KLDAPCore::LdapSearch
#include <ldapsearch.h>
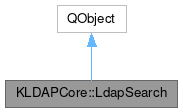
Signals | |
void | data (KLDAPCore::LdapSearch *search, const KLDAPCore::LdapObject &obj) |
void | result (KLDAPCore::LdapSearch *search) |
Public Member Functions | |
LdapSearch () | |
LdapSearch (LdapConnection &connection) | |
void | abandon () |
void | continueSearch () |
int | error () const |
QString | errorString () const |
bool | isFinished () const |
bool | search (const LdapDN &base, LdapUrl::Scope scope=LdapUrl::Sub, const QString &filter=QString(), const QStringList &attributes=QStringList(), int pagesize=0, int count=0) |
bool | search (const LdapServer &server, const QStringList &attributes=QStringList(), int count=0) |
bool | search (const LdapUrl &url, int count=0) |
void | setClientControls (const LdapControls &ctrls) |
void | setConnection (LdapConnection &connection) |
void | setServerControls (const LdapControls &ctrls) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
This class starts a search operation on a LDAP server and returns the search values via a Qt signal.
Definition at line 32 of file ldapsearch.h.
Constructor & Destructor Documentation
◆ LdapSearch() [1/2]
LdapSearch::LdapSearch | ( | ) |
Constructs an LdapSearch object.
Definition at line 239 of file ldapsearch.cpp.
◆ LdapSearch() [2/2]
|
explicit |
Constructs an LdapConnection object with the given connection.
If this form of constructor used, then always this connection will be used regardless of the LDAP Url or LdapServer object passed to search().
- Parameters
-
connection the connection used to construct LdapConnection object
Definition at line 246 of file ldapsearch.cpp.
◆ ~LdapSearch()
|
override |
Definition at line 253 of file ldapsearch.cpp.
Member Function Documentation
◆ abandon()
void LdapSearch::abandon | ( | ) |
Tries to abandon the search.
Definition at line 321 of file ldapsearch.cpp.
◆ continueSearch()
void LdapSearch::continueSearch | ( | ) |
Continues the search (if you set count to non-zero in search(), and isFinished() is false)
Definition at line 307 of file ldapsearch.cpp.
◆ data
|
signal |
Emitted for each result object.
◆ error()
|
nodiscard |
Returns the error code of the search operation (0 if no error).
Definition at line 326 of file ldapsearch.cpp.
◆ errorString()
|
nodiscard |
Returns the error description of the search operation.
Definition at line 331 of file ldapsearch.cpp.
◆ isFinished()
|
nodiscard |
Returns true if the search is finished else returns false.
Definition at line 316 of file ldapsearch.cpp.
◆ result
|
signal |
Emitted when the searching finished.
◆ search() [1/3]
|
nodiscard |
Starts a search operation if the LdapConnection object already set in the constructor.
Definition at line 301 of file ldapsearch.cpp.
◆ search() [2/3]
|
nodiscard |
Starts a search operation on the LDAP server.
- Parameters
-
server,returning the attributes specified with attributes. count means how many entries to list. If it's >0, then result() will be emitted when the number of entries is reached, but with isFinished() set to false.
Definition at line 275 of file ldapsearch.cpp.
◆ search() [3/3]
|
nodiscard |
Starts a search operation on the given LDAP URL.
Definition at line 287 of file ldapsearch.cpp.
◆ setClientControls()
void LdapSearch::setClientControls | ( | const LdapControls & | ctrls | ) |
Sets the client controls which will sent with each operation.
Definition at line 265 of file ldapsearch.cpp.
◆ setConnection()
void LdapSearch::setConnection | ( | LdapConnection & | connection | ) |
Sets the connection for this object to use for searches from now onwards, regardless of the LDAP Url or LdapServer object passed to search().
Definition at line 258 of file ldapsearch.cpp.
◆ setServerControls()
void LdapSearch::setServerControls | ( | const LdapControls & | ctrls | ) |
Sets the server controls which will sent with each operation.
Definition at line 270 of file ldapsearch.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 4 2025 12:11:23 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.