KWindowShadow
#include <kwindowshadow.h>
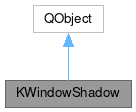
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
The KWindowShadow class represents a drop-shadow that is drawn by the compositor.
The KWindowShadow is composed of multiple tiles. The top left tile, the top right tile, the bottom left tile, and the bottom right tile are rendered as they are. The top tile and the bottom tile are stretched in x direction; the left tile and the right tile are stretched in y direction. Several KWindowShadow objects can share shadow tiles to reduce memory usage. You have to specify padding() along the shadow tiles. The padding values indicate how much the KWindowShadow sticks outside the decorated window.
Once the KWindowShadow is created, you're not allowed to attach or detach any shadow tiles, change padding(), or change window(). In order to do so, you have to destroy() the shadow first, update relevant properties, and create() the shadow again.
Definition at line 79 of file kwindowshadow.h.
Constructor & Destructor Documentation
◆ KWindowShadow()
|
explicit |
Definition at line 57 of file kwindowshadow.cpp.
◆ ~KWindowShadow()
|
override |
Definition at line 63 of file kwindowshadow.cpp.
Member Function Documentation
◆ bottomLeftTile()
KWindowShadowTile::Ptr KWindowShadow::bottomLeftTile | ( | ) | const |
Returns the bottom-left tile attached to the KWindowShadow.
Definition at line 187 of file kwindowshadow.cpp.
◆ bottomRightTile()
KWindowShadowTile::Ptr KWindowShadow::bottomRightTile | ( | ) | const |
Returns the bottom-right tile attached to the KWindowShadow.
Definition at line 153 of file kwindowshadow.cpp.
◆ bottomTile()
KWindowShadowTile::Ptr KWindowShadow::bottomTile | ( | ) | const |
Returns the bottom tile attached to the KWindowShadow.
Definition at line 170 of file kwindowshadow.cpp.
◆ create()
bool KWindowShadow::create | ( | ) |
Allocates the platform resources associated with the KWindowShadow.
Once the native platform resources have been allocated, you're not allowed to attach or detach shadow tiles, change the padding or the target window. If you want to do so, you must destroy() the shadow, change relevant attributes and call create() again.
Returns true
if the creation succeeded, otherwise returns false
.
Definition at line 243 of file kwindowshadow.cpp.
◆ destroy()
void KWindowShadow::destroy | ( | ) |
Releases the platform resources associated with the KWindowShadow.
Calling destroy() after window() had been destroyed will result in a no-op.
Definition at line 261 of file kwindowshadow.cpp.
◆ isCreated()
bool KWindowShadow::isCreated | ( | ) | const |
Returns true
if the platform resources associated with the shadow have been allocated.
Definition at line 238 of file kwindowshadow.cpp.
◆ leftTile()
KWindowShadowTile::Ptr KWindowShadow::leftTile | ( | ) | const |
Returns the left tile attached to the KWindowShadow.
Definition at line 68 of file kwindowshadow.cpp.
◆ padding()
QMargins KWindowShadow::padding | ( | ) | const |
Returns the padding of the KWindowShadow.
The padding values specify the visible extents of the shadow. The top left tile is rendered with an offset of -padding().left() and -padding().top().
Definition at line 204 of file kwindowshadow.cpp.
◆ rightTile()
KWindowShadowTile::Ptr KWindowShadow::rightTile | ( | ) | const |
Returns the right tile attached to the KWindowShadow.
Definition at line 136 of file kwindowshadow.cpp.
◆ setBottomLeftTile()
void KWindowShadow::setBottomLeftTile | ( | KWindowShadowTile::Ptr | tile | ) |
Attaches the bottom-left tile
to the KWindowShadow.
Definition at line 192 of file kwindowshadow.cpp.
◆ setBottomRightTile()
void KWindowShadow::setBottomRightTile | ( | KWindowShadowTile::Ptr | tile | ) |
Attaches the bottom-right tile to the KWindowShadow.
Definition at line 158 of file kwindowshadow.cpp.
◆ setBottomTile()
void KWindowShadow::setBottomTile | ( | KWindowShadowTile::Ptr | tile | ) |
Attaches the bottom tile
to the KWindowShadow.
Definition at line 175 of file kwindowshadow.cpp.
◆ setLeftTile()
void KWindowShadow::setLeftTile | ( | KWindowShadowTile::Ptr | tile | ) |
Attaches the left tile
to the KWindowShadow.
Definition at line 73 of file kwindowshadow.cpp.
◆ setPadding()
void KWindowShadow::setPadding | ( | const QMargins & | padding | ) |
Sets the padding on the KWindowShadow.
If the padding values are smaller than the sizes of the shadow tiles, then the shadow will overlap with the window() and will be rendered behind window(). E.g. if all padding values are set to 0, then the shadow will be completely occluded by the window().
Definition at line 209 of file kwindowshadow.cpp.
◆ setRightTile()
void KWindowShadow::setRightTile | ( | KWindowShadowTile::Ptr | tile | ) |
Attaches the right tile
to the KWindowShadow.
Definition at line 141 of file kwindowshadow.cpp.
◆ setTopLeftTile()
void KWindowShadow::setTopLeftTile | ( | KWindowShadowTile::Ptr | tile | ) |
Attaches the top-left tile
to the KWindowShadow.
Definition at line 90 of file kwindowshadow.cpp.
◆ setTopRightTile()
void KWindowShadow::setTopRightTile | ( | KWindowShadowTile::Ptr | tile | ) |
Attaches the top-right tile
to the KWindowShadow.
Definition at line 124 of file kwindowshadow.cpp.
◆ setTopTile()
void KWindowShadow::setTopTile | ( | KWindowShadowTile::Ptr | tile | ) |
Attaches the top tile
to the KWindowShadow.
Definition at line 107 of file kwindowshadow.cpp.
◆ setWindow()
void KWindowShadow::setWindow | ( | QWindow * | window | ) |
Sets the window behind which the KWindowShadow will be rendered.
Note that the KWindowShadow does not track the platform surface. If for whatever reason the native platform surface is deleted and then created, you must to destroy() the shadow and create() it again yourself.
Definition at line 226 of file kwindowshadow.cpp.
◆ topLeftTile()
KWindowShadowTile::Ptr KWindowShadow::topLeftTile | ( | ) | const |
Returns the top-left tile attached to the KWindowShadow.
Definition at line 85 of file kwindowshadow.cpp.
◆ topRightTile()
KWindowShadowTile::Ptr KWindowShadow::topRightTile | ( | ) | const |
Returns the top-right tile attached to the KWindowShadow.
Definition at line 119 of file kwindowshadow.cpp.
◆ topTile()
KWindowShadowTile::Ptr KWindowShadow::topTile | ( | ) | const |
Returns the top tile attached to the KWindowShadow.
Definition at line 102 of file kwindowshadow.cpp.
◆ window()
QWindow * KWindowShadow::window | ( | ) | const |
Returns the window behind which the KWindowShadow will be rendered.
Definition at line 221 of file kwindowshadow.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:55:23 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.