kviewshell
GP< TYPE > Class Template Reference
Reference counting pointer. More...
#include <GSmartPointer.h>
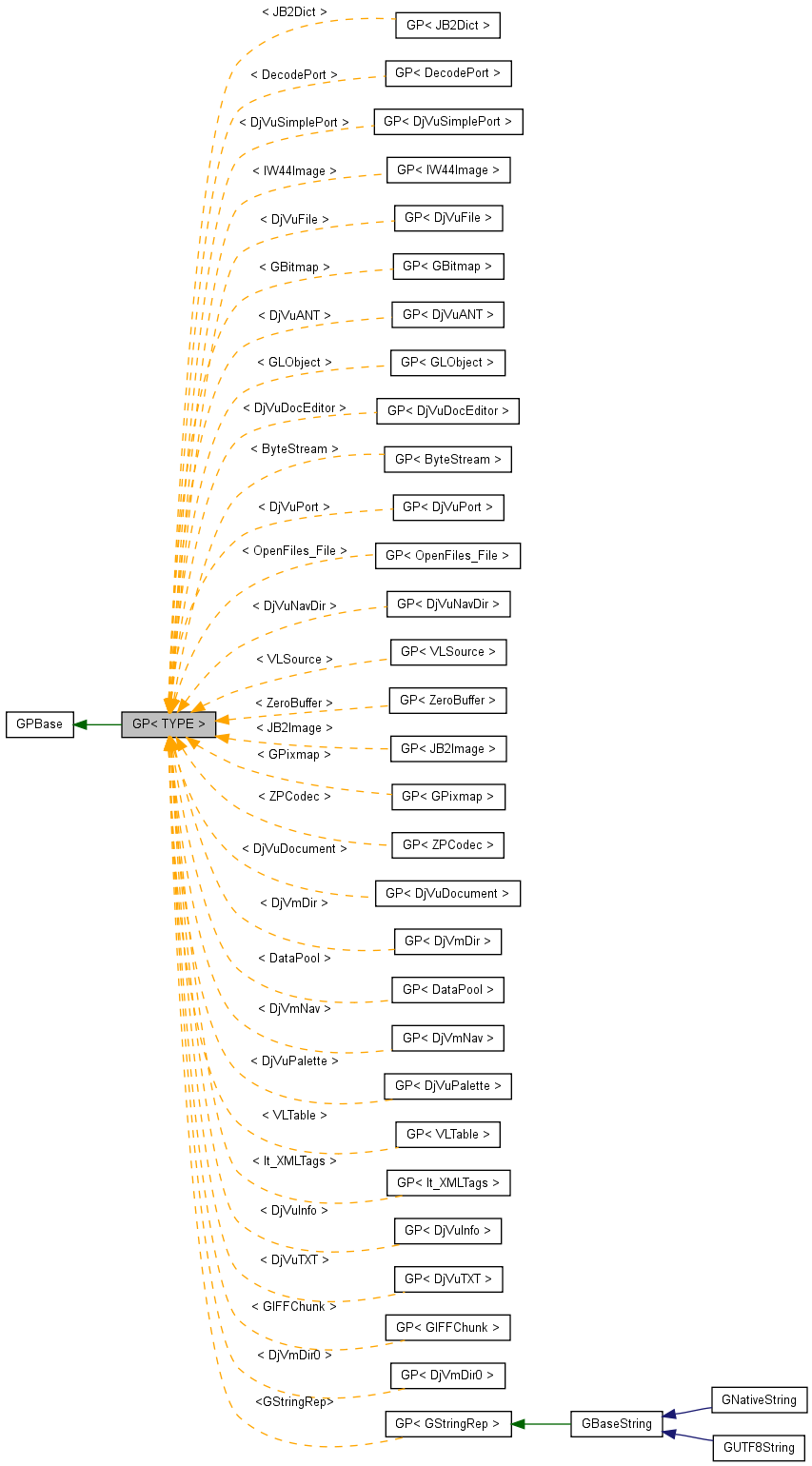
Public Member Functions | |
GP (TYPE *nptr) | |
GP (const GP< TYPE > &sptr) | |
GP () | |
operator TYPE * () const | |
int | operator! () const |
int | operator!= (TYPE *nptr) const |
TYPE & | operator* () const |
TYPE * | operator-> () const |
GP< TYPE > & | operator= (const GP< TYPE > &sptr) |
GP< TYPE > & | operator= (TYPE *nptr) |
int | operator== (TYPE *nptr) const |
Detailed Description
template<class TYPE>
class GP< TYPE >
Reference counting pointer.
Class GP<TYPE># represents a smart-pointer to an object of type TYPE#. Type TYPE# must be a subclass of GPEnabled#. This class overloads the usual pointer assignment and dereferencing operators. The overloaded operators maintain the reference counters and destroy the pointed object as soon as their reference counter reaches zero. Transparent type conversions are provided between smart-pointers and regular pointers.
Using a smart-pointer is a convenience and not an obligation. There is no need to use a smart-pointer to access a GPEnabled# object. As long as you never use a smart-pointer to access a GPEnabled# object, its reference counter remains zero. Since the reference counter is never decremented from one to zero, the object is never destroyed by the reference counting code. You can therefore choose to only use regular pointers to access objects allocated on the stack (automatic variables) or objects allocated dynamically. In the latter case you must explicitly destroy the dynamically allocated object with operator delete#.
The first time you use a smart-pointer to access GPEnabled# object, the reference counter is incremented to one. Object destruction will then happen automatically when the reference counter is decremented back to zero (i.e. when the last smart-pointer referencing this object stops doing so). This will happen regardless of how many regular pointers reference this object. In other words, if you start using smart-pointers with a GPEnabled# object, you engage automatic mode for this object. You should only do this with objects dynamically allocated with operator new#. You should never destroy the object yourself, but let the smart-pointers control the life of the object.
{ Performance considerations} --- Thread safe reference counting incurs a significant overhead. Smart-pointer are best used with sizeable objects for which the cost of maintaining the counters represent a small fraction of the processing time. It is always possible to cache a smart-pointer into a regular pointer. The cached pointer will remain valid until the smart-pointer object is destroyed or the smart-pointer value is changed.
{ Safety considerations} --- As explained above, a GPEnabled# object switches to automatic mode as soon as it becomes referenced by a smart-pointer. There is no way to switch the object back to manual mode. Suppose that you have decided to only use regular pointers with a particular GPEnabled# object. You therefore plan to destroy the object explicitly when you no longer need it. When you pass a regular pointer to this object as argument to a function, you really need to be certain that the function implementation will not assign this pointer to a smart-pointer. Doing so would indeed destroy the object as soon as the function returns. The bad news is that the fact that a function assigns a pointer argument to a smart-pointer does not necessarily appear in the function prototype. Such a behavior must be {documented} with the function public interface. As a convention, we usually write such functions with smart-pointer arguments instead of a regular pointer arguments. This is not enough to catch the error at compile time, but this is a simple way to document such a behavior. We still believe that this is a small problem in regard to the benefits of the smart-pointer. But one has to be aware of its existence.
Definition at line 270 of file GSmartPointer.h.
Constructor & Destructor Documentation
Constructs a copy of a smart-pointer.
- Parameters:
-
sptr smart-pointer to copy.
Definition at line 429 of file GSmartPointer.h.
Constructs a smart-pointer from a regular pointer.
The pointed object must be dynamically allocated (with operator new#). You should no longer explicitly destroy the object referenced by sptr# since the object life is now controlled by smart-pointers.
- Parameters:
-
nptr regular pointer to a {dynamically allocated object}.
Definition at line 423 of file GSmartPointer.h.
Member Function Documentation
GP< TYPE >::operator TYPE * | ( | ) | const [inline] |
Converts a smart-pointer into a regular pointer.
This is useful for caching the value of a smart-pointer for performances purposes. The cached pointer will remain valid until the smart-pointer is destroyed or until the smart-pointer value is changed.
Definition at line 435 of file GSmartPointer.h.
int GP< TYPE >::operator! | ( | ) | const [inline] |
Test operator.
Returns true if the smart-pointer is null. The automatic conversion from smart-pointers to regular pointers allows you to test whether a smart-pointer is non-null. You can use both following constructs: {verbatim} if (gp) { ... } while (! gp) { ... } {verbatim}
Reimplemented in GBaseString.
Definition at line 477 of file GSmartPointer.h.
int GP< TYPE >::operator!= | ( | TYPE * | nptr | ) | const [inline] |
Comparison operator.
Returns true if this smart-pointer and pointer nptr# point to different objects. The automatic conversion from smart-pointers to regular pointers allows you to compare two smart-pointers as well.
- Parameters:
-
nptr pointer to compare with.
Definition at line 471 of file GSmartPointer.h.
TYPE & GP< TYPE >::operator* | ( | ) | const [inline] |
Dereferencement operator.
This operator provides a convenient access to the smart-pointed object. Operator #*# works with smart-pointers exactly as with regular pointers.
Definition at line 447 of file GSmartPointer.h.
TYPE * GP< TYPE >::operator-> | ( | ) | const [inline] |
Indirection operator.
This operator provides a convenient access to the members of a smart-pointed object. Operator #-># works with smart-pointers exactly as with regular pointers.
Definition at line 441 of file GSmartPointer.h.
Assigns a smart-pointer to a smart-pointer lvalue.
- Parameters:
-
sptr smart-pointer copied into this smart-pointer.
Reimplemented in GUTF8String.
Definition at line 459 of file GSmartPointer.h.
Assigns a regular pointer to a smart-pointer lvalue.
The pointed object must be dynamically allocated (with operator new#). You should no longer explicitly destroy the object referenced by sptr# since the object life is now controlled by smart-pointers.
- Parameters:
-
nptr regular pointer to a {dynamically allocated object}.
Definition at line 453 of file GSmartPointer.h.
int GP< TYPE >::operator== | ( | TYPE * | nptr | ) | const [inline] |
Comparison operator.
Returns true if both this smart-pointer and pointer nptr# point to the same object. The automatic conversion from smart-pointers to regular pointers allows you to compare two smart-pointers as well.
- Parameters:
-
nptr pointer to compare with.
Definition at line 465 of file GSmartPointer.h.
The documentation for this class was generated from the following file: