Akonadi::Server::DataStore
#include <datastore.h>
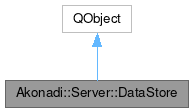
Signals | |
void | transactionCommitted () |
void | transactionRolledBack () |
Public Member Functions | |
~DataStore () override | |
virtual void | activeCachePolicy (Collection &col) |
virtual bool | addCollectionAttribute (const Collection &col, const QByteArray &key, const QByteArray &value, bool silent=false) |
virtual bool | appendCollection (Collection &collection, const QStringList &mimeTypes, const QMap< QByteArray, QByteArray > &attributes) |
virtual bool | appendItemsFlags (const PimItem::List &items, const QList< Flag > &flags, bool *flagsChanged=nullptr, bool checkIfExists=true, const Collection &col=Collection(), bool silent=false) |
virtual bool | appendItemsTags (const PimItem::List &items, const Tag::List &tags, bool *tagsChanged=nullptr, bool checkIfExists=true, const Collection &col=Collection(), bool silent=false) |
virtual bool | appendMimeTypeForCollection (qint64 collectionId, const QStringList &mimeTypes) |
virtual bool | appendPimItem (QList< Part > &parts, const QList< Flag > &flags, const MimeType &mimetype, const Collection &collection, const QDateTime &dateTime, const QString &remote_id, const QString &remoteRevision, const QString &gid, PimItem &pimItem) |
virtual bool | beginTransaction (const QString &name) |
virtual bool | cleanupCollection (Collection &collection) |
virtual bool | cleanupPimItems (const PimItem::List &items, bool silent=false) |
void | close () |
virtual bool | commitTransaction () |
QSqlDatabase | database () |
bool | doRollback () |
virtual bool | init () |
bool | inTransaction () const |
virtual bool | invalidateItemCache (const PimItem &item) |
bool | isOpened () const |
virtual bool | moveCollection (Collection &collection, const Collection &newParent) |
NotificationCollector * | notificationCollector () |
virtual void | open () |
virtual bool | removeCollectionAttribute (const Collection &col, const QByteArray &key) |
virtual bool | removeItemParts (const PimItem &item, const QSet< QByteArray > &parts) |
virtual bool | removeItemsFlags (const PimItem::List &items, const QList< Flag > &flags, bool *tagsChanged=nullptr, const Collection &collection=Collection(), bool silent=false) |
virtual bool | removeItemsTags (const PimItem::List &items, const Tag::List &tags, bool *tagsChanged=nullptr, bool silent=false) |
virtual bool | removeTags (const Tag::List &tags, bool silent=false) |
virtual bool | rollbackTransaction () |
virtual bool | setItemsFlags (const PimItem::List &items, const QList< Flag > *currentFlags, const QList< Flag > &newFlags, bool *flagsChanged=nullptr, const Collection &col=Collection(), bool silent=false) |
virtual bool | setItemsTags (const PimItem::List &items, const Tag::List &tags, bool *tagsChanged=nullptr, bool silent=false) |
void | setSessionId (const QByteArray &sessionId) |
void | transactionKilledByDB () |
virtual bool | unhideAllPimItems () |
virtual bool | unhidePimItem (PimItem &pimItem) |
QMap< Server::Entity::Id, QList< PimItem > > | virtualCollections (const Akonadi::Server::PimItem::List &items) |
QList< Collection > | virtualCollections (const PimItem &item) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static QString | collectionDelimiter () |
static DataStore * | dataStoreForDatabase (const QSqlDatabase &db) |
static bool | hasDataStore () |
static DataStore * | self () |
static void | setFactory (std::unique_ptr< DataStoreFactory > factory) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Static Public Attributes | |
const static constexpr bool | Silent = true |
Protected Member Functions | |
DataStore (AkonadiServer *akonadi, DbConfig *dbConfig) | |
DataStore (DbConfig *config) | |
void | debugLastDbError (QStringView actionDescription) const |
void | debugLastQueryError (const QSqlQuery &query, const char *actionDescription) const |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Protected Attributes | |
AkonadiServer *const | m_akonadi = nullptr |
DbConfig *const | m_dbConfig = nullptr |
std::unique_ptr< NotificationCollector > | mNotificationCollector |
Static Protected Attributes | |
static std::unique_ptr< DataStoreFactory > | sFactory |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
Detailed Description
This class handles all the database access.
Database configuration
You can select between various database backends during runtime using the $HOME/
.config/akonadi/akonadiserverrc configuration file.
Example:
[%General] Driver=QMYSQL [QMYSQL_EMBEDDED] Name=akonadi Options=SERVER_DATADIR=/home/foo/.local/share/akonadi/db_data [QMYSQL] Name=akonadi Host=localhost User=foo Password=***** #Options=UNIX_SOCKET=/home/foo/.local/share/akonadi/socket-bar/mysql.socket StartServer=true ServerPath=/usr/sbin/mysqld [QSQLITE] Name=/home/foo/.local/share/akonadi/akonadi.db
Use General/Driver
to select the QSql driver to use for database access. The following drivers are currently supported, other might work but are untested:
- QMYSQL
- QMYSQL_EMBEDDED
- QSQLITE
The options for each driver are read from the corresponding group. The following options are supported, dependent on the driver not all of them might have an effect:
- Name: Database name, for sqlite that's the file name of the database.
- Host: Hostname of the database server
- User: Username for the database server
- Password: Password for the database server
- Options: Additional options, format is driver-dependent
- StartServer: Start the database locally just for Akonadi instead of using an existing one
- ServerPath: Path to the server executable
Definition at line 94 of file datastore.h.
Constructor & Destructor Documentation
◆ ~DataStore()
|
override |
Closes the database connection and destroys the DataStore object.
Definition at line 128 of file datastore.cpp.
◆ DataStore() [1/2]
|
protected |
Creates a new DataStore object and opens it.
Definition at line 106 of file datastore.cpp.
◆ DataStore() [2/2]
|
explicitprotected |
Definition at line 123 of file datastore.cpp.
Member Function Documentation
◆ activeCachePolicy()
|
virtual |
Determines the active cache policy for this Collection.
The active cache policy is set in the corresponding Collection fields.
Definition at line 997 of file datastore.cpp.
◆ addCollectionAttribute()
|
virtual |
Definition at line 1207 of file datastore.cpp.
◆ appendCollection()
|
virtual |
Definition at line 824 of file datastore.cpp.
◆ appendItemsFlags()
|
virtual |
Definition at line 389 of file datastore.cpp.
◆ appendItemsTags()
|
virtual |
Definition at line 599 of file datastore.cpp.
◆ appendMimeTypeForCollection()
|
virtual |
Definition at line 977 of file datastore.cpp.
◆ appendPimItem()
|
virtual |
Definition at line 1083 of file datastore.cpp.
◆ beginTransaction()
|
virtual |
Begins a transaction.
No changes will be written to the database and no notification signal will be emitted unless you call commitTransaction().
- Returns
true
if successful.
Definition at line 1326 of file datastore.cpp.
◆ cleanupCollection()
|
virtual |
removes the given collection and all its content
Definition at line 851 of file datastore.cpp.
◆ cleanupPimItems()
|
virtual |
Removes the pim item and all referenced data ( e.g.
flags )
Definition at line 1175 of file datastore.cpp.
◆ close()
void DataStore::close | ( | ) |
Closes the database connection.
Definition at line 179 of file datastore.cpp.
◆ collectionDelimiter()
|
inlinestatic |
Definition at line 184 of file datastore.h.
◆ commitTransaction()
|
virtual |
Commits all changes within the current transaction and emits all collected notification signals.
If committing fails, the transaction will be rolled back.
Definition at line 1388 of file datastore.cpp.
◆ database()
QSqlDatabase DataStore::database | ( | ) |
Returns the QSqlDatabase object.
Use this for generating queries yourself.
Will [re-]open the database, if it is closed.
Definition at line 171 of file datastore.cpp.
◆ dataStoreForDatabase()
|
static |
Returns DataStore associated with the given database connection.
Definition at line 98 of file datastore.cpp.
◆ debugLastDbError()
|
protected |
Definition at line 1263 of file datastore.cpp.
◆ debugLastQueryError()
|
protected |
Definition at line 1276 of file datastore.cpp.
◆ doRollback()
bool DataStore::doRollback | ( | ) |
Definition at line 1305 of file datastore.cpp.
◆ hasDataStore()
|
static |
Returns whether per thread DataStore has been created.
Definition at line 260 of file datastore.cpp.
◆ init()
|
virtual |
Initializes the database.
Should be called during startup by the main thread.
Definition at line 207 of file datastore.cpp.
◆ inTransaction()
bool DataStore::inTransaction | ( | ) | const |
Returns true if there is a transaction in progress.
Definition at line 1423 of file datastore.cpp.
◆ invalidateItemCache()
|
virtual |
Definition at line 794 of file datastore.cpp.
◆ isOpened()
|
inline |
Returns if the database is currently open.
Definition at line 295 of file datastore.h.
◆ moveCollection()
|
virtual |
moves the collection collection
to newParent
.
Definition at line 931 of file datastore.cpp.
◆ notificationCollector()
NotificationCollector * DataStore::notificationCollector | ( | ) |
Returns the notification collector of this DataStore object.
Use this to listen to change notification signals.
Definition at line 242 of file datastore.cpp.
◆ open()
|
virtual |
Opens the database connection.
Definition at line 133 of file datastore.cpp.
◆ removeCollectionAttribute()
|
virtual |
Removes the given collection attribute for col
.
- Exceptions
-
HandlerException on database errors
- Returns
true
if the attribute existed,false
otherwise
Definition at line 1240 of file datastore.cpp.
◆ removeItemParts()
|
virtual |
Definition at line 768 of file datastore.cpp.
◆ removeItemsFlags()
|
virtual |
Definition at line 446 of file datastore.cpp.
◆ removeItemsTags()
|
virtual |
Definition at line 650 of file datastore.cpp.
◆ removeTags()
|
virtual |
Definition at line 685 of file datastore.cpp.
◆ rollbackTransaction()
|
virtual |
Reverts all changes within the current transaction.
Definition at line 1366 of file datastore.cpp.
◆ self()
|
static |
Per thread singleton.
Definition at line 252 of file datastore.cpp.
◆ setFactory()
|
static |
Definition at line 93 of file datastore.cpp.
◆ setItemsFlags()
|
virtual |
Definition at line 267 of file datastore.cpp.
◆ setItemsTags()
|
virtual |
Definition at line 500 of file datastore.cpp.
◆ setSessionId()
|
inline |
Sets the current session id.
Definition at line 287 of file datastore.h.
◆ transactionCommitted
|
signal |
Emitted if a transaction has been successfully committed.
◆ transactionKilledByDB()
void DataStore::transactionKilledByDB | ( | ) |
Definition at line 1319 of file datastore.cpp.
◆ transactionRolledBack
|
signal |
Emitted if a transaction has been aborted.
◆ unhideAllPimItems()
|
virtual |
Unhides all the items which have the "hidden" flag set.
This function doesn't emit any notification about the items being unhidden so it's meant to be called only in rare circumstances. The most notable call to this function is at server startup when we attempt to restore a clean state of the database.
Definition at line 1159 of file datastore.cpp.
◆ unhidePimItem()
|
virtual |
Unhides the specified PimItem.
Emits the itemAdded() notification as the hidden flag is assumed to have been set by appendPimItem() before pushing the item to the preprocessor chain. The hidden item had his notifications disabled until now (so for the clients the "unhide" operation is actually a new item arrival).
This function does NOT verify if the item was really hidden: this is responsibility of the caller.
Definition at line 1147 of file datastore.cpp.
◆ virtualCollections()
QList< Collection > DataStore::virtualCollections | ( | const PimItem & | item | ) |
Returns all virtual collections the item
is linked to.
Definition at line 1022 of file datastore.cpp.
Member Data Documentation
◆ m_akonadi
|
protected |
Definition at line 349 of file datastore.h.
◆ m_dbConfig
|
protected |
Definition at line 350 of file datastore.h.
◆ mNotificationCollector
|
protected |
Definition at line 348 of file datastore.h.
◆ sFactory
|
staticprotected |
Definition at line 347 of file datastore.h.
◆ Silent
|
staticconstexpr |
Definition at line 98 of file datastore.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Feb 28 2025 11:52:30 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.