kviewshell
GUTF8String Class Reference
General purpose character string. More...
#include <GString.h>
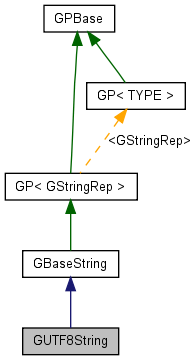
Public Types | |
typedef enum GStringRep::EncodeType | EncodeType |
Public Member Functions | |
GUTF8String | downcase (void) const |
GUTF8String & | format (const char *fmt,...) |
GUTF8String | fromEscaped (const GMap< GUTF8String, GUTF8String > ConvMap) const |
GUTF8String | fromEscaped (void) const |
GP< GStringRep::Unicode > | get_remainder (void) const |
char * | getbuf (int n=-1) |
GUTF8String (const double number) | |
GUTF8String (const int number) | |
GUTF8String (const GUTF8String &fmt, va_list &args) | |
GUTF8String (const GBaseString &gs, int from, int len) | |
GUTF8String (const GNativeString &str) | |
GUTF8String (const GUTF8String &str) | |
GUTF8String (const GBaseString &str) | |
GUTF8String (const GP< GStringRep > &str) | |
GUTF8String (const unsigned long *dat, unsigned int len) | |
GUTF8String (const unsigned short *dat, unsigned int len) | |
GUTF8String (const char *dat, unsigned int len) | |
GUTF8String (const unsigned long *dat) | |
GUTF8String (const unsigned short *dat) | |
GUTF8String (const unsigned char *str) | |
GUTF8String (const char *str) | |
GUTF8String (const char dat) | |
GUTF8String (void) | |
GUTF8String & | init (const GP< GStringRep > &rep) |
void | init (void) |
GUTF8String | operator+ (const char *s2) const |
GUTF8String | operator+ (const GNativeString &s2) const |
GUTF8String | operator+ (const GUTF8String &s2) const |
GUTF8String | operator+ (const GBaseString &s2) const |
GUTF8String & | operator+= (const GBaseString &str) |
GUTF8String & | operator+= (const char *str) |
GUTF8String & | operator+= (char ch) |
GUTF8String & | operator= (const GNativeString &str) |
GUTF8String & | operator= (const GUTF8String &str) |
GUTF8String & | operator= (const GBaseString &str) |
GUTF8String & | operator= (const GP< GStringRep > &str) |
GUTF8String & | operator= (const char *str) |
GUTF8String & | operator= (const char str) |
void | setat (const int n, const char ch) |
GUTF8String | substr (int from, int len) const |
GUTF8String | toEscaped (const bool tosevenbit=false) const |
GUTF8String | upcase (void) const |
GUTF8String & | vformat (const GUTF8String &fmt, va_list &args) |
~GUTF8String () | |
Static Public Member Functions | |
static GUTF8String | create (const unsigned long *buf, const unsigned int bufsize) |
static GUTF8String | create (const unsigned short *buf, const unsigned int bufsize) |
static GUTF8String | create (const char *buf, const unsigned int bufsize) |
static GUTF8String | create (void const *const buf, const unsigned int size, const GP< GStringRep::Unicode > &remainder) |
static GUTF8String | create (void const *const buf, const unsigned int size, const GUTF8String &encoding) |
static GUTF8String | create (void const *const buf, unsigned int size, const EncodeType encodetype) |
static GUTF8String | create (void const *const buf, const unsigned int size, const EncodeType encodetype, const GUTF8String &encoding) |
Friends | |
GUTF8String | operator+ (const char *s1, const GUTF8String &s2) |
Detailed Description
General purpose character string.Each instance of class GUTF8String# represents a character string. Overloaded operators provide a value semantic to GUTF8String# objects. Conversion operators and constructors transparently convert between GUTF8String# objects and const char*# pointers.
Functions taking strings as arguments should declare their arguments as "#const char*#". Such functions will work equally well with GUTF8String# objects since there is a fast conversion operator from GUTF8String# to "#const char*#". Functions returning strings should return GUTF8String# or GNativeString# objects because the class will automatically manage the necessary memory.
Characters in the string can be identified by their position. The first character of a string is numbered zero. Negative positions represent characters relative to the end of the string (i.e. position #-1# accesses the last character of the string, position #-2# represents the second last character, etc.)
Definition at line 736 of file GString.h.
Member Typedef Documentation
typedef enum GStringRep::EncodeType GUTF8String::EncodeType |
Constructor & Destructor Documentation
GUTF8String::~GUTF8String | ( | ) |
Definition at line 114 of file GString.cpp.
GUTF8String::GUTF8String | ( | void | ) | [inline] |
GUTF8String::GUTF8String | ( | const char | dat | ) |
GUTF8String::GUTF8String | ( | const char * | str | ) |
Constructs a string from a null terminated character array.
Definition at line 2680 of file GString.cpp.
GUTF8String::GUTF8String | ( | const unsigned char * | str | ) |
Constructs a string from a null terminated character array.
Definition at line 2683 of file GString.cpp.
GUTF8String::GUTF8String | ( | const unsigned short * | dat | ) |
Definition at line 2686 of file GString.cpp.
GUTF8String::GUTF8String | ( | const unsigned long * | dat | ) |
Definition at line 2689 of file GString.cpp.
GUTF8String::GUTF8String | ( | const char * | dat, | |
unsigned int | len | |||
) |
Constructs a string from a character array.
Elements of the character array dat# are added into the string until the string length reaches len# or until encountering a null character (whichever comes first).
Definition at line 2692 of file GString.cpp.
GUTF8String::GUTF8String | ( | const unsigned short * | dat, | |
unsigned int | len | |||
) |
Definition at line 2695 of file GString.cpp.
GUTF8String::GUTF8String | ( | const unsigned long * | dat, | |
unsigned int | len | |||
) |
Definition at line 2698 of file GString.cpp.
GUTF8String::GUTF8String | ( | const GP< GStringRep > & | str | ) | [inline] |
GUTF8String::GUTF8String | ( | const GBaseString & | str | ) | [inline] |
GUTF8String::GUTF8String | ( | const GUTF8String & | str | ) | [inline] |
GUTF8String::GUTF8String | ( | const GNativeString & | str | ) | [inline] |
GUTF8String::GUTF8String | ( | const GBaseString & | gs, | |
int | from, | |||
int | len | |||
) |
Constructs a string from a character array.
Elements of the character array dat# are added into the string until the string length reaches len# or until encountering a null character (whichever comes first).
Definition at line 2701 of file GString.cpp.
GUTF8String::GUTF8String | ( | const GUTF8String & | fmt, | |
va_list & | args | |||
) |
Constructs a string with a formatted string (as in vprintf#).
The string is re-initialized with the characters generated according to the specified format fmt# and using the optional arguments. See the ANSI-C function vprintf()# for more information. The current implementation will cause a segmentation violation if the resulting string is longer than 32768 characters.
Definition at line 2672 of file GString.cpp.
GUTF8String::GUTF8String | ( | const int | number | ) |
Constructs a string from a character.
Constructs a string with a human-readable representation of integer number#. The format is similar to format #"%d"# in function printf#.
Definition at line 2704 of file GString.cpp.
GUTF8String::GUTF8String | ( | const double | number | ) |
Constructs a string with a human-readable representation of floating point number number#.
The format is similar to format #"%f"# in function printf#.
Definition at line 2707 of file GString.cpp.
Member Function Documentation
GUTF8String GUTF8String::create | ( | const unsigned long * | buf, | |
const unsigned int | bufsize | |||
) | [inline, static] |
GUTF8String GUTF8String::create | ( | const unsigned short * | buf, | |
const unsigned int | bufsize | |||
) | [inline, static] |
GUTF8String GUTF8String::create | ( | const char * | buf, | |
const unsigned int | bufsize | |||
) | [inline, static] |
GUTF8String GUTF8String::create | ( | void const *const | buf, | |
const unsigned int | size, | |||
const GP< GStringRep::Unicode > & | remainder | |||
) | [static] |
Definition at line 767 of file GUnicode.cpp.
GUTF8String GUTF8String::create | ( | void const *const | buf, | |
const unsigned int | size, | |||
const GUTF8String & | encoding | |||
) | [static] |
Definition at line 776 of file GUnicode.cpp.
GUTF8String GUTF8String::create | ( | void const *const | buf, | |
unsigned int | size, | |||
const EncodeType | encodetype | |||
) | [static] |
Definition at line 758 of file GUnicode.cpp.
GUTF8String GUTF8String::create | ( | void const *const | buf, | |
const unsigned int | size, | |||
const EncodeType | encodetype, | |||
const GUTF8String & | encoding | |||
) | [static] |
Definition at line 749 of file GUnicode.cpp.
GUTF8String GUTF8String::downcase | ( | void | ) | const [inline] |
GUTF8String& GUTF8String::format | ( | const char * | fmt, | |
... | ||||
) |
Initializes a string with a formatted string (as in printf#).
The string is re-initialized with the characters generated according to the specified format fmt# and using the optional arguments. See the ANSI-C function printf()# for more information. The current implementation will cause a segmentation violation if the resulting string is longer than 32768 characters.
GUTF8String GUTF8String::fromEscaped | ( | const GMap< GUTF8String, GUTF8String > | ConvMap | ) | const |
Converts strings containing HTML/XML escaped characters (e.g., "<" for "<") into their unescaped forms.
The conversion is partially defined by the ConvMap argument which specifies the conversion strings to be recognized. Numeric representations of characters (e.g., "&" or "&" for "*") are always converted.
Definition at line 1497 of file GString.cpp.
GUTF8String GUTF8String::fromEscaped | ( | void | ) | const |
Converts strings containing HTML/XML escaped characters into their unescaped forms.
Numeric representations of characters (e.g., "&" or "&" for "*") are the only forms converted by this function.
Definition at line 1574 of file GString.cpp.
GP< GStringRep::Unicode > GUTF8String::get_remainder | ( | void | ) | const [inline] |
char * GUTF8String::getbuf | ( | int | n = -1 |
) |
Provides a direct access to the string buffer.
Returns a pointer for directly accessing the string buffer. This pointer valid remains valid as long as the string is not modified by other means. Positive values for argument n# represent the length of the returned buffer. The returned string buffer will be large enough to hold at least n# characters plus a null character. If n# is positive but smaller than the string length, the string will be truncated to n# characters.
Definition at line 2640 of file GString.cpp.
GUTF8String & GUTF8String::init | ( | const GP< GStringRep > & | rep | ) | [inline] |
void GUTF8String::init | ( | void | ) | [inline] |
GUTF8String GUTF8String::operator+ | ( | const char * | s2 | ) | const |
Definition at line 2636 of file GString.cpp.
GUTF8String GUTF8String::operator+ | ( | const GNativeString & | s2 | ) | const |
GUTF8String GUTF8String::operator+ | ( | const GUTF8String & | s2 | ) | const |
Concatenates strings.
Returns a string composed by concatenating the characters of strings s1# and s2#.
Reimplemented from GBaseString.
Definition at line 2632 of file GString.cpp.
GUTF8String GUTF8String::operator+ | ( | const GBaseString & | s2 | ) | const |
Concatenates strings.
Returns a string composed by concatenating the characters of strings s1# and s2#.
Definition at line 2628 of file GString.cpp.
GUTF8String & GUTF8String::operator+= | ( | const GBaseString & | str | ) |
GUTF8String & GUTF8String::operator+= | ( | const char * | str | ) |
Appends the null terminated character array str# to the string.
Definition at line 2612 of file GString.cpp.
GUTF8String & GUTF8String::operator+= | ( | char | ch | ) |
GUTF8String & GUTF8String::operator= | ( | const GNativeString & | str | ) | [inline] |
GUTF8String & GUTF8String::operator= | ( | const GUTF8String & | str | ) | [inline] |
GUTF8String & GUTF8String::operator= | ( | const GBaseString & | str | ) | [inline] |
GUTF8String & GUTF8String::operator= | ( | const GP< GStringRep > & | sptr | ) | [inline] |
Assigns a smart-pointer to a smart-pointer lvalue.
- Parameters:
-
sptr smart-pointer copied into this smart-pointer.
Reimplemented from GP< GStringRep >.
GUTF8String & GUTF8String::operator= | ( | const char * | str | ) |
Definition at line 2713 of file GString.cpp.
GUTF8String & GUTF8String::operator= | ( | const char | str | ) |
Copy a null terminated character array.
Resets this string with the character string contained in the null terminated character array str#.
Definition at line 2710 of file GString.cpp.
void GUTF8String::setat | ( | const int | n, | |
const char | ch | |||
) |
Set the character at position n# to value ch#.
An exception {GException} is thrown if number n# is not in range #-len# to len#, where len# is the length of the string. If character ch# is zero, the string is truncated at position n#. The first character of a string is numbered zero. Negative positions represent characters relative to the end of the string. If position n# is equal to the length of the string, this function appends character ch# to the end of the string.
Definition at line 2652 of file GString.cpp.
GUTF8String GUTF8String::substr | ( | int | from, | |
int | len | |||
) | const |
Returns a sub-string.
The sub-string is composed by copying len# characters starting at position from# in this string. The length of the resulting string may be smaller than len# if the specified range is too large.
Definition at line 2624 of file GString.cpp.
GUTF8String GUTF8String::toEscaped | ( | const bool | tosevenbit = false |
) | const [inline] |
GUTF8String GUTF8String::upcase | ( | void | ) | const [inline] |
GUTF8String & GUTF8String::vformat | ( | const GUTF8String & | fmt, | |
va_list & | args | |||
) | [inline] |
Initializes a string with a formatted string (as in vprintf#).
The string is re-initialized with the characters generated according to the specified format fmt# and using the optional arguments. See the ANSI-C function vprintf()# for more information. The current implementation will cause a segmentation violation if the resulting string is longer than 32768 characters.
Friends And Related Function Documentation
GUTF8String operator+ | ( | const char * | s1, | |
const GUTF8String & | s2 | |||
) | [friend] |
Definition at line 2740 of file GString.cpp.
The documentation for this class was generated from the following files: