KFontChooser
#include <KFontChooser>
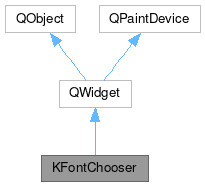
Public Types | |
enum | DisplayFlag { NoDisplayFlags = 0 , FixedFontsOnly = 1 , DisplayFrame = 2 , ShowDifferences = 4 } |
typedef QFlags< DisplayFlag > | DisplayFlags |
enum | FontColumn { FamilyList = 0x01 , StyleList = 0x02 , SizeList = 0x04 } |
enum | FontDiff { NoFontDiffFlags = 0 , FontDiffFamily = 1 , FontDiffStyle = 2 , FontDiffSize = 4 , AllFontDiffs = FontDiffFamily | FontDiffStyle | FontDiffSize } |
typedef QFlags< FontDiff > | FontDiffFlags |
enum | FontListCriteria { FixedWidthFonts = 0x01 , ScalableFonts = 0x02 , SmoothScalableFonts = 0x04 } |
![]() | |
enum | RenderFlag |
![]() | |
enum | PaintDeviceMetric |
Signals | |
void | fontSelected (const QFont &font) |
Public Member Functions | |
KFontChooser (DisplayFlags flags, QWidget *parent=nullptr) | |
KFontChooser (QWidget *parent=nullptr) | |
~KFontChooser () override | |
QColor | backgroundColor () const |
QColor | color () const |
void | enableColumn (int column, bool state) |
QFont | font () const |
FontDiffFlags | fontDiffFlags () const |
QString | sampleText () const |
void | setBackgroundColor (const QColor &col) |
void | setColor (const QColor &col) |
void | setFont (const QFont &font, bool onlyFixed=false) |
void | setFontListItems (const QStringList &fontList) |
void | setMinVisibleItems (int visibleItems) |
void | setSampleBoxVisible (bool visible) |
void | setSampleText (const QString &text) |
QSize | sizeHint (void) const override |
![]() | |
QWidget (QWidget *parent, Qt::WindowFlags f) | |
bool | acceptDrops () const const |
QString | accessibleDescription () const const |
QString | accessibleName () const const |
QList< QAction * > | actions () const const |
void | activateWindow () |
QAction * | addAction (const QIcon &icon, const QString &text) |
QAction * | addAction (const QIcon &icon, const QString &text, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QIcon &icon, const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text) |
QAction * | addAction (const QString &text, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
void | addAction (QAction *action) |
void | addActions (const QList< QAction * > &actions) |
void | adjustSize () |
bool | autoFillBackground () const const |
QPalette::ColorRole | backgroundRole () const const |
QBackingStore * | backingStore () const const |
QSize | baseSize () const const |
QWidget * | childAt (const QPoint &p) const const |
QWidget * | childAt (int x, int y) const const |
QRect | childrenRect () const const |
QRegion | childrenRegion () const const |
void | clearFocus () |
void | clearMask () |
bool | close () |
QMargins | contentsMargins () const const |
QRect | contentsRect () const const |
Qt::ContextMenuPolicy | contextMenuPolicy () const const |
QCursor | cursor () const const |
void | customContextMenuRequested (const QPoint &pos) |
WId | effectiveWinId () const const |
void | ensurePolished () const const |
Qt::FocusPolicy | focusPolicy () const const |
QWidget * | focusProxy () const const |
QWidget * | focusWidget () const const |
const QFont & | font () const const |
QFontInfo | fontInfo () const const |
QFontMetrics | fontMetrics () const const |
QPalette::ColorRole | foregroundRole () const const |
QRect | frameGeometry () const const |
QSize | frameSize () const const |
const QRect & | geometry () const const |
QPixmap | grab (const QRect &rectangle) |
void | grabGesture (Qt::GestureType gesture, Qt::GestureFlags flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const const |
QGraphicsProxyWidget * | graphicsProxyWidget () const const |
bool | hasEditFocus () const const |
bool | hasFocus () const const |
virtual bool | hasHeightForWidth () const const |
bool | hasMouseTracking () const const |
bool | hasTabletTracking () const const |
int | height () const const |
virtual int | heightForWidth (int w) const const |
void | hide () |
Qt::InputMethodHints | inputMethodHints () const const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, const QList< QAction * > &actions) |
bool | isActiveWindow () const const |
bool | isAncestorOf (const QWidget *child) const const |
bool | isEnabled () const const |
bool | isEnabledTo (const QWidget *ancestor) const const |
bool | isFullScreen () const const |
bool | isHidden () const const |
bool | isMaximized () const const |
bool | isMinimized () const const |
bool | isModal () const const |
bool | isTopLevel () const const |
bool | isVisible () const const |
bool | isVisibleTo (const QWidget *ancestor) const const |
bool | isWindow () const const |
bool | isWindowModified () const const |
QLayout * | layout () const const |
Qt::LayoutDirection | layoutDirection () const const |
QLocale | locale () const const |
void | lower () |
QPoint | mapFrom (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapFrom (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapFromGlobal (const QPoint &pos) const const |
QPointF | mapFromGlobal (const QPointF &pos) const const |
QPoint | mapFromParent (const QPoint &pos) const const |
QPointF | mapFromParent (const QPointF &pos) const const |
QPoint | mapTo (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapTo (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapToGlobal (const QPoint &pos) const const |
QPointF | mapToGlobal (const QPointF &pos) const const |
QPoint | mapToParent (const QPoint &pos) const const |
QPointF | mapToParent (const QPointF &pos) const const |
QRegion | mask () const const |
int | maximumHeight () const const |
QSize | maximumSize () const const |
int | maximumWidth () const const |
int | minimumHeight () const const |
QSize | minimumSize () const const |
virtual QSize | minimumSizeHint () const const |
int | minimumWidth () const const |
void | move (const QPoint &) |
void | move (int x, int y) |
QWidget * | nativeParentWidget () const const |
QWidget * | nextInFocusChain () const const |
QRect | normalGeometry () const const |
void | overrideWindowFlags (Qt::WindowFlags flags) |
virtual QPaintEngine * | paintEngine () const const override |
const QPalette & | palette () const const |
QWidget * | parentWidget () const const |
QPoint | pos () const const |
QWidget * | previousInFocusChain () const const |
QWIDGETSIZE_MAX QWIDGETSIZE_MAX | |
void | raise () |
QRect | rect () const const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | repaint () |
void | repaint (const QRect &rect) |
void | repaint (const QRegion &rgn) |
void | repaint (int x, int y, int w, int h) |
void | resize (const QSize &) |
void | resize (int w, int h) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const const |
QScreen * | screen () const const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setContentsMargins (const QMargins &margins) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus () |
void | setFocus (Qt::FocusReason reason) |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (const QRect &) |
void | setGeometry (int x, int y, int w, int h) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setInputMethodHints (Qt::InputMethodHints hints) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, Qt::WindowFlags f) |
void | setScreen (QScreen *screen) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
void | setStyleSheet (const QString &styleSheet) |
void | setTabletTracking (bool enable) |
void | setToolTip (const QString &) |
void | setToolTipDuration (int msec) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
virtual void | setVisible (bool visible) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlag (Qt::WindowType flag, bool on) |
void | setWindowFlags (Qt::WindowFlags type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (Qt::WindowStates windowState) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const const |
QSize | sizeIncrement () const const |
QSizePolicy | sizePolicy () const const |
void | stackUnder (QWidget *w) |
QString | statusTip () const const |
QStyle * | style () const const |
QString | styleSheet () const const |
bool | testAttribute (Qt::WidgetAttribute attribute) const const |
QString | toolTip () const const |
int | toolTipDuration () const const |
QWidget * | topLevelWidget () const const |
bool | underMouse () const const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | update () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | updateGeometry () |
bool | updatesEnabled () const const |
QRegion | visibleRegion () const const |
QString | whatsThis () const const |
int | width () const const |
QWidget * | window () const const |
QString | windowFilePath () const const |
Qt::WindowFlags | windowFlags () const const |
QWindow * | windowHandle () const const |
QIcon | windowIcon () const const |
void | windowIconChanged (const QIcon &icon) |
QString | windowIconText () const const |
void | windowIconTextChanged (const QString &iconText) |
Qt::WindowModality | windowModality () const const |
qreal | windowOpacity () const const |
QString | windowRole () const const |
Qt::WindowStates | windowState () const const |
QString | windowTitle () const const |
void | windowTitleChanged (const QString &title) |
Qt::WindowType | windowType () const const |
WId | winId () const const |
int | x () const const |
int | y () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
int | colorCount () const const |
int | depth () const const |
qreal | devicePixelRatio () const const |
qreal | devicePixelRatioF () const const |
int | height () const const |
int | heightMM () const const |
int | logicalDpiX () const const |
int | logicalDpiY () const const |
bool | paintingActive () const const |
int | physicalDpiX () const const |
int | physicalDpiY () const const |
int | width () const const |
int | widthMM () const const |
Static Public Member Functions | |
static QStringList | createFontList (uint fontListCriteria) |
![]() | |
QWidget * | createWindowContainer (QWindow *window, QWidget *parent, Qt::WindowFlags flags) |
QWidget * | find (WId id) |
QWidget * | keyboardGrabber () |
QWidget * | mouseGrabber () |
void | setTabOrder (QWidget *first, QWidget *second) |
void | setTabOrder (std::initializer_list< QWidget * > widgets) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Detailed Description
A font selection widget.
While KFontChooser as an ordinary widget can be embedded in custom dialogs and therefore is very flexible, in most cases it is preferable to use the convenience functions in QFontDialog.
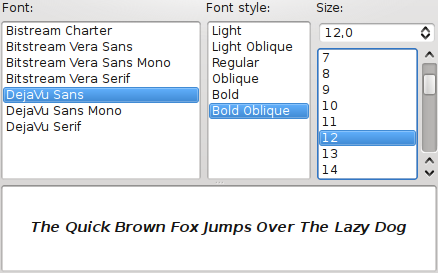
- See also
- KFontRequester
Definition at line 34 of file kfontchooser.h.
Member Typedef Documentation
◆ DisplayFlags
typedef QFlags< DisplayFlag > KFontChooser::DisplayFlags |
Stores a combination of DisplayFlag values.
Definition at line 82 of file kfontchooser.h.
◆ FontDiffFlags
typedef QFlags< FontDiff > KFontChooser::FontDiffFlags |
Stores an combination of FontDiff values.
Definition at line 66 of file kfontchooser.h.
Member Enumeration Documentation
◆ DisplayFlag
Flags for selecting what is displayed in the widget.
- See also
- DisplayFlags
Definition at line 72 of file kfontchooser.h.
◆ FontColumn
Displayed columns.
Enumerator | |
---|---|
FamilyList | Identifies the family (leftmost) list. |
StyleList | Identifies the style (center) list. |
SizeList | Identifies the size (rightmost) list. |
Definition at line 46 of file kfontchooser.h.
◆ FontDiff
Flags for selecting which font attributes to change.
- See also
- FontDiffFlags
Definition at line 56 of file kfontchooser.h.
◆ FontListCriteria
The selection criteria for the font families shown in the dialog.
Definition at line 184 of file kfontchooser.h.
Property Documentation
◆ backgroundColor
|
readwrite |
Definition at line 39 of file kfontchooser.h.
◆ color
|
readwrite |
Definition at line 38 of file kfontchooser.h.
◆ font
|
readwrite |
Definition at line 37 of file kfontchooser.h.
◆ sampleText
|
readwrite |
Definition at line 40 of file kfontchooser.h.
Constructor & Destructor Documentation
◆ KFontChooser() [1/2]
|
explicit |
Constructs a font picker widget.
- Parameters
-
parent the parent widget
- Since
- 5.86
Definition at line 134 of file kfontchooser.cpp.
◆ KFontChooser() [2/2]
|
explicit |
Create a font picker widget.
- Parameters
-
flags a combination of OR-ed values from the KFontChooser::DisplayFlags
enum, the default isDisplayFonts::NoDisplayFlags
parent the parent widget, if not nullptr the windowing system will use it to position the chooser widget relative to it
- Since
- 5.86
Definition at line 141 of file kfontchooser.cpp.
◆ ~KFontChooser()
|
overridedefault |
Destructor.
Member Function Documentation
◆ backgroundColor()
QColor KFontChooser::backgroundColor | ( | ) | const |
Returns the background color currently used in the preview area (default: the base color of the active colorgroup)
Definition at line 312 of file kfontchooser.cpp.
◆ color()
QColor KFontChooser::color | ( | ) | const |
Returns the color currently used for the font in the preview area (default: the text color of the active color group).
Definition at line 299 of file kfontchooser.cpp.
◆ createFontList()
|
static |
Returns a list of font faimly name strings filtered based on fontListCriteria
.
- Parameters
-
fontListCriteria specifies the criteria used to select fonts to add to the list, a combination of OR-ed values from KFontChooser::FontListCriteria
- Since
- 5.86
Definition at line 845 of file kfontchooser.cpp.
◆ enableColumn()
void KFontChooser::enableColumn | ( | int | column, |
bool | state ) |
Enables or disables a column (family, style, size) in the widget.
Use this function if your application does not need or support all font properties.
- Parameters
-
column specify the column(s) to enable/disable, an OR-ed combination of KFontChooser::FontColumn
enum valuesstate if false
the columns are disabled, and vice-versa
Definition at line 337 of file kfontchooser.cpp.
◆ font()
QFont KFontChooser::font | ( | ) | const |
Returns the currently selected font in the chooser.
Definition at line 385 of file kfontchooser.cpp.
◆ fontDiffFlags()
KFontChooser::FontDiffFlags KFontChooser::fontDiffFlags | ( | ) | const |
Returns the bitmask corresponding to the attributes the user wishes to change.
Definition at line 366 of file kfontchooser.cpp.
◆ fontSelected
|
signal |
Emitted when the selected font changes.
◆ sampleText()
QString KFontChooser::sampleText | ( | ) | const |
- Returns
- The current text in the sample text input area.
Definition at line 317 of file kfontchooser.cpp.
◆ setBackgroundColor()
void KFontChooser::setBackgroundColor | ( | const QColor & | col | ) |
Sets the background color to use in the preview area.
Definition at line 304 of file kfontchooser.cpp.
◆ setColor()
void KFontChooser::setColor | ( | const QColor & | col | ) |
Sets the color to use for the font in the preview area.
Definition at line 287 of file kfontchooser.cpp.
◆ setFont()
void KFontChooser::setFont | ( | const QFont & | font, |
bool | onlyFixed = false ) |
Sets the currently selected font in the widget.
- Parameters
-
font the font to select onlyFixed if true
, the font list will only display fixed-width fonts, otherwise all fonts are displayed. The default isfalse
.
Definition at line 351 of file kfontchooser.cpp.
◆ setFontListItems()
void KFontChooser::setFontListItems | ( | const QStringList & | fontList | ) |
Uses fontList
to fill the font family list in the widget.
You can create a custom list of fonts using the static createFontList(uint
criteria)
to only include fonts that meet certain criteria (e.g. only smooth-scalable fonts).
Note that if fontList
is empty, the font list in the chooser will show all the available fonts on the system.
- Since
- 5.86
Definition at line 881 of file kfontchooser.cpp.
◆ setMinVisibleItems()
void KFontChooser::setMinVisibleItems | ( | int | visibleItems | ) |
Sets the minimum number of items that should be visible in the child list widgets; this number will be used to compute and set the minimum heights for those widgets.
- Since
- 5.86
Definition at line 927 of file kfontchooser.cpp.
◆ setSampleBoxVisible()
void KFontChooser::setSampleBoxVisible | ( | bool | visible | ) |
If visible
is true
the preview area will be shown, and vice-versa is it's false
.
Definition at line 327 of file kfontchooser.cpp.
◆ setSampleText()
void KFontChooser::setSampleText | ( | const QString & | text | ) |
Sets the sample text in the preview area; this is useful if you want to use text in your native language.
- Parameters
-
text the new sample text (it will replace the current text)
Definition at line 322 of file kfontchooser.cpp.
◆ sizeHint()
|
overridevirtual |
Reimplemented for internal reasons.
Reimplemented from QWidget.
Definition at line 332 of file kfontchooser.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:54:50 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.