BarChart
#include <BarChart.h>
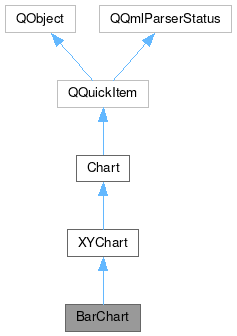
Public Types | |
enum | Orientation { HorizontalOrientation = Qt::Horizontal , VerticalOrientation = Qt::Vertical } |
enum | WidthMode { AutoWidth = -2 } |
![]() | |
enum class | Direction { ZeroAtStart , ZeroAtEnd } |
![]() | |
using | DataSourcesProperty = QQmlListProperty<ChartDataSource> |
enum | IndexingMode { IndexSourceValues = 1 , IndexEachSource , IndexAllValues } |
![]() | |
enum | Flag |
enum | ItemChange |
enum | TransformOrigin |
Properties | |
QColor | backgroundColor |
qreal | barWidth |
Orientation | orientation |
qreal | radius |
qreal | spacing |
![]() | |
Direction | direction |
bool | stacked |
RangeGroup * | xRange |
RangeGroup * | yRange |
![]() | |
ChartDataSource * | colorSource |
int | highlight |
IndexingMode | indexingMode |
ChartDataSource * | nameSource |
ChartDataSource * | shortNameSource |
QQmlListProperty< ChartDataSource > | valueSources |
![]() | |
activeFocus | |
activeFocusOnTab | |
antialiasing | |
baselineOffset | |
childrenRect | |
clip | |
containmentMask | |
enabled | |
focus | |
height | |
implicitHeight | |
implicitWidth | |
opacity | |
parent | |
rotation | |
scale | |
smooth | |
state | |
transformOrigin | |
visible | |
width | |
x | |
y | |
z | |
![]() | |
objectName | |
Public Member Functions | |
BarChart (QQuickItem *parent=nullptr) | |
QColor | backgroundColor () const |
Q_SIGNAL void | backgroundColorChanged () |
qreal | barWidth () const |
Q_SIGNAL void | barWidthChanged () |
Orientation | orientation () const |
Q_SIGNAL void | orientationChanged () |
qreal | radius () const |
Q_SIGNAL void | radiusChanged () |
void | setBackgroundColor (const QColor &newBackgroundColor) |
void | setBarWidth (qreal newBarWidth) |
void | setOrientation (Orientation newOrientation) |
void | setRadius (qreal newRadius) |
void | setSpacing (qreal newSpacing) |
qreal | spacing () const |
Q_SIGNAL void | spacingChanged () |
![]() | |
XYChart (QQuickItem *parent=nullptr) | |
~XYChart () override=default | |
ComputedRange | computedRange () const |
Q_SIGNAL void | computedRangeChanged () |
virtual XYChart::Direction | direction () const |
Q_SIGNAL void | directionChanged () |
virtual void | setDirection (XYChart::Direction newDirection) |
void | setStacked (bool newStacked) |
bool | stacked () const |
Q_SIGNAL void | stackedChanged () |
virtual RangeGroup * | xRange () const |
virtual RangeGroup * | yRange () const |
![]() | |
Chart (QQuickItem *parent=nullptr) | |
ChartDataSource * | colorSource () const |
Q_SIGNAL void | colorSourceChanged () |
Q_SIGNAL void | dataChanged () |
int | highlight () const |
Q_SIGNAL void | highlightChanged () |
IndexingMode | indexingMode () const |
Q_SIGNAL void | indexingModeChanged () |
Q_INVOKABLE void | insertValueSource (int index, ChartDataSource *source) |
ChartDataSource * | nameSource () const |
Q_SIGNAL void | nameSourceChanged () |
Q_INVOKABLE void | removeValueSource (int index) |
Q_INVOKABLE void | removeValueSource (QObject *source) |
void | resetHighlight () |
void | setColorSource (ChartDataSource *colorSource) |
void | setHighlight (int highlight) |
void | setIndexingMode (IndexingMode newIndexingMode) |
void | setNameSource (ChartDataSource *nameSource) |
void | setShortNameSource (ChartDataSource *shortNameSource) |
ChartDataSource * | shortNameSource () const |
Q_SIGNAL void | shortNameSourceChanged () |
QList< ChartDataSource * > | valueSources () const |
Q_SIGNAL void | valueSourcesChanged () |
DataSourcesProperty | valueSourcesProperty () |
![]() | |
QQuickItem (QQuickItem *parent) | |
Qt::MouseButtons | acceptedMouseButtons () const const |
bool | acceptHoverEvents () const const |
bool | acceptTouchEvents () const const |
void | activeFocusChanged (bool) |
bool | activeFocusOnTab () const const |
void | activeFocusOnTabChanged (bool) |
bool | antialiasing () const const |
void | antialiasingChanged (bool) |
qreal | baselineOffset () const const |
void | baselineOffsetChanged (qreal) |
QBindable< qreal > | bindableHeight () |
QBindable< qreal > | bindableWidth () |
QBindable< qreal > | bindableX () |
QBindable< qreal > | bindableY () |
virtual QRectF | boundingRect () const const |
QQuickItem * | childAt (qreal x, qreal y) const const |
QList< QQuickItem * > | childItems () const const |
QRectF | childrenRect () |
void | childrenRectChanged (const QRectF &) |
bool | clip () const const |
void | clipChanged (bool) |
virtual QRectF | clipRect () const const |
QObject * | containmentMask () const const |
void | containmentMaskChanged () |
virtual bool | contains (const QPointF &point) const const |
QCursor | cursor () const const |
void | dumpItemTree () const const |
void | enabledChanged () |
void | ensurePolished () |
bool | filtersChildMouseEvents () const const |
Flags | flags () const const |
void | focusChanged (bool) |
void | forceActiveFocus () |
void | forceActiveFocus (Qt::FocusReason reason) |
void | grabMouse () |
QSharedPointer< QQuickItemGrabResult > | grabToImage (const QSize &targetSize) |
void | grabTouchPoints (const QList< int > &ids) |
bool | hasActiveFocus () const const |
bool | hasFocus () const const |
qreal | height () const const |
void | heightChanged () |
qreal | implicitHeight () const const |
void | implicitHeightChanged () |
qreal | implicitWidth () const const |
void | implicitWidthChanged () |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const const |
bool | isAncestorOf (const QQuickItem *child) const const |
bool | isEnabled () const const |
bool | isFocusScope () const const |
virtual bool | isTextureProvider () const const |
bool | isVisible () const const |
bool | keepMouseGrab () const const |
bool | keepTouchGrab () const const |
QPointF | mapFromGlobal (const QPointF &point) const const |
QPointF | mapFromItem (const QQuickItem *item, const QPointF &point) const const |
QPointF | mapFromScene (const QPointF &point) const const |
QRectF | mapRectFromItem (const QQuickItem *item, const QRectF &rect) const const |
QRectF | mapRectFromScene (const QRectF &rect) const const |
QRectF | mapRectToItem (const QQuickItem *item, const QRectF &rect) const const |
QRectF | mapRectToScene (const QRectF &rect) const const |
QPointF | mapToGlobal (const QPointF &point) const const |
QPointF | mapToItem (const QQuickItem *item, const QPointF &point) const const |
QPointF | mapToScene (const QPointF &point) const const |
QQuickItem * | nextItemInFocusChain (bool forward) |
qreal | opacity () const const |
void | opacityChanged () |
void | parentChanged (QQuickItem *) |
QQuickItem * | parentItem () const const |
void | polish () |
void | resetAntialiasing () |
void | resetHeight () |
void | resetWidth () |
qreal | rotation () const const |
void | rotationChanged () |
qreal | scale () const const |
void | scaleChanged () |
QQuickItem * | scopedFocusItem () const const |
void | setAcceptedMouseButtons (Qt::MouseButtons buttons) |
void | setAcceptHoverEvents (bool enabled) |
void | setAcceptTouchEvents (bool enabled) |
void | setActiveFocusOnTab (bool) |
void | setAntialiasing (bool) |
void | setBaselineOffset (qreal) |
void | setClip (bool) |
void | setContainmentMask (QObject *mask) |
void | setCursor (const QCursor &cursor) |
void | setEnabled (bool) |
void | setFiltersChildMouseEvents (bool filter) |
void | setFlag (Flag flag, bool enabled) |
void | setFlags (Flags flags) |
void | setFocus (bool focus, Qt::FocusReason reason) |
void | setFocus (bool) |
void | setHeight (qreal) |
void | setImplicitHeight (qreal) |
void | setImplicitWidth (qreal) |
void | setKeepMouseGrab (bool keep) |
void | setKeepTouchGrab (bool keep) |
void | setOpacity (qreal) |
void | setParentItem (QQuickItem *parent) |
void | setRotation (qreal) |
void | setScale (qreal) |
void | setSize (const QSizeF &size) |
void | setSmooth (bool) |
void | setState (const QString &) |
void | setTransformOrigin (TransformOrigin) |
void | setVisible (bool) |
void | setWidth (qreal) |
void | setX (qreal) |
void | setY (qreal) |
void | setZ (qreal) |
QSizeF | size () const const |
bool | smooth () const const |
void | smoothChanged (bool) |
void | stackAfter (const QQuickItem *sibling) |
void | stackBefore (const QQuickItem *sibling) |
QString | state () const const |
void | stateChanged (const QString &) |
virtual QSGTextureProvider * | textureProvider () const const |
TransformOrigin | transformOrigin () const const |
void | transformOriginChanged (TransformOrigin) |
void | ungrabMouse () |
void | ungrabTouchPoints () |
void | unsetCursor () |
void | update () |
QQuickItem * | viewportItem () const const |
void | visibleChanged () |
qreal | width () const const |
void | widthChanged () |
QQuickWindow * | window () const const |
void | windowChanged (QQuickWindow *window) |
qreal | x () const const |
void | xChanged () |
qreal | y () const const |
void | yChanged () |
qreal | z () const const |
void | zChanged () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
void | onDataChanged () override |
QSGNode * | updatePaintNode (QSGNode *node, QQuickItem::UpdatePaintNodeData *) override |
![]() | |
void | setComputedRange (ComputedRange range) |
virtual void | updateComputedRange () |
![]() | |
void | componentComplete () override |
QColor | desaturate (const QColor &input) |
![]() | |
virtual bool | childMouseEventFilter (QQuickItem *item, QEvent *event) |
virtual void | classBegin () override |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual bool | event (QEvent *ev) override |
virtual void | focusInEvent (QFocusEvent *) |
virtual void | focusOutEvent (QFocusEvent *) |
virtual void | geometryChange (const QRectF &newGeometry, const QRectF &oldGeometry) |
bool | heightValid () const const |
virtual void | hoverEnterEvent (QHoverEvent *event) |
virtual void | hoverLeaveEvent (QHoverEvent *event) |
virtual void | hoverMoveEvent (QHoverEvent *event) |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
bool | isComponentComplete () const const |
virtual void | itemChange (ItemChange change, const ItemChangeData &value) |
virtual void | keyPressEvent (QKeyEvent *event) |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) |
virtual void | mouseMoveEvent (QMouseEvent *event) |
virtual void | mousePressEvent (QMouseEvent *event) |
virtual void | mouseReleaseEvent (QMouseEvent *event) |
virtual void | mouseUngrabEvent () |
virtual void | releaseResources () |
virtual void | touchEvent (QTouchEvent *event) |
virtual void | touchUngrabEvent () |
void | updateInputMethod (Qt::InputMethodQueries queries) |
virtual QSGNode * | updatePaintNode (QSGNode *oldNode, UpdatePaintNodeData *updatePaintNodeData) |
virtual void | updatePolish () |
virtual void | wheelEvent (QWheelEvent *event) |
bool | widthValid () const const |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
An item to render a bar chart.
This chart renders
Usage example
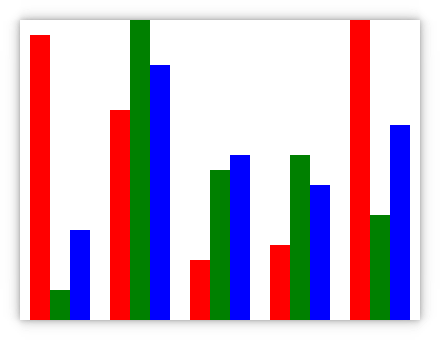
Definition at line 27 of file BarChart.h.
Member Enumeration Documentation
◆ Orientation
Definition at line 39 of file BarChart.h.
◆ WidthMode
enum BarChart::WidthMode |
Helper enum to provide an easy way to set barWidth to auto.
Definition at line 36 of file BarChart.h.
Property Documentation
◆ backgroundColor
|
readwrite |
The background color of bars in the chart.
By default this is Qt::transparent. If set to something non-transparent, the chart will render backgrounds for the bars. These backgrounds will have the same width as the bars but stretch the full height.
Definition at line 96 of file BarChart.h.
◆ barWidth
|
readwrite |
The width of individual bars in the chart.
If set to WidthMode::AutoWidth (also the default), the width will be calculated automatically based on total chart width and item count.
Definition at line 64 of file BarChart.h.
◆ orientation
|
readwrite |
The orientation of bars in the chart.
By default this is Vertical.
Definition at line 84 of file BarChart.h.
◆ radius
|
readwrite |
The radius of the ends of bars in the chart in pixels.
By default this is 0, which means no rounding will be done.
Definition at line 74 of file BarChart.h.
◆ spacing
|
readwrite |
The spacing between bars for each value source.
Note that spacing between each X axis value is determined automatically based on barWidth, spacing and total chart width. The default is 0.
Definition at line 53 of file BarChart.h.
Constructor & Destructor Documentation
◆ BarChart()
|
explicit |
Definition at line 16 of file BarChart.cpp.
Member Function Documentation
◆ backgroundColor()
QColor BarChart::backgroundColor | ( | ) | const |
Definition at line 86 of file BarChart.cpp.
◆ barWidth()
qreal BarChart::barWidth | ( | ) | const |
Definition at line 37 of file BarChart.cpp.
◆ onDataChanged()
|
overrideprotectedvirtual |
◆ orientation()
BarChart::Orientation BarChart::orientation | ( | ) | const |
Definition at line 69 of file BarChart.cpp.
◆ radius()
qreal BarChart::radius | ( | ) | const |
Definition at line 53 of file BarChart.cpp.
◆ setBackgroundColor()
void BarChart::setBackgroundColor | ( | const QColor & | newBackgroundColor | ) |
Definition at line 91 of file BarChart.cpp.
◆ setBarWidth()
void BarChart::setBarWidth | ( | qreal | newBarWidth | ) |
Definition at line 42 of file BarChart.cpp.
◆ setOrientation()
void BarChart::setOrientation | ( | BarChart::Orientation | newOrientation | ) |
Definition at line 74 of file BarChart.cpp.
◆ setRadius()
void BarChart::setRadius | ( | qreal | newRadius | ) |
Definition at line 58 of file BarChart.cpp.
◆ setSpacing()
void BarChart::setSpacing | ( | qreal | newSpacing | ) |
Definition at line 26 of file BarChart.cpp.
◆ spacing()
qreal BarChart::spacing | ( | ) | const |
Definition at line 21 of file BarChart.cpp.
◆ updatePaintNode()
|
overrideprotected |
Reimplemented from QQuickItem.
Definition at line 102 of file BarChart.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Mar 28 2025 11:47:45 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.