EventViews::MonthItem
#include <monthitem.h>
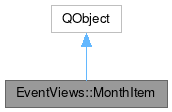
Public Member Functions | |
MonthItem (MonthScene *monthWidget) | |
virtual bool | allDay () const =0 |
void | beginMove () |
void | beginResize () |
virtual QColor | bgColor () const =0 |
int | daySpan () const |
void | deleteAll () |
QDate | endDate () const |
void | endMove () |
void | endResize () |
virtual QColor | frameColor () const =0 |
virtual bool | greaterThanFallback (const MonthItem *other) const |
virtual QList< QPixmap > | icons () const =0 |
virtual bool | isMoveable () const =0 |
bool | isMoving () const |
virtual bool | isResizable () const =0 |
bool | isResizing () const |
QList< MonthGraphicsItem * > | monthGraphicsItems () const |
MonthScene * | monthScene () const |
void | moveBy (int offsetFromPreviousDate) |
void | moveTo (QDate date) |
QWidget * | parentWidget () const |
int | position () const |
virtual QDate | realEndDate () const =0 |
virtual QDate | realStartDate () const =0 |
bool | resizeBy (int offsetFromPreviousDate) |
bool | selected () const |
void | setSelected (bool selected) |
QDate | startDate () const |
virtual QString | text (bool end) const =0 |
virtual QString | toolTipText (const QDate &date) const =0 |
void | updateGeometry () |
void | updateMonthGraphicsItems () |
void | updatePosition () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static bool | greaterThan (const MonthItem *e1, const MonthItem *e2) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
virtual void | finalizeMove (const QDate &newStartDate)=0 |
virtual void | finalizeResize (const QDate &newStartDate, const QDate &newEndDate)=0 |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
Detailed Description
A month item manages different MonthGraphicsItems.
Definition at line 26 of file monthitem.h.
Constructor & Destructor Documentation
◆ MonthItem()
|
explicit |
Definition at line 33 of file monthitem.cpp.
◆ ~MonthItem()
|
override |
Definition at line 38 of file monthitem.cpp.
Member Function Documentation
◆ allDay()
|
pure virtual |
True if this item last all the day.
◆ beginMove()
void MonthItem::beginMove | ( | ) |
Begin a move.
Definition at line 118 of file monthitem.cpp.
◆ beginResize()
void MonthItem::beginResize | ( | ) |
Begin a resize.
Definition at line 100 of file monthitem.cpp.
◆ bgColor()
|
nodiscardpure virtual |
Returns the background color of the item.
◆ daySpan()
|
nodiscard |
The number of days this item spans.
Definition at line 202 of file monthitem.cpp.
◆ deleteAll()
void MonthItem::deleteAll | ( | ) |
Deletes all MonthGraphicsItem this item handles.
Clear the list.
Definition at line 43 of file monthitem.cpp.
◆ endDate()
|
nodiscard |
The end date of the incidence, generally realEndDate.
But it reflect changes, even during move.
Definition at line 193 of file monthitem.cpp.
◆ endMove()
void MonthItem::endMove | ( | ) |
End a move.
Definition at line 126 of file monthitem.cpp.
◆ endResize()
void MonthItem::endResize | ( | ) |
End a resize.
Definition at line 108 of file monthitem.cpp.
◆ finalizeMove()
|
protectedpure virtual |
Called after a move operation.
◆ finalizeResize()
|
protectedpure virtual |
Called after a resize operation.
◆ frameColor()
|
nodiscardpure virtual |
Returns the frame color of the item.
◆ greaterThan()
Compares two items to decide which to place in the view first.
The month view displays a list of items. When loading (which occurs each time there is a change), the items are sorted in an order intended to avoid unsightly gaps:
- biggest durations first
- earliest date
- finally, time in the day Holidays are sorted before events with the same start date and length, so they appear at the top of the day's box.
Definition at line 217 of file monthitem.cpp.
◆ greaterThanFallback()
|
virtual |
Compare this event with a second one, if the former function is not able to sort them.
Definition at line 242 of file monthitem.cpp.
◆ icons()
Returns a list of pixmaps to draw next to the items.
◆ isMoveable()
|
pure virtual |
Returns true if the item can be moved.
◆ isMoving()
|
inlinenodiscard |
Returns true if the item is being moved.
Definition at line 167 of file monthitem.h.
◆ isResizable()
|
pure virtual |
Returns true if the item can be resized.
◆ isResizing()
|
inlinenodiscard |
Returns true if the item is being resized.
Definition at line 175 of file monthitem.h.
◆ monthGraphicsItems()
QList< MonthGraphicsItem * > EventViews::MonthItem::monthGraphicsItems | ( | ) | const |
Definition at line 279 of file monthitem.cpp.
◆ monthScene()
|
inlinenodiscard |
Returns the associated month scene to this item.
Definition at line 121 of file monthitem.h.
◆ moveBy()
void MonthItem::moveBy | ( | int | offsetFromPreviousDate | ) |
Called during move to move the item a bit, relative to the previous move step.
Definition at line 158 of file monthitem.cpp.
◆ moveTo()
void MonthItem::moveTo | ( | QDate | date | ) |
Called during a drag to move the item to a particular date.
An invalid date indicates a drag outside the month grid.
Definition at line 164 of file monthitem.cpp.
◆ parentWidget()
|
nodiscard |
Definition at line 49 of file monthitem.cpp.
◆ position()
|
inlinenodiscard |
Returns the position of the item ( > 0 ).
Definition at line 113 of file monthitem.h.
◆ realEndDate()
|
pure virtual |
This is the real end date, usually the end date of the incidence.
◆ realStartDate()
|
pure virtual |
This is the real start date, usually the start date of the incidence.
◆ resizeBy()
bool MonthItem::resizeBy | ( | int | offsetFromPreviousDate | ) |
Called during resize to resize the item a bit, relative to the previous resize step.
Definition at line 136 of file monthitem.cpp.
◆ selected()
|
inlinenodiscard |
Returns true if this item is selected.
Definition at line 105 of file monthitem.h.
◆ setSelected()
|
inline |
Sets the selection state of this item.
Definition at line 210 of file monthitem.h.
◆ startDate()
|
nodiscard |
The start date of the incidence, generally realStartDate.
But it reflect changes, even during move.
Definition at line 184 of file monthitem.cpp.
◆ text()
|
pure virtual |
Returns the text to draw in an item.
- Parameters
-
end True if the text at the end of an item should be returned.
◆ toolTipText()
Returns the text for the tooltip of the item.
◆ updateGeometry()
void MonthItem::updateGeometry | ( | ) |
Updates geometry of all MonthGraphicsItems.
Definition at line 170 of file monthitem.cpp.
◆ updateMonthGraphicsItems()
void MonthItem::updateMonthGraphicsItems | ( | ) |
Update the monthgraphicsitems.
This basically deletes and rebuild all the MonthGraphicsItems but tries to do it wisely:
- If there is a moving item, it won't be deleted because then the new item won't receive anymore the MouseMove events.
- If there is an item on a line where the new state needs an item, it is used and not deleted. This will avoid flickers.
Definition at line 54 of file monthitem.cpp.
◆ updatePosition()
void MonthItem::updatePosition | ( | ) |
Find the lowest possible position for this item.
The position of an item in a cell is it's vertical position. This is used to avoid overlapping of items. An item keeps the same position in every cell it crosses. The position is measured from top to bottom.
Definition at line 250 of file monthitem.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:51:37 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.