KDbUtils::AutodeletedHash
#include <KDbUtils.h>
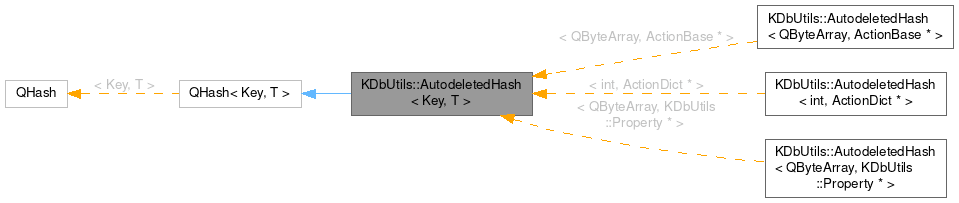
Public Member Functions | |
AutodeletedHash (bool autoDelete=true) | |
AutodeletedHash (const AutodeletedHash &other) | |
bool | autoDelete () const |
void | clear () |
QHash< Key, T >::iterator | erase (typename QHash< Key, T >::iterator pos) |
QHash< Key, T >::iterator | insert (const Key &key, const T &value) |
int | remove (const Key &key) |
void | setAutoDelete (bool set) |
![]() | |
QHash (const QHash< Key, T > &other) | |
QHash (InputIterator begin, InputIterator end) | |
QHash (QHash< Key, T > &&other) | |
QHash (std::initializer_list< std::pair< Key, T > > list) | |
auto | asKeyValueRange () & |
auto | asKeyValueRange () && |
auto | asKeyValueRange () const &&const |
auto | asKeyValueRange () const &const |
iterator | begin () |
const_iterator | begin () const const |
qsizetype | capacity () const const |
const_iterator | cbegin () const const |
const_iterator | cend () const const |
void | clear () |
const_iterator | constBegin () const const |
const_iterator | constEnd () const const |
const_iterator | constFind (const Key &key) const const |
const_key_value_iterator | constKeyValueBegin () const const |
const_key_value_iterator | constKeyValueEnd () const const |
bool | contains (const Key &key) const const |
qsizetype | count () const const |
qsizetype | count (const Key &key) const const |
iterator | emplace (const Key &key, Args &&... args) |
iterator | emplace (Key &&key, Args &&... args) |
bool | empty () const const |
iterator | end () |
const_iterator | end () const const |
iterator | erase (const_iterator pos) |
qsizetype | erase_if (QHash< Key, T > &hash, Predicate pred) |
iterator | find (const Key &key) |
const_iterator | find (const Key &key) const const |
iterator | insert (const Key &key, const T &value) |
void | insert (const QHash< Key, T > &other) |
bool | isEmpty () const const |
Key | key (const T &value) const const |
Key | key (const T &value, const Key &defaultKey) const const |
key_iterator | keyBegin () const const |
key_iterator | keyEnd () const const |
QList< Key > | keys () const const |
QList< Key > | keys (const T &value) const const |
key_value_iterator | keyValueBegin () |
const_key_value_iterator | keyValueBegin () const const |
key_value_iterator | keyValueEnd () |
const_key_value_iterator | keyValueEnd () const const |
float | load_factor () const const |
bool | operator!= (const QHash< Key, T > &other) const const |
QDataStream & | operator<< (QDataStream &out, const QHash< Key, T > &hash) |
QHash< Key, T > & | operator= (const QHash< Key, T > &other) |
QHash< Key, T > & | operator= (QHash< Key, T > &&other) |
bool | operator== (const QHash< Key, T > &other) const const |
QDataStream & | operator>> (QDataStream &in, QHash< Key, T > &hash) |
T & | operator[] (const Key &key) |
const T | operator[] (const Key &key) const const |
int | qGlobalQHashSeed () |
size_t | qHash (char key, size_t seed) |
size_t | qHash (char16_t key, size_t seed) |
size_t | qHash (char32_t key, size_t seed) |
size_t | qHash (char8_t key, size_t seed) |
size_t | qHash (const QBitArray &key, size_t seed) |
size_t | qHash (const QByteArray &key, size_t seed) |
size_t | qHash (const QChar key, size_t seed) |
size_t | qHash (const QDateTime &key, size_t seed) |
size_t | qHash (const QHash< Key, T > &key, size_t seed) |
size_t | qHash (const QSet< T > &key, size_t seed) |
size_t | qHash (const QString &key, size_t seed) |
size_t | qHash (const QTypeRevision &key, size_t seed) |
size_t | qHash (const QUrl &url, size_t seed) |
size_t | qHash (const QVersionNumber &key, size_t seed) |
size_t | qHash (const std::pair< T1, T2 > &key, size_t seed) |
size_t | qHash (const T *key, size_t seed) |
size_t | qHash (double key, size_t seed) |
size_t | qHash (float key, size_t seed) |
size_t | qHash (int key, size_t seed) |
size_t | qHash (long double key, size_t seed) |
size_t | qHash (long key, size_t seed) |
size_t | qHash (QDate key, size_t seed) |
size_t | qHash (qint64 key, size_t seed) |
size_t | qHash (QLatin1StringView key, size_t seed) |
size_t | qHash (QPoint key, size_t seed) |
size_t | qHash (QTime key, size_t seed) |
size_t | qHash (quint64 key, size_t seed) |
size_t | qHash (short key, size_t seed) |
size_t | qHash (signed char key, size_t seed) |
size_t | qHash (std::nullptr_t key, size_t seed) |
size_t | qHash (uchar key, size_t seed) |
size_t | qHash (uint key, size_t seed) |
size_t | qHash (ulong key, size_t seed) |
size_t | qHash (ushort key, size_t seed) |
size_t | qHash (wchar_t key, size_t seed) |
size_t | qHashBits (const void *p, size_t len, size_t seed) |
size_t | qHashMulti (size_t seed, const T &... args) |
size_t | qHashMultiCommutative (size_t seed, const T &... args) |
size_t | qHashRange (InputIterator first, InputIterator last, size_t seed) |
size_t | qHashRangeCommutative (InputIterator first, InputIterator last, size_t seed) |
void | qSetGlobalQHashSeed (int newSeed) |
bool | remove (const Key &key) |
qsizetype | removeIf (Predicate pred) |
void | reserve (qsizetype size) |
qsizetype | size () const const |
void | squeeze () |
void | swap (QHash< Key, T > &other) |
T | take (const Key &key) |
T | value (const Key &key) const const |
T | value (const Key &key, const T &defaultValue) const const |
QList< T > | values () const const |
Additional Inherited Members | |
![]() | |
typedef | const_key_value_iterator |
typedef | ConstIterator |
typedef | difference_type |
typedef | Iterator |
typedef | key_type |
typedef | key_value_iterator |
typedef | mapped_type |
typedef | size_type |
Detailed Description
class KDbUtils::AutodeletedHash< Key, T >
Autodeleting hash.
Definition at line 178 of file KDbUtils.h.
Constructor & Destructor Documentation
◆ AutodeletedHash() [1/2]
|
inline |
Creates autodeleting hash as a copy of other.
Auto-deletion is not enabled as it would cause double deletion for items. If you enable auto-deletion on here, make sure you disable it in the other hash.
Definition at line 184 of file KDbUtils.h.
◆ AutodeletedHash() [2/2]
|
inline |
Creates empty autodeleting hash.
Auto-deletion is enabled by default.
Definition at line 188 of file KDbUtils.h.
◆ ~AutodeletedHash()
|
inline |
Definition at line 190 of file KDbUtils.h.
Member Function Documentation
◆ autoDelete()
|
inline |
Definition at line 198 of file KDbUtils.h.
◆ clear()
|
inline |
Definition at line 201 of file KDbUtils.h.
◆ erase()
|
inline |
Definition at line 207 of file KDbUtils.h.
◆ insert()
|
inline |
Definition at line 215 of file KDbUtils.h.
◆ remove()
|
inline |
Definition at line 224 of file KDbUtils.h.
◆ setAutoDelete()
|
inline |
Definition at line 195 of file KDbUtils.h.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:05:18 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.