cpp.
◆ ~KRecentFilesAction()
|
overridedefault |
Destructor.
Member Function Documentation
◆ addAction()
void KRecentFilesAction::addAction | ( | QAction * | action, |
const QUrl & | url, | ||
const QString & | name, | ||
const QMimeType & | mimeType = QMimeType() ) |
Adds action to the list of URLs, with url and title name.
Do not use addAction(QAction*), as no url will be associated, and consequently urlSelected() will not be emitted when action is selected.
Definition at line 270 of file krecentfilesaction.cpp.
◆ addUrl() [1/2]
void KRecentFilesAction::addUrl | ( | const QUrl & | url, |
const QString & | name, | ||
const QString & | mimeType ) |
Add URL to the recent files list.
This will enable this action.
- Parameters
-
url The URL of the file name The user visible pretty name that appears before the URL mimeType Specify the mimeType of the file
- Note
- URLs corresponding to local files in the temporary directory (see QDir::tempPath()) are automatically ignored by this method.
- Since
- 6.9
Definition at line 194 of file krecentfilesaction.cpp.
◆ addUrl() [2/2]
Add URL to the recent files list.
This will enable this action.
- Parameters
-
url The URL of the file name The user visible pretty name that appears before the URL
- Note
- URLs corresponding to local files in the temporary directory (see QDir::tempPath()) are automatically ignored by this method.
Definition at line 189 of file krecentfilesaction.cpp.
◆ clear
|
virtualslot |
Clears the recent files list.
Note that there is also an action shown to the user for clearing the list.
Definition at line 313 of file krecentfilesaction.cpp.
◆ loadEntries()
void KRecentFilesAction::loadEntries | ( | const KConfigGroup & | config | ) |
Loads the recent files entries from a given KConfigGroup object.
You can provide the name of the group used to load the entries. If the groupname is empty, entries are loaded from a group called 'RecentFiles'. Local file entries that do not exist anymore are not restored.
Definition at line 330 of file krecentfilesaction.cpp.
◆ maxItems()
int KRecentFilesAction::maxItems | ( | ) | const |
Returns the maximum of items in the recent files list.
Definition at line 131 of file krecentfilesaction.cpp.
◆ recentListCleared
|
signal |
This signal gets emitted when the user clear list.
So when user store url in specific config file it can saveEntry.
- Since
- 4.3
◆ removeAction()
Reimplemented for internal reasons.
Reimplemented from KSelectAction.
Definition at line 277 of file krecentfilesaction.cpp.
◆ removeUrl()
void KRecentFilesAction::removeUrl | ( | const QUrl & | url | ) |
Remove an URL from the recent files list.
- Parameters
-
url The URL of the file
Definition at line 286 of file krecentfilesaction.cpp.
◆ saveEntries()
void KRecentFilesAction::saveEntries | ( | const KConfigGroup & | config | ) |
Saves the current recent files entries to a given KConfigGroup object.
You can provide the name of the group used to load the entries. If the groupname is empty, entries are saved to a group called 'RecentFiles'.
Definition at line 389 of file krecentfilesaction.cpp.
◆ setMaxItems()
void KRecentFilesAction::setMaxItems | ( | int | maxItems | ) |
Sets the maximum of items in the recent files list.
The default for this value is 10 set in the constructor.
If this value is lesser than the number of items currently in the recent files list the last items are deleted until the number of items are equal to the new maximum.
Negative values will be normalized to 0.
Definition at line 137 of file krecentfilesaction.cpp.
◆ urls()
Retrieve a list of all URLs in the recent files list.
Definition at line 297 of file krecentfilesaction.cpp.
◆ urlSelected
|
signal |
This signal gets emitted when the user selects an URL.
- Parameters
-
url The URL that the user selected.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Dec 20 2024 11:56:38 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.