Akonadi::SpecialCollections
#include <specialcollections.h>
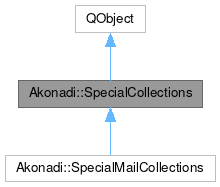
Signals | |
void | collectionsChanged (const Akonadi::AgentInstance &instance) |
void | defaultCollectionsChanged () |
Public Member Functions | |
~SpecialCollections () override | |
Akonadi::Collection | collection (const QByteArray &type, const AgentInstance &instance) const |
Akonadi::Collection | defaultCollection (const QByteArray &type) const |
bool | hasCollection (const QByteArray &type, const AgentInstance &instance) const |
bool | hasDefaultCollection (const QByteArray &type) const |
bool | isSpecialAgent (const QString &instanceIdentifier) const |
bool | registerCollection (const QByteArray &type, const Akonadi::Collection &collection) |
bool | unregisterCollection (const Collection &collection) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static void | setSpecialCollectionType (const QByteArray &type, const Akonadi::Collection &collection) |
static void | unsetSpecialCollection (const Akonadi::Collection &collection) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
SpecialCollections (KCoreConfigSkeleton *config, QObject *parent=nullptr) | |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
Detailed Description
An interface to special collections.
This class is the central interface to special collections like inbox or outbox in a mail resource or recent contacts in a contacts resource. The class is not meant to be used directly, but to inherit the a type specific special collections class from it (e.g. SpecialMailCollections).
To check whether a special collection is available, simply use the hasCollection() and hasDefaultCollection() methods. Available special collections are accessible through the collection() and defaultCollection() methods.
To create a special collection, use a SpecialCollectionsRequestJob. This will create the special collections you request and automatically register them with SpecialCollections, so that it now knows they are available.
This class monitors all special collections known to it, and removes it from the known list if they are deleted. Note that this class does not automatically rebuild the collections that disappeared.
The defaultCollectionsChanged() and collectionsChanged() signals are emitted when the special collections for a resource change (i.e. some became available or some become unavailable).
- Since
- 4.4
Definition at line 51 of file specialcollections.h.
Constructor & Destructor Documentation
◆ ~SpecialCollections()
|
overridedefault |
Destroys the special collections object.
◆ SpecialCollections()
|
explicitprotected |
Creates a new special collections object.
- Parameters
-
config The configuration skeleton that provides the default resource id. parent The parent object.
Definition at line 170 of file specialcollections.cpp.
Member Function Documentation
◆ collection()
|
nodiscard |
Returns the special collection of the given type
in the given agent instance
, or an invalid collection if such a collection is unknown.
Definition at line 183 of file specialcollections.cpp.
◆ collectionsChanged
|
signal |
Emitted when the special collections for a resource have been changed (for example, some become available, or some become unavailable).
- Parameters
-
instance The instance of the resource the collection belongs to.
◆ defaultCollection()
|
nodiscard |
Returns the special collection of given type
in the default resource, or an invalid collection if such a collection is unknown.
Definition at line 261 of file specialcollections.cpp.
◆ defaultCollectionsChanged
|
signal |
Emitted when the special collections for the default resource have been changed (for example, some become available, or some become unavailable).
◆ hasCollection()
|
nodiscard |
Returns whether the given agent instance
has a special collection of the given type
.
Definition at line 178 of file specialcollections.cpp.
◆ hasDefaultCollection()
|
nodiscard |
Returns whether the default resource has a special collection of the given type
.
Definition at line 256 of file specialcollections.cpp.
◆ isSpecialAgent()
|
nodiscard |
Returns whether the instanceIdentifier is a special agent that should not be deleted.
Definition at line 266 of file specialcollections.cpp.
◆ registerCollection()
bool SpecialCollections::registerCollection | ( | const QByteArray & | type, |
const Akonadi::Collection & | collection ) |
Registers the given collection
as a special collection with the given type
.
- Parameters
-
type the special type of collection
collection the given collection to register The collection must be owned by a valid resource. Registering a new collection of a previously registered type forgets the old collection.
Definition at line 228 of file specialcollections.cpp.
◆ setSpecialCollectionType()
|
static |
Sets the special collection attribute which marks collection
as being a special collection of type type
.
This is typically used by configuration dialog for resources, when the user can choose a specific special collection (ex: IMAP trash).
- Since
- 4.11
Definition at line 188 of file specialcollections.cpp.
◆ unregisterCollection()
bool SpecialCollections::unregisterCollection | ( | const Collection & | collection | ) |
Unregisters the given collection
as a special collection.
- Parameters
-
type the special type of collection
- Since
- 4.12
Definition at line 207 of file specialcollections.cpp.
◆ unsetSpecialCollection()
|
static |
unsets the special collection attribute which marks collection
as being a special collection.
- Since
- 4.12
Definition at line 198 of file specialcollections.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Mar 28 2025 11:53:22 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.