MailCommon::FilterManager
#include <filtermanager.h>
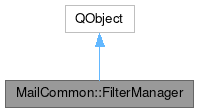
Public Types | |
enum | FilterSet { NoSet = 0x0 , Inbound = 0x1 , Outbound = 0x2 , Explicit = 0x4 , BeforeOutbound = 0x8 , AllFolders = 0x16 , All = Inbound | BeforeOutbound | Outbound | Explicit | AllFolders } |
Signals | |
void | filtersChanged () |
void | loadingFiltersDone () |
void | tagListingFinished () |
Public Member Functions | |
void | appendFilters (const QList< MailCommon::MailFilter * > &filters, bool replaceIfNameExists=false) |
void | beginUpdate () |
void | cleanup () |
QString | createUniqueFilterName (const QString &name) const |
void | endUpdate () |
void | filter (const Akonadi::Collection &collection, const QStringList &listFilters) const |
void | filter (const Akonadi::Collection &collection, FilterSet set=Explicit) const |
void | filter (const Akonadi::Collection::List &collections, const QStringList &listFilters, FilterSet set=Explicit) const |
void | filter (const Akonadi::Collection::List &collections, FilterSet set=Explicit) const |
void | filter (const Akonadi::Item &item, const QString &identifier, const QString &resourceId) const |
void | filter (const Akonadi::Item &item, FilterSet set=Inbound, bool account=false, const QString &resourceId=QString()) const |
void | filter (const Akonadi::Item::List &messages, FilterSet set=Explicit) const |
void | filter (const Akonadi::Item::List &messages, SearchRule::RequiredPart requiredPart, const QStringList &listFilters) const |
QList< MailCommon::MailFilter * > | filters () const |
bool | initialized () const |
bool | isValid () const |
void | removeFilter (MailCommon::MailFilter *filter) |
void | setFilters (const QList< MailCommon::MailFilter * > &filters) |
void | showFilterLogDialog (qlonglong windowId) |
QMap< QUrl, QString > | tagList () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static FilterActionDict * | filterActionDict () |
static FilterManager * | instance () |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
A wrapper class that allows easy access to the mail filters.
This class communicates with the mailfilter agent via DBus.
Definition at line 26 of file filtermanager.h.
Member Enumeration Documentation
◆ FilterSet
Describes the list of filters.
Enumerator | |
---|---|
AllFolders | Apply the filter on all folders, not just inbox. |
Definition at line 35 of file filtermanager.h.
Constructor & Destructor Documentation
◆ ~FilterManager()
|
override |
Definition at line 126 of file filtermanager.cpp.
Member Function Documentation
◆ appendFilters()
void FilterManager::appendFilters | ( | const QList< MailCommon::MailFilter * > & | filters, |
bool | replaceIfNameExists = false ) |
Manage filters interface.
Appends the list of filters
to the current list of filters and write everything back into the configuration. The filter manager takes ownership of the filters in the list.
Definition at line 295 of file filtermanager.cpp.
◆ beginUpdate()
void FilterManager::beginUpdate | ( | ) |
Should be called at the beginning of an filter list update.
Definition at line 323 of file filtermanager.cpp.
◆ cleanup()
void FilterManager::cleanup | ( | ) |
Definition at line 131 of file filtermanager.cpp.
◆ createUniqueFilterName()
Checks for existing filters with the name
and extend the "name" to "name (i)" until no match is found for i=1..n.
Definition at line 207 of file filtermanager.cpp.
◆ endUpdate()
void FilterManager::endUpdate | ( | ) |
Should be called at the end of an filter list update.
Definition at line 327 of file filtermanager.cpp.
◆ filter() [1/8]
void FilterManager::filter | ( | const Akonadi::Collection & | collection, |
const QStringList & | listFilters ) const |
Apply specified filters on all messages in given collection.
Definition at line 243 of file filtermanager.cpp.
◆ filter() [2/8]
void FilterManager::filter | ( | const Akonadi::Collection & | collection, |
FilterSet | set = Explicit ) const |
Process all messages in given collection by applying the filters rules one by one.
You can select which set of filters (incoming or outgoing) should be used.
Definition at line 227 of file filtermanager.cpp.
◆ filter() [3/8]
void FilterManager::filter | ( | const Akonadi::Collection::List & | collections, |
const QStringList & | listFilters, | ||
FilterSet | set = Explicit ) const |
Apply specified filters on all messages in given collection.
Definition at line 248 of file filtermanager.cpp.
◆ filter() [4/8]
void FilterManager::filter | ( | const Akonadi::Collection::List & | collections, |
FilterSet | set = Explicit ) const |
Process all messages in given collections by applying the filters rules one by one.
You can select which set of filters (incoming or outgoing) should be used.
Definition at line 232 of file filtermanager.cpp.
◆ filter() [5/8]
void FilterManager::filter | ( | const Akonadi::Item & | item, |
const QString & | identifier, | ||
const QString & | resourceId ) const |
Apply filters interface.
Applies filter with the given identifier
on the message item
.
- Returns
true
on success,false
otherwise.
Definition at line 217 of file filtermanager.cpp.
◆ filter() [6/8]
void FilterManager::filter | ( | const Akonadi::Item & | item, |
FilterSet | set = Inbound, | ||
bool | account = false, | ||
const QString & | resourceId = QString() ) const |
Process given message item by applying the filter rules one by one.
You can select which set of filters (incoming or outgoing) should be used.
- Parameters
-
item The message item to process. set Select the filter set to use. account true
if an account id is specified elsefalse
accountId The id of the resource that the message was retrieved from
Definition at line 222 of file filtermanager.cpp.
◆ filter() [7/8]
void FilterManager::filter | ( | const Akonadi::Item::List & | messages, |
FilterManager::FilterSet | set = Explicit ) const |
Process given messages
by applying the filter rules one by one.
You can select which set of filters (incoming or outgoing) should be used.
- Parameters
-
item The message item to process. set Select the filter set to use.
Definition at line 259 of file filtermanager.cpp.
◆ filter() [8/8]
void FilterManager::filter | ( | const Akonadi::Item::List & | messages, |
SearchRule::RequiredPart | requiredPart, | ||
const QStringList & | listFilters ) const |
Definition at line 271 of file filtermanager.cpp.
◆ filterActionDict()
|
static |
Returns the global filter action dictionary.
Definition at line 96 of file filtermanager.cpp.
◆ filters()
|
nodiscard |
Returns the filter list of the manager.
Definition at line 290 of file filtermanager.cpp.
◆ filtersChanged
|
signal |
This signal is emitted whenever the filter list has been updated.
◆ initialized()
|
nodiscard |
Definition at line 151 of file filtermanager.cpp.
◆ instance()
|
static |
Returns the global filter manager object.
Definition at line 87 of file filtermanager.cpp.
◆ isValid()
|
nodiscard |
Returns whether the filter manager is in a usable state.
Definition at line 202 of file filtermanager.cpp.
◆ removeFilter()
void FilterManager::removeFilter | ( | MailCommon::MailFilter * | filter | ) |
Removes the given filter
from the list.
The filter object is not deleted.
Definition at line 316 of file filtermanager.cpp.
◆ setFilters()
void FilterManager::setFilters | ( | const QList< MailCommon::MailFilter * > & | filters | ) |
Replace the list of filters of the filter manager with the given list of filters
.
The manager takes ownership of the filters.
Definition at line 282 of file filtermanager.cpp.
◆ showFilterLogDialog()
void FilterManager::showFilterLogDialog | ( | qlonglong | windowId | ) |
Shows the filter log dialog.
This is used to debug problems with filters.
Definition at line 212 of file filtermanager.cpp.
◆ tagList()
Definition at line 197 of file filtermanager.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Mar 28 2025 11:51:59 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.