KSvg::FrameSvg
#include <KSvg/FrameSvg>
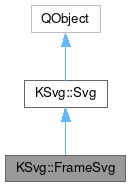
Public Types | |
enum | EnabledBorder { NoBorder = 0 , TopBorder = 1 , BottomBorder = 2 , LeftBorder = 4 , RightBorder = 8 , AllBorders = TopBorder | BottomBorder | LeftBorder | RightBorder } |
typedef QFlags< EnabledBorder > | EnabledBorders |
enum | LocationPrefix { Floating = 0 , TopEdge , BottomEdge , LeftEdge , RightEdge } |
enum | MarginEdge { TopMargin = 0 , BottomMargin , LeftMargin , RightMargin } |
![]() | |
enum | ColorSet { View , Window , Button , Selection , Tooltip , Complementary , Header } |
enum | Status { Normal = 0 , Selected , Inactive } |
enum | StyleSheetColor { Text , Background , Highlight , HighlightedText , PositiveText , NeutralText , NegativeText , ButtonText , ButtonBackground , ButtonHover , ButtonFocus , ButtonHighlightedText , ButtonPositiveText , ButtonNeutralText , ButtonNegativeText , ViewText , ViewBackground , ViewHover , ViewFocus , ViewHighlightedText , ViewPositiveText , ViewNeutralText , ViewNegativeText , TooltipText , TooltipBackground , TooltipHover , TooltipFocus , TooltipHighlightedText , TooltipPositiveText , TooltipNeutralText , TooltipNegativeText , ComplementaryText , ComplementaryBackground , ComplementaryHover , ComplementaryFocus , ComplementaryHighlightedText , ComplementaryPositiveText , ComplementaryNeutralText , ComplementaryNegativeText , HeaderText , HeaderBackground , HeaderHover , HeaderFocus , HeaderHighlightedText , HeaderPositiveText , HeaderNeutralText , HeaderNegativeText } |
Properties | |
EnabledBorders | enabledBorders |
![]() | |
KSvg::Svg::ColorSet | colorSet |
bool | fromCurrentImageSet |
QString | imagePath |
bool | multipleImages |
QSizeF | size |
KSvg::Svg::Status | status |
bool | usingRenderingCache |
![]() | |
objectName | |
Public Member Functions | |
QString | actualPrefix () const |
QPixmap | alphaMask () const |
Q_INVOKABLE bool | cacheAllRenderedFrames () const |
Q_INVOKABLE void | clearCache () |
Q_INVOKABLE QRectF | contentsRect () const |
EnabledBorders | enabledBorders () const |
Q_INVOKABLE qreal | fixedMarginSize (const FrameSvg::MarginEdge edge) const |
Q_INVOKABLE QPixmap | framePixmap () |
Q_INVOKABLE QSizeF | frameSize () const |
Q_INVOKABLE void | getFixedMargins (qreal &left, qreal &top, qreal &right, qreal &bottom) const |
Q_INVOKABLE void | getInset (qreal &left, qreal &top, qreal &right, qreal &bottom) const |
Q_INVOKABLE void | getMargins (qreal &left, qreal &top, qreal &right, qreal &bottom) const |
Q_INVOKABLE bool | hasElementPrefix (const QString &prefix) const |
Q_INVOKABLE bool | hasElementPrefix (KSvg::FrameSvg::LocationPrefix location) const |
Q_INVOKABLE qreal | insetSize (const FrameSvg::MarginEdge edge) const |
bool | isRepaintBlocked () const |
Q_INVOKABLE qreal | marginSize (const FrameSvg::MarginEdge edge) const |
Q_INVOKABLE QRegion | mask () const |
Q_INVOKABLE int | minimumDrawingHeight () |
Q_INVOKABLE int | minimumDrawingWidth () |
Q_INVOKABLE void | paintFrame (QPainter *painter, const QPointF &pos=QPointF(0, 0)) |
Q_INVOKABLE void | paintFrame (QPainter *painter, const QRectF &target, const QRectF &source=QRectF()) |
Q_INVOKABLE QString | prefix () |
Q_INVOKABLE void | resizeFrame (const QSizeF &size) |
Q_INVOKABLE void | setCacheAllRenderedFrames (bool cache) |
Q_INVOKABLE void | setElementPrefix (const QString &prefix) |
Q_INVOKABLE void | setElementPrefix (KSvg::FrameSvg::LocationPrefix location) |
void | setEnabledBorders (const EnabledBorders borders) |
Q_INVOKABLE void | setImagePath (const QString &path) override |
void | setRepaintBlocked (bool blocked) |
![]() | |
void | clearColorOverrides () |
QColor | color (StyleSheetColor colorName) const |
KSvg::Svg::ColorSet | colorSet () const |
bool | containsMultipleImages () const |
qreal | devicePixelRatio () const |
Q_INVOKABLE QRectF | elementRect (const QString &elementId) const |
QRectF | elementRect (QStringView elementId) const |
Q_INVOKABLE QSizeF | elementSize (const QString &elementId) const |
QSizeF | elementSize (QStringView elementId) const |
bool | fromCurrentImageSet () const |
Q_INVOKABLE bool | hasElement (const QString &elementId) const |
bool | hasElement (QStringView elementId) const |
Q_INVOKABLE QImage | image (const QSize &size, const QString &elementID=QString()) |
QString | imagePath () const |
ImageSet * | imageSet () const |
bool | isUsingRenderingCache () const |
Q_INVOKABLE bool | isValid () const |
Q_INVOKABLE void | paint (QPainter *painter, const QPointF &point, const QString &elementID=QString()) |
Q_INVOKABLE void | paint (QPainter *painter, const QRectF &rect, const QString &elementID=QString()) |
Q_INVOKABLE void | paint (QPainter *painter, int x, int y, const QString &elementID=QString()) |
Q_INVOKABLE void | paint (QPainter *painter, int x, int y, int width, int height, const QString &elementID=QString()) |
Q_INVOKABLE QPixmap | pixmap (const QString &elementID=QString()) |
Q_ENUM (StyleSheetColor) | |
Q_INVOKABLE void | resize () |
Q_INVOKABLE void | resize (const QSizeF &size) |
Q_INVOKABLE void | resize (qreal width, qreal height) |
void | setColor (StyleSheetColor colorName, const QColor &color) |
void | setColorSet (ColorSet colorSet) |
void | setContainsMultipleImages (bool multiple) |
void | setDevicePixelRatio (qreal factor) |
void | setImageSet (KSvg::ImageSet *theme) |
void | setStatus (Svg::Status status) |
void | setUsingRenderingCache (bool useCache) |
QSizeF | size () const |
Svg::Status | status () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Related Symbols | |
(Note that these are not member symbols.) | |
FrameSvg (QObject *parent=nullptr) | |
![]() | |
Svg (QObject *parent=nullptr) | |
Additional Inherited Members | |
![]() | |
void | colorHintChanged () |
void | colorSetChanged (KSvg::Svg::ColorSet colorSet) |
void | fromCurrentImageSetChanged (bool fromCurrentImageSet) |
void | imagePathChanged () |
void | imageSetChanged (ImageSet *imageSet) |
void | repaintNeeded () |
void | sizeChanged () |
void | statusChanged (KSvg::Svg::Status status) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Provides an SVG with borders.
When using SVG images for a background of an object that may change its aspect ratio, such as a dialog, simply scaling a single image may not be enough.
FrameSvg allows SVGs to provide several elements for borders as well as a central element, each of which are scaled individually. These elements should be named:
center
- the central element, which will be scaled in both directionstop
- the top border; the height is fixed, but it will be scaled horizontally to the same width ascenter
bottom
- the bottom border; scaled in the same way astop
left
- the left border; the width is fixed, but it will be scaled vertically to the same height ascenter
right
- the right border; scaled in the same way asleft
topleft
- fixed size; must be the same height astop
and the same width asleft
bottomleft
,topright
,bottomright
- similar totopleft
center
must exist, but all the others are optional. topleft
and topright
will be ignored if top
does not exist, and similarly for bottomleft
and bottomright
.
- See also
- KSvg::Svg
Definition at line 60 of file framesvg.h.
Member Typedef Documentation
◆ EnabledBorders
typedef QFlags< EnabledBorder > KSvg::FrameSvg::EnabledBorders |
Definition at line 78 of file framesvg.h.
Member Enumeration Documentation
◆ EnabledBorder
This flag enum specifies which borders should be drawn.
Definition at line 70 of file framesvg.h.
◆ LocationPrefix
Definition at line 82 of file framesvg.h.
◆ MarginEdge
Enumerator | |
---|---|
TopMargin | The top margin. |
BottomMargin | The bottom margin. |
LeftMargin | The left margin. |
RightMargin | The right margin. |
Definition at line 91 of file framesvg.h.
Property Documentation
◆ enabledBorders
|
readwrite |
Definition at line 64 of file framesvg.h.
Constructor & Destructor Documentation
◆ ~FrameSvg()
|
override |
Definition at line 50 of file framesvg.cpp.
Member Function Documentation
◆ actualPrefix()
QString KSvg::FrameSvg::actualPrefix | ( | ) | const |
This method returns the prefix that is actually being used (including a '-' at the end if not empty).
- See also
- prefix()
Definition at line 994 of file framesvg.cpp.
◆ alphaMask()
QPixmap KSvg::FrameSvg::alphaMask | ( | ) | const |
This method returns a pixmap whose alpha channel is the opacity of the frame.
It may be the frame itself or a special frame with the "mask-" prefix.
Definition at line 324 of file framesvg.cpp.
◆ cacheAllRenderedFrames()
bool KSvg::FrameSvg::cacheAllRenderedFrames | ( | ) | const |
This method returns whether all the different prefixes should be kept in a cache when rendered.
Definition at line 369 of file framesvg.cpp.
◆ clearCache()
void KSvg::FrameSvg::clearCache | ( | ) |
This method deletes the internal cache.
Calling this method frees memeory. Use this if you want to switch the rendered element and you don't plan to switch back to the previous one for a long time.
This only works if setUsingRenderingCache(true
) has been called.
Definition at line 374 of file framesvg.cpp.
◆ contentsRect()
QRectF KSvg::FrameSvg::contentsRect | ( | ) | const |
This method returns the rectangle of the center element, taking the margins into account.
Definition at line 314 of file framesvg.cpp.
◆ enabledBorders()
FrameSvg::EnabledBorders KSvg::FrameSvg::enabledBorders | ( | ) | const |
This is a convenience method to get the enabled borders.
- Returns
- what borders are painted
Definition at line 83 of file framesvg.cpp.
◆ fixedMarginSize()
qreal KSvg::FrameSvg::fixedMarginSize | ( | const FrameSvg::MarginEdge | edge | ) | const |
This method returns the margin size for the specified edge.
Compared to marginSize(), this does not depend on whether the margin is enabled or not.
- Parameters
-
edge the margin edge we want, top, bottom, left or right
- Returns
- the margin size
Definition at line 249 of file framesvg.cpp.
◆ framePixmap()
QPixmap KSvg::FrameSvg::framePixmap | ( | ) |
This method returns a pixmap of the SVG represented by this object.
- Parameters
-
elelementId the ID string of the element to render, or an empty string for the whole SVG (the default).
- Returns
- a QPixmap of the rendered SVG
Definition at line 386 of file framesvg.cpp.
◆ frameSize()
QSizeF KSvg::FrameSvg::frameSize | ( | ) | const |
- Returns
- the size of the frame
Definition at line 188 of file framesvg.cpp.
◆ getFixedMargins()
void KSvg::FrameSvg::getFixedMargins | ( | qreal & | left, |
qreal & | top, | ||
qreal & | right, | ||
qreal & | bottom ) const |
This is a convenience method that extracts the size of the four margins and saves their size into the passed variables.
Compared to getMargins(), this doesn't depend on whether the margins are enabled or not.
- Parameters
-
left left margin size top top margin size right right margin size bottom bottom margin size
Definition at line 288 of file framesvg.cpp.
◆ getInset()
void KSvg::FrameSvg::getInset | ( | qreal & | left, |
qreal & | top, | ||
qreal & | right, | ||
qreal & | bottom ) const |
This is a convenience method that extracts the size of the four inset margins and saves their size into the passed variables.
- Parameters
-
left left margin size top top margin size right right margin size bottom bottom margin size
- Since
- 5.77
Definition at line 301 of file framesvg.cpp.
◆ getMargins()
void KSvg::FrameSvg::getMargins | ( | qreal & | left, |
qreal & | top, | ||
qreal & | right, | ||
qreal & | bottom ) const |
This is a convenience method that extracts the size of the four margins and saves their size into the passed variables.
If you don't care about the margins being on or off, use getFixedMargins() instead.
- Parameters
-
left left margin size top top margin size right right margin size bottom bottom margin size
Definition at line 275 of file framesvg.cpp.
◆ hasElementPrefix() [1/2]
bool KSvg::FrameSvg::hasElementPrefix | ( | const QString & | prefix | ) | const |
This method returns whether the SVG has the necessary elements with the given prefix to draw a frame.
- Parameters
-
prefix the given prefix we want to check if drawable (can have trailing '-' since 5.59)
Definition at line 130 of file framesvg.cpp.
◆ hasElementPrefix() [2/2]
bool KSvg::FrameSvg::hasElementPrefix | ( | KSvg::FrameSvg::LocationPrefix | location | ) | const |
This is an overloaded method provided for convenience that is equivalent to hasElementPrefix("north"), hasElementPrefix("south") hasElementPrefix("west") and hasElementPrefix("east").
- Returns
- true if the svg has the necessary elements with the given prefix to draw a frame.
- Parameters
-
location the given prefix we want to check if drawable
Definition at line 144 of file framesvg.cpp.
◆ insetSize()
qreal KSvg::FrameSvg::insetSize | ( | const FrameSvg::MarginEdge | edge | ) | const |
This method returns the insets margin size for the specified edge.
- Parameters
-
edge the margin edge we want, top, bottom, left or right
- Returns
- the margin size
- Since
- 5.77
Definition at line 223 of file framesvg.cpp.
◆ isRepaintBlocked()
bool KSvg::FrameSvg::isRepaintBlocked | ( | ) | const |
This method returns whether we are in a transaction of many changes at once.
This is used to restrict rebuilding generated graphics for each change made.
- Since
- 5.31
Definition at line 999 of file framesvg.cpp.
◆ marginSize()
qreal KSvg::FrameSvg::marginSize | ( | const FrameSvg::MarginEdge | edge | ) | const |
This method returns the margin size for the given edge.
Note that 0
will be returned if the given margin is disabled.
If you don't care about the margin being on or off, use fixedMarginSize() instead.
- Parameters
-
edge the margin edge we want, top, bottom, left or right
- Returns
- the margin size
Definition at line 197 of file framesvg.cpp.
◆ mask()
QRegion KSvg::FrameSvg::mask | ( | ) | const |
This method returns a mask that tightly contains the fully opaque areas of the SVG.
- Returns
- a region of opaque areas
Definition at line 330 of file framesvg.cpp.
◆ minimumDrawingHeight()
int KSvg::FrameSvg::minimumDrawingHeight | ( | ) |
This method returns the minimum height required to correctly draw this SVG.
- Since
- 5.101
Definition at line 419 of file framesvg.cpp.
◆ minimumDrawingWidth()
int KSvg::FrameSvg::minimumDrawingWidth | ( | ) |
This method returns the minimum width required to correctly draw this SVG.
- Since
- 5.101
Definition at line 427 of file framesvg.cpp.
◆ paintFrame() [1/2]
This method paints the loaded SVG with the elements that represents the border.
This is an overloaded member provided for convenience
- Parameters
-
painter the QPainter to use pos where to paint the svg
Definition at line 407 of file framesvg.cpp.
◆ paintFrame() [2/2]
void KSvg::FrameSvg::paintFrame | ( | QPainter * | painter, |
const QRectF & | target, | ||
const QRectF & | source = QRectF() ) |
This method paints the loaded SVG with the elements that represents the border.
- Parameters
-
painter the QPainter to use target the target rectangle on the paint device source the portion rectangle of the source image
Definition at line 395 of file framesvg.cpp.
◆ prefix()
QString KSvg::FrameSvg::prefix | ( | ) |
This method returns the prefix for SVG elements of the FrameSvg (including a '-' at the end if not empty).
- Returns
- the prefix
- See also
- actualPrefix()
Definition at line 160 of file framesvg.cpp.
◆ resizeFrame()
void KSvg::FrameSvg::resizeFrame | ( | const QSizeF & | size | ) |
This method resizes the frame, maintaining the same border size.
- Parameters
-
size the new size of the frame
Definition at line 165 of file framesvg.cpp.
◆ setCacheAllRenderedFrames()
void KSvg::FrameSvg::setCacheAllRenderedFrames | ( | bool | cache | ) |
This method sets whether saving all the rendered prefixes in a cache or not.
- Parameters
-
cache whether to use the cache.
Definition at line 360 of file framesvg.cpp.
◆ setElementPrefix() [1/2]
void KSvg::FrameSvg::setElementPrefix | ( | const QString & | prefix | ) |
This method sets the prefix for the SVG elements to be used for painting.
For example, if prefix is 'active', then instead of using the 'top' element of the SVG file to paint the top border, the 'active-top' element will be used. The same goes for other SVG elements.
If the elements with prefixes are not present, the default ones are used. (for the sake of speed, the test is present only for the 'center' element)
Setting the prefix manually resets the location to Floating.
The prefix must exist in the SVG document, which means that this can only be called successfully after setImagePath is called.
- Parameters
-
prefix prefix for the SVG elements that make up the frame
Definition at line 111 of file framesvg.cpp.
◆ setElementPrefix() [2/2]
void KSvg::FrameSvg::setElementPrefix | ( | KSvg::FrameSvg::LocationPrefix | location | ) |
This method sets the prefix (.
- See also
- setElementPrefix) to 'north', 'south', 'west' and 'east' when the location is TopEdge, BottomEdge, LeftEdge and RightEdge, respectively. Clears the prefix in other cases.
The prefix must exist in the SVG document, which means that this can only be called successfully after setImagePath is called.
- Parameters
-
location location in the UI this frame will be drawn
Definition at line 88 of file framesvg.cpp.
◆ setEnabledBorders()
void KSvg::FrameSvg::setEnabledBorders | ( | const EnabledBorders | borders | ) |
This method sets which borders should be painted.
- Parameters
-
flags borders we want to paint
- See also
- EnabledBorder
- Parameters
-
borders
Definition at line 70 of file framesvg.cpp.
◆ setImagePath()
|
overridevirtual |
Loads a new Svg.
- Parameters
-
imagePath the new file
Reimplemented from KSvg::Svg.
Definition at line 55 of file framesvg.cpp.
◆ setRepaintBlocked()
void KSvg::FrameSvg::setRepaintBlocked | ( | bool | blocked | ) |
This method sets whether we should block rebuilding generated graphics for each change made.
Setting this to true
will block rebuilding the generated graphics for each change made and will do these changes in blocks instead.
How to use this method: When making several changes at once to the frame properties–such as prefix, enabled borders, and size–set this property to true to avoid regenerating the graphics for each change. Set it to false again after applying all required changes.
Note that any change will not be visible in the painted frame while this property is set to true.
- Since
- 5.31
Definition at line 1004 of file framesvg.cpp.
Friends And Related Symbol Documentation
◆ FrameSvg()
|
related |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Apr 27 2024 22:10:42 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.