MessageList::Core::ModelInvariantRowMapper
#include <modelinvariantrowmapper.h>
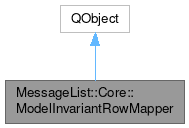
Public Member Functions | |
void | createModelInvariantIndex (int modelIndexRow, ModelInvariantIndex *invariantToFill) |
QList< ModelInvariantIndex * > * | modelIndexRowRangeToModelInvariantIndexList (int startIndexRow, int count) |
ModelInvariantIndex * | modelIndexRowToModelInvariantIndex (int modelIndexRow) |
int | modelInvariantIndexToModelIndexRow (ModelInvariantIndex *invariant) |
void | modelReset () |
void | modelRowsInserted (int modelIndexRowPosition, int count) |
QList< ModelInvariantIndex * > * | modelRowsRemoved (int modelIndexRowPosition, int count) |
void | setLazyUpdateChunkInterval (int chunkInterval) |
void | setLazyUpdateIdleInterval (int idleInterval) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
This class is an optimizing helper for dealing with large flat QAbstractItemModel objects.
The problem:
When you're an user of a flat QAbstractItemModel you access its contents by the means of QModelIndex. The model index is basically a row index (for flat models). You usually fetch some data for a row and then store the row index in order to fetch more data later (think of a tree view that shows the "name" for an item but when clicked needs to open a window with the details associated to the item).
The problem is that your row indexes may become invalid when rows are added or removed from the model. For instance, if a row is added at position 10, then any cached index after position 10 must be updated in order to point to the same content. With very large models this can become a problem since the update must be somewhat "atomic" in order to preserve consistency. Atomic, then, means "unbreakable": you can't chunk it in smaller pieces. This also means that your application will simply freeze if you have a model with 100000 cached indexes and a row is added/removed at the beginning. Yet worse: your app will freeze EVERY time a row is added. This means that if you don't really optimize, or just add non contiguous rows, you must do the update multiple times...
This class tries to solve this problem with a little overhead. It basically gives you a ModelInvariantIndex for each "new" row you query. Later the model may change by addition/removal of rows but with a ModelInvariantIndex you will still be able to retrieve the content that the index was pointing to at the time it was created.
You don't need to implement any row update in your rowsInserted() / rowsRemoved() handlers. Just call the modelRowsInserted() modelRowsRemoved() functions: they will do everything for you in a substantially constant time.
As the model structure changes the lookups will get a bit slower since the row mapper must apply the changes sequentially to each invariant. To avoid this, and to avoid storing the whole history of changes the ModelInvariantRowMapper will perform a background update of your ModelInvariantIndex objects. You don't need to care about this: it will happen automagically.
The ModelInvariantIndex allocation and destruction is in fact left to you. This is a GOOD approach because you're very likely to have some sort of structures associated to the items you display. You may then simply derive your objects from ModelInvariantIndex. This will save an additional memory allocation for each one of your items (we are optimizing, aren't we ?) and you will be able to dynamic_cast<> the ModelInvariantIndex pointers directly to your structure pointers (which will likely save a large QHash<>...). Even in the unlikely case in that you don't have a data structure for your items, it's still an operator new() call that YOU make instead of this implementation. It doesn't impact performance at all. You just have to remember to delete your ModelInvariantIndex objects when you no longer need them.
Definition at line 75 of file modelinvariantrowmapper.h.
Constructor & Destructor Documentation
◆ ModelInvariantRowMapper()
|
explicit |
Definition at line 50 of file modelinvariantrowmapper.cpp.
◆ ~ModelInvariantRowMapper()
|
override |
Definition at line 66 of file modelinvariantrowmapper.cpp.
Member Function Documentation
◆ createModelInvariantIndex()
void ModelInvariantRowMapper::createModelInvariantIndex | ( | int | modelIndexRow, |
ModelInvariantIndex * | invariantToFill ) |
Binds a ModelInvariantIndex structure to the specified CURRENT modelIndexRow.
Later you can use the ModelInvariantIndex to retrieve the model contents that the parameter modelIndexRow refers to... even if the model changes. Call this function only if you're sure that there is no such invariant yet, otherwise take a look at modelIndexRowToModelInvariantIndex().
This function ASSUMES that invariantToFill is a newly allocated ModelInvariantIndex.
Definition at line 326 of file modelinvariantrowmapper.cpp.
◆ modelIndexRowRangeToModelInvariantIndexList()
QList< ModelInvariantIndex * > * ModelInvariantRowMapper::modelIndexRowRangeToModelInvariantIndexList | ( | int | startIndexRow, |
int | count ) |
This basically applies modelIndexRowToModelInvariantIndex() to a range of elements.
The returned pointer can be null if no existing ModelInvariantIndex object were present in the range (this can happen if you don't request some of them). If the returned value is not 0 then you're responsible of deleting it.
Definition at line 345 of file modelinvariantrowmapper.cpp.
◆ modelIndexRowToModelInvariantIndex()
ModelInvariantIndex * ModelInvariantRowMapper::modelIndexRowToModelInvariantIndex | ( | int | modelIndexRow | ) |
Finds the existing ModelInvariantIndex that belongs to the specified CURRENT modelIndexRow.
Returns the ModelInvariantIndex found or 0 if such an invariant wasn't yet created (by the means of createModelInvariantIndex()).
Definition at line 340 of file modelinvariantrowmapper.cpp.
◆ modelInvariantIndexToModelIndexRow()
int ModelInvariantRowMapper::modelInvariantIndexToModelIndexRow | ( | ModelInvariantIndex * | invariant | ) |
Maps a ModelInvariantIndex to the CURRENT associated row index in the model.
As long as the underlying model is consistent, the returned row index is guaranteed to point to the content that this ModelInvariantIndex pointed at the time it was created.
Returns the current associated row index in the model or -1 if the invariant does not belong to this mapper (model) or is marked as invalid at all.
Definition at line 234 of file modelinvariantrowmapper.cpp.
◆ modelReset()
void ModelInvariantRowMapper::modelReset | ( | ) |
Call this function from your handlers of reset() and layoutChanged() AFTER you ve last accessed the model underlying data.
You probably want this function to be the first call of your reset() or layoutChanged() handlers.
This function assumes that all the ModelInvariantIndex are being invalidated and need to be required.
Definition at line 547 of file modelinvariantrowmapper.cpp.
◆ modelRowsInserted()
void ModelInvariantRowMapper::modelRowsInserted | ( | int | modelIndexRowPosition, |
int | count ) |
Call this function when rows are inserted to the underlying model BEFORE scanning the model for the new items.
You probably want this function to be the first call in your rowsInserted() handler or the last call in the rowsAboutToBeInserted() handler.
Definition at line 374 of file modelinvariantrowmapper.cpp.
◆ modelRowsRemoved()
QList< ModelInvariantIndex * > * ModelInvariantRowMapper::modelRowsRemoved | ( | int | modelIndexRowPosition, |
int | count ) |
Call this function when rows are removed from the underlying model AFTER accessing the removed rows for the last time.
You probably want this function to be the first call of your rowsRemoved() handler or the last call in the rowsAboutToBeRemoved() handler.
This function will invalidate any ModelInvariantIndex instances that are affected by the change. It will also do you a favor by returning the list of the invalidated ModelInvariantIndex objects since you'll probably want to delete them. The returned pointer can be null if no existing ModelInvariantIndex object were affected by the change (this can happen if you don't request some of them). If the returned value is not 0 then you're responsible of deleting it.
Definition at line 437 of file modelinvariantrowmapper.cpp.
◆ setLazyUpdateChunkInterval()
void ModelInvariantRowMapper::setLazyUpdateChunkInterval | ( | int | chunkInterval | ) |
Sets the maximum time we can spend inside a single lazy update step.
The larger this time, the more resources we consume and leave less to UI processing but also larger the update throughput (that is, we update more items per second). This is 50 msec by default.
Definition at line 224 of file modelinvariantrowmapper.cpp.
◆ setLazyUpdateIdleInterval()
void ModelInvariantRowMapper::setLazyUpdateIdleInterval | ( | int | idleInterval | ) |
Sets the idle time between two lazy updates in milliseconds.
The larger this time, the less resources we consume and leave more to UI processing but also smaller the update throughput (that is, we update less items per second). This is 50 msec by default.
Definition at line 229 of file modelinvariantrowmapper.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:51:55 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.