SliderSpinBox
#include <SliderSpinBox.h>
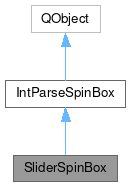
Signals | |
void | draggingFinished () |
![]() | |
void | errorWhileParsing (const QString &expr) const |
void | noMoreParsingError () const |
Public Slots | |
int | fastSliderStep () const |
bool | isDragging () const |
void | setBlockUpdateSignalOnDrag (bool newBlockUpdateSignalOnDrag) |
void | setExponentRatio (qreal newExponentRatio) |
void | setFastSliderStep (int newFastSliderStep) |
void | setMaximum (int newMaximum, bool computeNewFastSliderStep=true) |
void | setMinimum (int newMinimum, bool computeNewFastSliderStep=true) |
void | setRange (int newMinimum, int newMaximum, bool computeNewFastSliderStep=true) |
void | setSoftMaximum (int newSoftMaximum) |
void | setSoftMinimum (int newSoftMinimum) |
void | setSoftRange (int newSoftMinimum, int newSoftMaximum) |
void | setValue (int newValue) |
int | softMaximum () const |
int | softMinimum () const |
QWidget * | widget () const |
![]() | |
bool | isLastValid () const |
void | setValue (int value, bool overwriteExpression=false) |
void | stepBy (int steps) |
virtual QString | veryCleanText () const |
QSpinBox * | widget () const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
This class is a wrapper around KisSliderSpinBox, a spinbox in which you can click and drag to set the value, with a slider like bar displayed inside.
The widget itself is accessed with the widget() function.
The value can be set by click and dragging with the mouse or pen or by typing in with the keyboard. To enter the edit mode, in which the keyboard can be used, one has to right-click inside the spinbox or click and hold the pointer inside or press the enter key. To leave the edit mode, one can press the enter key again, in which case the value is committed, or press the escape key, in which case the value is rejected.
When dragging with the pointer, one can fine tune the value by dragging far away vertically from the spinbox. The farther the pointer is, the slower the value will change. If the pointer is inside the spinbox plus a certain margin, the value will not be scaled. By pressing the shift key the slow down will be even more pronounced and by pressing the control key the value will snap to the increment set by setFastSliderStep. The two keys can be used at the same time.
A "soft range" can be set to make the slider display only a sub-range of the spinbox range. This way one can have a large range but display and set with the pointer and with more precision only the most commonly used sub-set of values. A value outside the "soft range" can be set by entering the edit mode and using the keyboard. The "soft range" is considered valid if the "soft maximum" is greater than the "soft minimum".
Definition at line 50 of file SliderSpinBox.h.
Constructor & Destructor Documentation
◆ SliderSpinBox()
|
explicit |
Definition at line 17 of file SliderSpinBox.cpp.
◆ ~SliderSpinBox()
|
override |
Definition at line 27 of file SliderSpinBox.cpp.
Member Function Documentation
◆ fastSliderStep
|
slot |
Get the value to which multiples the spinbox value snaps when the control key is pressed.
- Returns
- the value to which multiples the spinbox value snaps when the control key is pressed
- See also
- setFastSliderStep(int)
Definition at line 36 of file SliderSpinBox.cpp.
◆ isDragging
|
slot |
Get if the user is currently dragging the slider with the pointer.
- Returns
- true if the user is currently dragging the slider with the pointer, false otherwise
Definition at line 51 of file SliderSpinBox.cpp.
◆ setBlockUpdateSignalOnDrag
|
slot |
Set if the spinbox should not Q_EMIT signals when dragging the slider.
This is useful to prevent multiple updates when changing the value if the update operation is costly. A valueChanged signal will be emitted when the pointer is released from the slider.
- Parameters
-
newBlockUpdateSignalOnDrag true if the spinbox should not emit signals when dragging the slider. false otherwise
Definition at line 85 of file SliderSpinBox.cpp.
◆ setExponentRatio
|
slot |
Set the exponent used by a power function to modify the values as a function of the horizontal position.
This allows having more values concentrated in one side of the slider than the other
- Parameters
-
newExponentRatio the new exponent to be used by the power function
Definition at line 76 of file SliderSpinBox.cpp.
◆ setFastSliderStep
|
slot |
Set the value to which multiples the spinbox value snaps when the control key is pressed.
- Parameters
-
newFastSliderStep value to which multiples the spinbox value snaps when the control key is pressed
- See also
- fastSliderStep() const
Definition at line 90 of file SliderSpinBox.cpp.
◆ setMaximum
|
slot |
Set the maximum value of the range.
The soft range will be adapted to fit inside the range
- Parameters
-
newMaximum the new maximum value computeNewFastSliderStep true if a new "fast slider step" must be computed based on the range
- See also
- setRange(int,int)
- setMinimum(int)
Definition at line 71 of file SliderSpinBox.cpp.
◆ setMinimum
|
slot |
Set the minimum value of the range.
The soft range will be adapted to fit inside the range
- Parameters
-
newMinimum the new minimum value computeNewFastSliderStep true if a new "fast slider step" must be computed based on the range
- See also
- setRange(int,int)
- setMaximum(int)
Definition at line 66 of file SliderSpinBox.cpp.
◆ setRange
|
slot |
Set the minimum and the maximum values of the range, computing a new "fast slider step" based on the range if required.
The soft range will be adapted to fit inside the range
- Parameters
-
newMinimum the new minimum value newMaximum the new maximum value computeNewFastSliderStep true if a new "fast slider step" must be computed based on the range
- See also
- setMinimum(int)
- setMaximum(int)
Definition at line 61 of file SliderSpinBox.cpp.
◆ setSoftMaximum
|
slot |
Set the maximum value of the soft range.
- Parameters
-
newSoftMaximum the new maximum value
Definition at line 105 of file SliderSpinBox.cpp.
◆ setSoftMinimum
|
slot |
Set the minimum value of the soft range.
- Parameters
-
newSoftMinimum the new minimum value
Definition at line 100 of file SliderSpinBox.cpp.
◆ setSoftRange
|
slot |
Set the minimum and the maximum values of the soft range.
- Parameters
-
newSoftMinimum the new minimum value newSoftMaximum the new maximum value
Definition at line 95 of file SliderSpinBox.cpp.
◆ setValue
|
slot |
◆ softMaximum
|
slot |
Get the maximum value of the "soft range".
- Returns
- the maximum value of the "soft range"
- See also
- setSoftMaximum(int)
- setSoftRange(int, int)
- softMinimum) const
Definition at line 46 of file SliderSpinBox.cpp.
◆ softMinimum
|
slot |
Get the minimum value of the "soft range".
- Returns
- the minimum value of the "soft range"
Definition at line 41 of file SliderSpinBox.cpp.
◆ widget
|
slot |
Get the internal KisSliderSpinBox as a QWidget, so it may be added to a UI.
- Returns
- the internal KisSliderSpinBox as a QWidget
Definition at line 32 of file SliderSpinBox.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Mar 28 2025 11:58:32 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.