Akonadi::History
#include <history.h>
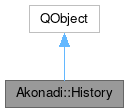
Public Types | |
enum | ResultCode { ResultCodeSuccess = 0 , ResultCodeError } |
Signals | |
void | changed () |
void | redone (Akonadi::History::ResultCode resultCode) |
void | undone (Akonadi::History::ResultCode resultCode) |
Public Slots | |
bool | clear () |
void | redo (QWidget *parent=nullptr) |
void | undo (QWidget *parent=nullptr) |
Public Member Functions | |
~History () override | |
QString | lastErrorString () const |
QString | nextRedoDescription () const |
QString | nextUndoDescription () const |
void | recordCreation (const Akonadi::Item &item, const QString &description, const uint atomicOperationId=0) |
void | recordDeletion (const Akonadi::Item &item, const QString &description, const uint atomicOperationId=0) |
void | recordDeletions (const Akonadi::Item::List &items, const QString &description, const uint atomicOperationId=0) |
void | recordModification (const Akonadi::Item &oldItem, const Akonadi::Item &newItem, const QString &description, const uint atomicOperationId=0) |
bool | redoAvailable () const |
void | undoAll (QWidget *parent=nullptr) |
bool | undoAvailable () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
History class for implementing undo/redo of calendar operations.
Whenever you use IncidenceChanger to create, delete or modify incidences, this class is used to record those changes onto a stack, so they can be undone or redone.
If needed, groupware invitations will be sent to attendees and organizers when undoing or redoing changes.
This class can't be instantiated directly, use it through IncidenceChanger:
- Since
- 4.11
Member Enumeration Documentation
◆ ResultCode
This enum describes the possible result codes (success/error values) for an undo or redo operation.
Enumerator | |
---|---|
ResultCodeSuccess | Success. |
ResultCodeError | An error occurred. Call lastErrorString() for the error message. This isn't very verbose because IncidenceChanger hasn't been refactored yet. |
Constructor & Destructor Documentation
◆ ~History()
|
overridedefault |
Destroys the History instance.
Member Function Documentation
◆ changed
|
signal |
The redo/undo stacks have changed.
◆ clear
|
slot |
Clears the undo and redo stacks.
Won't do anything if there's a undo/redo job currently running.
- Returns
- true if the stacks were cleared, false if there was a job running
Definition at line 119 of file history.cpp.
◆ lastErrorString()
|
nodiscard |
Returns the last error message.
Call this immediately after catching the undone()/redone() signal with an ResultCode != ResultCodeSuccess.
The message is translated.
Definition at line 134 of file history.cpp.
◆ nextRedoDescription()
|
nodiscard |
Returns the description of the next redo.
This is the description that was passed when calling recordCreation(), recordDeletion() or recordModification().
- See also
- nextUndoDescription()
Definition at line 87 of file history.cpp.
◆ nextUndoDescription()
|
nodiscard |
Returns the description of the next undo.
This is the description that was passed when calling recordCreation(), recordDeletion() or recordModification().
- See also
- nextRedoDescription()
Definition at line 78 of file history.cpp.
◆ recordCreation()
void History::recordCreation | ( | const Akonadi::Item & | item, |
const QString & | description, | ||
const uint | atomicOperationId = 0 ) |
Pushes an incidence creation onto the undo stack.
The creation can be undone calling undo().
- Parameters
-
item the item that was created. Must be valid and have a Incidence::Ptr payload description text that can be used in the undo/redo menu item to describe the operation If empty, a default one will be provided. atomicOperationId if not 0, specifies which group of changes this change belongs to. When a change is undone/redone, all other changes which are in the same group are also undone/redone
Definition at line 32 of file history.cpp.
◆ recordDeletion()
void History::recordDeletion | ( | const Akonadi::Item & | item, |
const QString & | description, | ||
const uint | atomicOperationId = 0 ) |
Pushes an incidence deletion onto the undo stack.
The deletion can be undone calling undo().
- Parameters
-
item The item to delete. Must be valid, doesn't need to contain a payload. description text that can be used in the undo/redo menu item to describe the operation If empty, a default one will be provided. atomicOperationId if not 0, specifies which group of changes this change belongs to. When a change is undone/redone, all other changes which are in the same group are also undone/redone
Definition at line 57 of file history.cpp.
◆ recordDeletions()
void History::recordDeletions | ( | const Akonadi::Item::List & | items, |
const QString & | description, | ||
const uint | atomicOperationId = 0 ) |
Pushes a list of incidence deletions onto the undo stack.
The deletions can be undone calling undo() once.
- Parameters
-
items The list of items to delete. All items must be valid. description text that can be used in the undo/redo menu item to describe the operation If empty, a default one will be provided. atomicOperationId If != 0, specifies which group of changes thischange belongs to. Will be useful for atomic undoing/redoing, not implemented yet.
Definition at line 65 of file history.cpp.
◆ recordModification()
void History::recordModification | ( | const Akonadi::Item & | oldItem, |
const Akonadi::Item & | newItem, | ||
const QString & | description, | ||
const uint | atomicOperationId = 0 ) |
Pushes an incidence modification onto the undo stack.
The modification can be undone calling undo().
- Parameters
-
oldItem item containing the payload before the change. Must be valid and contain an Incidence::Ptr payload. newItem item containing the new payload. Must be valid and contain an Incidence::Ptr payload. description text that can be used in the undo/redo menu item to describe the operation If empty, a default one will be provided. atomicOperationId if not 0, specifies which group of changes this change belongs to. When a change is undone/redone, all other changes which are in the same group are also undone/redone
Definition at line 43 of file history.cpp.
◆ redo
|
slot |
Reverts the change that's on top of the redo stack.
Can't be called if there's an undo/redo operation running, asserts. Can be called if the stack is empty, in this case, nothing happens. This function is async, catch signal redone() to know when the operation finishes.
- Parameters
-
parent will be passed to dialogs created by IncidenceChanger, for example those asking if you want to send invitations.
Definition at line 101 of file history.cpp.
◆ redoAvailable()
|
nodiscard |
Returns true if there are changes that can be redone.
Definition at line 144 of file history.cpp.
◆ redone
|
signal |
This signal is emitted when an redo operation finishes.
- Parameters
-
resultCode History::ResultCodeSuccess on success.
- See also
- lastErrorString()
◆ undo
|
slot |
Reverts the change that's on top of the undo stack.
Can't be called if there's an undo/redo operation running, asserts. Can be called if the stack is empty, in this case, nothing happens. This function is async, catch signal undone() to know when the operation finishes.
- Parameters
-
parent will be passed to dialogs created by IncidenceChanger, for example those asking if you want to send invitations.
Definition at line 96 of file history.cpp.
◆ undoAll()
void History::undoAll | ( | QWidget * | parent = nullptr | ) |
Reverts every change in the undo stack.
- Parameters
-
parent will be passed to dialogs created by IncidenceChanger, for example those asking if you want to send invitations.
Definition at line 106 of file history.cpp.
◆ undoAvailable()
|
nodiscard |
Returns true if there are changes that can be undone.
Definition at line 139 of file history.cpp.
◆ undone
|
signal |
This signal is emitted when an undo operation finishes.
- Parameters
-
resultCode History::ResultCodeSuccess on success.
- See also
- lastErrorString()
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Mar 28 2025 11:54:26 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.