kviewshell
GStringRep Class Reference
#include <GString.h>
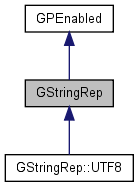
Classes | |
class | UTF8 |
Public Types | |
enum | EncodeType { XUCS4, XUCS4BE, XUCS4LE, XUCS4_2143, XUCS4_3412, XUTF16, XUTF16BE, XUTF16LE, XUTF8, XEBCDIC, XOTHER } |
enum | EscapeMode { UNKNOWN_ESCAPED = 0, IS_ESCAPED = 1, NOT_ESCAPED = 2 } |
Public Member Functions | |
GP< GStringRep > | append (const char *s2) const |
virtual GP< GStringRep > | append (const GP< GStringRep > &s2) const =0 |
virtual GP< GStringRep > | blank (const unsigned int sz) const =0 |
int | cmp (const char *s2, const int len=(-1)) const |
virtual int | cmp (const GP< GStringRep > &s2, const int len=(-1)) const =0 |
GP< GStringRep > | concat (const char *s1, const char *s2) const |
GP< GStringRep > | concat (const GP< GStringRep > &s1, const char *s2) const |
GP< GStringRep > | concat (const char *s1, const GP< GStringRep > &s2) const |
GP< GStringRep > | concat (const GP< GStringRep > &s1, const GP< GStringRep > &s2) const |
int | contains (char const accept[], int from=0) const |
GP< GStringRep > | downcase (void) const |
int | firstEndSpace (int from=0, const int len=(-1)) const |
GP< GStringRep > | getbuf (int n) const |
int | getUCS4 (unsigned long &w, const int from) const |
GStringRep (void) | |
virtual bool | is_valid (void) const =0 |
virtual bool | isNative (void) const |
virtual bool | isUTF8 (void) const |
virtual int | ncopy (wchar_t *const buf, const int buflen) const =0 |
int | nextChar (const int from=0) const |
int | nextNonSpace (const int from=0, const int len=(-1)) const |
int | nextSpace (const int from=0, const int len=(-1)) const |
int | rcontains (char const accept[], int from=0) const |
int | rsearch (char const *str, int from=0) const |
int | rsearch (char c, int from=0) const |
int | search (char const *str, int from=0) const |
int | search (char c, int from=0) const |
GP< GStringRep > | setat (int n, char ch) const |
GP< GStringRep > | strdup (const char *s) const |
GP< GStringRep > | substr (const unsigned long *s, const int start, const int length=(-1)) const |
GP< GStringRep > | substr (const unsigned short *s, const int start, const int length=(-1)) const |
GP< GStringRep > | substr (const char *s, const int start, const int length=(-1)) const |
virtual double | toDouble (const int pos, int &endpos) const =0 |
GP< GStringRep > | toEscaped (const bool tosevenbit) const |
virtual int | toInt (void) const =0 |
virtual long int | toLong (const int pos, int &endpos, const int base=10) const =0 |
virtual GP< GStringRep > | toNative (const EscapeMode escape=UNKNOWN_ESCAPED) const =0 |
virtual GP< GStringRep > | toThis (const GP< GStringRep > &rep, const GP< GStringRep > &locale=0) const =0 |
virtual unsigned long | toULong (const int pos, int &endpos, const int base=10) const =0 |
virtual GP< GStringRep > | toUTF8 (const bool nothrow=false) const =0 |
virtual unsigned char * | UCS4toString (const unsigned long w, unsigned char *ptr, mbstate_t *ps=0) const =0 |
GP< GStringRep > | upcase (void) const |
GP< GStringRep > | vformat (va_list args) const |
virtual | ~GStringRep () |
Static Public Member Functions | |
static int | cmp (const char *s1, const char *s2, const int len=(-1)) |
static int | cmp (const char *s1, const GP< GStringRep > &s2, const int len=(-1)) |
static int | cmp (const GP< GStringRep > &s1, const char *s2, const int len=(-1)) |
static int | cmp (const GP< GStringRep > &s1, const GP< GStringRep > &s2, const int len=(-1)) |
template<class TYPE > | |
static GP< GStringRep > | create (const unsigned int sz, TYPE *) |
static GP< GStringRep > | NativeToUTF8 (const char *s) |
static unsigned char * | UCS4toNative (const unsigned long w, unsigned char *ptr, mbstate_t *ps) |
static int | UCS4toUTF16 (unsigned long w, unsigned short &w1, unsigned short &w2) |
static unsigned char * | UCS4toUTF8 (const unsigned long w, unsigned char *ptr) |
static int | UTF16toUCS4 (unsigned long &w, unsigned short const *const s, void const *const eptr) |
static GP< GStringRep > | UTF8ToNative (const char *s, const EscapeMode escape=UNKNOWN_ESCAPED) |
static int | UTF8toUCS4 (unsigned long &w, unsigned char const s[], void const *const endptr) |
static unsigned long | UTF8toUCS4 (unsigned char const *&s, void const *const endptr) |
Protected Member Functions | |
virtual GP< Unicode > | get_remainder (void) const |
virtual unsigned long | getValidUCS4 (const char *&source) const =0 |
const char * | isCharType (bool(*xiswtest)(const unsigned long wc), const char *ptr, const bool reverse=false) const |
int | nextCharType (bool(*xiswtest)(const unsigned long wc), const int from, const int len, const bool reverse=false) const |
virtual void | set_remainder (const GP< Unicode > &remainder) |
virtual void | set_remainder (void const *const buf, const unsigned int size, const GP< GStringRep > &encoding) |
virtual void | set_remainder (void const *const buf, const unsigned int size, const EncodeType encodetype) |
GP< GStringRep > | tocase (bool(*xiswcase)(const unsigned long wc), unsigned long(*xtowcase)(const unsigned long wc)) const |
Static Protected Member Functions | |
static bool | giswlower (const unsigned long w) |
static bool | giswspace (const unsigned long w) |
static bool | giswupper (const unsigned long w) |
static unsigned long | gtowlower (const unsigned long w) |
static unsigned long | gtowupper (const unsigned long w) |
Protected Attributes | |
char * | data |
int | size |
Friends | |
unsigned int | hash (const GBaseString &ref) |
Detailed Description
Definition at line 144 of file GString.h.
Member Enumeration Documentation
Constructor & Destructor Documentation
GStringRep::GStringRep | ( | void | ) |
Definition at line 2240 of file GString.cpp.
GStringRep::~GStringRep | ( | ) | [virtual] |
Definition at line 2249 of file GString.cpp.
Member Function Documentation
GP< GStringRep > GStringRep::append | ( | const char * | s2 | ) | const |
Definition at line 1166 of file GString.cpp.
virtual GP<GStringRep> GStringRep::append | ( | const GP< GStringRep > & | s2 | ) | const [pure virtual] |
Implemented in GStringRep::UTF8.
virtual GP<GStringRep> GStringRep::blank | ( | const unsigned int | sz | ) | const [pure virtual] |
Implemented in GStringRep::UTF8.
int GStringRep::cmp | ( | const char * | s1, | |
const char * | s2, | |||
const int | len = (-1) | |||
) | [static] |
Definition at line 2270 of file GString.cpp.
int GStringRep::cmp | ( | const char * | s1, | |
const GP< GStringRep > & | s2, | |||
const int | len = (-1) | |||
) | [static] |
Definition at line 2298 of file GString.cpp.
int GStringRep::cmp | ( | const GP< GStringRep > & | s1, | |
const char * | s2, | |||
const int | len = (-1) | |||
) | [static] |
Definition at line 2291 of file GString.cpp.
int GStringRep::cmp | ( | const GP< GStringRep > & | s1, | |
const GP< GStringRep > & | s2, | |||
const int | len = (-1) | |||
) | [static] |
Definition at line 2284 of file GString.cpp.
int GStringRep::cmp | ( | const char * | s2, | |
const int | len = (-1) | |||
) | const |
Definition at line 2264 of file GString.cpp.
virtual int GStringRep::cmp | ( | const GP< GStringRep > & | s2, | |
const int | len = (-1) | |||
) | const [pure virtual] |
Implemented in GStringRep::UTF8.
GP< GStringRep > GStringRep::concat | ( | const char * | s1, | |
const char * | s2 | |||
) | const |
Definition at line 1198 of file GString.cpp.
GP< GStringRep > GStringRep::concat | ( | const GP< GStringRep > & | s1, | |
const char * | s2 | |||
) | const |
Definition at line 2187 of file GString.cpp.
GP< GStringRep > GStringRep::concat | ( | const char * | s1, | |
const GP< GStringRep > & | s2 | |||
) | const |
Definition at line 2161 of file GString.cpp.
GP< GStringRep > GStringRep::concat | ( | const GP< GStringRep > & | s1, | |
const GP< GStringRep > & | s2 | |||
) | const |
Definition at line 2211 of file GString.cpp.
int GStringRep::contains | ( | char const | accept[], | |
int | from = 0 | |||
) | const |
Definition at line 1802 of file GString.cpp.
GP< GStringRep > GStringRep::create | ( | const unsigned int | sz, | |
TYPE * | ||||
) | [inline, static] |
Definition at line 1013 of file GString.cpp.
GP< GStringRep > GStringRep::downcase | ( | void | ) | const |
Definition at line 151 of file GString.cpp.
int GStringRep::firstEndSpace | ( | int | from = 0 , |
|
const int | len = (-1) | |||
) | const |
Definition at line 2521 of file GString.cpp.
virtual GP<Unicode> GStringRep::get_remainder | ( | void | ) | const [protected, virtual] |
GP< GStringRep > GStringRep::getbuf | ( | int | n | ) | const |
Definition at line 1230 of file GString.cpp.
int GStringRep::getUCS4 | ( | unsigned long & | w, | |
const int | from | |||
) | const |
Definition at line 2473 of file GString.cpp.
virtual unsigned long GStringRep::getValidUCS4 | ( | const char *& | source | ) | const [protected, pure virtual] |
Implemented in GStringRep::UTF8.
bool GStringRep::giswlower | ( | const unsigned long | w | ) | [static, protected] |
Definition at line 1318 of file GString.cpp.
bool GStringRep::giswspace | ( | const unsigned long | w | ) | [static, protected] |
Definition at line 1293 of file GString.cpp.
bool GStringRep::giswupper | ( | const unsigned long | w | ) | [static, protected] |
Definition at line 1307 of file GString.cpp.
unsigned long GStringRep::gtowlower | ( | const unsigned long | w | ) | [static, protected] |
Definition at line 1340 of file GString.cpp.
unsigned long GStringRep::gtowupper | ( | const unsigned long | w | ) | [static, protected] |
Definition at line 1329 of file GString.cpp.
virtual bool GStringRep::is_valid | ( | void | ) | const [pure virtual] |
Implemented in GStringRep::UTF8.
const char * GStringRep::isCharType | ( | bool(*)(const unsigned long wc) | xiswtest, | |
const char * | ptr, | |||
const bool | reverse = false | |||
) | const [protected] |
Definition at line 1246 of file GString.cpp.
virtual bool GStringRep::isNative | ( | void | ) | const [inline, virtual] |
virtual bool GStringRep::isUTF8 | ( | void | ) | const [inline, virtual] |
GP< GStringRep > GStringRep::NativeToUTF8 | ( | const char * | s | ) | [static] |
Definition at line 261 of file GString.cpp.
virtual int GStringRep::ncopy | ( | wchar_t *const | buf, | |
const int | buflen | |||
) | const [pure virtual] |
Implemented in GStringRep::UTF8.
int GStringRep::nextChar | ( | const int | from = 0 |
) | const |
Definition at line 2513 of file GString.cpp.
int GStringRep::nextCharType | ( | bool(*)(const unsigned long wc) | xiswtest, | |
const int | from, | |||
const int | len, | |||
const bool | reverse = false | |||
) | const [protected] |
Definition at line 1261 of file GString.cpp.
int GStringRep::nextNonSpace | ( | const int | from = 0 , |
|
const int | len = (-1) | |||
) | const |
Definition at line 2501 of file GString.cpp.
int GStringRep::nextSpace | ( | const int | from = 0 , |
|
const int | len = (-1) | |||
) | const |
Definition at line 2507 of file GString.cpp.
int GStringRep::rcontains | ( | char const | accept[], | |
int | from = 0 | |||
) | const |
Definition at line 1824 of file GString.cpp.
int GStringRep::rsearch | ( | char const * | str, | |
int | from = 0 | |||
) | const |
Definition at line 1787 of file GString.cpp.
int GStringRep::rsearch | ( | char | c, | |
int | from = 0 | |||
) | const |
Definition at line 1768 of file GString.cpp.
int GStringRep::search | ( | char const * | str, | |
int | from = 0 | |||
) | const |
Definition at line 1749 of file GString.cpp.
int GStringRep::search | ( | char | c, | |
int | from = 0 | |||
) | const |
Definition at line 1734 of file GString.cpp.
virtual void GStringRep::set_remainder | ( | const GP< Unicode > & | remainder | ) | [protected, virtual] |
virtual void GStringRep::set_remainder | ( | void const *const | buf, | |
const unsigned int | size, | |||
const GP< GStringRep > & | encoding | |||
) | [protected, virtual] |
virtual void GStringRep::set_remainder | ( | void const *const | buf, | |
const unsigned int | size, | |||
const EncodeType | encodetype | |||
) | [protected, virtual] |
GP< GStringRep > GStringRep::setat | ( | int | n, | |
char | ch | |||
) | const |
Definition at line 1581 of file GString.cpp.
GP< GStringRep > GStringRep::strdup | ( | const char * | s | ) | const |
Definition at line 1028 of file GString.cpp.
GP< GStringRep > GStringRep::substr | ( | const unsigned long * | s, | |
const int | start, | |||
const int | length = (-1) | |||
) | const |
Definition at line 1133 of file GString.cpp.
GP< GStringRep > GStringRep::substr | ( | const unsigned short * | s, | |
const int | start, | |||
const int | length = (-1) | |||
) | const |
Definition at line 1095 of file GString.cpp.
GP< GStringRep > GStringRep::substr | ( | const char * | s, | |
const int | start, | |||
const int | length = (-1) | |||
) | const |
Definition at line 1047 of file GString.cpp.
GP< GStringRep > GStringRep::tocase | ( | bool(*)(const unsigned long wc) | xiswcase, | |
unsigned long(*)(const unsigned long wc) | xtowcase | |||
) | const [protected] |
Definition at line 1351 of file GString.cpp.
virtual double GStringRep::toDouble | ( | const int | pos, | |
int & | endpos | |||
) | const [pure virtual] |
Implemented in GStringRep::UTF8.
GP< GStringRep > GStringRep::toEscaped | ( | const bool | tosevenbit | ) | const |
Definition at line 1410 of file GString.cpp.
virtual int GStringRep::toInt | ( | void | ) | const [pure virtual] |
Implemented in GStringRep::UTF8.
virtual long int GStringRep::toLong | ( | const int | pos, | |
int & | endpos, | |||
const int | base = 10 | |||
) | const [pure virtual] |
Implemented in GStringRep::UTF8.
virtual GP<GStringRep> GStringRep::toNative | ( | const EscapeMode | escape = UNKNOWN_ESCAPED |
) | const [pure virtual] |
Implemented in GStringRep::UTF8.
virtual GP<GStringRep> GStringRep::toThis | ( | const GP< GStringRep > & | rep, | |
const GP< GStringRep > & | locale = 0 | |||
) | const [pure virtual] |
Implemented in GStringRep::UTF8.
virtual unsigned long GStringRep::toULong | ( | const int | pos, | |
int & | endpos, | |||
const int | base = 10 | |||
) | const [pure virtual] |
Implemented in GStringRep::UTF8.
virtual GP<GStringRep> GStringRep::toUTF8 | ( | const bool | nothrow = false |
) | const [pure virtual] |
Implemented in GStringRep::UTF8.
static unsigned char* GStringRep::UCS4toNative | ( | const unsigned long | w, | |
unsigned char * | ptr, | |||
mbstate_t * | ps | |||
) | [static] |
virtual unsigned char* GStringRep::UCS4toString | ( | const unsigned long | w, | |
unsigned char * | ptr, | |||
mbstate_t * | ps = 0 | |||
) | const [pure virtual] |
Implemented in GStringRep::UTF8.
int GStringRep::UCS4toUTF16 | ( | unsigned long | w, | |
unsigned short & | w1, | |||
unsigned short & | w2 | |||
) | [static] |
Definition at line 2547 of file GString.cpp.
unsigned char * GStringRep::UCS4toUTF8 | ( | const unsigned long | w, | |
unsigned char * | ptr | |||
) | [static] |
Definition at line 2111 of file GString.cpp.
GP< GStringRep > GStringRep::upcase | ( | void | ) | const |
Definition at line 147 of file GString.cpp.
int GStringRep::UTF16toUCS4 | ( | unsigned long & | w, | |
unsigned short const *const | s, | |||
void const *const | eptr | |||
) | [static] |
GP< GStringRep > GStringRep::UTF8ToNative | ( | const char * | s, | |
const EscapeMode | escape = UNKNOWN_ESCAPED | |||
) | [static] |
Definition at line 2664 of file GString.cpp.
static int GStringRep::UTF8toUCS4 | ( | unsigned long & | w, | |
unsigned char const | s[], | |||
void const *const | endptr | |||
) | [inline, static] |
unsigned long GStringRep::UTF8toUCS4 | ( | unsigned char const *& | s, | |
void const *const | endptr | |||
) | [static] |
Returns the next UCS4 character, and updates the pointer s.
Definition at line 2006 of file GString.cpp.
GP< GStringRep > GStringRep::vformat | ( | va_list | args | ) | const |
Initializes a string with a formatted string (as in vprintf#).
The string is re-initialized with the characters generated according to the specified format fmt# and using the optional arguments. See the ANSI-C function vprintf()# for more information. The current implementation will cause a segmentation violation if the resulting string is longer than 32768 characters.
Definition at line 1635 of file GString.cpp.
Friends And Related Function Documentation
unsigned int hash | ( | const GBaseString & | ref | ) | [friend] |
Definition at line 1867 of file GString.cpp.
Member Data Documentation
char* GStringRep::data [protected] |
int GStringRep::size [protected] |
The documentation for this class was generated from the following files: