KPublicTransport::Manager
#include <manager.h>
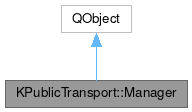
Properties | |
bool | allowInsecureBackends |
QVariantList | attributions |
QVariantList | backends |
bool | backendsEnabledByDefault |
QStringList | disabledBackends |
QStringList | enabledBackends |
![]() | |
objectName | |
Signals | |
void | attributionsChanged () |
void | configurationChanged () |
Public Member Functions | |
Manager (QObject *parent=nullptr) | |
bool | allowInsecureBackends () const |
const std::vector< Attribution > & | attributions () const |
const std::vector< Backend > & | backends () const |
bool | backendsEnabledByDefault () const |
QStringList | disabledBackends () const |
QStringList | enabledBackends () const |
Q_INVOKABLE bool | isBackendEnabled (const QString &backendId) const |
JourneyReply * | queryJourney (const JourneyRequest &req) const |
LocationReply * | queryLocation (const LocationRequest &req) const |
StopoverReply * | queryStopover (const StopoverRequest &req) const |
VehicleLayoutReply * | queryVehicleLayout (const VehicleLayoutRequest &req) const |
void | setAllowInsecureBackends (bool insecure) |
void | setBackendEnabled (const QString &backendId, bool enabled) |
void | setBackendsEnabledByDefault (bool byDefault) |
void | setDisabledBackends (const QStringList &backendIds) |
void | setEnabledBackends (const QStringList &backendIds) |
void | setNetworkAccessManager (QNetworkAccessManager *nam) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Entry point for starting public transport queries.
Queries return reply objects, you are responsible for deleting those, typically by calling deleteLater() on them after having retrieved their result (similar to how QNetworkAccessManager works).
Property Documentation
◆ allowInsecureBackends
|
readwrite |
◆ attributions
|
read |
QML-compatible access to attributions().
◆ backends
|
read |
QML-compatible access to backends().
◆ backendsEnabledByDefault
|
readwrite |
- See also
- backendsEnabledByDefault()
◆ disabledBackends
|
readwrite |
- See also
- disabledBackends()
◆ enabledBackends
|
readwrite |
- See also
- enabledBackends()
Constructor & Destructor Documentation
◆ Manager()
|
explicit |
Definition at line 485 of file manager.cpp.
Member Function Documentation
◆ allowInsecureBackends()
bool Manager::allowInsecureBackends | ( | ) | const |
Returns whether access to insecure backends is allowed.
Definition at line 516 of file manager.cpp.
◆ attributions()
const std::vector< Attribution > & Manager::attributions | ( | ) | const |
Returns all static attribution information, as well as all dynamic ones found in the cache or accumulated during the lifetime of this instance.
Definition at line 850 of file manager.cpp.
◆ backends()
const std::vector< Backend > & Manager::backends | ( | ) | const |
Returns information about all available backends.
Definition at line 867 of file manager.cpp.
◆ backendsEnabledByDefault()
bool Manager::backendsEnabledByDefault | ( | ) | const |
Returns wheter backends are enabled by default.
Defaults to true.
Definition at line 939 of file manager.cpp.
◆ disabledBackends()
QStringList Manager::disabledBackends | ( | ) | const |
Returns the identifiers of explicitly disabled backends.
Use this for persisting settings, not for checking for disabled backends.
Definition at line 926 of file manager.cpp.
◆ enabledBackends()
QStringList Manager::enabledBackends | ( | ) | const |
Returns the identifiers of explicitly enabled backends.
Use this for persisting the settings, not for checking for enabled backends.
Definition at line 913 of file manager.cpp.
◆ isBackendEnabled()
bool Manager::isBackendEnabled | ( | const QString & | backendId | ) | const |
Returns whether the use of the backend with a given identifier is enabled.
Definition at line 873 of file manager.cpp.
◆ queryJourney()
JourneyReply * Manager::queryJourney | ( | const JourneyRequest & | req | ) | const |
Query a journey.
Definition at line 530 of file manager.cpp.
◆ queryLocation()
LocationReply * Manager::queryLocation | ( | const LocationRequest & | req | ) | const |
Query location information based on coordinates or (parts of) the name.
Definition at line 733 of file manager.cpp.
◆ queryStopover()
StopoverReply * Manager::queryStopover | ( | const StopoverRequest & | req | ) | const |
Query arrivals or departures from a specific station.
Definition at line 633 of file manager.cpp.
◆ queryVehicleLayout()
VehicleLayoutReply * Manager::queryVehicleLayout | ( | const VehicleLayoutRequest & | req | ) | const |
Query vehicle and platform layout information.
This is only available for some trains and some operators, so be prepared for empty results.
Definition at line 795 of file manager.cpp.
◆ setAllowInsecureBackends()
void Manager::setAllowInsecureBackends | ( | bool | insecure | ) |
Allow usage of insecure backends, that is services not using transport encryption.
Definition at line 521 of file manager.cpp.
◆ setBackendEnabled()
void Manager::setBackendEnabled | ( | const QString & | backendId, |
bool | enabled ) |
Sets whether the backend with the given identifier should be used.
- Note
- If allowInsecureBackends() is
false
, this has precedence.
Definition at line 901 of file manager.cpp.
◆ setBackendsEnabledByDefault()
void Manager::setBackendsEnabledByDefault | ( | bool | byDefault | ) |
Set wheter backends are enabled by default.
Definition at line 944 of file manager.cpp.
◆ setDisabledBackends()
void Manager::setDisabledBackends | ( | const QStringList & | backendIds | ) |
Sets the explicitly disabled backends.
Use for restoring persisted settings.
Definition at line 931 of file manager.cpp.
◆ setEnabledBackends()
void Manager::setEnabledBackends | ( | const QStringList & | backendIds | ) |
Sets the explicitly enabled backends.
Use for restoring persisted settings.
Definition at line 918 of file manager.cpp.
◆ setNetworkAccessManager()
void Manager::setNetworkAccessManager | ( | QNetworkAccessManager * | nam | ) |
Set the network access manager to use for network operations.
If not set, an instance is created internally. Ownership is not transferred.
Definition at line 503 of file manager.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Jul 26 2024 11:59:22 by doxygen 1.11.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.