MessageList::Core::Aggregation
#include <aggregation.h>
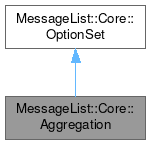
Public Types | |
enum | FillViewStrategy { FavorInteractivity , FavorSpeed , BatchNoInteractivity } |
enum | GroupExpandPolicy { NeverExpandGroups , ExpandRecentGroups , AlwaysExpandGroups } |
enum | Grouping { NoGrouping , GroupByDate , GroupByDateRange , GroupBySenderOrReceiver , GroupBySender , GroupByReceiver } |
enum | ThreadExpandPolicy { NeverExpandThreads , ExpandThreadsWithNewMessages , ExpandThreadsWithUnreadMessages , AlwaysExpandThreads , ExpandThreadsWithUnreadOrImportantMessages } |
enum | Threading { NoThreading , PerfectOnly , PerfectAndReferences , PerfectReferencesAndSubject } |
enum | ThreadLeader { TopmostMessage , MostRecentMessage } |
Static Public Member Functions | |
static bool | compareName (Aggregation *agg1, Aggregation *agg2) |
static QList< QPair< QString, int > > | enumerateFillViewStrategyOptions () |
static QList< QPair< QString, int > > | enumerateGroupExpandPolicyOptions (Grouping g) |
static QList< QPair< QString, int > > | enumerateGroupingOptions () |
static QList< QPair< QString, int > > | enumerateThreadExpandPolicyOptions (Threading t) |
static QList< QPair< QString, int > > | enumerateThreadingOptions () |
static QList< QPair< QString, int > > | enumerateThreadLeaderOptions (Grouping g, Threading t) |
Additional Inherited Members | |
![]() | |
QString | mDescription |
QString | mId |
QString | mName |
bool | mReadOnly = false |
Detailed Description
A set of aggregation options that can be applied to the MessageList::Model in a single shot.
The set defines the behaviours related to the population of the model, threading of messages and grouping.
Definition at line 28 of file aggregation.h.
Member Enumeration Documentation
◆ FillViewStrategy
The available fill view strategies.
If you add values here please look at the implementations of the enumerate* functions and add appropriate descriptors.
Definition at line 104 of file aggregation.h.
◆ GroupExpandPolicy
The available group expand policies.
If you add values here please look at the implementations of the enumerate* functions and add appropriate descriptors.
Definition at line 53 of file aggregation.h.
◆ Grouping
Message grouping.
If you add values here please look at the implementations of the enumerate* functions and add appropriate descriptors.
Definition at line 36 of file aggregation.h.
◆ ThreadExpandPolicy
The available thread expand policies.
Meaningless when threading is set to NoThreading. If you add values here please look at the implementations of the enumerate* functions and add appropriate descriptors.
Definition at line 90 of file aggregation.h.
◆ Threading
The available threading methods.
If you add values here please look at the implementations of the enumerate* functions and add appropriate descriptors.
Definition at line 65 of file aggregation.h.
◆ ThreadLeader
The available thread leading options.
Meaningless when threading is set to NoThreading. If you add values here please look at the implementations of the enumerate* functions and add appropriate descriptors.
Definition at line 78 of file aggregation.h.
Constructor & Destructor Documentation
◆ Aggregation() [1/2]
|
explicit |
Definition at line 47 of file aggregation.cpp.
◆ Aggregation() [2/2]
|
explicit |
Definition at line 19 of file aggregation.cpp.
Member Function Documentation
◆ compareName()
|
inlinestaticnodiscard |
Definition at line 131 of file aggregation.h.
◆ enumerateFillViewStrategyOptions()
Enumerates the fill view strategies.
The returned descriptors are pairs in that the first item is the localized description of the option value and the second item is the integer option value itself.
Definition at line 234 of file aggregation.cpp.
◆ enumerateGroupExpandPolicyOptions()
Enumerates the group sort direction options compatible with the specified Grouping.
The returned descriptors are pairs in that the first item is the localized description of the option value and the second item is the integer option value itself. If the returned list is empty then the value of the option is meaningless in the current context.
Definition at line 186 of file aggregation.cpp.
◆ enumerateGroupingOptions()
Enumerates the available grouping options as a QList of pairs in that the first item is the localized description of the option value and the second item is the integer option value itself.
Definition at line 176 of file aggregation.cpp.
◆ enumerateThreadExpandPolicyOptions()
|
static |
Enumerates the thread expand policies compatible with the specified Threading option.
The returned descriptors are pairs in that the first item is the localized description of the option value and the second item is the integer option value itself. If the returned list is empty then the value of the option is meaningless in the current context.
Definition at line 222 of file aggregation.cpp.
◆ enumerateThreadingOptions()
Enumerates the available threading method options.
The returned descriptors are pairs in that the first item is the localized description of the option value and the second item is the integer option value itself.
Definition at line 200 of file aggregation.cpp.
◆ enumerateThreadLeaderOptions()
|
static |
Enumerates the thread leader determination methods compatible with the specified Threading and the specified Grouping options.
The returned descriptors are pairs in that the first item is the localized description of the option value and the second item is the integer option value itself. If the returned list is empty then the value of the option is meaningless in the current context.
Definition at line 208 of file aggregation.cpp.
◆ fillViewStrategy()
|
inlinenodiscard |
Returns the current fill view strategy.
Definition at line 261 of file aggregation.h.
◆ groupExpandPolicy()
|
inlinenodiscard |
Returns the current GroupExpandPolicy.
Definition at line 160 of file aggregation.h.
◆ grouping()
|
nodiscard |
Returns the currently set Grouping option.
Definition at line 38 of file aggregation.cpp.
◆ load()
|
overridevirtual |
Pure virtual reimplemented from OptionSet.
Implements MessageList::Core::OptionSet.
Definition at line 58 of file aggregation.cpp.
◆ save()
|
overridevirtual |
Pure virtual reimplemented from OptionSet.
Implements MessageList::Core::OptionSet.
Definition at line 161 of file aggregation.cpp.
◆ setFillViewStrategy()
|
inline |
Sets the current fill view strategy.
Definition at line 269 of file aggregation.h.
◆ setGroupExpandPolicy()
|
inline |
Sets the GroupExpandPolicy for the groups.
Note that this option has no meaning if grouping is set to NoGrouping.
Definition at line 169 of file aggregation.h.
◆ setGrouping()
|
inline |
Sets the Grouping option.
Definition at line 145 of file aggregation.h.
◆ setThreadExpandPolicy()
|
inline |
Sets the current thread expand policy.
Please note that when Threading is set to NoThreading this value is meaningless and by policy should be set to NeverExpandThreads.
Definition at line 245 of file aggregation.h.
◆ setThreading()
|
inline |
Sets the threading method option.
Definition at line 193 of file aggregation.h.
◆ setThreadLeader()
|
inline |
Sets the current thread leader determination method.
Please note that when Threading is set to NoThreading this value is meaningless and by policy should be set to TopmostMessage.
Definition at line 218 of file aggregation.h.
◆ threadExpandPolicy()
|
inline |
Returns the current thread expand policy.
Definition at line 235 of file aggregation.h.
◆ threading()
|
inlinenodiscard |
Returns the current threading method.
Definition at line 185 of file aggregation.h.
◆ threadLeader()
|
inlinenodiscard |
Returns the current thread leader determination method.
Definition at line 208 of file aggregation.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Feb 21 2025 11:47:10 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.