Akonadi::ServerManager
#include <servermanager.h>
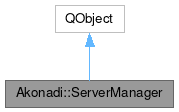
Public Types | |
enum | OpenMode { ReadOnly , ReadWrite } |
enum | ServiceAgentType { Agent , Resource , Preprocessor } |
enum | ServiceType { Server , Control , ControlLock , UpgradeIndicator } |
enum | State { NotRunning , Starting , Running , Stopping , Broken , Upgrading } |
Signals | |
void | started () |
void | stateChanged (Akonadi::ServerManager::State state) |
void | stopped () |
Detailed Description
Provides methods to control the Akonadi server process.
Asynchronous, low-level control of the Akonadi server. Akonadi::Control provides a synchronous interface to some of the methods in here.
- See also
- Akonadi::Control
- Since
- 4.2
Definition at line 28 of file servermanager.h.
Member Enumeration Documentation
◆ OpenMode
enum Akonadi::ServerManager::OpenMode |
Definition at line 161 of file servermanager.h.
◆ ServiceAgentType
◆ ServiceType
◆ State
Enum for the various states the server can be in.
- Since
- 4.5
Definition at line 36 of file servermanager.h.
Member Function Documentation
◆ addNamespace()
Adds the multi-instance namespace to string
if required (with '_' as separator).
Use whenever a multi-instance safe name is required (configfiles, identifiers, ...).
- Parameters
-
string the string to adapt
- Since
- 4.10
Definition at line 367 of file servermanager.cpp.
◆ agentConfigFilePath()
Returns absolute path to configuration file of an agent identified by given identifier
.
Definition at line 362 of file servermanager.cpp.
◆ agentServiceName()
|
static |
Returns the namespaced D-Bus service name for an agent of type agentType
with agent identifier identifier
.
- Parameters
-
agentType the agent type to use for D-Bus base name identifier the agent identifier to include in the D-Bus name
- Since
- 4.10
Definition at line 343 of file servermanager.cpp.
◆ brokenReason()
|
static |
Returns the reason why the Server is broken, if known.
If state() is Broken
, then you can use this method to obtain a more detailed description of the problem and present it to users. Note that the message can be empty if the reason is not known.
- Since
- 5.6
Definition at line 309 of file servermanager.cpp.
◆ generation()
|
static |
Returns current Akonadi database generation identifier.
Generation is guaranteed to never change unless as long as the database backend is not removed and re-created. In such case it is guaranteed that the new generation number will be higher than the previous one.
Generation can be used by applications to detect when Akonadi database has been recreated and thus some of the configuration (for example collection IDs stored in a config file) must be invalidated.
- Note
- Note that the generation number is only available if the server is running. If this function is called before the server starts it will return 0.
- Since
- 5.4
Definition at line 375 of file servermanager.cpp.
◆ hasInstanceIdentifier()
|
static |
Returns true
if we are connected to a non-default Akonadi server instance.
- Since
- 4.10
Definition at line 322 of file servermanager.cpp.
◆ instanceIdentifier()
|
static |
Returns the identifier of the Akonadi instance we are connected to.
This is usually an empty string (representing the default instance), unless you have explicitly set the AKONADI_INSTANCE environment variable to connect to a different one.
- Since
- 4.10
Definition at line 317 of file servermanager.cpp.
◆ isRunning()
|
static |
Checks if the server is available currently.
For more detailed status information see state().
- See also
- state()
Definition at line 238 of file servermanager.cpp.
◆ self()
|
static |
Returns the singleton instance of this class, for connecting to its signals.
Definition at line 178 of file servermanager.cpp.
◆ serverConfigFilePath()
|
static |
Returns absolute path to akonadiserverrc file with Akonadi server configuration.
Definition at line 357 of file servermanager.cpp.
◆ serviceName()
|
static |
Returns the namespaced D-Bus service name for serviceType
.
Use this rather the raw service name strings in order to support usage of a non-default instance of the Akonadi server.
- Parameters
-
serviceType the service type for which to return the D-Bus name
- Since
- 4.10
Definition at line 327 of file servermanager.cpp.
◆ showSelfTestDialog()
Shows the Akonadi self test dialog, which tests Akonadi for various problems and reports these to the user if.
- Parameters
-
parent the parent widget for the dialog
Definition at line 229 of file servermanager.cpp.
◆ start()
|
static |
Starts the server.
This method returns immediately and does not wait until the server is actually up and running.
- Returns
true
if the start was possible (which not necessarily means the server is really running though) andfalse
if an immediate error occurred.
- See also
- Akonadi::Control::start()
Definition at line 183 of file servermanager.cpp.
◆ started
|
signal |
Emitted whenever the server becomes fully operational.
◆ state()
|
static |
◆ stateChanged
|
signal |
Emitted whenever the server state changes.
- Parameters
-
state the new server state
- Since
- 4.5
◆ stop()
|
static |
Stops the server.
This methods returns immediately after the shutdown command has been send and does not wait until the server is actually shut down.
- Returns
true
if the shutdown command was sent successfully,false
otherwise
Definition at line 216 of file servermanager.cpp.
◆ stopped
|
signal |
Emitted whenever the server becomes unavailable.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Apr 27 2024 22:07:20 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.