KPropertySet
#include <KPropertySet.h>
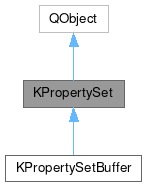
Signals | |
void | aboutToBeCleared () |
void | aboutToBeDeleted () |
void | aboutToDeleteProperty (KPropertySet &set, KProperty &property) |
void | propertyChanged (KPropertySet &set, KProperty &property) |
void | propertyChangedInternal (KPropertySet &set, KProperty &property) |
void | propertyReset (KPropertySet &set, KProperty &property) |
void | readOnlyFlagChanged () |
Public Slots | |
void | addProperty (KProperty *property, const QByteArray &group="common") |
void | changeProperty (const QByteArray &property, const QVariant &value) |
void | changePropertyIfExists (const QByteArray &property, const QVariant &value) |
void | clear () |
void | removeProperty (const QByteArray &name) |
void | removeProperty (KProperty *property) |
void | setGroupCaption (const QByteArray &group, const QString &caption) |
void | setGroupIconName (const QByteArray &group, const QString &iconName) |
void | setPreviousSelection (const QByteArray &prevSelection) |
void | setReadOnly (bool readOnly) |
Public Member Functions | |
KPropertySet (const KPropertySet &set) | |
KPropertySet (QObject *parent=nullptr) | |
void | clearModifiedFlags () |
bool | contains (const QByteArray &name) const |
int | count () const |
int | count (const KPropertySelector &selector) const |
void | debug () const |
QString | groupCaption (const QByteArray &group) const |
QString | groupIconName (const QByteArray &group) const |
QByteArray | groupNameForProperty (const KProperty &property) const |
QByteArray | groupNameForProperty (const QByteArray &propertyName) const |
QList< QByteArray > | groupNames () const |
bool | hasProperties (const KPropertySelector &selector) const |
bool | hasVisibleProperties () const |
bool | isEmpty () const |
bool | isModified () const |
bool | isReadOnly () const |
KPropertySet & | operator= (const KPropertySet &set) |
KProperty & | operator[] (const QByteArray &name) const |
QByteArray | previousSelection () const |
KProperty & | property (const QByteArray &name) const |
QList< QByteArray > | propertyNamesForGroup (const QByteArray &group) const |
QVariant | propertyValue (const QByteArray &name, const QVariant &defaultValue=QVariant()) const |
QMap< QByteArray, QVariant > | propertyValues () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
KPropertySet (bool propertyOwner) | |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
Set of properties.
Definition at line 119 of file KPropertySet.h.
Constructor & Destructor Documentation
◆ KPropertySet() [1/3]
|
explicit |
Constructs a new property set object.
Definition at line 417 of file KPropertySet.cpp.
◆ KPropertySet() [2/3]
|
explicit |
Constructs a deep copy of set. The new object will not have a QObject parent even if set has such parent.
Definition at line 424 of file KPropertySet.cpp.
◆ ~KPropertySet()
|
override |
Definition at line 438 of file KPropertySet.cpp.
◆ KPropertySet() [3/3]
|
explicitprotected |
Constructs a set which owns or does not own it's properties.
Definition at line 432 of file KPropertySet.cpp.
Member Function Documentation
◆ aboutToBeCleared
|
signal |
Emitted when property set object is about to be cleared (using clear()). This signal is also emitted from destructor before emitting aboutToBeDeleted().
◆ aboutToBeDeleted
|
signal |
Emitted when property set object is about to be deleted.
◆ aboutToDeleteProperty
|
signal |
Emitted when property is about to be deleted.
◆ addProperty
|
slot |
Adds the property to the set, in the group. The property becomes owned by the set. Any name can be used for the group. "common" is the default for a basic top-level group.
Definition at line 449 of file KPropertySet.cpp.
◆ changeProperty
|
slot |
Change the value of property whose key is property to value.
Definition at line 608 of file KPropertySet.cpp.
◆ changePropertyIfExists
|
slot |
Change the value of property whose key is property to value only if it exists in the set.
- See also
- void changeProperty(const QByteArray &, const QVariant &)
Definition at line 576 of file KPropertySet.cpp.
◆ clear
|
slot |
Removes all property objects from the property set and deletes them.
Definition at line 468 of file KPropertySet.cpp.
◆ clearModifiedFlags()
void KPropertySet::clearModifiedFlags | ( | ) |
Clears "modified" flag of all properties in this set, i.e.
calls clearModifiedFlag() for each property.
- Since
- 3.1
- See also
- KProperty::clearModifiedFlag()
Definition at line 664 of file KPropertySet.cpp.
◆ contains()
bool KPropertySet::contains | ( | const QByteArray & | name | ) | const |
- Returns
- true if the set contains property names name.
Definition at line 565 of file KPropertySet.cpp.
◆ count() [1/2]
int KPropertySet::count | ( | ) | const |
- Returns
- the number of top-level properties in the set.
Definition at line 519 of file KPropertySet.cpp.
◆ count() [2/2]
int KPropertySet::count | ( | const KPropertySelector & | selector | ) | const |
- Returns
- the number of top-level properties in the set matching criteria defined by selector.
Definition at line 524 of file KPropertySet.cpp.
◆ debug()
void KPropertySet::debug | ( | ) | const |
Prints debug output for this set.
Definition at line 615 of file KPropertySet.cpp.
◆ groupCaption()
QString KPropertySet::groupCaption | ( | const QByteArray & | group | ) | const |
- Returns
- the user-visible translated caption string for group that will be shown in property editor to represent group. If there is no special caption set for the group, group is just returned.
Definition at line 502 of file KPropertySet.cpp.
◆ groupIconName()
QString KPropertySet::groupIconName | ( | const QByteArray & | group | ) | const |
- Returns
- icon name for group.
Definition at line 512 of file KPropertySet.cpp.
◆ groupNameForProperty() [1/2]
QByteArray KPropertySet::groupNameForProperty | ( | const KProperty & | property | ) | const |
Definition at line 481 of file KPropertySet.cpp.
◆ groupNameForProperty() [2/2]
QByteArray KPropertySet::groupNameForProperty | ( | const QByteArray & | propertyName | ) | const |
- Returns
- group name for property propertyName Empty value is returned if there is no such property.
- Since
- 3.1
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Since
- 3.1
Definition at line 475 of file KPropertySet.cpp.
◆ groupNames()
QList< QByteArray > KPropertySet::groupNames | ( | ) | const |
- Returns
- a list of all group names. The order of items is undefined.
Definition at line 486 of file KPropertySet.cpp.
◆ hasProperties()
bool KPropertySet::hasProperties | ( | const KPropertySelector & | selector | ) | const |
- Returns
- true if the set is contains properties matching criteria defined by selector.
Definition at line 543 of file KPropertySet.cpp.
◆ hasVisibleProperties()
bool KPropertySet::hasVisibleProperties | ( | ) | const |
- Returns
- true if the set is contains visible properties.
Definition at line 538 of file KPropertySet.cpp.
◆ isEmpty()
bool KPropertySet::isEmpty | ( | ) | const |
- Returns
- true if the set is empty, i.e. count() is 0; otherwise returns false.
Definition at line 533 of file KPropertySet.cpp.
◆ isModified()
bool KPropertySet::isModified | ( | ) | const |
Returns true
if at least one property in this set is modified, i.e.
returns true
for isModified()
- Since
- 3.1
Definition at line 671 of file KPropertySet.cpp.
◆ isReadOnly()
bool KPropertySet::isReadOnly | ( | ) | const |
- Returns
- true if the set is read-only when used in a property editor.
false
by default. In a read-only property set no property can be modified by the user regardless of read-only flag of any property (KProperty::isReadOnly()). On the other hand if KProperty::isReadOnly() istrue
and KPropertySet::isReadOnly() isfalse
, the property is still read-only. Read-only property set prevents editing in the property editor but it is still possible to change value or other parameters of property programatically using KProperty::setValue(), KProperty::resetValue(), etc.
Definition at line 550 of file KPropertySet.cpp.
◆ operator=()
KPropertySet & KPropertySet::operator= | ( | const KPropertySet & | set | ) |
Creates a deep copy of set and assigns it to this property set.
Definition at line 590 of file KPropertySet.cpp.
◆ operator[]()
KProperty & KPropertySet::operator[] | ( | const QByteArray & | name | ) | const |
Accesses a property by name. A property reference is returned, so all property modifications are allowed. If there is no such property, null property (KProperty()) is returned, so it's good practice to use contains() if it's not known if the property exists. For example to set a value of a property, use:
- Returns
- Property with given name.
- See also
- changeProperty(const QByteArray &, const QVariant &)
- changePropertyIfExists(const QByteArray &, const QVariant &)
Definition at line 584 of file KPropertySet.cpp.
◆ previousSelection()
QByteArray KPropertySet::previousSelection | ( | ) | const |
Used by property editor to preserve previous selection when this set is assigned again.
Definition at line 645 of file KPropertySet.cpp.
◆ property()
KProperty & KPropertySet::property | ( | const QByteArray & | name | ) | const |
- Returns
- property named with name. If no such property is found, null property (KProperty()) is returned.
Definition at line 571 of file KPropertySet.cpp.
◆ propertyChanged
|
signal |
Emitted when the value of the property is changed.
◆ propertyChangedInternal
|
signal |
Exists to be sure that we emitted it before propertyChanged(), so editor object can handle this.
◆ propertyNamesForGroup()
QList< QByteArray > KPropertySet::propertyNamesForGroup | ( | const QByteArray & | group | ) | const |
- Returns
- a list of all property names for group group. The order of items is undefined.
Definition at line 491 of file KPropertySet.cpp.
◆ propertyReset
|
signal |
Emitted when the value of the property is reset.
◆ propertyValue()
QVariant KPropertySet::propertyValue | ( | const QByteArray & | name, |
const QVariant & | defaultValue = QVariant() ) const |
- Returns
- value for property named with name. If no such property is found, default value defaultValue is returned.
Definition at line 601 of file KPropertySet.cpp.
◆ propertyValues()
QMap< QByteArray, QVariant > KPropertySet::propertyValues | ( | ) | const |
- Returns
- property values for this set
Definition at line 655 of file KPropertySet.cpp.
◆ readOnlyFlagChanged
|
signal |
Emitted when property set object's read-only flag has changed.
◆ removeProperty [1/2]
|
slot |
Removes property with the given name from the set and deletes the object. Emits aboutToDeleteProperty() before removing.
Definition at line 461 of file KPropertySet.cpp.
◆ removeProperty [2/2]
|
slot |
Removes property from the set and deletes the object. Emits aboutToDeleteProperty before removing.
Definition at line 455 of file KPropertySet.cpp.
◆ setGroupCaption
|
slot |
Sets caption as a user-visible translated string that will be shown in editor to represent group.
Definition at line 497 of file KPropertySet.cpp.
◆ setGroupIconName
|
slot |
Sets the icon name icon to be displayed for group.
Definition at line 507 of file KPropertySet.cpp.
◆ setPreviousSelection
|
slot |
◆ setReadOnly
|
slot |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:56:16 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.