KI18n
#include <ki18n_export.h>
#include <QChar>
#include <QLatin1Char>
#include <QSet>
#include <QString>
#include <QStringList>
#include <kuitsetup.h>
#include <klocalizedcontext.h>
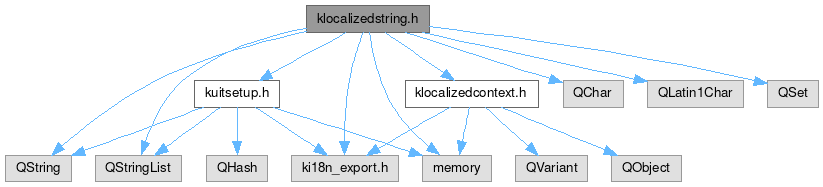

Go to the source code of this file.
Classes | |
class | KLocalizedString |
Functions | |
QString | i18n (const char *text, const TYPE &arg...) |
QString | i18nc (const char *context, const char *text, const TYPE &arg...) |
QString | i18ncp (const char *context, const char *singular, const char *plural, const TYPE &arg...) |
QString | i18nd (const char *domain, const char *text, const TYPE &arg...) |
QString | i18ndc (const char *domain, const char *context, const char *text, const TYPE &arg...) |
QString | i18ndcp (const char *domain, const char *context, const char *singular, const char *plural, const TYPE &arg...) |
QString | i18ndp (const char *domain, const char *singular, const char *plural, const TYPE &arg...) |
QString | i18np (const char *singular, const char *plural, const TYPE &arg...) |
KLocalizedString KI18N_EXPORT | ki18n (const char *text) |
KLocalizedString KI18N_EXPORT | ki18nc (const char *context, const char *text) |
KLocalizedString KI18N_EXPORT | ki18ncp (const char *context, const char *singular, const char *plural) |
KLocalizedString KI18N_EXPORT | ki18nd (const char *domain, const char *text) |
KLocalizedString KI18N_EXPORT | ki18ndc (const char *domain, const char *context, const char *text) |
KLocalizedString KI18N_EXPORT | ki18ndcp (const char *domain, const char *context, const char *singular, const char *plural) |
KLocalizedString KI18N_EXPORT | ki18ndp (const char *domain, const char *singular, const char *plural) |
KLocalizedString KI18N_EXPORT | ki18np (const char *singular, const char *plural) |
KLocalizedString KI18N_EXPORT | kxi18n (const char *text) |
KLocalizedString KI18N_EXPORT | kxi18nc (const char *context, const char *text) |
KLocalizedString KI18N_EXPORT | kxi18ncp (const char *context, const char *singular, const char *plural) |
KLocalizedString KI18N_EXPORT | kxi18nd (const char *domain, const char *text) |
KLocalizedString KI18N_EXPORT | kxi18ndc (const char *domain, const char *context, const char *text) |
KLocalizedString KI18N_EXPORT | kxi18ndcp (const char *domain, const char *context, const char *singular, const char *plural) |
KLocalizedString KI18N_EXPORT | kxi18ndp (const char *domain, const char *singular, const char *plural) |
KLocalizedString KI18N_EXPORT | kxi18np (const char *singular, const char *plural) |
QString | tr2i18n (const char *text, const char *comment=nullptr) |
QString | tr2i18nd (const char *domain, const char *text, const char *comment=nullptr) |
QString | tr2xi18n (const char *text, const char *comment=nullptr) |
QString | tr2xi18nd (const char *domain, const char *text, const char *comment=nullptr) |
QString | xi18n (const char *text, const TYPE &arg...) |
QString | xi18nc (const char *context, const char *text, const TYPE &arg...) |
QString | xi18ncp (const char *context, const char *singular, const char *plural, const TYPE &arg...) |
QString | xi18nd (const char *domain, const char *text, const TYPE &arg...) |
QString | xi18ndc (const char *domain, const char *context, const char *text, const TYPE &arg...) |
QString | xi18ndcp (const char *domain, const char *context, const char *singular, const char *plural, const TYPE &arg...) |
QString | xi18ndp (const char *domain, const char *singular, const char *plural, const TYPE &arg...) |
QString | xi18np (const char *singular, const char *plural, const TYPE &arg...) |
Function Documentation
◆ i18n()
QString i18n | ( | const char * | text, |
const TYPE & | arg... ) |
Translate a string and substitute any arguments.
- Parameters
-
text string to translate arg arguments to insert (0 to 9), admissible types according to KLocalizedString::subs
methods
- Returns
- translated string
◆ i18nc()
QString i18nc | ( | const char * | context, |
const char * | text, | ||
const TYPE & | arg... ) |
Translate a string with context and substitute any arguments.
- Parameters
-
context context of the string text string to translate arg arguments to insert (0 to 9), admissible types according to KLocalizedString::subs
methods
- Returns
- translated string
◆ i18ncp()
QString i18ncp | ( | const char * | context, |
const char * | singular, | ||
const char * | plural, | ||
const TYPE & | arg... ) |
Translate a string with context and plural and substitute any arguments.
- Parameters
-
context context of the string singular singular form of the string to translate plural plural form of the string to translate arg arguments to insert (0 to 9), admissible types according to KLocalizedString::subs
methods
- Returns
- translated string
◆ i18nd()
QString i18nd | ( | const char * | domain, |
const char * | text, | ||
const TYPE & | arg... ) |
Translate a string from domain and substitute any arguments.
- Parameters
-
domain domain in which to look for translations text string to translate arg arguments to insert (0 to 9), admissible types according to KLocalizedString::subs
methods
- Returns
- translated string
◆ i18ndc()
QString i18ndc | ( | const char * | domain, |
const char * | context, | ||
const char * | text, | ||
const TYPE & | arg... ) |
Translate a string from domain with context and substitute any arguments.
- Parameters
-
domain domain in which to look for translations context context of the string text string to translate arg arguments to insert (0 to 9), admissible types according to KLocalizedString::subs
methods
- Returns
- translated string
◆ i18ndcp()
QString i18ndcp | ( | const char * | domain, |
const char * | context, | ||
const char * | singular, | ||
const char * | plural, | ||
const TYPE & | arg... ) |
Translate a string from domain with context and plural and substitute any arguments.
- Parameters
-
domain domain in which to look for translations context context of the string singular singular form of the string to translate plural plural form of the string to translate arg arguments to insert (0 to 9), admissible types according to KLocalizedString::subs
methods
- Returns
- translated string
◆ i18ndp()
QString i18ndp | ( | const char * | domain, |
const char * | singular, | ||
const char * | plural, | ||
const TYPE & | arg... ) |
Translate a string from domain with plural and substitute any arguments.
- Parameters
-
domain domain in which to look for translations singular singular form of the string to translate plural plural form of the string to translate arg arguments to insert (0 to 9), admissible types according to KLocalizedString::subs
methods
- Returns
- translated string
◆ i18np()
QString i18np | ( | const char * | singular, |
const char * | plural, | ||
const TYPE & | arg... ) |
Translate a string with plural and substitute any arguments.
- Parameters
-
singular singular form of the string to translate plural plural form of the string to translate arg arguments to insert (0 to 9), admissible types according to KLocalizedString::subs
methods
- Returns
- translated string
◆ ki18n()
KLocalizedString KI18N_EXPORT ki18n | ( | const char * | text | ) |
Create non-finalized translated string.
- Parameters
-
text string to translate
- Returns
- non-finalized translated string
Definition at line 49 of file klocalizedstring.cpp.
◆ ki18nc()
KLocalizedString KI18N_EXPORT ki18nc | ( | const char * | context, |
const char * | text ) |
Create non-finalized translated string with context.
- Parameters
-
context context of the string text string to translate
- Returns
- non-finalized translated string
Definition at line 50 of file klocalizedstring.cpp.
◆ ki18ncp()
KLocalizedString KI18N_EXPORT ki18ncp | ( | const char * | context, |
const char * | singular, | ||
const char * | plural ) |
Create non-finalized translated string with context and plural.
- Parameters
-
context context of the string singular singular form of the string to translate plural plural form of the string to translate
- Returns
- non-finalized translated string
Definition at line 52 of file klocalizedstring.cpp.
◆ ki18nd()
KLocalizedString KI18N_EXPORT ki18nd | ( | const char * | domain, |
const char * | text ) |
Create non-finalized translated string from domain.
- Parameters
-
domain domain in which to look for translations text string to translate
- Returns
- non-finalized translated string
Definition at line 53 of file klocalizedstring.cpp.
◆ ki18ndc()
KLocalizedString KI18N_EXPORT ki18ndc | ( | const char * | domain, |
const char * | context, | ||
const char * | text ) |
Create non-finalized translated string from domain with context.
- Parameters
-
domain domain in which to look for translations context context of the string text string to translate
- Returns
- non-finalized translated string
Definition at line 54 of file klocalizedstring.cpp.
◆ ki18ndcp()
KLocalizedString KI18N_EXPORT ki18ndcp | ( | const char * | domain, |
const char * | context, | ||
const char * | singular, | ||
const char * | plural ) |
Create non-finalized translated string from domain with context and plural.
- Parameters
-
domain domain in which to look for translations context context of the string singular singular form of the string to translate plural plural form of the string to translate
- Returns
- non-finalized translated string
Definition at line 56 of file klocalizedstring.cpp.
◆ ki18ndp()
KLocalizedString KI18N_EXPORT ki18ndp | ( | const char * | domain, |
const char * | singular, | ||
const char * | plural ) |
Create non-finalized translated string from domain with plural.
- Parameters
-
domain domain in which to look for translations singular singular form of the string to translate plural plural form of the string to translate
- Returns
- non-finalized translated string
Definition at line 55 of file klocalizedstring.cpp.
◆ ki18np()
KLocalizedString KI18N_EXPORT ki18np | ( | const char * | singular, |
const char * | plural ) |
Create non-finalized translated string with plural.
- Parameters
-
singular singular form of the string to translate plural plural form of the string to translate
- Returns
- non-finalized translated string
Definition at line 51 of file klocalizedstring.cpp.
◆ kxi18n()
KLocalizedString KI18N_EXPORT kxi18n | ( | const char * | text | ) |
Create non-finalized markup-aware translated string.
- Parameters
-
text string to translate
- Returns
- non-finalized translated string
Definition at line 58 of file klocalizedstring.cpp.
◆ kxi18nc()
KLocalizedString KI18N_EXPORT kxi18nc | ( | const char * | context, |
const char * | text ) |
Create non-finalized markup-aware translated string with context.
- Parameters
-
context context of the string text string to translate
- Returns
- non-finalized translated string
Definition at line 59 of file klocalizedstring.cpp.
◆ kxi18ncp()
KLocalizedString KI18N_EXPORT kxi18ncp | ( | const char * | context, |
const char * | singular, | ||
const char * | plural ) |
Create non-finalized markup-aware translated string.
with context and plural.
- Parameters
-
context context of the string singular singular form of the string to translate plural plural form of the string to translate
- Returns
- non-finalized translated string
Definition at line 61 of file klocalizedstring.cpp.
◆ kxi18nd()
KLocalizedString KI18N_EXPORT kxi18nd | ( | const char * | domain, |
const char * | text ) |
Create non-finalized markup-aware translated string from domain.
- Parameters
-
domain domain in which to look for translations text string to translate
- Returns
- non-finalized translated string
Definition at line 62 of file klocalizedstring.cpp.
◆ kxi18ndc()
KLocalizedString KI18N_EXPORT kxi18ndc | ( | const char * | domain, |
const char * | context, | ||
const char * | text ) |
Create non-finalized markup-aware translated string from domain with context.
- Parameters
-
domain domain in which to look for translations context context of the string text string to translate
- Returns
- non-finalized translated string
Definition at line 63 of file klocalizedstring.cpp.
◆ kxi18ndcp()
KLocalizedString KI18N_EXPORT kxi18ndcp | ( | const char * | domain, |
const char * | context, | ||
const char * | singular, | ||
const char * | plural ) |
Create non-finalized markup-aware translated string from domain with context and plural.
- Parameters
-
domain domain in which to look for translations context context of the string singular singular form of the string to translate plural plural form of the string to translate
- Returns
- non-finalized translated string
Definition at line 65 of file klocalizedstring.cpp.
◆ kxi18ndp()
KLocalizedString KI18N_EXPORT kxi18ndp | ( | const char * | domain, |
const char * | singular, | ||
const char * | plural ) |
Create non-finalized markup-aware translated string from domain with plural.
- Parameters
-
domain domain in which to look for translations singular singular form of the string to translate plural plural form of the string to translate
- Returns
- non-finalized translated string
Definition at line 64 of file klocalizedstring.cpp.
◆ kxi18np()
KLocalizedString KI18N_EXPORT kxi18np | ( | const char * | singular, |
const char * | plural ) |
Create non-finalized markup-aware translated string with plural.
- Parameters
-
singular singular form of the string to translate plural plural form of the string to translate
- Returns
- non-finalized translated string
Definition at line 60 of file klocalizedstring.cpp.
◆ tr2i18n()
|
inline |
Redirect Qt's uic
-generated translation calls to Ki18n.
Use -tr tr2i18n
option to uic
to have it redirect calls.
- Parameters
-
text string to translate comment Qt equivalent of disambiguation context
- Returns
- translated string
Definition at line 894 of file klocalizedstring.h.
◆ tr2i18nd()
|
inline |
Like tr2i18n
, but look for translation in a specific domain.
Use -tr tr2i18nd
option to uic
to have it redirect calls.
- Parameters
-
domain domain in which to look for translations text string to translate comment Qt equivalent of disambiguation context
- Returns
- translated string
Definition at line 915 of file klocalizedstring.h.
◆ tr2xi18n()
|
inline |
Like tr2i18n
, but when UI strings are KUIT markup-aware.
Use -tr tr2xi18n
option to uic
to have it redirect calls.
- Parameters
-
text markup-aware string to translate comment Qt equivalent of disambiguation context
- Returns
- translated string
Definition at line 935 of file klocalizedstring.h.
◆ tr2xi18nd()
|
inline |
Like tr2xi18n
, but look for translation in a specific domain.
Use -tr tr2xi18nd
option to uic
to have it redirect calls.
- Parameters
-
domain domain in which to look for translations text markup-aware string to translate comment Qt equivalent of disambiguation context
- Returns
- translated string
Definition at line 956 of file klocalizedstring.h.
◆ xi18n()
QString xi18n | ( | const char * | text, |
const TYPE & | arg... ) |
Translate a markup-aware string and substitute any arguments.
- Parameters
-
text string to translate arg arguments to insert (0 to 9), admissible types according to KLocalizedString::subs
methods
- Returns
- translated string
◆ xi18nc()
QString xi18nc | ( | const char * | context, |
const char * | text, | ||
const TYPE & | arg... ) |
Translate a markup-aware string with context and substitute any arguments.
- Parameters
-
context context of the string text string to translate arg arguments to insert (0 to 9), admissible types according to KLocalizedString::subs
methods
- Returns
- translated string
◆ xi18ncp()
QString xi18ncp | ( | const char * | context, |
const char * | singular, | ||
const char * | plural, | ||
const TYPE & | arg... ) |
Translate a markup-aware string with context and plural and substitute any arguments.
- Parameters
-
context context of the string singular singular form of the string to translate plural plural form of the string to translate arg arguments to insert (0 to 9), admissible types according to KLocalizedString::subs
methods
- Returns
- translated string
◆ xi18nd()
QString xi18nd | ( | const char * | domain, |
const char * | text, | ||
const TYPE & | arg... ) |
Translate a markup-aware string from domain and substitute any arguments.
- Parameters
-
domain domain in which to look for translations text string to translate arg arguments to insert (0 to 9), admissible types according to KLocalizedString::subs
methods
- Returns
- translated string
◆ xi18ndc()
QString xi18ndc | ( | const char * | domain, |
const char * | context, | ||
const char * | text, | ||
const TYPE & | arg... ) |
Translate a markup-aware string from domain with context and substitute any arguments.
- Parameters
-
domain domain in which to look for translations context context of the string text string to translate arg arguments to insert (0 to 9), admissible types according to KLocalizedString::subs
methods
- Returns
- translated string
◆ xi18ndcp()
QString xi18ndcp | ( | const char * | domain, |
const char * | context, | ||
const char * | singular, | ||
const char * | plural, | ||
const TYPE & | arg... ) |
Translate a markup-aware string from domain with context and plural and substitute any arguments.
- Parameters
-
domain domain in which to look for translations context context of the string singular singular form of the string to translate plural plural form of the string to translate arg arguments to insert (0 to 9), admissible types according to KLocalizedString::subs
methods
- Returns
- translated string
◆ xi18ndp()
QString xi18ndp | ( | const char * | domain, |
const char * | singular, | ||
const char * | plural, | ||
const TYPE & | arg... ) |
Translate a markup-aware string from domain with plural and substitute any arguments.
- Parameters
-
domain domain in which to look for translations singular singular form of the string to translate plural plural form of the string to translate arg arguments to insert (0 to 9), admissible types according to KLocalizedString::subs
methods
- Returns
- translated string
◆ xi18np()
QString xi18np | ( | const char * | singular, |
const char * | plural, | ||
const TYPE & | arg... ) |
Translate a markup-aware string with plural and substitute any arguments.
- Parameters
-
singular singular form of the string to translate plural plural form of the string to translate arg arguments to insert (0 to 9), admissible types according to KLocalizedString::subs
methods
- Returns
- translated string
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:05:36 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.