Akonadi::ItemSync
#include <itemsync.h>
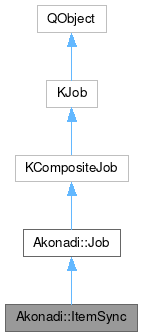
Public Types | |
enum | MergeMode { RIDMerge , GIDMerge } |
enum | TransactionMode { SingleTransaction , MultipleTransactions , NoTransaction } |
![]() | |
enum | Error { ConnectionFailed = UserDefinedError , ProtocolVersionMismatch , UserCanceled , Unknown , UserError = UserDefinedError + 42 } |
using | List = QList<Job *> |
![]() | |
typedef QFlags< Capability > | Capabilities |
enum | Capability |
enum | Unit |
Signals | |
void | readyForNextBatch (int remainingBatchSize) |
void | transactionCommitted () |
![]() | |
void | aboutToStart (Akonadi::Job *job) |
void | writeFinished (Akonadi::Job *job) |
![]() | |
void | description (KJob *job, const QString &title, const QPair< QString, QString > &field1=QPair< QString, QString >(), const QPair< QString, QString > &field2=QPair< QString, QString >()) |
void | finished (KJob *job) |
void | infoMessage (KJob *job, const QString &message) |
void | percentChanged (KJob *job, unsigned long percent) |
void | processedAmountChanged (KJob *job, KJob::Unit unit, qulonglong amount) |
void | processedSize (KJob *job, qulonglong size) |
void | result (KJob *job) |
void | resumed (KJob *job) |
void | speed (KJob *job, unsigned long speed) |
void | suspended (KJob *job) |
void | totalAmountChanged (KJob *job, KJob::Unit unit, qulonglong amount) |
void | totalSize (KJob *job, qulonglong size) |
void | warning (KJob *job, const QString &message) |
Public Member Functions | |
ItemSync (const Collection &collection, const QDateTime ×tamp={}, QObject *parent=nullptr) | |
~ItemSync () override | |
int | batchSize () const |
void | deliveryDone () |
MergeMode | mergeMode () const |
void | rollback () |
void | setBatchSize (int) |
void | setDisableAutomaticDeliveryDone (bool disable) |
void | setFullSyncItems (const Item::List &items) |
void | setIncrementalSyncItems (const Item::List &changedItems, const Item::List &removedItems) |
void | setMergeMode (MergeMode mergeMode) |
void | setStreamingEnabled (bool enable) |
void | setTotalItems (int amount) |
void | setTransactionMode (TransactionMode mode) |
![]() | |
Job (QObject *parent=nullptr) | |
~Job () override | |
QString | errorString () const final |
void | start () override |
![]() | |
KCompositeJob (QObject *parent=nullptr) | |
![]() | |
KJob (QObject *parent=nullptr) | |
Capabilities | capabilities () const |
qint64 | elapsedTime () const |
int | error () const |
QString | errorText () const |
bool | exec () |
bool | isAutoDelete () const |
bool | isFinishedNotificationHidden () const |
bool | isStartedWithExec () const |
bool | isSuspended () const |
unsigned long | percent () const |
Q_SCRIPTABLE qulonglong | processedAmount (Unit unit) const |
void | setAutoDelete (bool autodelete) |
void | setFinishedNotificationHidden (bool hide=true) |
void | setUiDelegate (KJobUiDelegate *delegate) |
Q_SCRIPTABLE qulonglong | totalAmount (Unit unit) const |
KJobUiDelegate * | uiDelegate () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
void | doStart () override |
void | slotResult (KJob *job) override |
![]() | |
bool | addSubjob (KJob *job) override |
virtual bool | doHandleResponse (qint64 tag, const Protocol::CommandPtr &response) |
bool | doKill () override |
void | emitWriteFinished () |
bool | removeSubjob (KJob *job) override |
![]() | |
void | clearSubjobs () |
bool | hasSubjobs () const |
const QList< KJob * > & | subjobs () const |
![]() | |
virtual bool | doResume () |
virtual bool | doSuspend () |
void | emitPercent (qulonglong processedAmount, qulonglong totalAmount) |
void | emitResult () |
void | emitSpeed (unsigned long speed) |
bool | isFinished () const |
void | setCapabilities (Capabilities capabilities) |
void | setError (int errorCode) |
void | setErrorText (const QString &errorText) |
void | setPercent (unsigned long percentage) |
void | setProcessedAmount (Unit unit, qulonglong amount) |
void | setProgressUnit (Unit unit) |
void | setTotalAmount (Unit unit, qulonglong amount) |
void | startElapsedTimer () |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
bool | kill (KJob::KillVerbosity verbosity=KJob::Quietly) |
bool | resume () |
bool | suspend () |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
Bytes | |
Directories | |
Files | |
Items | |
Killable | |
NoCapabilities | |
Suspendable | |
UnitsCount | |
![]() | |
typedef | QObjectList |
![]() | |
void | slotResult (KJob *job) override |
![]() | |
virtual void | slotInfoMessage (KJob *job, const QString &message) |
Detailed Description
Syncs between items known to a client (usually a resource) and the Akonadi storage.
Remote Id must only be set by the resource storing the item, other clients should leave it empty, since the resource responsible for the target collection will be notified about the addition and then create a suitable remote Id.
There are two different forms of ItemSync usage:
- Full-Sync: meaning the client provides all valid items, i.e. any item not part of the list but currently stored in Akonadi will be removed
- Incremental-Sync: meaning the client provides two lists, one for items which are new or modified and one for items which should be removed. Any item not part of either list but currently stored in Akonadi will not be changed.
- Note
- This is provided for convenience to implement "save all" like behavior, however it is strongly recommended to use single item jobs whenever possible, e.g. ItemCreateJob, ItemModifyJob and ItemDeleteJob
Definition at line 40 of file itemsync.h.
Member Enumeration Documentation
◆ MergeMode
enum Akonadi::ItemSync::MergeMode |
Definition at line 45 of file itemsync.h.
◆ TransactionMode
Transaction mode used by ItemSync.
- Since
- 4.6
Definition at line 129 of file itemsync.h.
Constructor & Destructor Documentation
◆ ItemSync()
|
explicit |
Creates a new item synchronizer.
- Parameters
-
collection The collection we are syncing. timestamp Optional timestamp of itemsync start. Will be used to detect local changes that happen while the ItemSync is running. parent The parent object.
Definition at line 183 of file itemsync.cpp.
◆ ~ItemSync()
|
override |
Destroys the item synchronizer.
Definition at line 194 of file itemsync.cpp.
Member Function Documentation
◆ batchSize()
|
nodiscard |
Minimum number of items required to start processing in streaming mode.
When MultipleTransactions is used, one transaction per batch will be created.
- See also
- setBatchSize()
- Since
- 4.14
Definition at line 533 of file itemsync.cpp.
◆ deliveryDone()
void ItemSync::deliveryDone | ( | ) |
Notify ItemSync that all remote items have been delivered.
Only call this in streaming mode.
Definition at line 491 of file itemsync.cpp.
◆ doStart()
|
overrideprotectedvirtual |
This method must be reimplemented in the concrete jobs.
It will be called after the job has been started and a connection to the Akonadi backend has been established.
Implements Akonadi::Job.
Definition at line 269 of file itemsync.cpp.
◆ mergeMode()
|
nodiscard |
Returns current merge mode.
- See also
- setMergeMode()
- Since
- 5.1
Definition at line 545 of file itemsync.cpp.
◆ readyForNextBatch
|
signal |
Signals the resource that new items can be delivered.
- Parameters
-
remainingBatchSize the number of items required to complete the batch (typically the same as batchSize())
- Since
- 4.14
◆ rollback()
void ItemSync::rollback | ( | ) |
Aborts the sync process and rolls back all not yet committed transactions.
Use this if an external error occurred during the sync process (such as the user canceling it).
- Since
- 4.5
Definition at line 515 of file itemsync.cpp.
◆ setBatchSize()
void ItemSync::setBatchSize | ( | int | size | ) |
Set the batch size.
The default is 10.
- Note
- You must call this method before starting the sync, changes afterwards lead to undefined results.
- See also
- batchSize()
- Since
- 4.14
Definition at line 539 of file itemsync.cpp.
◆ setDisableAutomaticDeliveryDone()
void ItemSync::setDisableAutomaticDeliveryDone | ( | bool | disable | ) |
Disables the automatic completion of the item sync, based on the number of delivered items.
This ensures that the item sync only finishes once deliveryDone() is called, while still making it possible to use the progress reporting of the ItemSync.
- Note
- You must call this method before starting the sync, changes afterwards lead to undefined results.
- See also
- setTotalItems
- Since
- 4.14
Definition at line 238 of file itemsync.cpp.
◆ setFullSyncItems()
void ItemSync::setFullSyncItems | ( | const Item::List & | items | ) |
Sets the full item list for the collection.
Usually the result of a full item listing.
- Warning
- If the client using this is a resource, all items must have a valid remote identifier.
- Parameters
-
items A list of items.
Definition at line 198 of file itemsync.cpp.
◆ setIncrementalSyncItems()
void ItemSync::setIncrementalSyncItems | ( | const Item::List & | changedItems, |
const Item::List & | removedItems ) |
Sets the item lists for incrementally syncing the collection.
Usually the result of an incremental remote item listing.
- Warning
- If the client using this is a resource, all items must have a valid remote identifier.
- Parameters
-
changedItems A list of items added or changed by the client. removedItems A list of items deleted by the client.
Definition at line 244 of file itemsync.cpp.
◆ setMergeMode()
void ItemSync::setMergeMode | ( | MergeMode | mergeMode | ) |
Set what merge method should be used for next ItemSync run.
By default ItemSync uses RIDMerge method.
See ItemCreateJob for details on Item merging.
- Note
- You must call this method before starting the sync, changes afterwards lead to undefined results.
- See also
- mergeMode
- Since
- 4.14.11
Definition at line 551 of file itemsync.cpp.
◆ setStreamingEnabled()
void ItemSync::setStreamingEnabled | ( | bool | enable | ) |
Enable item streaming.
Item streaming means that the items delivered by setXItems() calls are delivered in chunks and you manually indicate when all items have been delivered by calling deliveryDone().
- Parameters
-
enable true
to enable item streaming
Definition at line 485 of file itemsync.cpp.
◆ setTotalItems()
void ItemSync::setTotalItems | ( | int | amount | ) |
Set the amount of items which you are going to return in total by using the setFullSyncItems()/setIncrementalSyncItems() methods.
- Warning
- By default the item sync will automatically end once sufficient items have been provided. To disable this use setDisableAutomaticDeliveryDone
- See also
- setDisableAutomaticDeliveryDone
- Parameters
-
amount The amount of items in total.
Definition at line 223 of file itemsync.cpp.
◆ setTransactionMode()
void ItemSync::setTransactionMode | ( | ItemSync::TransactionMode | mode | ) |
Set the transaction mode to use for this sync.
- Note
- You must call this method before starting the sync, changes afterwards lead to undefined results.
- Parameters
-
mode the transaction mode to use
- Since
- 4.6
Definition at line 527 of file itemsync.cpp.
◆ slotResult()
|
overrideprotectedvirtual |
Reimplemented from KCompositeJob.
Definition at line 499 of file itemsync.cpp.
◆ transactionCommitted
|
signal |
Emitted whenever a transaction is committed. This is for testing only.
- Since
- 4.14
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Feb 28 2025 11:52:30 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.