KMime::Message
#include <message.h>
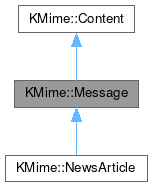
Public Types | |
typedef QSharedPointer< Message > | Ptr |
![]() | |
enum | DecodedTextTrimOption { NoTrim , TrimNewlines , TrimSpaces } |
typedef QList< KMime::Content * > | List |
Static Public Member Functions | |
static QString | mimeType () |
Protected Member Functions | |
QByteArray | assembleHeaders () override |
Detailed Description
Represents a (email) message.
Sample how to create a multipart message:
Member Typedef Documentation
◆ Ptr
typedef QSharedPointer<Message> KMime::Message::Ptr |
Constructor & Destructor Documentation
◆ Message()
|
default |
Creates an empty Message.
◆ ~Message()
|
overridedefault |
Destroys this Message.
Member Function Documentation
◆ assembleHeaders()
|
overrideprotectedvirtual |
Reimplement this method if you need to assemble additional headers in a derived class.
Don't forget to call the implementation of the base class.
- Returns
- The raw, assembled headers.
Reimplemented from KMime::Content.
Reimplemented in KMime::NewsArticle.
Definition at line 22 of file message.cpp.
◆ bcc() [1/2]
|
nodiscard |
Returns the Bcc header.
Can be nullptr
if the header doesn't exist.
- Since
- 24.08
◆ bcc() [2/2]
KMime::Headers::Bcc * KMime::Message::bcc | ( | bool | create = true | ) |
Returns the Bcc header.
- Parameters
-
create If true, create the header if it doesn't exist yet.
◆ cc() [1/2]
|
nodiscard |
Returns the Cc header.
Can be nullptr
if the header doesn't exist.
- Since
- 24.08
◆ cc() [2/2]
KMime::Headers::Cc * KMime::Message::cc | ( | bool | create = true | ) |
Returns the Cc header.
- Parameters
-
create If true, create the header if it doesn't exist yet.
◆ date() [1/2]
|
nodiscard |
Returns the Date header.
Can be nullptr
if the header doesn't exist.
- Since
- 24.08
◆ date() [2/2]
KMime::Headers::Date * KMime::Message::date | ( | bool | create = true | ) |
Returns the Date header.
- Parameters
-
create If true, create the header if it doesn't exist yet.
◆ from() [1/2]
|
nodiscard |
Returns the From header.
Can be nullptr
if the header doesn't exist.
- Since
- 24.08
◆ from() [2/2]
KMime::Headers::From * KMime::Message::from | ( | bool | create = true | ) |
Returns the From header.
- Parameters
-
create If true, create the header if it doesn't exist yet.
◆ inReplyTo() [1/2]
|
nodiscard |
Returns the In-Reply-To header.
Can be nullptr
if the header doesn't exist.
- Since
- 24.08
◆ inReplyTo() [2/2]
KMime::Headers::InReplyTo * KMime::Message::inReplyTo | ( | bool | create = true | ) |
Returns the In-Reply-To header.
- Parameters
-
create If true, create the header if it doesn't exist yet.
◆ mainBodyPart() [1/2]
|
nodiscard |
Returns the first main body part of a given type, taking multipart/mixed and multipart/alternative nodes into consideration.
Eg. bodyPart
("text/html") will return a html content object if that is provided in a multipart/alternative node, but not if it's the non-first child node of a multipart/mixed node (ie. an attachment).
- Parameters
-
type The mimetype of the body part, if not given, the first body part will be returned, regardless of it's type.
Definition at line 36 of file message.cpp.
◆ mainBodyPart() [2/2]
|
nodiscard |
Definition at line 42 of file message.cpp.
◆ messageID() [1/2]
|
nodiscard |
Returns the Message-ID header.
Can be nullptr
if the header doesn't exist.
- Since
- 24.08
◆ messageID() [2/2]
KMime::Headers::MessageID * KMime::Message::messageID | ( | bool | create = true | ) |
Returns the Message-ID header.
- Parameters
-
create If true, create the header if it doesn't exist yet.
◆ mimeType()
|
staticnodiscard |
Returns the MIME type used for Messages.
Definition at line 80 of file message.cpp.
◆ organization() [1/2]
|
nodiscard |
Returns the Organization header.
Can be nullptr
if the header doesn't exist.
- Since
- 24.08
◆ organization() [2/2]
KMime::Headers::Organization * KMime::Message::organization | ( | bool | create = true | ) |
Returns the Organization header.
- Parameters
-
create If true, create the header if it doesn't exist yet.
◆ references() [1/2]
|
nodiscard |
Returns the References header.
Can be nullptr
if the header doesn't exist.
- Since
- 24.08
◆ references() [2/2]
KMime::Headers::References * KMime::Message::references | ( | bool | create = true | ) |
Returns the References header.
- Parameters
-
create If true, create the header if it doesn't exist yet.
◆ replyTo() [1/2]
|
nodiscard |
Returns the Reply-To header.
Can be nullptr
if the header doesn't exist.
- Since
- 24.08
◆ replyTo() [2/2]
KMime::Headers::ReplyTo * KMime::Message::replyTo | ( | bool | create = true | ) |
Returns the Reply-To header.
- Parameters
-
create If true, create the header if it doesn't exist yet.
◆ sender() [1/2]
|
nodiscard |
Returns the Sender header.
Can be nullptr
if the header doesn't exist.
- Since
- 24.08
◆ sender() [2/2]
KMime::Headers::Sender * KMime::Message::sender | ( | bool | create = true | ) |
Returns the Sender header.
- Parameters
-
create If true, create the header if it doesn't exist yet.
◆ subject() [1/2]
|
nodiscard |
Returns the Subject header.
Can be nullptr
if the header doesn't exist.
- Since
- 24.08
◆ subject() [2/2]
KMime::Headers::Subject * KMime::Message::subject | ( | bool | create = true | ) |
Returns the Subject header.
- Parameters
-
create If true, create the header if it doesn't exist yet.
◆ to() [1/2]
|
nodiscard |
Returns the To header.
Can be nullptr
if the header doesn't exist.
- Since
- 24.08
◆ to() [2/2]
KMime::Headers::To * KMime::Message::to | ( | bool | create = true | ) |
Returns the To header.
- Parameters
-
create If true, create the header if it doesn't exist yet.
◆ userAgent() [1/2]
|
nodiscard |
Returns the User-Agent header.
Can be nullptr
if the header doesn't exist.
- Since
- 24.08
◆ userAgent() [2/2]
KMime::Headers::UserAgent * KMime::Message::userAgent | ( | bool | create = true | ) |
Returns the User-Agent header.
- Parameters
-
create If true, create the header if it doesn't exist yet.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:04:14 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.