KGAPI2::Drive::Revision
#include <revision.h>
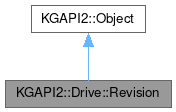
Public Member Functions | |
Revision (const Revision &other) | |
QUrl | downloadUrl () const |
QMap< QString, QUrl > | exportLinks () const |
qlonglong | fileSize () const |
QString | id () const |
UserPtr | lastModifyingUser () const |
QString | lastModifyingUserName () const |
QString | md5Checksum () const |
QString | mimeType () const |
QDateTime | modifiedDate () const |
bool | operator!= (const Revision &other) const |
bool | operator== (const Revision &other) const |
QString | originalFilename () const |
bool | pinned () const |
bool | publishAuto () const |
bool | published () const |
QUrl | publishedLink () const |
bool | publishedOutsideDomain () const |
QUrl | selfLink () const |
void | setPinned (bool pinned) |
void | setPublishAuto (bool publishAuto) |
void | setPublished (bool published) |
void | setPublishedOutsideDomain (bool publishedOutsideDomain) |
![]() | |
Object () | |
Object (const Object &other) | |
virtual | ~Object () |
QString | etag () const |
bool | operator== (const Object &other) const |
void | setEtag (const QString &etag) |
Static Public Member Functions | |
static RevisionPtr | fromJSON (const QByteArray &jsonData) |
static RevisionsList | fromJSONFeed (const QByteArray &jsonData) |
static QByteArray | toJSON (const RevisionPtr &revision) |
Detailed Description
Revision contains a revision of a file.
Getters and setters' documentation is based on Google Drive's API v2 reference
- See also
- Revisions
- Since
- 2.0
Definition at line 34 of file revision.h.
Constructor & Destructor Documentation
◆ Revision() [1/2]
|
explicit |
Definition at line 104 of file revision.cpp.
◆ Revision() [2/2]
|
explicit |
Definition at line 110 of file revision.cpp.
◆ ~Revision()
|
override |
Definition at line 116 of file revision.cpp.
Member Function Documentation
◆ downloadUrl()
|
nodiscard |
Returns a short term download URL for the file.
This will only be populated on files with content stored in Drive.
Definition at line 210 of file revision.cpp.
◆ exportLinks()
Returns the links for exporting Google Docs to specific formats.
This is a map from the export format to URL.
Definition at line 215 of file revision.cpp.
◆ fileSize()
|
nodiscard |
Returns the size of the revision in bytes.
This will only be populated on files with content stored in Drive.
Definition at line 240 of file revision.cpp.
◆ fromJSON()
|
static |
Definition at line 245 of file revision.cpp.
◆ fromJSONFeed()
|
static |
Definition at line 256 of file revision.cpp.
◆ id()
|
nodiscard |
Returns the id of the revision.
Definition at line 145 of file revision.cpp.
◆ lastModifyingUser()
|
nodiscard |
Returns object representing the last user to modify this revision.
Definition at line 225 of file revision.cpp.
◆ lastModifyingUserName()
|
nodiscard |
Returns the name of the last user to modify this revision.
Definition at line 220 of file revision.cpp.
◆ md5Checksum()
|
nodiscard |
Returns an MD5 checksum for the content of this revision.
This will only be populated on files with content stored in Drive
Definition at line 235 of file revision.cpp.
◆ mimeType()
|
nodiscard |
Returns the MIME type of the revision.
Definition at line 155 of file revision.cpp.
◆ modifiedDate()
|
nodiscard |
Returns the last time this revision was modified.
Definition at line 160 of file revision.cpp.
◆ operator!=()
|
inline |
Definition at line 41 of file revision.h.
◆ operator==()
bool Revision::operator== | ( | const Revision & | other | ) | const |
Definition at line 121 of file revision.cpp.
◆ originalFilename()
|
nodiscard |
Returns the original filename when this revision was created.
This will only be populated on files with content stored in Drive.
Definition at line 230 of file revision.cpp.
◆ pinned()
|
nodiscard |
Returns whether this revision is pinned to prevent automatic purging.
This will only be populated and can only be modified on files with content stored in Drive which are not Google Docs.
Revisions can also be pinned when they are created through the drive.files.insert/update/copy by using the pinned query parameter.
Definition at line 165 of file revision.cpp.
◆ publishAuto()
|
nodiscard |
Returns whether subsequent revisions will be automatically republished.
This is only populated and can only be modified for Google Docs.
Definition at line 190 of file revision.cpp.
◆ published()
|
nodiscard |
Returns whether this revision is published.
This is only populated and can only be modified for Google Docs.
Definition at line 175 of file revision.cpp.
◆ publishedLink()
|
nodiscard |
Returns a link to the published revision.
Definition at line 185 of file revision.cpp.
◆ publishedOutsideDomain()
|
nodiscard |
Returns whether this revision is published outside the domain.
This is only populated and can only be modified for Google Docs.
Definition at line 200 of file revision.cpp.
◆ selfLink()
|
nodiscard |
Returns a link back to this revision.
Definition at line 150 of file revision.cpp.
◆ setPinned()
void Revision::setPinned | ( | bool | pinned | ) |
Sets whether this revision is pinned to prevent automatic purging.
This will only be populated and can only be modified on files with content stored in Drive which are not Google Docs.
Revisions can also be pinned when they are created through the drive.files.insert/update/copy by using the pinned query parameter.
- Parameters
-
pinned
Definition at line 170 of file revision.cpp.
◆ setPublishAuto()
void Revision::setPublishAuto | ( | bool | publishAuto | ) |
Sets whether subsequent revisions will be automatically republished.
This is only populated and can only be modified for Google Docs.
- Parameters
-
publishAuto
Definition at line 195 of file revision.cpp.
◆ setPublished()
void Revision::setPublished | ( | bool | published | ) |
Sets whether this revision is published.
- Parameters
-
published
Definition at line 180 of file revision.cpp.
◆ setPublishedOutsideDomain()
void Revision::setPublishedOutsideDomain | ( | bool | publishedOutsideDomain | ) |
Sets whether this revision is published outside the domain.
This is only populated and can only be modified for Google Docs.
- Parameters
-
publishedOutsideDomain
Definition at line 205 of file revision.cpp.
◆ toJSON()
|
static |
Definition at line 283 of file revision.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:03:45 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.