KSvg::Svg
#include <KSvg/Svg>
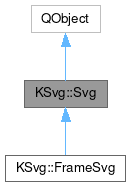
Properties | |
KSvg::Svg::ColorSet | colorSet |
bool | fromCurrentImageSet |
QString | imagePath |
bool | multipleImages |
QSizeF | size |
KSvg::Svg::Status | status |
bool | usingRenderingCache |
![]() | |
objectName | |
Signals | |
void | colorHintChanged () |
void | colorSetChanged (KSvg::Svg::ColorSet colorSet) |
void | fromCurrentImageSetChanged (bool fromCurrentImageSet) |
void | imagePathChanged () |
void | imageSetChanged (ImageSet *imageSet) |
void | repaintNeeded () |
void | sizeChanged () |
void | statusChanged (KSvg::Svg::Status status) |
Public Member Functions | |
void | clearColorOverrides () |
QColor | color (StyleSheetColor colorName) const |
KSvg::Svg::ColorSet | colorSet () const |
bool | containsMultipleImages () const |
qreal | devicePixelRatio () const |
Q_INVOKABLE QRectF | elementRect (const QString &elementId) const |
QRectF | elementRect (QStringView elementId) const |
Q_INVOKABLE QSizeF | elementSize (const QString &elementId) const |
QSizeF | elementSize (QStringView elementId) const |
bool | fromCurrentImageSet () const |
Q_INVOKABLE bool | hasElement (const QString &elementId) const |
bool | hasElement (QStringView elementId) const |
Q_INVOKABLE QImage | image (const QSize &size, const QString &elementID=QString()) |
QString | imagePath () const |
ImageSet * | imageSet () const |
bool | isUsingRenderingCache () const |
Q_INVOKABLE bool | isValid () const |
Q_INVOKABLE void | paint (QPainter *painter, const QPointF &point, const QString &elementID=QString()) |
Q_INVOKABLE void | paint (QPainter *painter, const QRectF &rect, const QString &elementID=QString()) |
Q_INVOKABLE void | paint (QPainter *painter, int x, int y, const QString &elementID=QString()) |
Q_INVOKABLE void | paint (QPainter *painter, int x, int y, int width, int height, const QString &elementID=QString()) |
Q_INVOKABLE QPixmap | pixmap (const QString &elementID=QString()) |
Q_ENUM (StyleSheetColor) | |
Q_INVOKABLE void | resize () |
Q_INVOKABLE void | resize (const QSizeF &size) |
Q_INVOKABLE void | resize (qreal width, qreal height) |
void | setColor (StyleSheetColor colorName, const QColor &color) |
void | setColorSet (ColorSet colorSet) |
void | setContainsMultipleImages (bool multiple) |
void | setDevicePixelRatio (qreal factor) |
virtual void | setImagePath (const QString &svgFilePath) |
void | setImageSet (KSvg::ImageSet *theme) |
void | setStatus (Svg::Status status) |
void | setUsingRenderingCache (bool useCache) |
QSizeF | size () const |
Svg::Status | status () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Related Symbols | |
(Note that these are not member symbols.) | |
Svg (QObject *parent=nullptr) | |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
A theme aware image-centric SVG class.
KSvg::Svg provides a class for rendering SVG images to a QPainter in a convenient manner. Unless an absolute path to a file is provided, it loads the SVG document using KSvg::ImageSet. It also provides a number of internal optimizations to help lower the cost of painting SVGs, such as caching.
- See also
- KSvg::FrameSvg
Member Enumeration Documentation
◆ ColorSet
◆ Status
◆ StyleSheetColor
Property Documentation
◆ colorSet
◆ fromCurrentImageSet
◆ imagePath
◆ multipleImages
◆ size
◆ status
◆ usingRenderingCache
Constructor & Destructor Documentation
◆ ~Svg()
Member Function Documentation
◆ clearColorOverrides()
◆ color()
◆ colorHintChanged
|
signal |
This signal is emitted whenever the color hint has changed.
◆ colorSet()
Svg::ColorSet KSvg::Svg::colorSet | ( | ) | const |
◆ colorSetChanged
|
signal |
This signal is emitted when the color set has changed.
◆ containsMultipleImages()
bool KSvg::Svg::containsMultipleImages | ( | ) | const |
This method returns whether the SVG contains multiple images.
If this is true
, the SVG will be treated as a collection of related images, rather than a consistent drawing.
- Returns
true
if the SVG will be treated as containing multiple images,false
if it will be treated as a coherent image.
◆ devicePixelRatio()
qreal KSvg::Svg::devicePixelRatio | ( | ) | const |
◆ elementRect() [1/2]
This method returns the bounding rect of a given element.
This is the bounding rect of the element with ID elementId
after the SVG has been scaled (see resize()). Note that this is unaffected by the containsMultipleImages property.
- Parameters
-
elementId the id of the element to check
- Returns
- the current rect of a given element, given the current size of the SVG
◆ elementRect() [2/2]
QRectF KSvg::Svg::elementRect | ( | QStringView | elementId | ) | const |
◆ elementSize() [1/2]
This method returns the size of a given element.
This is the size of the element with ID elementId
after the SVG has been scaled (see resize()). Note that this is unaffected by the containsMultipleImages property.
- Parameters
-
elementId the id of the element to check
- Returns
- the size of a given element, given the current size of the SVG
◆ elementSize() [2/2]
QSizeF KSvg::Svg::elementSize | ( | QStringView | elementId | ) | const |
◆ fromCurrentImageSet()
bool KSvg::Svg::fromCurrentImageSet | ( | ) | const |
This method returns whether the current theme has this SVG, without having to fall back to the default theme.
- Returns
- true if the svg is loaded from the current theme
◆ fromCurrentImageSetChanged
|
signal |
This signal is emitted when the value of fromCurrentImageSet() has changed.
◆ hasElement() [1/2]
bool KSvg::Svg::hasElement | ( | const QString & | elementId | ) | const |
◆ hasElement() [2/2]
bool KSvg::Svg::hasElement | ( | QStringView | elementId | ) | const |
◆ image()
This method returns an image of the SVG represented by this object.
The size of the image will be the size of this Svg object (size()) if containsMultipleImages is true
; otherwise, it will be the size of the requested element after the whole SVG has been scaled to size().
- Parameters
-
elementId the ID string of the element to render, or an empty string for the whole SVG (the default)
- Returns
- a QPixmap of the rendered SVG
◆ imagePath()
QString KSvg::Svg::imagePath | ( | ) | const |
This method returns the SVG file to render.
If this SVG is themed, this will be a relative path, and will not include a file extension.
- Returns
- either an absolute path to an SVG file, or an image name
- See also
- ImageSet::imagePath()
◆ imagePathChanged
|
signal |
This signal is emitted whenever the image path has changed.
◆ imageSet()
ImageSet * KSvg::Svg::imageSet | ( | ) | const |
This method returns the KSvg::ImageSet used by this Svg object.
This determines how relative image paths are interpreted.
- Returns
- the theme used by this Svg
◆ imageSetChanged
|
signal |
This signal is emitted when the image set has changed.
◆ isUsingRenderingCache()
bool KSvg::Svg::isUsingRenderingCache | ( | ) | const |
◆ isValid()
bool KSvg::Svg::isValid | ( | ) | const |
◆ paint() [1/4]
void KSvg::Svg::paint | ( | QPainter * | painter, |
const QPointF & | point, | ||
const QString & | elementID = QString() ) |
This method paints all or part of the SVG represented by this object.
The size of the painted area will be the size of this Svg object (size()) if containsMultipleImages is true
; otherwise, it will be the size of the requested element after the whole SVG has been scaled to size().
- Parameters
-
painter the QPainter to use point the position to start drawing; the entire svg will be drawn starting at this point. elementId the ID string of the element to render, or an empty string for the whole SVG (the default)
◆ paint() [2/4]
void KSvg::Svg::paint | ( | QPainter * | painter, |
const QRectF & | rect, | ||
const QString & | elementID = QString() ) |
This method paints all or part of the SVG represented by this object.
- Parameters
-
painter the QPainter to use rect the rect to draw into; if smaller than the current size the drawing is starting at this point. elementId the ID string of the element to render, or an empty string for the whole SVG (the default)
◆ paint() [3/4]
This method paints all or part of the SVG represented by this object.
The size of the painted area will be the size of this Svg object (size()) if containsMultipleImages is true
; otherwise, it will be the size of the requested element after the whole SVG has been scaled to size().
- Parameters
-
painter the QPainter to use x the horizontal coordinate to start painting from y the vertical coordinate to start painting from elementId the ID string of the element to render, or an empty string for the whole SVG (the default)
◆ paint() [4/4]
void KSvg::Svg::paint | ( | QPainter * | painter, |
int | x, | ||
int | y, | ||
int | width, | ||
int | height, | ||
const QString & | elementID = QString() ) |
This method paints all or part of the SVG represented by this object.
- Parameters
-
painter the QPainter to use x the horizontal coordinate to start painting from y the vertical coordinate to start painting from width the width of the element to draw height the height of the element do draw elementId the ID string of the element to render, or an empty string for the whole SVG (the default)
◆ pixmap()
This method returns a pixmap of the SVG represented by this object.
The size of the pixmap will be the size of this Svg object (size()) if containsMultipleImages is true
; otherwise, it will be the size of the requested element after the whole SVG has been scaled to size().
- Parameters
-
elementId the ID string of the element to render, or an empty string for the whole SVG (the default)
- Returns
- a QPixmap of the rendered SVG
◆ repaintNeeded
|
signal |
This signal is emitted whenever the SVG data has changed in such a way that a repaint is required.
Any usage of an SVG object that does the painting itself must connect to this signal and respond by updating the painting. Note that connecting to ImageSet::imageSetChanged is incorrect in such a use case as the SVG itself may not be updated yet nor may theme change be the only case when a repaint is needed. Also note that classes or QML code which take Svg objects as parameters for their own painting all respond to this signal so that in those cases manually responding to the signal is unnecessary; ONLY when direct, manual painting with an Svg object is done in application code is this signal used.
◆ resize() [1/3]
void KSvg::Svg::resize | ( | ) |
◆ resize() [2/3]
void KSvg::Svg::resize | ( | const QSizeF & | size | ) |
This method resizes the rendered image.
Rendering will actually take place on the next call to paint.
If containsMultipleImages is true
, each element of the SVG will be rendered at this size by default; otherwise, the entire image will be scaled to this size and each element will be scaled appropriately.
- Parameters
-
size the new size of the image
◆ resize() [3/3]
void KSvg::Svg::resize | ( | qreal | width, |
qreal | height ) |
This method resizes the rendered image.
Rendering will actually take place on the next call to paint.
If containsMultipleImages is true
, each element of the SVG will be rendered at this size by default; otherwise, the entire image will be scaled to this size and each element will be scaled appropriately.
- Parameters
-
width the new width height the new height
◆ setColor()
void KSvg::Svg::setColor | ( | StyleSheetColor | colorName, |
const QColor & | color ) |
◆ setColorSet()
void KSvg::Svg::setColorSet | ( | ColorSet | colorSet | ) |
◆ setContainsMultipleImages()
void KSvg::Svg::setContainsMultipleImages | ( | bool | multiple | ) |
This method sets whether the SVG contains a single image or multiple ones.
If this is set to true
, the SVG will be treated as a collection of related images, rather than a consistent drawing.
In particular, when individual elements are rendered, this affects whether the elements are resized to size() by default. See paint() and pixmap().
- Parameters
-
multiple true if the svg contains multiple images
◆ setDevicePixelRatio()
void KSvg::Svg::setDevicePixelRatio | ( | qreal | factor | ) |
This method sets the device pixel ratio for the Svg.
This is the ratio between image pixels and device-independent pixels. The SVG will produce pixmaps scaled by devicePixelRatio, but all the sizes and element rects will not be altered. The default value is 1.0 and the scale will be done rounded to the floor integer.
Setting it to something higher will make all the elements of this SVG appear bigger.
◆ setImagePath()
|
virtual |
This method sets the SVG file to render.
Relative paths are looked for in the current Svg theme, and should not include the file extension (.svg and .svgz files will be searched for). include the file extension; files with the .svg and .svgz extensions will be found automatically.
- See also
- ImageSet::imagePath()
If the parent object of this Svg is a KSvg::Applet, relative paths will be searched for in the applet's package first.
- Parameters
-
svgFilePath either an absolute path to an SVG file, or an image name.
Reimplemented in KSvg::FrameSvg.
◆ setImageSet()
void KSvg::Svg::setImageSet | ( | KSvg::ImageSet * | theme | ) |
This method sets the KSvg::ImageSet to use with this Svg object.
By default, Svg objects use KSvg::ImageSet::default().
This determines how relative image paths are interpreted.
- Parameters
-
theme the theme object to use
- Since
- 4.3
◆ setStatus()
void KSvg::Svg::setStatus | ( | Svg::Status | status | ) |
This method sets the image in a selected status.
SVGs can be colored with system color themes. If status
is selected, TextColor will become HighlightedText color, and BackgroundColor will become HighlightColor. This can be used to make SVG-based graphics such as symbolic icons look correct together. Supported statuses are Normal and Selected.
- Since
- 5.23
◆ setUsingRenderingCache()
void KSvg::Svg::setUsingRenderingCache | ( | bool | useCache | ) |
This method sets whether or not to cache the results of rendering to pixmaps.
If the SVG is resized and re-rendered often (and does not keep using the same small set of pixmap dimensions), then it may be less efficient to do disk caching. A good example might be a progress meter that uses an Svg object to paint itself: the meter will be changing often enough, with enough unpredictability and without re-use of the previous pixmaps to not get a gain from caching.
Most Svg objects should use the caching feature, however. Therefore, the default is to use the render cache.
- Parameters
-
useCache true to cache rendered pixmaps
- Since
- 4.3
◆ size()
QSizeF KSvg::Svg::size | ( | ) | const |
This method returns the size of the SVG.
If the SVG has been resized with resize(), that size will be returned; otherwise, the natural size of the SVG will be returned.
If containsMultipleImages is true
, each element of the SVG will be rendered at this size by default.
- Returns
- the current size of the SVG
◆ sizeChanged
|
signal |
This signal is emitted whenever the size has changed.
- See also
- resize()
◆ status()
Svg::Status KSvg::Svg::status | ( | ) | const |
◆ statusChanged
|
signal |
This signal is emitted when the status has changed.
Friends And Related Symbol Documentation
◆ Svg()
|
This method constructs an SVG object that implicitly shares and caches rendering.
Unlike QSvgRenderer, which this class uses internally, KSvg::Svg represents an image generated from an SVG. As such, it has a related size and transform matrix (the latter being provided by the painter used to paint the image).
The size is initialized to be the SVG's native size.
- Parameters
-
parent options QObject to parent this to
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:58:01 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.