Kleo::KeyResolver
#include <keyresolver.h>
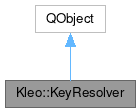
Classes | |
struct | Solution |
Signals | |
void | keysResolved (bool success, bool sendUnencrypted) |
Public Member Functions | |
KeyResolver (bool encrypt, bool sign, GpgME::Protocol protocol=GpgME::UnknownProtocol, bool allowMixed=true) | |
Solution | result () const |
void | setDialogWindowFlags (Qt::WindowFlags flags) |
void | setMinimumValidity (int validity) |
void | setOverrideKeys (const QMap< GpgME::Protocol, QMap< QString, QStringList > > &overrides) |
void | setPreferredProtocol (GpgME::Protocol proto) |
void | setRecipients (const QStringList &addresses) |
void | setSender (const QString &sender) |
void | setSigningKeys (const QStringList &fingerprints) |
void | start (bool showApproval, QWidget *parentWidget=nullptr) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Class to find Keys for E-Mail signing and encryption.
The KeyResolver uses the Keycache to find keys for signing or encryption.
Overrides can be provided for address book integration.
If no override key(s) are provided for an address and no KeyGroup for this address is found, then the key with a uid that matches the address and has the highest validity is used. If both keys have the same validity, then the key with the newest subkey is used.
The KeyResolver also supports groups so the number of encryption keys does not necessarily need to match the amount of sender addresses. For this reason maps are used to map addresses to lists of keys.
The keys can be OpenPGP keys and S/MIME (CMS) keys. As a caller you need to partition the keys by their protocol and send one message for each protocol for the recipients and signed by the signing keys.
Definition at line 57 of file keyresolver.h.
Constructor & Destructor Documentation
◆ KeyResolver()
|
explicit |
Creates a new key resolver object.
- Parameters
-
encrypt Should encryption keys be selected. sign Should signing keys be selected. protocol A specific key protocol (OpenPGP, S/MIME) for selection. Default: Both protocols. allowMixed Specify if multiple message formats may be resolved.
Definition at line 172 of file keyresolver.cpp.
Member Function Documentation
◆ keysResolved
|
signal |
Emitted when key resolution finished.
- Parameters
-
success The general result. If true continue sending, if false abort. sendUnencrypted If there could be no key found for one of the recipients the user was queried if the mail should be sent out unencrypted. sendUnencrypted is true if the user agreed to this.
◆ result()
KeyResolver::Solution KeyResolver::result | ( | ) | const |
Get the result of the resolution.
- Returns
- the resolved keys for signing and encryption.
Definition at line 199 of file keyresolver.cpp.
◆ setDialogWindowFlags()
void KeyResolver::setDialogWindowFlags | ( | Qt::WindowFlags | flags | ) |
Set window flags for a possible dialog.
Definition at line 204 of file keyresolver.cpp.
◆ setMinimumValidity()
void KeyResolver::setMinimumValidity | ( | int | validity | ) |
Set the minimum user id validity for autoresolution.
The default value is marginal
- Parameters
-
validity int representation of a GpgME::UserID::Validity.
Definition at line 214 of file keyresolver.cpp.
◆ setOverrideKeys()
void KeyResolver::setOverrideKeys | ( | const QMap< GpgME::Protocol, QMap< QString, QStringList > > & | overrides | ) |
Set up possible override keys for recipients addresses.
The keys for the fingerprints are looked up and used when found.
Overrides for GpgME::UnknownProtocol
are used regardless of the protocol. Overrides for a specific protocol are only used for this protocol. Overrides for GpgME::UnknownProtocol
takes precedence over overrides for a specific protocol.
- Parameters
-
overrides A map of <protocol> -> (<address> <fingerprints>)
Definition at line 189 of file keyresolver.cpp.
◆ setPreferredProtocol()
void KeyResolver::setPreferredProtocol | ( | GpgME::Protocol | proto | ) |
Set the protocol that is preferred to be displayed first when it is not clear from the keys.
E.g. if both OpenPGP and S/MIME can be resolved.
Definition at line 209 of file keyresolver.cpp.
◆ setRecipients()
void KeyResolver::setRecipients | ( | const QStringList & | addresses | ) |
Set the list of recipient addresses.
- Parameters
-
addresses A list of (not necessarily normalized) email addresses
Definition at line 179 of file keyresolver.cpp.
◆ setSender()
void KeyResolver::setSender | ( | const QString & | sender | ) |
Set the sender's address.
This address is added to the list of recipients (for encryption to self) and it is used for signing key resolution, if the signing keys are not explicitly set through setSigningKeys.
- Parameters
-
sender The sender of this message.
Definition at line 184 of file keyresolver.cpp.
◆ setSigningKeys()
void KeyResolver::setSigningKeys | ( | const QStringList & | fingerprints | ) |
Set explicit signing keys to use.
Definition at line 194 of file keyresolver.cpp.
◆ start()
void KeyResolver::start | ( | bool | showApproval, |
QWidget * | parentWidget = nullptr ) |
Starts the key resolving procedure.
Emits keysResolved on success or error.
- Parameters
-
showApproval If set to true a dialog listing the keys will always be shown. parentWidget Optional, a Widget to use as parent for dialogs.
Definition at line 152 of file keyresolver.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 11 2025 11:53:31 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.