kviewshell
GPixmap.cpp File Reference
#include "GPixmap.h"
#include "GString.h"
#include "GException.h"
#include "ByteStream.h"
#include "GRect.h"
#include "GBitmap.h"
#include "GThreads.h"
#include "Arrays.h"
#include "JPEGDecoder.h"
#include <stdlib.h>
#include <math.h>
#include <assert.h>
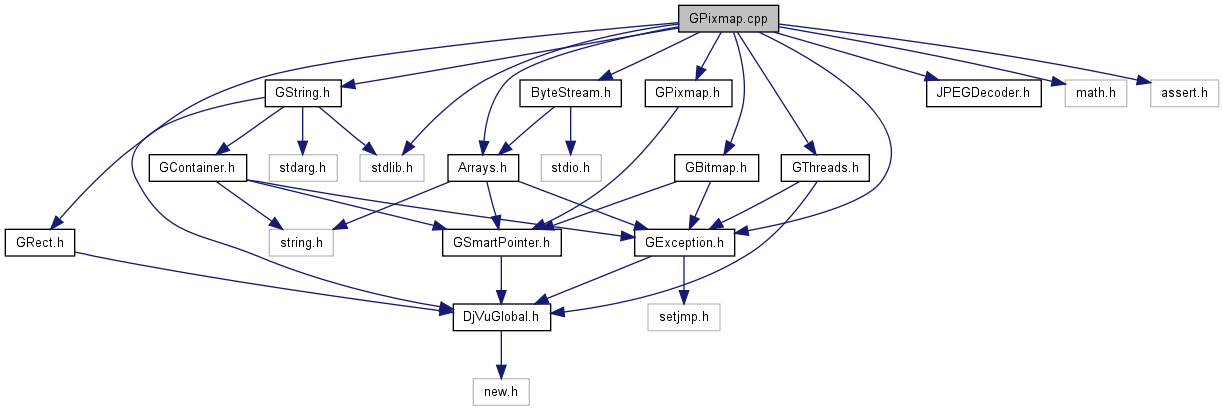
Go to the source code of this file.
Functions | |
static void | color_correction_table (double gamma, unsigned char gtable[256]) |
static void | color_correction_table_cache (double gamma, unsigned char gtable[256]) |
static void | compute_clip () |
static void | copy_from_partial (int w, int h, const GPixel *s, int sadd, int xmin, int xmax, int ymin, int ymax, GPixel *d, int dadd) |
static void | copy_line (const GPixel *s, int smin, int smax, GPixel *d, int dmin, int dmax) |
static void | copy_to_partial (int w, int h, const GPixel *s, int sadd, GPixel *d, int dadd, int xmin, int xmax, int ymin, int ymax) |
static void | downsample_4x4_to_3x3 (const GPixel *s, int sadd, GPixel *d, int dadd) |
static void | euclidian_ratio (int a, int b, int &q, int &r) |
static int | maxi (int x, int y) |
static int | mini (int x, int y) |
static const GPixel * | new_gray_ramp (int grays, GPixel *ramp) |
static GMonitor & | pixmap_monitor () |
static unsigned int | read_integer (char &c, ByteStream &bs) |
static void | upsample_2x2_to_3x3 (const GPixel *s, int sadd, GPixel *d, int dadd) |
Variables | |
static unsigned char | clip [512] |
static bool | clipok = false |
Function Documentation
static void color_correction_table | ( | double | gamma, | |
unsigned char | gtable[256] | |||
) | [static] |
Definition at line 596 of file GPixmap.cpp.
static void color_correction_table_cache | ( | double | gamma, | |
unsigned char | gtable[256] | |||
) | [static] |
Definition at line 628 of file GPixmap.cpp.
static void compute_clip | ( | ) | [static] |
Definition at line 1286 of file GPixmap.cpp.
static void copy_from_partial | ( | int | w, | |
int | h, | |||
const GPixel * | s, | |||
int | sadd, | |||
int | xmin, | |||
int | xmax, | |||
int | ymin, | |||
int | ymax, | |||
GPixel * | d, | |||
int | dadd | |||
) | [inline, static] |
Definition at line 1065 of file GPixmap.cpp.
static void copy_line | ( | const GPixel * | s, | |
int | smin, | |||
int | smax, | |||
GPixel * | d, | |||
int | dmin, | |||
int | dmax | |||
) | [inline, static] |
Definition at line 1042 of file GPixmap.cpp.
static void copy_to_partial | ( | int | w, | |
int | h, | |||
const GPixel * | s, | |||
int | sadd, | |||
GPixel * | d, | |||
int | dadd, | |||
int | xmin, | |||
int | xmax, | |||
int | ymin, | |||
int | ymax | |||
) | [inline, static] |
Definition at line 1015 of file GPixmap.cpp.
static void downsample_4x4_to_3x3 | ( | const GPixel * | s, | |
int | sadd, | |||
GPixel * | d, | |||
int | dadd | |||
) | [inline, static] |
Definition at line 947 of file GPixmap.cpp.
static void euclidian_ratio | ( | int | a, | |
int | b, | |||
int & | q, | |||
int & | r | |||
) | [inline, static] |
Definition at line 142 of file GPixmap.cpp.
static int maxi | ( | int | x, | |
int | y | |||
) | [inline, static] |
Definition at line 135 of file GPixmap.cpp.
static int mini | ( | int | x, | |
int | y | |||
) | [inline, static] |
Definition at line 128 of file GPixmap.cpp.
Definition at line 111 of file GPixmap.cpp.
static GMonitor& pixmap_monitor | ( | ) | [static] |
Definition at line 158 of file GPixmap.cpp.
static unsigned int read_integer | ( | char & | c, | |
ByteStream & | bs | |||
) | [static] |
Definition at line 437 of file GPixmap.cpp.
static void upsample_2x2_to_3x3 | ( | const GPixel * | s, | |
int | sadd, | |||
GPixel * | d, | |||
int | dadd | |||
) | [inline, static] |
Definition at line 986 of file GPixmap.cpp.
Variable Documentation
unsigned char clip[512] [static] |
Definition at line 1282 of file GPixmap.cpp.
bool clipok = false [static] |
Definition at line 1283 of file GPixmap.cpp.