KContacts::Addressee
#include <addressee.h>
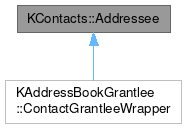
Public Types | |
typedef AddresseeList | List |
Detailed Description
address book entry
This class represents an entry in the address book.
The data of this class is implicitly shared. You can pass this class by value.
If you need the name of a field for presenting it to the user you should use the functions ending in Label(). They return a translated string which can be used as label for the corresponding field.
About the name fields:
givenName() is the first name and familyName() the last name. In some countries the family name comes first, that's the reason for the naming. formattedName() is the full name with the correct formatting. It is used as an override, when the correct formatting can't be generated from the other name fields automatically.
realName() returns a fully formatted name(). It uses formattedName, if set, otherwise it constructs the name from the name fields. As fallback, if nothing else is set it uses name().
name() is the NAME type of RFC2426. It can be used as internal name for the data entry, but shouldn't be used for displaying the data to the user.
Definition at line 69 of file addressee.h.
Member Typedef Documentation
◆ List
A list of addressee objects.
Definition at line 129 of file addressee.h.
Property Documentation
◆ additionalName
|
readwrite |
Definition at line 81 of file addressee.h.
◆ addresses
|
readwrite |
Definition at line 104 of file addressee.h.
◆ anniversary
|
readwrite |
Definition at line 109 of file addressee.h.
◆ assembledName
|
read |
Definition at line 100 of file addressee.h.
◆ assistantsName
|
readwrite |
Definition at line 110 of file addressee.h.
◆ birthday
|
readwrite |
Definition at line 85 of file addressee.h.
◆ birthdayHasTime
|
read |
Definition at line 86 of file addressee.h.
◆ blogFeed
|
readwrite |
Definition at line 111 of file addressee.h.
◆ categories
|
readwrite |
Definition at line 105 of file addressee.h.
◆ changed
|
readwrite |
Definition at line 107 of file addressee.h.
◆ customs
|
read |
Definition at line 106 of file addressee.h.
◆ department
|
readwrite |
Definition at line 92 of file addressee.h.
◆ emails
|
readwrite |
Definition at line 102 of file addressee.h.
◆ extraUrls
|
readwrite |
Definition at line 98 of file addressee.h.
◆ familyName
|
readwrite |
Definition at line 79 of file addressee.h.
◆ formattedName
|
readwrite |
Definition at line 78 of file addressee.h.
◆ geo
|
readwrite |
Definition at line 88 of file addressee.h.
◆ givenName
|
readwrite |
Definition at line 80 of file addressee.h.
◆ impps
|
readwrite |
Definition at line 108 of file addressee.h.
◆ isEmpty
|
read |
Definition at line 75 of file addressee.h.
◆ mailer
|
readwrite |
Definition at line 87 of file addressee.h.
◆ managersName
|
readwrite |
Definition at line 112 of file addressee.h.
◆ name
|
readwrite |
Definition at line 77 of file addressee.h.
◆ nickName
|
read |
Definition at line 84 of file addressee.h.
◆ note
|
readwrite |
Definition at line 93 of file addressee.h.
◆ office
|
readwrite |
Definition at line 113 of file addressee.h.
◆ organization
|
read |
Definition at line 91 of file addressee.h.
◆ phoneNumbers
|
readwrite |
Definition at line 103 of file addressee.h.
◆ photo
|
readwrite |
Definition at line 116 of file addressee.h.
◆ preferredEmail
|
read |
Definition at line 101 of file addressee.h.
◆ prefix
|
readwrite |
Definition at line 82 of file addressee.h.
◆ productId
|
readwrite |
Definition at line 94 of file addressee.h.
◆ profession
|
readwrite |
Definition at line 114 of file addressee.h.
◆ realName
|
read |
Definition at line 99 of file addressee.h.
◆ revision
|
read |
Definition at line 95 of file addressee.h.
◆ role
|
read |
Definition at line 90 of file addressee.h.
◆ sortString
|
readwrite |
Definition at line 96 of file addressee.h.
◆ spousesName
|
readwrite |
Definition at line 115 of file addressee.h.
◆ suffix
|
readwrite |
Definition at line 83 of file addressee.h.
◆ title
|
read |
Definition at line 89 of file addressee.h.
◆ uid
|
readwrite |
Definition at line 76 of file addressee.h.
◆ url
|
readwrite |
Definition at line 97 of file addressee.h.
Constructor & Destructor Documentation
◆ Addressee() [1/2]
Addressee::Addressee | ( | ) |
Construct an empty address book entry.
Definition at line 181 of file addressee.cpp.
◆ ~Addressee()
Addressee::~Addressee | ( | ) |
Destroys the address book entry.
Definition at line 186 of file addressee.cpp.
◆ Addressee() [2/2]
Addressee::Addressee | ( | const Addressee & | other | ) |
Copy constructor.
Definition at line 190 of file addressee.cpp.
Member Function Documentation
◆ addEmail()
void Addressee::addEmail | ( | const Email & | ) |
Adds an email address.
If the email address (i.e. email.mail()
) already exists in this addressee it won't be duplicated, instead email
is assigned to it.
- Since
- 5.88
Definition at line 1618 of file addressee.cpp.
◆ additionalName()
QString Addressee::additionalName | ( | ) | const |
Return additional names.
Definition at line 724 of file addressee.cpp.
◆ additionalNameLabel()
|
static |
Return translated label for additionalName field.
Definition at line 729 of file addressee.cpp.
◆ address()
Address Addressee::address | ( | Address::Type | type | ) | const |
Return address, which matches the given type.
- Parameters
-
type The type of address to look for
Definition at line 2019 of file addressee.cpp.
◆ addresses() [1/2]
Address::List Addressee::addresses | ( | ) | const |
Return list of all addresses.
Definition at line 2035 of file addressee.cpp.
◆ addresses() [2/2]
Address::List Addressee::addresses | ( | Address::Type | type | ) | const |
Return list of addresses with a special type.
- Parameters
-
type The type of addresses to look for
Definition at line 2040 of file addressee.cpp.
◆ anniversary()
QDate Addressee::anniversary | ( | ) | const |
Returns the contact's anniversary date.
- Note
- This is a non-standard extension using the
X-Anniversary
field.
- Since
- 5.12
Definition at line 2147 of file addressee.cpp.
◆ assembledName()
QString Addressee::assembledName | ( | ) | const |
Return the name that consists of all name parts.
Definition at line 1576 of file addressee.cpp.
◆ assistantsName()
QString Addressee::assistantsName | ( | ) | const |
Returns the contact's assistant's name.
- Note
- This is a non-standard extension using the
X-AssistantsName
field.
- Since
- 5.12
Definition at line 2161 of file addressee.cpp.
◆ birthday()
QDateTime Addressee::birthday | ( | ) | const |
Return birthday.
(If a valid date has been set, birthday().time() will always return a valid QTime!)
Definition at line 850 of file addressee.cpp.
◆ birthdayHasTime()
bool Addressee::birthdayHasTime | ( | ) | const |
Returns true if birthday has been set with a time.
Returns false otherwise.
Definition at line 855 of file addressee.cpp.
◆ birthdayLabel()
|
static |
Return translated label for birthday field.
Definition at line 860 of file addressee.cpp.
◆ blogFeed()
QUrl Addressee::blogFeed | ( | ) | const |
Returns the contact's blog feed.
- Note
- This is a non-standard extension using the
BlogFeed
field.
- Since
- 5.12
Definition at line 2175 of file addressee.cpp.
◆ businessAddressCountryLabel()
|
static |
Return translated label for businessAddressCountry field.
Definition at line 925 of file addressee.cpp.
◆ businessAddressLabelLabel()
|
static |
Return translated label for businessAddressLabel field.
Definition at line 930 of file addressee.cpp.
◆ businessAddressLocalityLabel()
|
static |
Return translated label for businessAddressLocality field.
Definition at line 910 of file addressee.cpp.
◆ businessAddressPostalCodeLabel()
|
static |
Return translated label for businessAddressPostalCode field.
Definition at line 920 of file addressee.cpp.
◆ businessAddressPostOfficeBoxLabel()
|
static |
Return translated label for businessAddressPostOfficeBox field.
Definition at line 905 of file addressee.cpp.
◆ businessAddressRegionLabel()
|
static |
Return translated label for businessAddressRegion field.
Definition at line 915 of file addressee.cpp.
◆ businessAddressStreetLabel()
|
static |
Return translated label for businessAddressStreet field.
Definition at line 900 of file addressee.cpp.
◆ businessFaxLabel()
|
static |
Return translated label for businessFax field.
Definition at line 955 of file addressee.cpp.
◆ businessPhoneLabel()
|
static |
Return translated label for businessPhone field.
Definition at line 940 of file addressee.cpp.
◆ calendarUrlList()
CalendarUrl::List Addressee::calendarUrlList | ( | ) | const |
Definition at line 649 of file addressee.cpp.
◆ carPhoneLabel()
|
static |
Return translated label for carPhone field.
Definition at line 960 of file addressee.cpp.
◆ categories()
QStringList Addressee::categories | ( | ) | const |
Return list of all set categories.
Definition at line 2089 of file addressee.cpp.
◆ changed()
bool Addressee::changed | ( | ) | const |
Return whether the addressee is changed.
Definition at line 2503 of file addressee.cpp.
◆ clientPidMapList()
ClientPidMap::List Addressee::clientPidMapList | ( | ) | const |
Definition at line 600 of file addressee.cpp.
◆ custom()
Return value of custom entry, identified by app and entry name.
- Parameters
-
app Name of the application which has inserted this custom entry name Name of this application specific custom entry
Definition at line 2274 of file addressee.cpp.
◆ customs()
QStringList Addressee::customs | ( | ) | const |
Return list of all custom entries.
The format of the custom entries is 'app-key:value' and the list is sorted alphabetically by 'app-key'.
Definition at line 2306 of file addressee.cpp.
◆ department()
QString Addressee::department | ( | ) | const |
Return department.
Definition at line 1203 of file addressee.cpp.
◆ departmentLabel()
|
static |
Return translated label for department field.
Definition at line 1208 of file addressee.cpp.
◆ emailLabel()
|
static |
Return translated label for email field.
Definition at line 975 of file addressee.cpp.
◆ emailList()
Email::List Addressee::emailList | ( | ) | const |
Definition at line 1672 of file addressee.cpp.
◆ emails()
QStringList Addressee::emails | ( | ) | const |
Return list of all email addresses.
Definition at line 1660 of file addressee.cpp.
◆ extraLogoList()
Picture::List Addressee::extraLogoList | ( | ) | const |
Definition at line 521 of file addressee.cpp.
◆ extraNickNameList()
NickName::List Addressee::extraNickNameList | ( | ) | const |
Definition at line 806 of file addressee.cpp.
◆ extraOrganizationList()
Org::List Addressee::extraOrganizationList | ( | ) | const |
Definition at line 1174 of file addressee.cpp.
◆ extraPhotoList()
Picture::List Addressee::extraPhotoList | ( | ) | const |
Definition at line 510 of file addressee.cpp.
◆ extraRoleList()
Role::List Addressee::extraRoleList | ( | ) | const |
Definition at line 1123 of file addressee.cpp.
◆ extraSoundList()
Sound::List Addressee::extraSoundList | ( | ) | const |
Definition at line 499 of file addressee.cpp.
◆ extraTitleList()
Title::List Addressee::extraTitleList | ( | ) | const |
Definition at line 1075 of file addressee.cpp.
◆ extraUrlList()
ResourceLocatorUrl::List Addressee::extraUrlList | ( | ) | const |
Definition at line 558 of file addressee.cpp.
◆ familyName()
QString Addressee::familyName | ( | ) | const |
Return family name.
Definition at line 684 of file addressee.cpp.
◆ familyNameLabel()
|
static |
Return translated label for familyName field.
Definition at line 689 of file addressee.cpp.
◆ fieldGroupList()
FieldGroup::List Addressee::fieldGroupList | ( | ) | const |
Definition at line 580 of file addressee.cpp.
◆ findAddress()
Return address with the given id.
- Parameters
-
id The identifier of the address to look for. See Address::id()
Definition at line 2051 of file addressee.cpp.
◆ findKey()
Return key with the given id.
- Parameters
-
id The identifier of the key to look for. See Key::id()
Definition at line 1902 of file addressee.cpp.
◆ findPhoneNumber()
PhoneNumber Addressee::findPhoneNumber | ( | const QString & | id | ) | const |
Return phone number with the given id.
- Parameters
-
id The identifier of the phone number to look for. See PhoneNumber::id()
Definition at line 1815 of file addressee.cpp.
◆ formattedName()
QString Addressee::formattedName | ( | ) | const |
Return formatted name.
Definition at line 664 of file addressee.cpp.
◆ formattedNameLabel()
|
static |
Return translated label for formattedName field.
Definition at line 669 of file addressee.cpp.
◆ fullEmail()
Return email address including real name.
- Parameters
-
email Email address to be used to construct the full email string. If this is QString() the preferred email address is used.
Definition at line 1589 of file addressee.cpp.
◆ gender()
Gender Addressee::gender | ( | ) | const |
Definition at line 1743 of file addressee.cpp.
◆ geo()
Geo Addressee::geo | ( | ) | const |
Return geographic position.
Definition at line 1030 of file addressee.cpp.
◆ geoLabel()
|
static |
Return translated label for geo field.
Definition at line 1035 of file addressee.cpp.
◆ givenName()
QString Addressee::givenName | ( | ) | const |
Return given name.
Definition at line 704 of file addressee.cpp.
◆ givenNameLabel()
|
static |
Return translated label for givenName field.
Definition at line 709 of file addressee.cpp.
◆ hasCategory()
bool Addressee::hasCategory | ( | const QString & | category | ) | const |
Return, if addressee has the given category.
Definition at line 2077 of file addressee.cpp.
◆ homeAddressCountryLabel()
|
static |
Return translated label for homeAddressCountry field.
Definition at line 890 of file addressee.cpp.
◆ homeAddressLabelLabel()
|
static |
Return translated label for homeAddressLabel field.
Definition at line 895 of file addressee.cpp.
◆ homeAddressLocalityLabel()
|
static |
Return translated label for homeAddressLocality field.
Definition at line 875 of file addressee.cpp.
◆ homeAddressPostalCodeLabel()
|
static |
Return translated label for homeAddressPostalCode field.
Definition at line 885 of file addressee.cpp.
◆ homeAddressPostOfficeBoxLabel()
|
static |
Return translated label for homeAddressPostOfficeBox field.
Definition at line 870 of file addressee.cpp.
◆ homeAddressRegionLabel()
|
static |
Return translated label for homeAddressRegion field.
Definition at line 880 of file addressee.cpp.
◆ homeAddressStreetLabel()
|
static |
Return translated label for homeAddressStreet field.
Definition at line 865 of file addressee.cpp.
◆ homeFaxLabel()
|
static |
Return translated label for homeFax field.
Definition at line 950 of file addressee.cpp.
◆ homePhoneLabel()
|
static |
Return translated label for homePhone field.
Definition at line 935 of file addressee.cpp.
◆ imppList()
Impp::List Addressee::imppList | ( | ) | const |
Definition at line 635 of file addressee.cpp.
◆ insertAddress()
void Addressee::insertAddress | ( | const Address & | address | ) |
Insert an address.
If an address with the same id already exists in this addressee it is not duplicated.
- Parameters
-
address The address to insert
Definition at line 1984 of file addressee.cpp.
◆ insertCalendarUrl()
void Addressee::insertCalendarUrl | ( | const CalendarUrl & | calendarUrl | ) |
Definition at line 640 of file addressee.cpp.
◆ insertCategory()
void Addressee::insertCategory | ( | const QString & | category | ) |
Insert category.
If the category already exists it is not duplicated.
Definition at line 2059 of file addressee.cpp.
◆ insertClientPidMap()
void Addressee::insertClientPidMap | ( | const ClientPidMap & | clientpidmap | ) |
Definition at line 611 of file addressee.cpp.
◆ insertCustom()
Insert custom entry.
The entry is identified by the name of the inserting application and a unique name. If an entry with the given app and name already exists its value is replaced with the new given value.
An empty value isn't allowed (nothing happens if this is called with any of the three arguments being empty)
- Parameters
-
app Name of the application inserting this custom entry name Name of this application specific custom entry value Value of this application specific custom entry
Definition at line 2245 of file addressee.cpp.
◆ insertExtraLogo()
void Addressee::insertExtraLogo | ( | const Picture & | logo | ) |
Definition at line 515 of file addressee.cpp.
◆ insertExtraNickName()
void Addressee::insertExtraNickName | ( | const NickName & | nickName | ) |
Definition at line 792 of file addressee.cpp.
◆ insertExtraOrganization()
void Addressee::insertExtraOrganization | ( | const Org & | organization | ) |
Definition at line 1160 of file addressee.cpp.
◆ insertExtraPhoto()
void Addressee::insertExtraPhoto | ( | const Picture & | picture | ) |
Definition at line 504 of file addressee.cpp.
◆ insertExtraRole()
void Addressee::insertExtraRole | ( | const Role & | role | ) |
Definition at line 1109 of file addressee.cpp.
◆ insertExtraSound()
void Addressee::insertExtraSound | ( | const Sound & | sound | ) |
Definition at line 493 of file addressee.cpp.
◆ insertExtraTitle()
void Addressee::insertExtraTitle | ( | const Title & | title | ) |
Definition at line 1058 of file addressee.cpp.
◆ insertExtraUrl()
void Addressee::insertExtraUrl | ( | const ResourceLocatorUrl & | url | ) |
Definition at line 544 of file addressee.cpp.
◆ insertFieldGroup()
void Addressee::insertFieldGroup | ( | const FieldGroup & | fieldGroup | ) |
Definition at line 591 of file addressee.cpp.
◆ insertImpp()
void Addressee::insertImpp | ( | const Impp & | impp | ) |
Definition at line 620 of file addressee.cpp.
◆ insertKey()
void Addressee::insertKey | ( | const Key & | key | ) |
Insert a key.
If a key with the same id already exists in this addressee it is not duplicated.
- Parameters
-
key The key to insert
Definition at line 1824 of file addressee.cpp.
◆ insertLang()
void Addressee::insertLang | ( | const Lang & | language | ) |
◆ insertMember()
void Addressee::insertMember | ( | const QString & | member | ) |
Definition at line 2094 of file addressee.cpp.
◆ insertPhoneNumber()
void Addressee::insertPhoneNumber | ( | const PhoneNumber & | phoneNumber | ) |
Insert a phone number.
If a phone number with the same id already exists in this addressee it is not duplicated.
- Parameters
-
phoneNumber The telephone number to insert to the addressee
Definition at line 1748 of file addressee.cpp.
◆ insertRelationship()
void Addressee::insertRelationship | ( | const Related & | related | ) |
Definition at line 2116 of file addressee.cpp.
◆ insertSourceUrl()
void Addressee::insertSourceUrl | ( | const QUrl & | url | ) |
Definition at line 563 of file addressee.cpp.
◆ isdnLabel()
|
static |
Return translated label for isdn field.
Definition at line 965 of file addressee.cpp.
◆ isEmpty()
bool Addressee::isEmpty | ( | ) | const |
Return if the address book entry is empty.
Definition at line 433 of file addressee.cpp.
◆ key()
Return key, which matches the given type.
If type
== Key::Custom you can specify a string that should match. If you leave the string empty, the first key with a custom value is returned.
- Parameters
-
type The type of key to look for customTypeString A string to match custom keys against when type
isKey::Custom
Definition at line 1846 of file addressee.cpp.
◆ keys() [1/2]
Key::List Addressee::keys | ( | ) | const |
Return list of all keys.
Definition at line 1872 of file addressee.cpp.
◆ keys() [2/2]
Return list of keys with a special type.
If type
== Key::Custom you can specify a string that should match. If you leave the string empty, all custom keys will be returned.
- Parameters
-
type The type of key to look for customTypeString A string to match custom keys against when type
isKey::Custom
Definition at line 1877 of file addressee.cpp.
◆ kind()
QString Addressee::kind | ( | ) | const |
Definition at line 488 of file addressee.cpp.
◆ langs()
Lang::List Addressee::langs | ( | ) | const |
◆ logo()
Picture Addressee::logo | ( | ) | const |
Return logo.
Definition at line 1349 of file addressee.cpp.
◆ logoLabel()
|
static |
Return translated label for logo field.
Definition at line 1354 of file addressee.cpp.
◆ mailer()
QString Addressee::mailer | ( | ) | const |
Return mail client.
Definition at line 990 of file addressee.cpp.
◆ mailerLabel()
|
static |
Return translated label for mailer field.
Definition at line 995 of file addressee.cpp.
◆ managersName()
QString Addressee::managersName | ( | ) | const |
Returns the contact's manager's name.
- Note
- This is a non-standard extension using the
X-ManagersName
field.
- Since
- 5.12
Definition at line 2189 of file addressee.cpp.
◆ members()
QStringList Addressee::members | ( | ) | const |
Definition at line 2111 of file addressee.cpp.
◆ mimeType()
|
static |
Returns the MIME type used for Addressees.
Definition at line 2508 of file addressee.cpp.
◆ mobilePhoneLabel()
|
static |
Return translated label for mobilePhone field.
Definition at line 945 of file addressee.cpp.
◆ name()
QString Addressee::name | ( | ) | const |
Return name.
Definition at line 468 of file addressee.cpp.
◆ nameLabel()
|
static |
Return translated label for name field.
Definition at line 473 of file addressee.cpp.
◆ nickName()
QString Addressee::nickName | ( | ) | const |
Return nick name.
Definition at line 811 of file addressee.cpp.
◆ nickNameLabel()
|
static |
Return translated label for nickName field.
Definition at line 820 of file addressee.cpp.
◆ note()
QString Addressee::note | ( | ) | const |
Return note.
Definition at line 1223 of file addressee.cpp.
◆ noteLabel()
|
static |
Return translated label for note field.
Definition at line 1228 of file addressee.cpp.
◆ office()
QString Addressee::office | ( | ) | const |
Returns the contact's office.
- Note
- This is a non-standard extension using the
X-Office
field.
- Since
- 5.12
Definition at line 2203 of file addressee.cpp.
◆ operator!=()
bool Addressee::operator!= | ( | const Addressee & | other | ) | const |
Not-equal operator.
- Returns
true
ifthis
and the given addressee are not equal, otherwisefalse
Definition at line 428 of file addressee.cpp.
◆ operator=()
◆ operator==()
bool Addressee::operator== | ( | const Addressee & | other | ) | const |
Equality operator.
- Returns
true
ifthis
and the given addressee are equal, otherwisefalse
Definition at line 204 of file addressee.cpp.
◆ organization()
QString Addressee::organization | ( | ) | const |
Return organization.
Definition at line 1179 of file addressee.cpp.
◆ organizationLabel()
|
static |
Return translated label for organization field.
Definition at line 1188 of file addressee.cpp.
◆ pagerLabel()
|
static |
Return translated label for pager field.
Definition at line 970 of file addressee.cpp.
◆ parseEmailAddress()
|
static |
Parse full email address.
The result is given back in fullName and email.
- Parameters
-
rawEmail The input string to parse for name and email fullName The name part of the rawEmail
input, if it contained oneemail The email part of the rawEmail
input, if it contained one
Definition at line 2319 of file addressee.cpp.
◆ phoneNumber()
PhoneNumber Addressee::phoneNumber | ( | PhoneNumber::Type | type | ) | const |
Return phone number, which matches the given type.
- Parameters
-
type The type of phone number to get
Definition at line 1776 of file addressee.cpp.
◆ phoneNumbers() [1/2]
PhoneNumber::List Addressee::phoneNumbers | ( | ) | const |
Return list of all phone numbers.
Definition at line 1793 of file addressee.cpp.
◆ phoneNumbers() [2/2]
PhoneNumber::List Addressee::phoneNumbers | ( | PhoneNumber::Type | type | ) | const |
Return list of phone numbers with a special type.
- Parameters
-
type The type of phone number to get
Definition at line 1805 of file addressee.cpp.
◆ photo()
Picture Addressee::photo | ( | ) | const |
Return photo.
Definition at line 1369 of file addressee.cpp.
◆ photoLabel()
|
static |
Return translated label for photo field.
Definition at line 1374 of file addressee.cpp.
◆ preferredEmail()
QString Addressee::preferredEmail | ( | ) | const |
Return preferred email address.
This is the first email address or the last one added with insertEmail() or addEmail() with a set preferred parameter.
Definition at line 1651 of file addressee.cpp.
◆ prefix()
QString Addressee::prefix | ( | ) | const |
Return honorific prefixes.
Definition at line 744 of file addressee.cpp.
◆ prefixLabel()
|
static |
Return translated label for prefix field.
Definition at line 749 of file addressee.cpp.
◆ productId()
QString Addressee::productId | ( | ) | const |
Return product identifier.
Definition at line 1243 of file addressee.cpp.
◆ productIdLabel()
|
static |
Return translated label for productId field.
Definition at line 1248 of file addressee.cpp.
◆ profession()
QString Addressee::profession | ( | ) | const |
Returns the contact's profession.
- Note
- This is a non-standard extension using the
X-Profession
field.
- Since
- 5.12
Definition at line 2217 of file addressee.cpp.
◆ realName()
QString Addressee::realName | ( | ) | const |
Return the name of the addressee.
This is calculated from all the name fields.
Definition at line 1556 of file addressee.cpp.
◆ relationships()
Related::List Addressee::relationships | ( | ) | const |
Definition at line 2133 of file addressee.cpp.
◆ removeAddress()
void Addressee::removeAddress | ( | const Address & | address | ) |
Remove address.
If no address with the given id exists for this addressee, nothing happens.
- Parameters
-
address The address to remove
Definition at line 2003 of file addressee.cpp.
◆ removeCategory()
void Addressee::removeCategory | ( | const QString & | category | ) |
Remove category.
Definition at line 2070 of file addressee.cpp.
◆ removeCustom()
Remove custom entry.
- Parameters
-
app Name of the application which has inserted this custom entry name Name of this application specific custom entry
Definition at line 2265 of file addressee.cpp.
◆ removeEmail()
void Addressee::removeEmail | ( | const QString & | ) |
Remove email address.
If the email address doesn't exist, nothing happens.
- Parameters
-
email Email address to remove
Definition at line 1642 of file addressee.cpp.
◆ removeKey()
void Addressee::removeKey | ( | const Key & | key | ) |
Remove a key.
If no key with the given id exists for this addressee, nothing happens.
- Parameters
-
key The key to remove
Definition at line 1838 of file addressee.cpp.
◆ removeLang()
void Addressee::removeLang | ( | const QString & | language | ) |
◆ removePhoneNumber()
void Addressee::removePhoneNumber | ( | const PhoneNumber & | phoneNumber | ) |
Remove phone number.
If no phone number with the given id exists for this addressee, nothing happens.
- Parameters
-
phoneNumber The telephone number to remove from the addressee
Definition at line 1765 of file addressee.cpp.
◆ revision()
QDateTime Addressee::revision | ( | ) | const |
Return revision date.
Definition at line 1263 of file addressee.cpp.
◆ revisionLabel()
|
static |
Return translated label for revision field.
Definition at line 1268 of file addressee.cpp.
◆ role()
QString Addressee::role | ( | ) | const |
Return role.
Definition at line 1128 of file addressee.cpp.
◆ roleLabel()
|
static |
Return translated label for role field.
Definition at line 1137 of file addressee.cpp.
◆ secrecy()
Secrecy Addressee::secrecy | ( | ) | const |
Return security class.
Definition at line 1329 of file addressee.cpp.
◆ secrecyLabel()
|
static |
Return translated label for secrecy field.
Definition at line 1334 of file addressee.cpp.
◆ setAdditionalName()
void Addressee::setAdditionalName | ( | const QString & | additionalName | ) |
Set additional names.
Definition at line 714 of file addressee.cpp.
◆ setAddresses()
void Addressee::setAddresses | ( | const Address::List & | addresses | ) |
Set the addressee.
- Parameters
-
addresses The new addresses
- Since
- 5.100
Definition at line 2013 of file addressee.cpp.
◆ setAnniversary()
void Addressee::setAnniversary | ( | const QDate & | anniversary | ) |
Sets the contact's anniversary date.
- Note
- This is a non-standard extension using the
X-Anniversary
field.
- Since
- 5.12
Definition at line 2152 of file addressee.cpp.
◆ setAssistantsName()
void Addressee::setAssistantsName | ( | const QString & | assistantsName | ) |
Set the contact's assistant's name.
- Note
- This is a non-standard extension using the
X-AssistantsName
field.
- Since
- 5.12
Definition at line 2166 of file addressee.cpp.
◆ setBirthday() [1/2]
void Addressee::setBirthday | ( | const QDate & | birthday | ) |
Set birthday (date only).
birthdayHasTime() will return false afterwards.
Definition at line 839 of file addressee.cpp.
◆ setBirthday() [2/2]
void Addressee::setBirthday | ( | const QDateTime & | birthday, |
bool | withTime = true ) |
Set birthday (date and time).
If withTime is false the time will be set to midnight and birthdayHasTime() will return false afterwards.
- Since
- 5.4
Definition at line 825 of file addressee.cpp.
◆ setBlogFeed()
void Addressee::setBlogFeed | ( | const QUrl & | blogFeed | ) |
Set the contact's blog feed.
- Note
- This is a non-standard extension using the
BlogFeed
field.
- Since
- 5.12
Definition at line 2180 of file addressee.cpp.
◆ setCategories()
void Addressee::setCategories | ( | const QStringList & | category | ) |
Set categories to given value.
Definition at line 2082 of file addressee.cpp.
◆ setChanged()
void Addressee::setChanged | ( | bool | value | ) |
Mark addressee as changed.
- Parameters
-
value Sets the status indicating changed data
Definition at line 2498 of file addressee.cpp.
◆ setClientPidMapList()
void Addressee::setClientPidMapList | ( | const ClientPidMap::List & | clientpidmaplist | ) |
Definition at line 605 of file addressee.cpp.
◆ setCustoms()
void Addressee::setCustoms | ( | const QStringList & | customs | ) |
Set all custom entries.
Definition at line 2281 of file addressee.cpp.
◆ setDepartment()
void Addressee::setDepartment | ( | const QString & | department | ) |
Set department.
Definition at line 1193 of file addressee.cpp.
◆ setEmailList()
void Addressee::setEmailList | ( | const Email::List & | list | ) |
Definition at line 1688 of file addressee.cpp.
◆ setEmails()
void Addressee::setEmails | ( | const QStringList & | list | ) |
Set the emails to list
.
The first email address gets the preferred one!
- Parameters
-
list The list of email addresses.
Definition at line 1677 of file addressee.cpp.
◆ setExtraLogoList()
void Addressee::setExtraLogoList | ( | const Picture::List & | logoList | ) |
Definition at line 538 of file addressee.cpp.
◆ setExtraNickNameList()
void Addressee::setExtraNickNameList | ( | const NickName::List & | nickNameList | ) |
Definition at line 800 of file addressee.cpp.
◆ setExtraOrganizationList()
void Addressee::setExtraOrganizationList | ( | const Org::List & | orgList | ) |
Definition at line 1168 of file addressee.cpp.
◆ setExtraPhotoList()
void Addressee::setExtraPhotoList | ( | const Picture::List & | pictureList | ) |
Definition at line 532 of file addressee.cpp.
◆ setExtraRoleList()
void Addressee::setExtraRoleList | ( | const Role::List & | roleList | ) |
Definition at line 1117 of file addressee.cpp.
◆ setExtraSoundList()
void Addressee::setExtraSoundList | ( | const Sound::List & | soundList | ) |
Definition at line 526 of file addressee.cpp.
◆ setExtraTitleList()
void Addressee::setExtraTitleList | ( | const Title::List & | urltitle | ) |
Definition at line 1080 of file addressee.cpp.
◆ setExtraUrlList()
void Addressee::setExtraUrlList | ( | const ResourceLocatorUrl::List & | urlList | ) |
Definition at line 552 of file addressee.cpp.
◆ setFamilyName()
void Addressee::setFamilyName | ( | const QString & | familyName | ) |
Set family name.
Definition at line 674 of file addressee.cpp.
◆ setFieldGroupList()
void Addressee::setFieldGroupList | ( | const FieldGroup::List & | fieldGroupList | ) |
Definition at line 585 of file addressee.cpp.
◆ setFormattedName()
void Addressee::setFormattedName | ( | const QString & | formattedName | ) |
Set formatted name.
Definition at line 654 of file addressee.cpp.
◆ setGender()
void Addressee::setGender | ( | const Gender & | gender | ) |
Definition at line 1733 of file addressee.cpp.
◆ setGeo()
void Addressee::setGeo | ( | const Geo & | geo | ) |
Set geographic position.
Definition at line 1020 of file addressee.cpp.
◆ setGivenName()
void Addressee::setGivenName | ( | const QString & | givenName | ) |
Set given name.
Definition at line 694 of file addressee.cpp.
◆ setImppList()
void Addressee::setImppList | ( | const Impp::List & | imppList | ) |
Definition at line 629 of file addressee.cpp.
◆ setKeys()
void Addressee::setKeys | ( | const Key::List & | keys | ) |
Set the list of keys.
- Parameters
-
keys The keys to be set.
Definition at line 1866 of file addressee.cpp.
◆ setKind()
void Addressee::setKind | ( | const QString & | kind | ) |
Definition at line 478 of file addressee.cpp.
◆ setLangs()
void Addressee::setLangs | ( | const Lang::List & | langs | ) |
Definition at line 1703 of file addressee.cpp.
◆ setLogo()
void Addressee::setLogo | ( | const Picture & | logo | ) |
Set logo.
Definition at line 1339 of file addressee.cpp.
◆ setMailer()
void Addressee::setMailer | ( | const QString & | mailer | ) |
Set mail client.
Definition at line 980 of file addressee.cpp.
◆ setManagersName()
void Addressee::setManagersName | ( | const QString & | managersName | ) |
Set the contact's manager's name.
- Note
- This is a non-standard extension using the
X-ManagersName
field.
- Since
- 5.12
Definition at line 2194 of file addressee.cpp.
◆ setMembers()
void Addressee::setMembers | ( | const QStringList & | c | ) |
Definition at line 2105 of file addressee.cpp.
◆ setName()
void Addressee::setName | ( | const QString & | name | ) |
Set name.
Definition at line 458 of file addressee.cpp.
◆ setNameFromString()
void Addressee::setNameFromString | ( | const QString & | s | ) |
Set name fields by parsing the given string and trying to associate the parts of the string with according fields.
This function should probably be a bit more clever.
Definition at line 1399 of file addressee.cpp.
◆ setNickName() [1/2]
void Addressee::setNickName | ( | const NickName & | nickName | ) |
Definition at line 787 of file addressee.cpp.
◆ setNickName() [2/2]
void Addressee::setNickName | ( | const QString & | nickName | ) |
Set nick name.
Definition at line 774 of file addressee.cpp.
◆ setNote()
void Addressee::setNote | ( | const QString & | note | ) |
Set note.
Definition at line 1213 of file addressee.cpp.
◆ setOffice()
void Addressee::setOffice | ( | const QString & | office | ) |
Set the contact's office.
- Note
- This is a non-standard extension using the
X-Office
field.
- Since
- 5.12
Definition at line 2208 of file addressee.cpp.
◆ setOrganization() [1/2]
void Addressee::setOrganization | ( | const Org & | organization | ) |
Definition at line 1155 of file addressee.cpp.
◆ setOrganization() [2/2]
void Addressee::setOrganization | ( | const QString & | organization | ) |
Set organization.
Definition at line 1142 of file addressee.cpp.
◆ setPhoneNumbers()
void Addressee::setPhoneNumbers | ( | const PhoneNumber::List & | phoneNumbers | ) |
Definition at line 1798 of file addressee.cpp.
◆ setPhoto()
void Addressee::setPhoto | ( | const Picture & | photo | ) |
Set photo.
Definition at line 1359 of file addressee.cpp.
◆ setPrefix()
void Addressee::setPrefix | ( | const QString & | prefix | ) |
Set honorific prefixes.
Definition at line 734 of file addressee.cpp.
◆ setProductId()
void Addressee::setProductId | ( | const QString & | productId | ) |
Set product identifier.
Definition at line 1233 of file addressee.cpp.
◆ setProfession()
void Addressee::setProfession | ( | const QString & | profession | ) |
Set the contact's profession.
- Note
- This is a non-standard extension using the
X-Profession
field.
- Since
- 5.12
Definition at line 2222 of file addressee.cpp.
◆ setRelationships()
void Addressee::setRelationships | ( | const Related::List & | c | ) |
Definition at line 2127 of file addressee.cpp.
◆ setRevision()
void Addressee::setRevision | ( | const QDateTime & | revision | ) |
Set revision date.
Definition at line 1253 of file addressee.cpp.
◆ setRole() [1/2]
void Addressee::setRole | ( | const QString & | role | ) |
Set role.
Definition at line 1091 of file addressee.cpp.
◆ setRole() [2/2]
void Addressee::setRole | ( | const Role & | role | ) |
Definition at line 1104 of file addressee.cpp.
◆ setSecrecy()
void Addressee::setSecrecy | ( | const Secrecy & | secrecy | ) |
Set security class.
Definition at line 1319 of file addressee.cpp.
◆ setSortString()
void Addressee::setSortString | ( | const QString & | sortString | ) |
Set sort string.
Definition at line 1273 of file addressee.cpp.
◆ setSound()
void Addressee::setSound | ( | const Sound & | sound | ) |
Set sound.
Definition at line 1379 of file addressee.cpp.
◆ setSourcesUrlList()
Definition at line 569 of file addressee.cpp.
◆ setSpousesName()
void Addressee::setSpousesName | ( | const QString & | spousesName | ) |
Set the contact's spouse's name.
- Note
- This is a non-standard extension using the
X-SpousesName
field.
- Since
- 5.12
Definition at line 2236 of file addressee.cpp.
◆ setSuffix()
void Addressee::setSuffix | ( | const QString & | suffix | ) |
Set honorific suffixes.
Definition at line 754 of file addressee.cpp.
◆ setTimeZone()
void Addressee::setTimeZone | ( | const TimeZone & | timeZone | ) |
Set time zone.
Definition at line 1000 of file addressee.cpp.
◆ setTitle() [1/2]
void Addressee::setTitle | ( | const QString & | title | ) |
Set title.
Definition at line 1040 of file addressee.cpp.
◆ setTitle() [2/2]
void Addressee::setTitle | ( | const Title & | title | ) |
Definition at line 1053 of file addressee.cpp.
◆ setUid()
void Addressee::setUid | ( | const QString & | uid | ) |
Set unique identifier.
- Parameters
-
uid the KABC unique identifier
Definition at line 438 of file addressee.cpp.
◆ setUrl() [1/2]
void Addressee::setUrl | ( | const QUrl & | url | ) |
Definition at line 1293 of file addressee.cpp.
◆ setUrl() [2/2]
void Addressee::setUrl | ( | const ResourceLocatorUrl & | url | ) |
Set homepage.
Definition at line 1300 of file addressee.cpp.
◆ sortString()
QString Addressee::sortString | ( | ) | const |
Return sort string.
Definition at line 1283 of file addressee.cpp.
◆ sortStringLabel()
|
static |
Return translated label for sortString field.
Definition at line 1288 of file addressee.cpp.
◆ sound()
Sound Addressee::sound | ( | ) | const |
Return sound.
Definition at line 1389 of file addressee.cpp.
◆ soundLabel()
|
static |
Return translated label for sound field.
Definition at line 1394 of file addressee.cpp.
◆ sourcesUrlList()
Definition at line 575 of file addressee.cpp.
◆ spousesName()
QString Addressee::spousesName | ( | ) | const |
Returns the contact's spouse's name.
- Note
- This is a non-standard extension using the
X-SpousesName
field.
- Since
- 5.12
Definition at line 2231 of file addressee.cpp.
◆ suffix()
QString Addressee::suffix | ( | ) | const |
Return honorific suffixes.
Definition at line 764 of file addressee.cpp.
◆ suffixLabel()
|
static |
Return translated label for suffix field.
Definition at line 769 of file addressee.cpp.
◆ timeZone()
TimeZone Addressee::timeZone | ( | ) | const |
Return time zone.
Definition at line 1010 of file addressee.cpp.
◆ timeZoneLabel()
|
static |
Return translated label for timeZone field.
Definition at line 1015 of file addressee.cpp.
◆ title()
QString Addressee::title | ( | ) | const |
Return title.
Definition at line 1066 of file addressee.cpp.
◆ titleLabel()
|
static |
Return translated label for title field.
Definition at line 1086 of file addressee.cpp.
◆ toString()
QString Addressee::toString | ( | ) | const |
Returns string representation of the addressee.
Definition at line 1911 of file addressee.cpp.
◆ uid()
QString Addressee::uid | ( | ) | const |
Return unique identifier.
Definition at line 448 of file addressee.cpp.
◆ uidLabel()
|
static |
Return translated label for uid field.
Definition at line 453 of file addressee.cpp.
◆ url()
ResourceLocatorUrl Addressee::url | ( | ) | const |
Return homepage.
Definition at line 1305 of file addressee.cpp.
◆ urlLabel()
|
static |
Return translated label for url field.
Definition at line 1314 of file addressee.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:54:01 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.