KNotification
#include <KNotification>
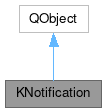
Public Types | |
enum | NotificationFlag { CloseOnTimeout = 0x00 , Persistent = 0x02 , LoopSound = 0x08 , SkipGrouping = 0x10 , CloseWhenWindowActivated = 0x20 , DefaultEvent = 0xF000 } |
typedef QFlags< NotificationFlag > | NotificationFlags |
enum | StandardEvent { Notification , Warning , Error , Catastrophe } |
enum | Urgency { DefaultUrgency = -1 , LowUrgency = 10 , NormalUrgency = 50 , HighUrgency = 70 , CriticalUrgency = 90 } |
Properties | |
bool | autoDelete |
QString | componentName |
QString | eventId |
NotificationFlags | flags |
QVariantMap | hints |
QString | iconName |
QString | text |
QString | title |
Urgency | urgency |
QList< QUrl > | urls |
QString | xdgActivationToken |
![]() | |
objectName | |
Signals | |
void | actionsChanged () |
void | autoDeleteChanged () |
void | closed () |
void | componentNameChanged () |
void | defaultActionChanged () |
void | eventIdChanged () |
void | flagsChanged () |
void | hintsChanged () |
void | iconNameChanged () |
void | ignored () |
void | textChanged () |
void | titleChanged () |
void | urgencyChanged () |
void | urlsChanged () |
void | xdgActivationTokenChanged () |
Public Slots | |
void | close () |
QVariantMap | hints () const |
void | sendEvent () |
Q_INVOKABLE void | setHint (const QString &hint, const QVariant &value) |
void | setHints (const QVariantMap &hints) |
Public Member Functions | |
KNotification (const QString &eventId, NotificationFlags flags=CloseOnTimeout, QObject *parent=nullptr) | |
KNotificationAction * | addAction (const QString &label) |
KNotificationAction * | addDefaultAction (const QString &label) |
QString | appName () const |
void | clearActions () |
QString | componentName () const |
KNotificationAction * | defaultAction () const |
virtual bool | event (QEvent *e) |
QString | eventId () const |
NotificationFlags | flags () const |
QString | iconName () const |
bool | isAutoDelete () const |
QPixmap | pixmap () const |
KNotificationReplyAction * | replyAction () const |
void | setAutoDelete (bool autoDelete) |
void | setComponentName (const QString &componentName) |
void | setEventId (const QString &eventId) |
void | setFlags (const NotificationFlags &flags) |
void | setIconName (const QString &icon) |
void | setPixmap (const QPixmap &pix) |
void | setReplyAction (std::unique_ptr< KNotificationReplyAction > replyAction) |
void | setText (const QString &text) |
void | setTitle (const QString &title) |
void | setUrgency (Urgency urgency) |
void | setUrls (const QList< QUrl > &urls) |
void | setWindow (QWindow *window) |
QString | text () const |
QString | title () const |
Urgency | urgency () const |
QList< QUrl > | urls () const |
QWindow * | window () const |
QString | xdgActivationToken () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
KNotification is the main class for creating notifications.
Definition at line 95 of file knotification.h.
Member Typedef Documentation
◆ NotificationFlags
Stores a combination of NotificationFlag values.
Definition at line 203 of file knotification.h.
Member Enumeration Documentation
◆ NotificationFlag
- See also
- NotificationFlags
Enumerator | |
---|---|
CloseOnTimeout | The notification will be automatically closed after a timeout. (this is the default) |
Persistent | The notification will NOT be automatically closed after a timeout. You will have to track the notification, and close it with the close function manually when the event is done, otherwise there will be a memory leak |
LoopSound | The audio plugin will loop the sound until the notification is closed. |
SkipGrouping | Sends a hint to Plasma to skip grouping for this notification.
|
CloseWhenWindowActivated | The notification will be automatically closed if the window() becomes activated. You need to set a window using setWindow().
|
DefaultEvent | The event is a standard kde event, and not an event of the application |
Definition at line 157 of file knotification.h.
◆ StandardEvent
default events you can use in the event function
Definition at line 209 of file knotification.h.
◆ Urgency
The urgency of a notification.
- Since
- 5.58
- See also
- setUrgency
Definition at line 222 of file knotification.h.
Property Documentation
◆ autoDelete
|
readwrite |
Sets whether this notification object will be automatically deleted after closing.
This is on by default for C++, and off by default for QML.
- Since
- 5.88
- 5.88
Definition at line 142 of file knotification.h.
◆ componentName
|
readwrite |
The componentData is used to determine the location of the config file.
If no componentName is set, the app name is used by default
- Parameters
-
componentName the new component name
- Since
- 5.88
Definition at line 127 of file knotification.h.
◆ eventId
|
readwrite |
Set the event id, if not already passed to the constructor.
- Since
- 5.88
- 5.88
Definition at line 102 of file knotification.h.
◆ flags
|
readwrite |
Set the notification flags.
These must be set before calling sendEvent()
- Since
- 5.88
Definition at line 122 of file knotification.h.
◆ hints
|
readwrite |
- Since
- 5.57 Adds a custom hint to the notification. Those are key-value pairs that can be interpreted by the respective notification backend to trigger additional, non-standard features.
- Parameters
-
hint the hint's key value the hint's value
- Since
- 5.101
Definition at line 151 of file knotification.h.
◆ iconName
|
readwrite |
Set the icon that will be shown in the popup.
- Parameters
-
icon the icon
- Since
- 5.4
- 5.88
Definition at line 117 of file knotification.h.
◆ text
|
readwrite |
Set the notification text that will appear in the popup.
In Plasma workspace, the text is shown in a QML label which uses Text.StyledText, ie. it supports a small subset of HTML entities (mostly just formatting tags)
If the notifications server does not advertise "body-markup" capability, all HTML tags are stripped before sending it to the server
- Parameters
-
text The text to display in the notification popup
- Since
- 5.88
Definition at line 112 of file knotification.h.
◆ title
|
readwrite |
Set the title of the notification popup.
If no title is set, the application name will be used.
- Parameters
-
title The title of the notification
- Since
- 4.3
- 5.88
Definition at line 107 of file knotification.h.
◆ urgency
|
readwrite |
Sets the urgency of the notification.
This defines the importance of the notification. For example, a track change in a media player would be a low urgency. "You have new mail" would be normal urgency. "Your battery level is low" would be a critical urgency.
Use critical notifications with care as they might be shown even when giving a presentation or when notifications are turned off.
- Parameters
-
urgency The urgency.
- Since
- 5.58
- 5.88
Definition at line 137 of file knotification.h.
◆ urls
Sets URLs associated with this notification.
For example, a screenshot application might want to provide the URL to the file that was just taken so the notification service can show a preview.
- Note
- This feature might not be supported by the user's notification service
- Parameters
-
urls A list of URLs
- Since
- 5.29
- 5.88
Definition at line 132 of file knotification.h.
◆ xdgActivationToken
|
read |
- Since
- 5.90
Definition at line 146 of file knotification.h.
Constructor & Destructor Documentation
◆ KNotification()
|
explicit |
Create a new notification.
You have to use sendEvent to show the notification.
The pointer is automatically deleted when the event is closed.
- Since
- 4.4
- Parameters
-
eventId is the name of the event flags is a bitmask of NotificationFlag parent parent object
Definition at line 81 of file knotification.cpp.
◆ ~KNotification()
|
override |
Definition at line 93 of file knotification.cpp.
Member Function Documentation
◆ actionsChanged
|
signal |
Emitted when actions
changed.
- Since
- 5.88
◆ addAction()
|
nodiscard |
Add an action to the notification.
The visual representation of actions depends on the notification server.
- Parameters
-
label the user-visible label of the action
- See also
- KNotificationAction
- Since
- 6.0
Definition at line 208 of file knotification.cpp.
◆ addDefaultAction()
|
nodiscard |
Add a default action that will be triggered when the notification is activated (typically, by clicking on the notification popup).
The default action typically raises a window belonging to the application that sent it.
The string will be used as a label for the action, so ideally it should be wrapped in i18n() or tr() calls.
The visual representation of actions depends on the notification server. In Plasma and Gnome desktops, the actions are performed by clicking on the notification popup, and the label is not presented to the user.
Calling this overrides the current default action
- Since
- 6.0
Definition at line 276 of file knotification.cpp.
◆ appName()
QString KNotification::appName | ( | ) | const |
appname used for the D-Bus object
Definition at line 528 of file knotification.cpp.
◆ autoDeleteChanged
|
signal |
Emitted when autoDelete
changed.
- Since
- 5.88
◆ beep()
This is a simple substitution for QApplication::beep()
- Parameters
-
reason a short text explaining what has happened (may be empty)
Definition at line 504 of file knotification.cpp.
◆ clearActions()
void KNotification::clearActions | ( | ) |
Removes all actions previously added by addAction() from the notification.
- See also
- addAction
- Since
- 6.0
Definition at line 194 of file knotification.cpp.
◆ close
|
slot |
Close the notification without activating it.
This will delete the notification.
Definition at line 400 of file knotification.cpp.
◆ closed
|
signal |
Emitted when the notification is closed.
Can be closed either by the user clicking the close button, the timeout running out or when an action was triggered.
◆ componentName()
QString KNotification::componentName | ( | ) | const |
Returns the component name used to determine the location of the configuration file.
- Since
- 5.88
Definition at line 344 of file knotification.cpp.
◆ componentNameChanged
|
signal |
Emitted when componentName
changed.
- Since
- 5.88
◆ defaultAction()
KNotificationAction * KNotification::defaultAction | ( | ) | const |
- Returns
- the default action, or nullptr if none is set
- Since
- 6.0
Definition at line 320 of file knotification.cpp.
◆ defaultActionChanged
|
signal |
Emitted when defaultAction
changed.
- Since
- 5.88
◆ event() [1/8]
|
static |
emit a standard event
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
This will emit a standard event
- Parameters
-
eventId is the name of the event text is the text of the notification to show in the popup. pixmap is a picture which may be shown in the popup. flags is a bitmask of NotificationFlag componentName used to determine the location of the config file. by default, plasma_workspace is used
Definition at line 447 of file knotification.cpp.
◆ event() [2/8]
|
static |
emit an event
This method creates the KNotification, setting every parameter, and fire the event. You don't need to call sendEvent
A popup may be displayed or a sound may be played, depending the config.
- Returns
- a KNotification . You may use that pointer to connect some signals or slot. the pointer is automatically deleted when the event is closed.
- Parameters
-
eventId is the name of the event title is title of the notification to show in the popup. text is the text of the notification to show in the popup. pixmap is a picture which may be shown in the popup. flags is a bitmask of NotificationFlag componentName used to determine the location of the config file. by default, appname is used
- Since
- 4.4
Definition at line 428 of file knotification.cpp.
◆ event() [3/8]
|
static |
emit a standard event with the possibility of setting an icon by icon name
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
This will emit a standard event
- Parameters
-
eventId is the name of the event title is title of the notification to show in the popup. text is the text of the notification to show in the popup iconName a Freedesktop compatible icon name to be shown in the popup flags is a bitmask of NotificationFlag componentName used to determine the location of the config file. by default, plasma_workspace is used
- Since
- 5.4
Definition at line 462 of file knotification.cpp.
◆ event() [4/8]
◆ event() [5/8]
|
static |
emit a standard event
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
This will emit a standard event
- Parameters
-
eventId is the name of the event text is the text of the notification to show in the popup pixmap is a picture which may be shown in the popup flags is a bitmask of NotificationFlag
Definition at line 457 of file knotification.cpp.
◆ event() [6/8]
|
static |
emit a standard event
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
This will emit a standard event with its standard icon
- Parameters
-
eventId the type of the standard (not app-defined) event title is title of the notification to show in the popup. text is the text of the notification to show in the popup flags is a bitmask of NotificationFlag
- Since
- 5.9
Definition at line 485 of file knotification.cpp.
◆ event() [7/8]
|
static |
emit a standard event
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
This will emit a standard event
- Parameters
-
eventId is the name of the event title is title of the notification to show in the popup. text is the text of the notification to show in the popup pixmap is a picture which may be shown in the popup flags is a bitmask of NotificationFlag
- Since
- 4.4
Definition at line 452 of file knotification.cpp.
◆ event() [8/8]
|
static |
emit a standard event with the possibility of setting an icon by icon name
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
This will emit a standard event with a custom icon
- Parameters
-
eventId the type of the standard (not app-defined) event title is title of the notification to show in the popup. text is the text of the notification to show in the popup iconName a Freedesktop compatible icon name to be shown in the popup flags is a bitmask of NotificationFlag
- Since
- 5.9
Definition at line 480 of file knotification.cpp.
◆ eventId()
QString KNotification::eventId | ( | ) | const |
- Returns
- the name of the event
Definition at line 105 of file knotification.cpp.
◆ eventIdChanged
|
signal |
Emitted when eventId
changed.
- Since
- 5.88
◆ flags()
KNotification::NotificationFlags KNotification::flags | ( | ) | const |
- Returns
- the notification flags.
Definition at line 325 of file knotification.cpp.
◆ flagsChanged
|
signal |
Emitted when flags
changed.
- Since
- 5.88
◆ hints
|
slot |
- Since
- 5.57 Returns the custom hints set by setHint()
Definition at line 619 of file knotification.cpp.
◆ hintsChanged
|
signal |
Emitted when hints
changes.
- Since
- 5.101
◆ iconName()
QString KNotification::iconName | ( | ) | const |
- Returns
- the icon shown in the popup
- See also
- setIconName
- Since
- 5.4
Definition at line 170 of file knotification.cpp.
◆ iconNameChanged
|
signal |
Emitted when iconName
changed.
- Since
- 5.88
◆ ignored
|
signal |
The notification has been ignored.
◆ isAutoDelete()
bool KNotification::isAutoDelete | ( | ) | const |
Returns whether this notification object will be automatically deleted after closing.
- Since
- 5.88
Definition at line 543 of file knotification.cpp.
◆ pixmap()
QPixmap KNotification::pixmap | ( | ) | const |
- Returns
- the pixmap shown in the popup
- See also
- setPixmap
Definition at line 175 of file knotification.cpp.
◆ replyAction()
KNotificationReplyAction * KNotification::replyAction | ( | ) | const |
◆ sendEvent
|
slot |
Send the notification to the server.
This will cause all the configured plugins to execute their actions on this notification (eg. a sound will play, a popup will show, a command will be executed etc).
Definition at line 509 of file knotification.cpp.
◆ setAutoDelete()
void KNotification::setAutoDelete | ( | bool | autoDelete | ) |
Sets whether this notification object will be automatically deleted after closing.
This is on by default for C++, and off by default for QML.
- Since
- 5.88
Definition at line 548 of file knotification.cpp.
◆ setComponentName()
void KNotification::setComponentName | ( | const QString & | componentName | ) |
The componentData is used to determine the location of the config file.
If no componentName is set, the app name is used by default
- Parameters
-
componentName the new component name
Definition at line 349 of file knotification.cpp.
◆ setEventId()
void KNotification::setEventId | ( | const QString & | eventId | ) |
Set the event id, if not already passed to the constructor.
- Since
- 5.88
Definition at line 110 of file knotification.cpp.
◆ setFlags()
void KNotification::setFlags | ( | const NotificationFlags & | flags | ) |
Set the notification flags.
These must be set before calling sendEvent()
Definition at line 330 of file knotification.cpp.
◆ setHint
- Since
- 5.57 Adds a custom hint to the notification. Those are key-value pairs that can be interpreted by the respective notification backend to trigger additional, non-standard features.
- Parameters
-
hint the hint's key value the hint's value
Definition at line 605 of file knotification.cpp.
◆ setHints
|
slot |
- Since
- 5.101 Set custom hints on the notification.
- See also
- setHint
Definition at line 624 of file knotification.cpp.
◆ setIconName()
void KNotification::setIconName | ( | const QString & | icon | ) |
Set the icon that will be shown in the popup.
- Parameters
-
icon the icon
- Since
- 5.4
Definition at line 156 of file knotification.cpp.
◆ setPixmap()
void KNotification::setPixmap | ( | const QPixmap & | pix | ) |
Set the pixmap that will be shown in the popup.
If you want to use an icon from the icon theme use setIconName instead.
- Parameters
-
pix the pixmap
Definition at line 180 of file knotification.cpp.
◆ setReplyAction()
void KNotification::setReplyAction | ( | std::unique_ptr< KNotificationReplyAction > | replyAction | ) |
Add an inline reply action to the notification.
On supported platforms this lets the user type a reply to a notification, such as replying to a chat message, from the notification popup, for example:
- Parameters
-
replyAction the reply action to set
- Since
- 5.81
Definition at line 263 of file knotification.cpp.
◆ setText()
void KNotification::setText | ( | const QString & | text | ) |
Set the notification text that will appear in the popup.
In Plasma workspace, the text is shown in a QML label which uses Text.StyledText, ie. it supports a small subset of HTML entities (mostly just formatting tags)
If the notifications server does not advertise "body-markup" capability, all HTML tags are stripped before sending it to the server
- Parameters
-
text The text to display in the notification popup
Definition at line 142 of file knotification.cpp.
◆ setTitle()
void KNotification::setTitle | ( | const QString & | title | ) |
Set the title of the notification popup.
If no title is set, the application name will be used.
- Parameters
-
title The title of the notification
- Since
- 4.3
Definition at line 128 of file knotification.cpp.
◆ setUrgency()
void KNotification::setUrgency | ( | Urgency | urgency | ) |
Sets the urgency of the notification.
This defines the importance of the notification. For example, a track change in a media player would be a low urgency. "You have new mail" would be normal urgency. "Your battery level is low" would be a critical urgency.
Use critical notifications with care as they might be shown even when giving a presentation or when notifications are turned off.
- Parameters
-
urgency The urgency.
- Since
- 5.58
Definition at line 373 of file knotification.cpp.
◆ setUrls()
Sets URLs associated with this notification.
For example, a screenshot application might want to provide the URL to the file that was just taken so the notification service can show a preview.
- Note
- This feature might not be supported by the user's notification service
- Parameters
-
urls A list of URLs
- Since
- 5.29
Definition at line 362 of file knotification.cpp.
◆ setWindow()
void KNotification::setWindow | ( | QWindow * | window | ) |
Sets the window associated with this notification.
This is relevant when using the CloseWhenWindowActivated flag.
- Since
- 6.0
Definition at line 643 of file knotification.cpp.
◆ text()
QString KNotification::text | ( | ) | const |
◆ textChanged
|
signal |
Emitted when text
changed.
- Since
- 5.88
◆ title()
QString KNotification::title | ( | ) | const |
- Returns
- the notification title
- See also
- setTitle
- Since
- 4.3
Definition at line 118 of file knotification.cpp.
◆ titleChanged
|
signal |
Emitted when title
changed.
- Since
- 5.88
◆ urgency()
KNotification::Urgency KNotification::urgency | ( | ) | const |
◆ urgencyChanged
|
signal |
Emitted when urgency
changed.
- Since
- 5.88
◆ urls()
◆ urlsChanged
|
signal |
Emitted when urls
changed.
- Since
- 5.88
◆ window()
QWindow * KNotification::window | ( | ) | const |
The window associated with this notification.
nullptr by default.
- Returns
- the window set by setWindow()
- Since
- 6.0
Definition at line 661 of file knotification.cpp.
◆ xdgActivationToken()
QString KNotification::xdgActivationToken | ( | ) | const |
Returns the activation token to use to activate a window.
- Since
- 5.90
Definition at line 638 of file knotification.cpp.
◆ xdgActivationTokenChanged
|
signal |
Emitted when xdgActivationToken
changes.
- Since
- 5.90
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:57:22 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.