KMime::Content
#include <content.h>
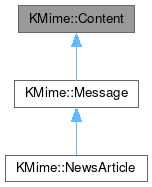
Public Types | |
typedef QList< KMime::Content * > | List |
Protected Member Functions | |
virtual QByteArray | assembleHeaders () |
Detailed Description
A class that encapsulates MIME encoded Content.
A Content object holds two representations of a content:
- the string representation: This is the content encoded as a string ready for transport. Accessible through the encodedContent() method.
- the broken-down representation: This is the tree of objects (headers, sub-Contents and (if present) the encapsulated message) that this Content is made of. Accessible through methods like header(), contents() and bodyAsMessage().
The parse() function updates the broken-down representation of the Content from its string representation. Calling it is necessary to access the headers, sub-Contents or the encapsulated message of this Content.
The assemble() function updates the string representation of the Content from its broken-down representation. Calling it is necessary for encodedContent() to reflect any changes made to the broken-down representation of the Content.
There are two basic types of a Content:
- A leaf Content: This is a content that is neither a multipart content nor an encapsulated message. Because of this, it will not have any children, it has no sub-contents and is therefore a leaf content. Only leaf contents have a body that is not empty, i.e. functions that operate on the body, such as body(), size() and decodedContent(), will work only on leaf contents.
- A non-leaf Content: This is a content that itself doesn't have any body, but that does have sub-contents. This is the case for contents that are of mimetype multipart/ or of mimetype message/rfc822. In case of a multipart content, contents() will return the multipart child contents. In case of an encapsulated message, the message can be accessed with bodyAsMessage(), and contents() will have one entry that is the message as well. On a non-leaf content, body() will have an empty return value and other functions working on the body will not work. A call to parse() is required before the child multipart contents or the encapsulated message is created.
Member Typedef Documentation
◆ List
typedef QList<KMime::Content *> KMime::Content::List |
Constructor & Destructor Documentation
◆ Content()
|
explicit |
Creates an empty Content object with a specified parent.
- Parameters
-
parent the parent Content object
- Since
- 4.3
Definition at line 40 of file content.cpp.
◆ ~Content()
|
virtual |
Destroys this Content object.
Definition at line 46 of file content.cpp.
Member Function Documentation
◆ appendContent()
void KMime::Content::appendContent | ( | Content * | content | ) |
Appends a new sub-Content.
If the sub-Content is already part of another Content object, it is removed from there and its parent is updated.
- Parameters
-
content The new sub-Content.
- See also
- prependContent()
- takeContent()
- Since
- 6.0
Definition at line 488 of file content.cpp.
◆ appendHeader()
void KMime::Content::appendHeader | ( | Headers::Base * | h | ) |
Appends the specified header to the headers of this Content.
- Parameters
-
h The header to append.
- Since
- 4.4
Definition at line 597 of file content.cpp.
◆ assemble()
void KMime::Content::assemble | ( | ) |
Generates the MIME content.
This means the string representation of this Content is updated from the broken-down object representation. Call this if you have made changes to the content, and want encodedContent() to reflect those changes.
- Note
- assemble() has no effect if the Content isFrozen(). You may want to freeze, for instance, signed sub-Contents, to make sure they are kept unmodified.
- If this content is an encapsulated message, i.e. bodyIsMessage() returns true, then calling assemble() will also assemble the message returned by bodyAsMessage().
- Warning
- assemble() may change the order of the headers, and other details such as where folding occurs. This may break things like signature verification, so you should ONLY call assemble() when you have actually modified the content.
Definition at line 190 of file content.cpp.
◆ assembleHeaders()
|
protectedvirtual |
Reimplement this method if you need to assemble additional headers in a derived class.
Don't forget to call the implementation of the base class.
- Returns
- The raw, assembled headers.
Reimplemented in KMime::Message, and KMime::NewsArticle.
Definition at line 204 of file content.cpp.
◆ attachments()
Returns all attachments below this node, recursively.
This does not include crypto parts, nodes of alternative or related multipart nodes, or the primary body part (see textContent()).
- See also
- KMime::isAttachment(), KMime::hasAttachment()
Definition at line 449 of file content.cpp.
◆ body()
|
nodiscard |
Returns the Content body raw data.
Note that this will be empty for multipart contents or for encapsulated messages, after parse() has been called.
- See also
- setBody().
Definition at line 79 of file content.cpp.
◆ bodyAsMessage()
|
nodiscard |
If this content is an encapsulated message, in which case bodyIsMessage() will return true, the message represented by the body of this content will be returned.
The returned message is already fully parsed. Calling this method is the aquivalent of calling contents().first() and casting the result to a KMime::Message*. bodyAsMessage() has the advantage that it will return a shared pointer that will not be destroyed when the container message is destroyed or re-parsed.
The message that is returned here is created when calling parse(), so make sure to call parse() first. Since each parse() creates a new message object, a different message object will be returned each time you call parse().
If you make changes to the returned message, you need to call assemble() on this content or on the message if you want that encodedContent() reflects these changes. This also means that calling assemble() on this content will assemble the returned message.
- Since
- 4.5
Definition at line 795 of file content.cpp.
◆ bodyIsMessage()
|
nodiscard |
- Returns
- true if this content is an encapsulated message, i.e. if it has the mimetype message/rfc822.
- Since
- 4.5
Definition at line 804 of file content.cpp.
◆ changeEncoding()
void KMime::Content::changeEncoding | ( | Headers::contentEncoding | e | ) |
Changes the encoding of this Content to e
.
If the Content is binary, this actually re-encodes the data to use the new encoding.
- Parameters
-
e The new encoding to use.
Definition at line 532 of file content.cpp.
◆ clear()
void KMime::Content::clear | ( | ) |
Clears the content, deleting all headers and sub-Contents.
Definition at line 217 of file content.cpp.
◆ clearContents()
void KMime::Content::clearContents | ( | bool | del = true | ) |
Removes all sub-Contents from this content.
Deletes them if del
is true. This is different from calling removeContent() on each sub-Content, because removeContent() will convert this to a single-part Content if only one sub-Content is left. Calling clearContents() does NOT make this Content single-part.
- Parameters
-
del Whether to delete the sub-Contents.
- See also
- removeContent()
- Since
- 4.4
Definition at line 227 of file content.cpp.
◆ content()
|
nodiscard |
◆ contentDescription() [1/2]
|
nodiscard |
Returns the Content-Description header.
Can be nullptr
if the header doesn't exist.
- Since
- 24.08
◆ contentDescription() [2/2]
Headers::ContentDescription * KMime::Content::contentDescription | ( | bool | create = true | ) |
Returns the Content-Description header.
- Parameters
-
create If true, create the header if it doesn't exist yet.
◆ contentDisposition() [1/2]
|
nodiscard |
Returns the Content-Disposition header.
Can be nullptr
if the header doesn't exist.
- Since
- 24.08
◆ contentDisposition() [2/2]
Headers::ContentDisposition * KMime::Content::contentDisposition | ( | bool | create = true | ) |
Returns the Content-Disposition header.
- Parameters
-
create If true, create the header if it doesn't exist yet.
◆ contentID() [1/2]
|
nodiscard |
Returns the Content-ID header.
Can be nullptr
if the header doesn't exist.
- Since
- 24.08
◆ contentID() [2/2]
Headers::ContentID * KMime::Content::contentID | ( | bool | create = true | ) |
Returns the Content-ID header.
- Parameters
-
create if true, create the header if it does not exist yet.
- Since
- 4.4
◆ contentLocation() [1/2]
|
nodiscard |
Returns the Content-Location header.
Can be nullptr
if the header doesn't exist.
- Since
- 24.08
◆ contentLocation() [2/2]
Headers::ContentLocation * KMime::Content::contentLocation | ( | bool | create = true | ) |
Returns the Content-Location header.
- Parameters
-
create If true, create the header if it doesn't exist yet.
- Since
- 4.2
◆ contents()
For multipart contents, this will return a list of all multipart child contents.
For contents that are of mimetype message/rfc822, this will return a list with one entry, and that entry is the encapsulated message, as it would be returned by bodyAsMessage().
Definition at line 469 of file content.cpp.
◆ contentTransferEncoding() [1/2]
|
nodiscard |
Returns the Content-Transfer-Encoding header.
Can be nullptr
if the header doesn't exist.
- Since
- 24.08
◆ contentTransferEncoding() [2/2]
Headers::ContentTransferEncoding * KMime::Content::contentTransferEncoding | ( | bool | create = true | ) |
Returns the Content-Transfer-Encoding header.
- Parameters
-
create If true, create the header if it doesn't exist yet.
◆ contentType() [1/2]
|
nodiscard |
Returns the Content-Type header.
Can be nullptr
if the header doesn't exist.
- Since
- 24.08
◆ contentType() [2/2]
Headers::ContentType * KMime::Content::contentType | ( | bool | create = true | ) |
Returns the Content-Type header.
- Parameters
-
create If true, create the header if it doesn't exist yet.
◆ decodedContent()
|
nodiscard |
Returns the decoded Content body.
Note that this will be empty for multipart contents or for encapsulated messages, after parse() has been called.
Definition at line 321 of file content.cpp.
◆ decodedText()
|
nodiscard |
Returns the decoded text.
Additional to decodedContent(), this also applies charset decoding. If this is not a text Content, decodedText() returns an empty QString.
- Parameters
-
trimText If true, then the decoded text will have all trailing whitespace removed. removeTrailingNewlines If true, then the decoded text will have all consecutive trailing newlines removed.
The last trailing new line of the decoded text is always removed.
Definition at line 372 of file content.cpp.
◆ encodedBody()
|
nodiscard |
Like encodedContent(), with the difference that only the body will be returned, i.e.
the headers are excluded.
- Since
- 4.6
Definition at line 260 of file content.cpp.
◆ encodedContent()
|
nodiscard |
Returns a QByteArray containing the encoded Content, including the Content header and all sub-Contents.
If you make changes to the broken-down representation of the message, be sure to first call assemble() before calling encodedContent(), otherwise the result will not be up-to-date.
If this content is an encapsulated message, i.e. bodyIsMessage() returns true, then encodedContent() will use the message returned by bodyAsMessage() as the body of the result, calling encodedContent() on the message.
Definition at line 237 of file content.cpp.
◆ epilogue()
|
nodiscard |
Returns the MIME preamble.
- Returns
- a QByteArray containing the MIME epilogue.
- Since
- 4.9
Definition at line 106 of file content.cpp.
◆ fromUnicodeString()
void KMime::Content::fromUnicodeString | ( | const QString & | s | ) |
Sets the Content body to the given string using charset of the content type.
If the charset can not be found, the system charset is taken and the content type header is changed to that charset. The charset of the content type header should be set to a charset that can encode the given string before calling this method.
This method does not set the content transfer encoding automatically, it needs to be set to a suitable value that can encode the given string before calling this method.
This method only makes sense for single-part contents, do not try to pass a multipart body or an encapsulated message here, that wouldn't work.
- Parameters
-
s Unicode-encoded string.
Definition at line 411 of file content.cpp.
◆ hasContent()
|
nodiscard |
Returns true if this Content object is not empty.
Definition at line 55 of file content.cpp.
◆ hasHeader()
|
nodiscard |
- Returns
- true if this Content has a header of type
type
.
- Parameters
-
type The type of the header to look for.
Definition at line 618 of file content.cpp.
◆ head()
|
nodiscard |
◆ header() [1/2]
|
nodiscard |
◆ header() [2/2]
T * KMime::Content::header | ( | bool | create = false | ) |
Returns the first header of type T, if it exists.
If the header does not exist and create
is true, creates an empty header and returns it. Otherwise returns 0. Note that the returned header may be empty.
- Parameters
-
create Whether to create the header if it does not exist.
- Since
- 4.4.
KDE5: BIC: FIXME: Why is the default argument false here? That is inconsistent with the methods in KMime::Message!
◆ headerByType()
Headers::Base * KMime::Content::headerByType | ( | const char * | type | ) | const |
Returns the first header of type type
, if it exists.
Otherwise returns 0. Note that the returned header may be empty.
- Parameters
-
type the header type to find
- Since
- 4.2
Definition at line 563 of file content.cpp.
◆ headers()
|
nodiscard |
◆ headersByType()
|
nodiscard |
Returns all type
headers in the Content.
Take care that this result is not cached, so could be slow.
- Parameters
-
type the header type to find
- Since
- 4.2
Definition at line 576 of file content.cpp.
◆ index()
|
nodiscard |
Returns the index of this Content based on the topLevel() object.
- Since
- 4.3
Definition at line 785 of file content.cpp.
◆ indexForContent()
|
nodiscard |
Returns the ContentIndex for the given Content, or an invalid index if the Content is not found within the hierarchy.
- Parameters
-
content the Content object to search.
Definition at line 714 of file content.cpp.
◆ isFrozen()
|
nodiscard |
Returns whether this Content is frozen.
A frozen content is immutable, i.e. calling assemble() will never modify its head or body, and encodedContent() will return the same data before and after parsing.
- Since
- 4.4.
- See also
- setFrozen().
Definition at line 180 of file content.cpp.
◆ isTopLevel()
|
nodiscard |
Returns true if this is the top-level node in the MIME tree.
The top-level node is always a Message or NewsArticle. However, a node can be a Message without being a top-level node when it is an encapsulated message.
Definition at line 734 of file content.cpp.
◆ parent() [1/2]
|
nodiscard |
Returns the parent content object, or 0 if the content doesn't have a parent.
- Since
- 4.3
Definition at line 757 of file content.cpp.
◆ parent() [2/2]
|
nodiscard |
Definition at line 762 of file content.cpp.
◆ parse()
void KMime::Content::parse | ( | ) |
Parses the Content.
This means the broken-down object representation of the Content is updated from the string representation of the Content.
Call this if you want to access or change headers, sub-Contents or the encapsulated message.
- Note
- Calling parse() twice will not work for multipart contents or for contents of which the body is an encapsulated message. The reason is that the first parse() will delete the body, so there is no body to work on for the second call of parse().
- Calling this will reset the message returned by bodyAsMessage(), as the message is re-parsed as well. Also, all old sub-contents will be deleted, so any old Content pointer will become invalid.
Definition at line 116 of file content.cpp.
◆ preamble()
|
nodiscard |
Returns the MIME preamble.
- Returns
- a QByteArray containing the MIME preamble.
- Since
- 4.9
Definition at line 96 of file content.cpp.
◆ prependContent()
void KMime::Content::prependContent | ( | Content * | content | ) |
Prepends a new sub-Content.
If the sub-Content is already part of another Content object, it is removed from there and its parent is updated.
- Parameters
-
content The new sub-Content.
- See also
- appendContent()
- takeContent()
- Since
- 6.0
Definition at line 502 of file content.cpp.
◆ removeHeader() [1/2]
bool KMime::Content::removeHeader | ( | ) |
◆ removeHeader() [2/2]
bool KMime::Content::removeHeader | ( | const char * | type | ) |
Searches for the first header of type type
, and deletes it, removing it from this Content.
- Parameters
-
type The type of the header to look for.
- Returns
- true if a header was found and removed.
Definition at line 603 of file content.cpp.
◆ replaceContent()
Definition at line 471 of file content.cpp.
◆ setBody()
void KMime::Content::setBody | ( | const QByteArray & | body | ) |
Sets the Content decoded body raw data.
This method operates on the string representation of the Content. Call parse() if you want to access individual sub-Contents or the encapsulated message.
- Parameters
-
body is a QByteArray containing the body data.
- Note
body
is assumed to be decoded as far as the content transfer encoding is concerned.
- See also
- setEncodedBody()
Definition at line 84 of file content.cpp.
◆ setContent()
void KMime::Content::setContent | ( | const QByteArray & | s | ) |
Sets the Content to the given raw data, containing the Content head and body separated by two linefeeds.
This method operates on the string representation of the Content. Call parse() if you want to access individual headers, sub-Contents or the encapsulated message.
- Note
- The passed data must not contain any CRLF sequences, only LF. Use CRLFtoLF for conversion before passing in the data.
- Parameters
-
s is a QByteArray containing the raw Content data.
Definition at line 60 of file content.cpp.
◆ setEncodedBody()
void KMime::Content::setEncodedBody | ( | const QByteArray & | body | ) |
Sets the Content body raw data encoded according to the content transfer encoding.
This method operates on the string representation of the Content. Call parse() if you want to access individual sub-Contents or the encapsulated message.
- Parameters
-
body is a QByteArray containing the body data.
- Note
body
is assumed to be encoded as far as the content transfer encoding is concerned.
- Since
- 24.08
- See also
- setBody()
Definition at line 90 of file content.cpp.
◆ setEpilogue()
void KMime::Content::setEpilogue | ( | const QByteArray & | epilogue | ) |
Sets the MIME preamble.
- Parameters
-
epilogue a QByteArray containing what will be used as the MIME epilogue.
- Since
- 4.9
Definition at line 111 of file content.cpp.
◆ setFrozen()
void KMime::Content::setFrozen | ( | bool | frozen = true | ) |
Freezes this Content if frozen
is true; otherwise unfreezes it.
- Parameters
-
frozen freeze content if true
, otherwise unfreeze
- Since
- 4.4
- See also
- isFrozen().
Definition at line 185 of file content.cpp.
◆ setHead()
void KMime::Content::setHead | ( | const QByteArray & | head | ) |
Sets the Content header raw data.
This method operates on the string representation of the Content. Call parse() if you want to access individual headers.
- Parameters
-
head is a QByteArray containing the header data.
- See also
- head().
Definition at line 71 of file content.cpp.
◆ setHeader()
void KMime::Content::setHeader | ( | Headers::Base * | h | ) |
Sets the specified header to this Content.
Any previous header of the same type is removed. If you need multiple headers of the same type, use appendHeader() or prependHeader().
- Parameters
-
h The header to set.
- See also
- appendHeader()
- removeHeader()
- Since
- 4.4
Definition at line 590 of file content.cpp.
◆ setParent()
void KMime::Content::setParent | ( | Content * | parent | ) |
Sets a new parent to the Content and add to its contents list.
If it already had a parent, it is removed from the old parents contents list.
- Parameters
-
parent the new parent
- Since
- 4.3
Definition at line 739 of file content.cpp.
◆ setPreamble()
void KMime::Content::setPreamble | ( | const QByteArray & | preamble | ) |
Sets the MIME preamble.
- Parameters
-
preamble a QByteArray containing what will be used as the MIME preamble.
- Since
- 4.9
Definition at line 101 of file content.cpp.
◆ size()
|
nodiscard |
Returns the size of the Content body after encoding.
(If the encoding is quoted-printable, this is only an approximate size.) This will return 0 for multipart contents or for encapsulated messages.
Definition at line 623 of file content.cpp.
◆ storageSize()
|
nodiscard |
Returns the size of this Content and all sub-Contents.
Definition at line 639 of file content.cpp.
◆ takeContent()
Removes the given sub-Content and, if that actually was a sub-content returns that.
- Parameters
-
content The Content to remove. It is not deleted, ownership goes back to the caller.
- Since
- 6.0
Definition at line 516 of file content.cpp.
◆ textContent() [1/2]
|
nodiscard |
Returns the first Content with mimetype text/.
Definition at line 425 of file content.cpp.
◆ textContent() [2/2]
|
nodiscard |
Returns the first Content with MIME type text/.
Const overload of the above, the returned Content cannot be modified.
- Since
- 24.08
Definition at line 431 of file content.cpp.
◆ topLevel() [1/2]
|
nodiscard |
Returns the toplevel content object, 0 if there is no such object.
- Since
- 4.3
Definition at line 767 of file content.cpp.
◆ topLevel() [2/2]
|
nodiscard |
Definition at line 776 of file content.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Jul 26 2024 11:51:33 by doxygen 1.11.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.