kviewshell
ByteStream Class Reference
Abstract class for a stream of bytes. More...
#include <ByteStream.h>
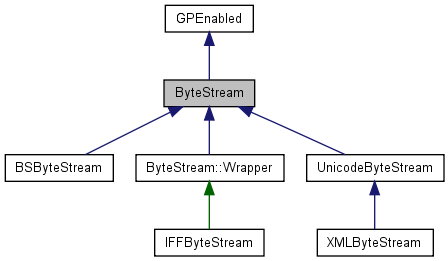
Classes | |
class | Wrapper |
ByteStream::Wrapper implements wrapping bytestream. More... | |
Public Types | |
enum | codepage_type { RAW, AUTO, NATIVE, UTF8 } |
Public Member Functions | |
GNativeString | getAsNative (void) |
GUTF8String | getAsUTF8 (void) |
Utility Functions. | |
Class ByteStream# implements these functions using the virtual interface functions only.
All subclasses of ByteStream# inherit these functions. | |
size_t | copy (ByteStream &bsfrom, size_t size=0) |
GP< ByteStream > | duplicate (const size_t size=0) const |
size_t | format (const char *fmt,...) |
void | formatmessage (const char *fmt,...) |
TArray< char > | get_data (void) |
virtual bool | is_static (void) const |
unsigned int | read16 () |
unsigned int | read24 () |
unsigned int | read32 () |
unsigned int | read8 () |
size_t | readall (void *buffer, size_t size) |
virtual size_t | readat (void *buffer, size_t sz, int pos) |
int | scanf (const char *fmt,...) |
virtual int | size (void) const |
size_t | writall (const void *buffer, size_t size) |
void | write16 (unsigned int card16) |
void | write24 (unsigned int card24) |
void | write32 (unsigned int card32) |
void | write8 (unsigned int card8) |
void | writemessage (const char *message) |
size_t | writestring (const GNativeString &s) |
size_t | writestring (const GUTF8String &s) |
Virtual Functions. | |
virtual void | flush (void) |
virtual size_t | read (void *buffer, size_t size) |
virtual int | seek (long offset, int whence=SEEK_SET, bool nothrow=false) |
virtual long | tell (void) const =0 |
virtual size_t | write (const void *buffer, size_t size) |
virtual | ~ByteStream () |
Static Public Member Functions | |
static GP< ByteStream > | create (FILE *const f, char const *const mode, const bool closeme) |
static GP< ByteStream > | create (const int fd, char const *const mode, const bool closeme) |
static GP< ByteStream > | create (char const *const mode) |
static GP< ByteStream > | create (const GURL &url, char const *const mode) |
static GP< ByteStream > | create (void const *const buffer, const size_t size) |
static GP< ByteStream > | create (void) |
static GP< ByteStream > | create_static (void const *const buffer, const size_t size) |
static GP< ByteStream > | get_stderr (char const *const mode=0) |
static GP< ByteStream > | get_stdin (char const *const mode=0) |
static GP< ByteStream > | get_stdout (char const *const mode=0) |
Public Attributes | |
enum ByteStream::codepage_type | cp |
Static Public Attributes | |
static const char * | EndOfFile = ERR_MSG("EOF") |
Protected Member Functions | |
ByteStream (void) |
Detailed Description
Abstract class for a stream of bytes.Class ByteStream# represent an object from which (resp. to which) bytes can be read (resp. written) as with a regular file. Virtual functions read# and write# must implement these two basic operations. In addition, function tell# returns an offset identifying the current position, and function seek# may be used to change the current position.
{ Note}. Both the copy constructor and the copy operator are declared as private members. It is therefore not possible to make multiple copies of instances of this class, as implied by the class semantic.
Definition at line 129 of file ByteStream.h.
Member Enumeration Documentation
Constructor & Destructor Documentation
ByteStream::~ByteStream | ( | ) | [virtual] |
ByteStream::ByteStream | ( | void | ) | [inline, protected] |
Definition at line 288 of file ByteStream.h.
Member Function Documentation
size_t ByteStream::copy | ( | ByteStream & | bsfrom, | |
size_t | size = 0 | |||
) |
Copy data from another ByteStream.
A maximum of size# bytes are read from the ByteStream bsfrom# and are written to the ByteStream #*this# at the current position. Less than size# bytes may be written if an end-of-file mark is reached on bsfrom#. This function returns the total number of bytes copied. Setting argument size# to zero (the default value) has a special meaning: the copying process will continue until reaching the end-of-file mark on ByteStream bsfrom#, regardless of the number of bytes transferred.
Reimplemented in IFFByteStream.
Definition at line 512 of file ByteStream.cpp.
GP< ByteStream > ByteStream::create | ( | FILE *const | f, | |
char const *const | mode, | |||
const bool | closeme | |||
) | [static] |
Constructs a ByteStream for accessing the stdio file f#.
Argument mode# indicates the type of the stdio file, as in the well known stdio function fopen#. Destroying the ByteStream object will not close the stdio file f# unless closeme is true.
Definition at line 1167 of file ByteStream.cpp.
GP< ByteStream > ByteStream::create | ( | const int | fd, | |
char const *const | mode, | |||
const bool | closeme | |||
) | [static] |
Constructs a ByteStream for accessing the stdio file f#.
Argument mode# indicates the type of the stdio file, as in the well known stdio function fopen#. Destroying the ByteStream object will not close the stdio file f# unless closeme is true.
Definition at line 1102 of file ByteStream.cpp.
GP< ByteStream > ByteStream::create | ( | char const *const | mode | ) | [static] |
GP< ByteStream > ByteStream::create | ( | const GURL & | url, | |
char const *const | mode | |||
) | [static] |
Constructs a ByteStream for accessing the file named url#.
Arguments url# and mode# are similar to the arguments of the well known stdio function fopen#. In addition a url of #-# will be interpreted as the standard output or the standard input according to mode#. This constructor will open a stdio file and construct a ByteStream object accessing this file. Destroying the ByteStream object will flush and close the associated stdio file. Exception {GException} is thrown with a plain text error message if the stdio file cannot be opened.
Definition at line 1038 of file ByteStream.cpp.
GP< ByteStream > ByteStream::create | ( | void const *const | buffer, | |
const size_t | size | |||
) | [static] |
Constructs a Memory ByteStream by copying initial data.
The Memory buffer is initialized with size# bytes copied from the memory area pointed to by buffer#.
Definition at line 1029 of file ByteStream.cpp.
GP< ByteStream > ByteStream::create | ( | void | ) | [static] |
Constructs an empty Memory ByteStream.
The buffer itself is organized as an array of 4096 byte blocks. The buffer is initially empty. You must first use function write# to store data into the buffer, use function seek# to rewind the current position, and function read# to read the data back.
Definition at line 1023 of file ByteStream.cpp.
GP< ByteStream > ByteStream::create_static | ( | void const *const | buffer, | |
const size_t | size | |||
) | [static] |
Creates a ByteStream object for allocating the memory area of length sz# starting at address buffer#.
This call impliments a read-only ByteStream interface for a memory area specified by the user at construction time. Calls to function read# directly access this memory area. The user must therefore make sure that its content remain valid long enough.
Definition at line 1199 of file ByteStream.cpp.
GP< ByteStream > ByteStream::duplicate | ( | const size_t | size = 0 |
) | const |
Create a new ByteStream# that copies the data from this ByteStream# starting from the current position, upto size# bytes.
Setting the size# to zero means copy to the end-of-file mark.
Definition at line 1205 of file ByteStream.cpp.
void ByteStream::flush | ( | void | ) | [virtual] |
Flushes all buffers in the ByteStream.
Calling this function guarantees that pending data have been actually written (i.e. passed to the operating system). Class ByteStream# provides a default implementation which does nothing.
Reimplemented in BSByteStream, ByteStream::Wrapper, IFFByteStream, and UnicodeByteStream.
Definition at line 374 of file ByteStream.cpp.
size_t ByteStream::format | ( | const char * | fmt, | |
... | ||||
) |
void ByteStream::formatmessage | ( | const char * | fmt, | |
... | ||||
) |
Formats the message string, looks up the external representation and writes it to the specified stream.
Looks up the message and writes it to the specified stream.
Definition at line 1313 of file ByteStream.cpp.
TArray< char > ByteStream::get_data | ( | void | ) |
Use at your own risk, only guarenteed to work for ByteStream::Memorys.
Definition at line 279 of file Arrays.cpp.
GP< ByteStream > ByteStream::get_stderr | ( | char const *const | mode = 0 |
) | [static] |
Definition at line 1305 of file ByteStream.cpp.
GP< ByteStream > ByteStream::get_stdin | ( | char const *const | mode = 0 |
) | [static] |
Easy access to preallocated stdin/stdout/stderr bytestreams.
Definition at line 1291 of file ByteStream.cpp.
GP< ByteStream > ByteStream::get_stdout | ( | char const *const | mode = 0 |
) | [static] |
Definition at line 1298 of file ByteStream.cpp.
GNativeString ByteStream::getAsNative | ( | void | ) |
Returns the contents of the file as a GNativeString.
Definition at line 1350 of file ByteStream.cpp.
GUTF8String ByteStream::getAsUTF8 | ( | void | ) |
virtual bool ByteStream::is_static | ( | void | ) | const [inline, virtual] |
Returns false, unless a subclass of ByteStream::Static.
Definition at line 285 of file ByteStream.h.
size_t ByteStream::read | ( | void * | buffer, | |
size_t | size | |||
) | [virtual] |
Reads data from a ByteStream.
This function {must} be implemented by each subclass of ByteStream#. At most size# bytes are read from the ByteStream and stored in the memory area pointed to by buffer#. Function read# returns immediately if size# is zero. The actual number of bytes read is returned. Function read# returns a number of bytes smaller than size# if the end-of-file mark is reached before filling the buffer. Subsequent invocations will always return value #0#. Function read# may also return a value greater than zero but smaller than size# for internal reasons. Programs must be ready to handle these cases or use function {readall}. Exception {GException} is thrown with a plain text error message whenever an error occurs.
Reimplemented in ByteStream::Wrapper, IFFByteStream, and UnicodeByteStream.
Definition at line 360 of file ByteStream.cpp.
unsigned int ByteStream::read16 | ( | ) |
Reads a two-bytes integer from a ByteStream.
The integer most significant byte is read first, regardless of the processor endianness.
Definition at line 589 of file ByteStream.cpp.
unsigned int ByteStream::read24 | ( | ) |
Reads a three-bytes integer from a ByteStream.
The integer most significant byte is read first, regardless of the processor endianness.
Definition at line 598 of file ByteStream.cpp.
unsigned int ByteStream::read32 | ( | ) |
Reads a four-bytes integer from a ByteStream.
The integer most significant bytes are read first, regardless of the processor endianness.
Definition at line 607 of file ByteStream.cpp.
unsigned int ByteStream::read8 | ( | ) |
size_t ByteStream::readall | ( | void * | buffer, | |
size_t | size | |||
) |
Reads data and blocks until everything has been read.
This function is essentially similar to function read#. Unlike function read# however, function readall# will never return a value smaller than size# unless an end-of-file mark is reached. This is implemented by repeatedly calling function read# until everything is read or until we reach an end-of-file mark. Note that read# and readall# are equivalent when size# is one.
Definition at line 431 of file ByteStream.cpp.
size_t ByteStream::readat | ( | void * | buffer, | |
size_t | sz, | |||
int | pos | |||
) | [inline, virtual] |
Reads data from a random position.
This function reads at most sz# bytes at position pos# into buffer# and returns the actual number of bytes read. The current position is unchanged.
Definition at line 354 of file ByteStream.h.
int ByteStream::scanf | ( | const char * | fmt, | |
... | ||||
) |
int ByteStream::seek | ( | long | offset, | |
int | whence = SEEK_SET , |
|||
bool | nothrow = false | |||
) | [virtual] |
Sets the current position for reading or writing the ByteStream.
Class ByteStream# provides a default implementation able to seek forward by calling function read# until reaching the desired position. Subclasses implementing better seek capabilities must override this default implementation. The new current position is computed by applying displacement offset# to the position represented by argument whence#. The following values are recognized for argument whence#: {description} [SEEK_SET#] Argument offset# indicates the position relative to the beginning of the ByteStream. [SEEK_CUR#] Argument offset# is a signed displacement relative to the current position. [SEEK_END#] Argument offset# is a displacement relative to the end of the file. It is then advisable to provide a negative value for offset#. {description} Results are undefined whenever the new position is greater than the total size of the ByteStream.
{ Error reporting}: If seek()# succeeds, #0# is returned. Otherwise it either returns #-1# (if nothrow# is set to FALSE#) or throws the {GException} exception.
Reimplemented in ByteStream::Wrapper, and UnicodeByteStream.
Definition at line 379 of file ByteStream.cpp.
int ByteStream::size | ( | void | ) | const [inline, virtual] |
Returns the total number of bytes contained in the buffer, file, etc.
Valid offsets for function seek# range from 0 to the value returned by this function.
Definition at line 365 of file ByteStream.h.
virtual long ByteStream::tell | ( | void | ) | const [pure virtual] |
Returns the offset of the current position in the ByteStream.
This function {must} be implemented by each subclass of ByteStream#.
Implemented in BSByteStream, ByteStream::Wrapper, IFFByteStream, and UnicodeByteStream.
size_t ByteStream::writall | ( | const void * | buffer, | |
size_t | size | |||
) |
Writes data and blocks until everything has been written.
This function is essentially similar to function write#. Unlike function write# however, function writall# will only return after all size# bytes have been written. This is implemented by repeatedly calling function write# until everything is written. Note that write# and writall# are equivalent when size# is one.
Definition at line 496 of file ByteStream.cpp.
size_t ByteStream::write | ( | const void * | buffer, | |
size_t | size | |||
) | [virtual] |
Writes data to a ByteStream.
This function {must} be implemented by each subclass of ByteStream#. At most size# bytes from buffer buffer# are written to the ByteStream. Function write# returns immediately if size# is zero. The actual number of bytes written is returned. Function write# may also return a value greater than zero but smaller than size# for internal reasons. Programs must be ready to handle these cases or use function {writall}. Exception {GException} is thrown with a plain text error message whenever an error occurs.
Reimplemented in ByteStream::Wrapper, IFFByteStream, and UnicodeByteStream.
Definition at line 367 of file ByteStream.cpp.
void ByteStream::write16 | ( | unsigned int | card16 | ) |
Writes a two-bytes integer to a ByteStream.
The integer most significant byte is written first, regardless of the processor endianness.
Definition at line 547 of file ByteStream.cpp.
void ByteStream::write24 | ( | unsigned int | card24 | ) |
Writes a three-bytes integer to a ByteStream.
The integer most significant byte is written first, regardless of the processor endianness.
Definition at line 557 of file ByteStream.cpp.
void ByteStream::write32 | ( | unsigned int | card32 | ) |
Writes a four-bytes integer to a ByteStream.
The integer most significant bytes are written first, regardless of the processor endianness.
Definition at line 568 of file ByteStream.cpp.
void ByteStream::write8 | ( | unsigned int | card8 | ) |
void ByteStream::writemessage | ( | const char * | message | ) |
Looks up the message and writes it to the specified stream.
Definition at line 1322 of file ByteStream.cpp.
size_t ByteStream::writestring | ( | const GNativeString & | s | ) |
size_t ByteStream::writestring | ( | const GUTF8String & | s | ) |
Member Data Documentation
const char * ByteStream::EndOfFile = ERR_MSG("EOF") [static] |
The documentation for this class was generated from the following files: