MailCommon::SearchRule
#include <searchrule.h>
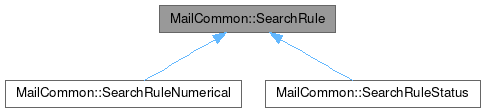
Public Types | |
enum | Function { FuncNone = -1 , FuncContains = 0 , FuncContainsNot , FuncEquals , FuncNotEqual , FuncRegExp , FuncNotRegExp , FuncIsGreater , FuncIsLessOrEqual , FuncIsLess , FuncIsGreaterOrEqual , FuncIsInAddressbook , FuncIsNotInAddressbook , FuncIsInCategory , FuncIsNotInCategory , FuncHasAttachment , FuncHasNoAttachment , FuncStartWith , FuncNotStartWith , FuncEndWith , FuncNotEndWith , FuncHasInvitation , FuncHasNoInvitation } |
using | Ptr = std::shared_ptr<SearchRule> |
enum | RequiredPart { Envelope = 0 , Header , CompleteMessage } |
Public Member Functions | |
SearchRule (const QByteArray &field=QByteArray(), Function function=FuncContains, const QString &contents=QString()) | |
SearchRule (const SearchRule &other) | |
virtual | ~SearchRule () |
virtual void | addQueryTerms (Akonadi::SearchTerm &groupTerm, bool &emptyIsNotAnError) const |
const QString | asString () const |
QString | contents () const |
QByteArray | field () const |
Function | function () const |
void | generateSieveScript (QStringList &requireModules, QString &code) |
virtual QString | informationAboutNotValidRules () const |
virtual bool | isEmpty () const =0 |
virtual bool | matches (const Akonadi::Item &item) const =0 |
const SearchRule & | operator= (const SearchRule &other) |
QDataStream & | operator>> (QDataStream &) const |
virtual SearchRule::RequiredPart | requiredPart () const =0 |
void | setContents (const QString &contents) |
void | setField (const QByteArray &name) |
void | setFunction (Function function) |
void | writeConfig (KConfigGroup &group, int index) const |
Static Public Member Functions | |
static SearchRule::Ptr | createInstance (const QByteArray &field, const char *function, const QString &contents) |
static SearchRule::Ptr | createInstance (const QByteArray &field=QByteArray(), Function function=FuncContains, const QString &contents=QString()) |
static SearchRule::Ptr | createInstance (const SearchRule &other) |
static SearchRule::Ptr | createInstance (QDataStream &stream) |
static SearchRule::Ptr | createInstanceFromConfig (const KConfigGroup &group, int index) |
Protected Member Functions | |
Akonadi::SearchTerm::Condition | akonadiComparator () const |
bool | isNegated () const |
Detailed Description
This class represents one search pattern rule.
Incoming mail is sent through the list of mail filter rules before it is placed in the associated mail folder (usually "inbox"). This class represents one mail filter rule. It is also used to represent a search rule as used by the search dialog and folders.
Definition at line 23 of file searchrule.h.
Member Typedef Documentation
◆ Ptr
using MailCommon::SearchRule::Ptr = std::shared_ptr<SearchRule> |
Defines a pointer to a search rule.
Definition at line 29 of file searchrule.h.
Member Enumeration Documentation
◆ Function
Describes operators for comparison of field and contents.
If you change the order or contents of the enum: do not forget to change funcConfigNames[], sFilterFuncList and matches() in SearchRule, too. Also, it is assumed that these functions come in pairs of logical opposites (ie. "=" <-> "!=", ">" <-> "<=", etc.).
Definition at line 40 of file searchrule.h.
◆ RequiredPart
Possible required parts.
Enumerator | |
---|---|
Envelope | Envelope. |
Header | Header. |
CompleteMessage | Whole message. |
Definition at line 70 of file searchrule.h.
Constructor & Destructor Documentation
◆ SearchRule() [1/2]
|
explicit |
Creates new new search rule.
- Parameters
-
field The field to search in. function The function to use for searching. contents The contents to search for.
Definition at line 55 of file searchrule.cpp.
◆ SearchRule() [2/2]
|
default |
Creates a new search rule from an other
rule.
◆ ~SearchRule()
|
virtualdefault |
Destroys the search rule.
Member Function Documentation
◆ addQueryTerms()
|
inlinevirtual |
Adds query terms to the given term group.
Reimplemented in MailCommon::SearchRuleNumerical, and MailCommon::SearchRuleStatus.
Definition at line 233 of file searchrule.h.
◆ akonadiComparator()
|
nodiscardprotected |
Converts the rule function into the corresponding Akonadi query operator.
Definition at line 542 of file searchrule.cpp.
◆ asString()
|
nodiscard |
Returns the rule as string for debugging purpose.
Definition at line 533 of file searchrule.cpp.
◆ contents()
|
nodiscard |
Returns the contents of the rule.
Definition at line 528 of file searchrule.cpp.
◆ createInstance() [1/4]
|
static |
Creates a new search rule of a certain type by instantiating the appropriate subclass depending on the field
.
- Parameters
-
field The field to search in. function The name of the function to use for searching. contents The contents to search for.
Definition at line 101 of file searchrule.cpp.
◆ createInstance() [2/4]
|
static |
Creates a new search rule of a certain type by instantiating the appropriate subclass depending on the field
.
- Parameters
-
field The field to search in. function The function to use for searching. contents The contents to search for.
Definition at line 79 of file searchrule.cpp.
◆ createInstance() [3/4]
|
static |
Creates a new search rule by cloning an other
rule.
Definition at line 106 of file searchrule.cpp.
◆ createInstance() [4/4]
|
static |
Creates a new search rule by deseralizing its structure from a data stream
.
Definition at line 130 of file searchrule.cpp.
◆ createInstanceFromConfig()
|
static |
Creates a new search rule from a given config group
.
- Parameters
-
group The config group to read the structure from. index The identifier that is used to distinguish rules within a single config group.
- Note
- This function does no validation of the data obtained from the config file. You should call isEmpty yourself if you need valid rules.
Definition at line 111 of file searchrule.cpp.
◆ field()
|
nodiscard |
Returns the message header field name (without the trailing ':').
There are also six pseudo-headers:
- <message>: Try to match against the whole message.
- <body>: Try to match against the body of the message.
- <any header>: Try to match against any header field.
- <recipients>: Try to match against both To: and Cc: header fields.
- <size>: Try to match against size of message (numerical).
- <age in days>: Try to match against age of message (numerical).
- <status>: Try to match against status of message (status).
- <tag>: Try to match against message tags.
Definition at line 518 of file searchrule.cpp.
◆ function()
SearchRule::Function SearchRule::function | ( | ) | const |
Returns the filter function of the rule.
Definition at line 508 of file searchrule.cpp.
◆ generateSieveScript()
void SearchRule::generateSieveScript | ( | QStringList & | requireModules, |
QString & | code ) |
Definition at line 257 of file searchrule.cpp.
◆ informationAboutNotValidRules()
|
inlinevirtual |
Definition at line 240 of file searchrule.h.
◆ isEmpty()
|
pure virtual |
Determines whether the rule is worth considering.
It isn't if either the field is not set or the contents is empty. The calling code should make sure that it's rule list contains only non-empty rules, as matches doesn't check this.
Implemented in MailCommon::SearchRuleNumerical, and MailCommon::SearchRuleStatus.
◆ isNegated()
|
nodiscardprotected |
Helper that returns whether the rule has a negated function.
Definition at line 583 of file searchrule.cpp.
◆ matches()
|
pure virtual |
Tries to match the rule against the KMime::Message in the given item
.
- Returns
- true if the rule matched, false otherwise.
- Note
- Must be implemented by subclasses.
Implemented in MailCommon::SearchRuleNumerical, and MailCommon::SearchRuleStatus.
◆ operator=()
const SearchRule & SearchRule::operator= | ( | const SearchRule & | other | ) |
Initializes this rule with an other
rule.
Definition at line 66 of file searchrule.cpp.
◆ operator>>()
QDataStream & SearchRule::operator>> | ( | QDataStream & | s | ) | const |
Definition at line 603 of file searchrule.cpp.
◆ requiredPart()
|
pure virtual |
Returns the required part from the item that is needed for the search to operate.
See RequiredPart
Implemented in MailCommon::SearchRuleNumerical, and MailCommon::SearchRuleStatus.
◆ setContents()
void SearchRule::setContents | ( | const QString & | contents | ) |
Set the contents
of the rule.
This can be either a substring to search for in or a regexp pattern to match against the header.
Definition at line 523 of file searchrule.cpp.
◆ setField()
void SearchRule::setField | ( | const QByteArray & | name | ) |
Sets the message header field name
.
- Note
- Make sure the name contains no trailing ':'.
Definition at line 513 of file searchrule.cpp.
◆ setFunction()
void SearchRule::setFunction | ( | Function | function | ) |
Sets the filter function
of the rule.
Definition at line 503 of file searchrule.cpp.
◆ writeConfig()
void SearchRule::writeConfig | ( | KConfigGroup & | group, |
int | index ) const |
Saves the object into a given config group
.
- Parameters
-
group The config group. index The identifier that is used to distinguish rules within a single config group.
- Note
- This function will happily write itself even when it's not valid, assuming higher layers to Do The Right Thing(TM).
Definition at line 168 of file searchrule.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:53:47 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.