KIdentityManagementCore::IdentityManager
#include <identitymanager.h>
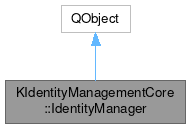
Public Types | |
using | ConstIterator = QList<Identity>::ConstIterator |
using | Iterator = QList<Identity>::Iterator |
Signals | |
void | added (const KIdentityManagementCore::Identity &ident) |
void | changed () |
void | changed (const KIdentityManagementCore::Identity &ident) |
void | changed (uint uoid) |
void | deleted (uint uoid) |
void | identitiesChanged (const QString &id) |
void | identitiesWereChanged () |
void | identityChanged (const KIdentityManagementCore::Identity &ident) |
void | needToReloadIdentitySettings () |
Public Member Functions | |
IdentityManager (bool readonly=false, QObject *parent=nullptr, const char *name=nullptr) | |
QStringList | allEmails () const |
ConstIterator | begin () const |
void | commit () |
const Identity & | defaultIdentity () const |
ConstIterator | end () const |
bool | hasPendingChanges () const |
QStringList | identities () const |
const Identity & | identityForAddress (const QString &addresses) const |
const Identity & | identityForUoid (uint uoid) const |
const Identity & | identityForUoidOrDefault (uint uoid) const |
bool | isUnique (const QString &name) const |
QString | makeUnique (const QString &name) const |
Iterator | modifyBegin () |
Iterator | modifyEnd () |
Identity & | modifyIdentityForName (const QString &identityName) |
Identity & | modifyIdentityForUoid (uint uoid) |
Identity & | newFromControlCenter (const QString &name) |
Identity & | newFromExisting (const Identity &other, const QString &name=QString()) |
Identity & | newFromScratch (const QString &name) |
bool | removeIdentity (const QString &identityName) |
bool | removeIdentityForced (const QString &identityName) |
void | rollback () |
void | saveIdentity (const Identity &ident) |
bool | setAsDefault (uint uoid) |
QStringList | shadowIdentities () const |
void | sort () |
bool | thatIsMe (const QString &addressList) const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static IdentityManager * | self () |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Slots | |
void | slotRollback () |
Protected Member Functions | |
virtual void | createDefaultIdentity (QString &, QString &) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
Detailed Description
Manages the list of identities.
Definition at line 25 of file identitymanager.h.
Member Typedef Documentation
◆ ConstIterator
using KIdentityManagementCore::IdentityManager::ConstIterator = QList<Identity>::ConstIterator |
Definition at line 51 of file identitymanager.h.
◆ Iterator
using KIdentityManagementCore::IdentityManager::Iterator = QList<Identity>::Iterator |
Definition at line 50 of file identitymanager.h.
Constructor & Destructor Documentation
◆ IdentityManager()
|
explicit |
Create an identity manager, which loads the emailidentities file to create identities.
- Parameters
-
readonly if true, no changes can be made to the identity manager This means in particular that if there is no identity configured, the default identity created here will not be saved. It is assumed that a minimum of one identity is always present.
Member Function Documentation
◆ added
|
signal |
Emitted on commit() for each new identity.
◆ allEmails()
|
nodiscard |
Returns the list of all email addresses (only name@host) from all identities.
◆ changed [1/3]
|
signal |
Emitted whenever a commit changes any configure option.
◆ changed [2/3]
|
signal |
Emitted whenever the identity ident
changed.
Useful for more fine-grained change notifications than what is possible with the standard changed() signal.
◆ changed [3/3]
|
signal |
Emitted whenever the identity with Unique Object Identifier (UOID) uoid
changed.
Useful for more fine-grained change notifications than what is possible with the standard changed() signal.
◆ commit()
void KIdentityManagementCore::IdentityManager::commit | ( | ) |
Commit changes to disk and emit changed() if necessary.
◆ createDefaultIdentity()
|
protectedvirtual |
This is called when no identity has been defined, so we need to create a default one.
The parameters are filled with some default values from KUser, but reimplementations of this method can give them another value.
◆ defaultIdentity()
const Identity & KIdentityManagementCore::IdentityManager::defaultIdentity | ( | ) | const |
- Returns
- the default identity
◆ deleted
|
signal |
Emitted on commit() for each deleted identity.
At the time this signal is emitted, the identity does still exist and can be retrieved by identityForUoid() if needed
◆ hasPendingChanges()
|
nodiscard |
Check whether there are any unsaved changes.
◆ identities()
|
nodiscard |
- Returns
- the list of identities
◆ identityForAddress()
const Identity & KIdentityManagementCore::IdentityManager::identityForAddress | ( | const QString & | addresses | ) | const |
- Returns
- an identity whose address matches any in
addresses
or Identity::null if no such identity exists.
- Parameters
-
addresses the string of addresses to scan for matches
◆ identityForUoid()
const Identity & KIdentityManagementCore::IdentityManager::identityForUoid | ( | uint | uoid | ) | const |
- Returns
- the identity with Unique Object Identifier (UOID)
uoid
or Identity::null if not found.
- Parameters
-
uoid the Unique Object Identifier to find identity with
◆ identityForUoidOrDefault()
const Identity & KIdentityManagementCore::IdentityManager::identityForUoidOrDefault | ( | uint | uoid | ) | const |
Convenience method.
- Returns
- the identity with Unique Object Identifier (UOID)
uoid
or the default identity if not found.
- Parameters
-
uoid the Unique Object Identifier to find identity with
◆ isUnique()
|
nodiscard |
- Returns
- whether the
name
is unique
- Parameters
-
name the name to be examined
◆ makeUnique()
|
nodiscard |
- Returns
- a unique name for a new identity based on
name
- Parameters
-
name the name of the base identity
◆ modifyBegin()
Iterator KIdentityManagementCore::IdentityManager::modifyBegin | ( | ) |
Iterator used by the configuration dialog, which works on a separate list of identities, for modification.
Changes are made effective by commit().
◆ modifyIdentityForName()
Identity & KIdentityManagementCore::IdentityManager::modifyIdentityForName | ( | const QString & | identityName | ) |
- Returns
- the identity named
identityName
. This method returns a reference to the identity that can be modified. To let others see this change, use commit.
- Parameters
-
identityName the identity name to return modifiable reference
◆ modifyIdentityForUoid()
Identity & KIdentityManagementCore::IdentityManager::modifyIdentityForUoid | ( | uint | uoid | ) |
- Returns
- the identity with Unique Object Identifier (UOID)
uoid
. This method returns a reference to the identity that can be modified. To let others see this change, use commit.
◆ removeIdentity()
|
nodiscard |
Removes the identity with name identityName
Will return false if the identity is not found, or when one tries to remove the last identity.
- Parameters
-
identityName the identity to remove
◆ removeIdentityForced()
|
nodiscard |
Removes the identity with name identityName
Will return false
if the identity is not found, true
otherwise.
- Note
- In opposite to removeIdentity, this method allows to remove the last remaining identity.
- Since
- 4.6
◆ rollback()
void KIdentityManagementCore::IdentityManager::rollback | ( | ) |
Re-read the config from disk and forget changes.
◆ saveIdentity()
void KIdentityManagementCore::IdentityManager::saveIdentity | ( | const Identity & | ident | ) |
Store a new identity or modify an existing identity based on an independent identity object.
- Parameters
-
ident the identity to be saved
◆ self()
|
static |
Creates or reuses the identity manager instance for this process.
It loads the emailidentities file to create identities. This sets readonly to false, so you should create a separate instance if you need it to be readonly.
- Since
- 5.2.91
◆ setAsDefault()
bool KIdentityManagementCore::IdentityManager::setAsDefault | ( | uint | uoid | ) |
Sets the identity with Unique Object Identifier (UOID) uoid
to be new the default identity.
As usual, use commit to make this permanent.
- Parameters
-
uoid the default identity to set
- Returns
- false if an identity with UOID
uoid
was not found
◆ shadowIdentities()
|
nodiscard |
Convenience method.
- Returns
- the list of (shadow) identities, ie. the ones currently under configuration.
◆ sort()
void KIdentityManagementCore::IdentityManager::sort | ( | ) |
Sort the identities by name (the default is always first).
This operates on the shadow list, so you need to commit for the changes to take effect.
◆ thatIsMe()
|
nodiscard |
- Returns
- true if
addressList
contains any of our addresses, false otherwise.
- Parameters
-
addressList the addressList to examine
- See also
- identityForAddress
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Feb 21 2025 11:57:11 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.