KGAPI2::Drive::Permission
#include <permission.h>
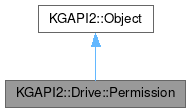
Classes | |
class | PermissionDetails |
Public Types | |
using | PermissionDetailsList = QList<PermissionDetailsPtr> |
using | PermissionDetailsPtr = QSharedPointer<PermissionDetails> |
enum | Role { UndefinedRole = -1 , OwnerRole = 0 , ReaderRole = 1 , WriterRole = 2 , CommenterRole = 3 , OrganizerRole = 4 , FileOrganizerRole = 5 } |
enum | Type { UndefinedType = -1 , TypeUser = 0 , TypeGroup = 1 , TypeDomain = 2 , TypeAnyone = 3 } |
Public Member Functions | |
Permission (const Permission &other) | |
QList< Role > | additionalRoles () const |
QString | authKey () const |
bool | deleted () const |
QString | domain () const |
QString | emailAddress () const |
QDateTime | expirationDate () const |
QString | id () const |
QString | name () const |
bool | operator!= (const Permission &other) const |
bool | operator== (const Permission &other) const |
Permission::PermissionDetailsList | permissionDetails () const |
QUrl | photoLink () const |
Permission::Role | role () const |
QUrl | selfLink () const |
void | setAdditionalRoles (const QList< Role > &additionalRoles) |
void | setId (const QString &id) |
void | setRole (Permission::Role role) |
void | setType (Permission::Type type) |
void | setValue (const QString &value) |
void | setWithLink (bool withLink) |
Permission::Type | type () const |
QString | value () const |
bool | withLink () const |
![]() | |
Object () | |
Object (const Object &other) | |
virtual | ~Object () |
QString | etag () const |
bool | operator== (const Object &other) const |
void | setEtag (const QString &etag) |
Static Public Member Functions | |
static PermissionPtr | fromJSON (const QByteArray &jsonData) |
static PermissionsList | fromJSONFeed (const QByteArray &jsonData) |
static QByteArray | toJSON (const PermissionPtr &permission) |
Detailed Description
Permission contains a permission for a file.
Getters and setters' documentation is based on Google Drive's API v2 reference
- See also
- Permissions
- Since
- 2.0
Definition at line 33 of file permission.h.
Member Typedef Documentation
◆ PermissionDetailsList
Definition at line 107 of file permission.h.
◆ PermissionDetailsPtr
Definition at line 106 of file permission.h.
Member Enumeration Documentation
◆ Role
enum KGAPI2::Drive::Permission::Role |
Definition at line 36 of file permission.h.
◆ Type
enum KGAPI2::Drive::Permission::Type |
Definition at line 46 of file permission.h.
Constructor & Destructor Documentation
◆ Permission() [1/2]
|
explicit |
Definition at line 232 of file permission.cpp.
◆ Permission() [2/2]
|
explicit |
Definition at line 238 of file permission.cpp.
◆ ~Permission()
|
override |
Definition at line 244 of file permission.cpp.
Member Function Documentation
◆ additionalRoles()
QList< Permission::Role > Permission::additionalRoles | ( | ) | const |
Returns additional roles for this user.
Only commenter is currently allowed.
Definition at line 302 of file permission.cpp.
◆ authKey()
QString Permission::authKey | ( | ) | const |
Returns the authkey parameter required for this permission.
Definition at line 322 of file permission.cpp.
◆ deleted()
bool Permission::deleted | ( | ) | const |
Whether the account associated with this permission has been deleted.
This field only pertains to user and group permissions.
Definition at line 367 of file permission.cpp.
◆ domain()
QString Permission::domain | ( | ) | const |
The domain name of the entity this permission refers to.
This is an output-only field which is present when the permission type is user, group or domain.
Definition at line 357 of file permission.cpp.
◆ emailAddress()
QString Permission::emailAddress | ( | ) | const |
The email address of the user or group this permission refers to.
This is an output-only field which is present when the permission type is user or group.
Definition at line 352 of file permission.cpp.
◆ expirationDate()
QDateTime Permission::expirationDate | ( | ) | const |
The time at which this permission will expire.
Definition at line 362 of file permission.cpp.
◆ fromJSON()
|
static |
Definition at line 377 of file permission.cpp.
◆ fromJSONFeed()
|
static |
Definition at line 389 of file permission.cpp.
◆ id()
QString Permission::id | ( | ) | const |
Returns the id of the permission.
Definition at line 272 of file permission.cpp.
◆ name()
QString Permission::name | ( | ) | const |
Returns the name of this permission.
Definition at line 287 of file permission.cpp.
◆ operator!=()
|
inline |
Definition at line 113 of file permission.h.
◆ operator==()
bool Permission::operator== | ( | const Permission & | other | ) | const |
Definition at line 249 of file permission.cpp.
◆ permissionDetails()
Permission::PermissionDetailsList Permission::permissionDetails | ( | ) | const |
Details of whether the permissions on this shared drive item are inherited or directly on this item.
Definition at line 372 of file permission.cpp.
◆ photoLink()
QUrl Permission::photoLink | ( | ) | const |
Returns a link to the profile photo, if available.
Definition at line 337 of file permission.cpp.
◆ role()
Permission::Role Permission::role | ( | ) | const |
Returns the primary role for this user.
Definition at line 292 of file permission.cpp.
◆ selfLink()
QUrl Permission::selfLink | ( | ) | const |
Returns a link back to this permission.
Definition at line 282 of file permission.cpp.
◆ setAdditionalRoles()
void Permission::setAdditionalRoles | ( | const QList< Role > & | additionalRoles | ) |
Sets additional roles for this user.
Only commenter is currently allowed.
- Parameters
-
additionalRoles
Definition at line 307 of file permission.cpp.
◆ setId()
void Permission::setId | ( | const QString & | id | ) |
◆ setRole()
void Permission::setRole | ( | Permission::Role | role | ) |
Sets the primary role for this user.
Definition at line 297 of file permission.cpp.
◆ setType()
void Permission::setType | ( | Permission::Type | type | ) |
◆ setValue()
void Permission::setValue | ( | const QString & | value | ) |
Sets the email address or domain name for the entity.
This is not populated in responses. You can use the alias "me" as the value for this property to refer to the current authorized user.
- Parameters
-
value
Definition at line 347 of file permission.cpp.
◆ setWithLink()
void Permission::setWithLink | ( | bool | withLink | ) |
Sets whether the link is required for this permission.
- Parameters
-
withLink
Definition at line 332 of file permission.cpp.
◆ toJSON()
|
static |
Definition at line 412 of file permission.cpp.
◆ type()
Permission::Type Permission::type | ( | ) | const |
Returns the account type.
Definition at line 312 of file permission.cpp.
◆ value()
QString Permission::value | ( | ) | const |
Returns the email address or domain name for the entity.
This is not populated in responses. You can use the alias "me" as the value for this property to refer to the current authorized user.
Definition at line 342 of file permission.cpp.
◆ withLink()
bool Permission::withLink | ( | ) | const |
Returns whether the link is required for this permission.
Definition at line 327 of file permission.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 3 2025 11:58:00 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.