kviewshell
GBaseString Class Reference
General purpose character string. More...
#include <GString.h>
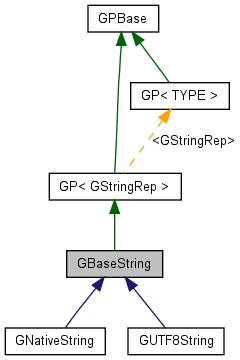
Public Types | |
enum | EscapeMode { UNKNOWN_ESCAPED = GStringRep::UNKNOWN_ESCAPED, IS_ESCAPED = GStringRep::IS_ESCAPED, NOT_ESCAPED = GStringRep::NOT_ESCAPED } |
Public Member Functions | |
int | cmp (const char s2) const |
int | cmp (const char *s2, const int len=(-1)) const |
int | cmp (const GBaseString &s2, const int len=(-1)) const |
int | contains (const char accept[], const int from=0) const |
void | empty (void) |
int | firstEndSpace (const int from=0, const int len=(-1)) const |
GUTF8String | getNative2UTF8 (void) const |
GNativeString | getUTF82Native (EscapeMode escape=UNKNOWN_ESCAPED) const |
bool | is_float (void) const |
bool | is_int (void) const |
bool | is_valid (void) const |
unsigned int | length (void) const |
GUTF8String | NativeToUTF8 (void) const |
int | ncopy (wchar_t *const buf, const int buflen) const |
int | nextChar (const int from=0) const |
int | nextNonSpace (const int from=0, const int len=(-1)) const |
int | nextSpace (const int from=0, const int len=(-1)) const |
operator const char * (void) const | |
bool | operator! (void) const |
bool | operator!= (const char *s2) const |
bool | operator!= (const GBaseString &s2) const |
GNativeString | operator+ (const GNativeString &s2) const |
GUTF8String | operator+ (const GUTF8String &s2) const |
bool | operator< (const char s2) const |
bool | operator< (const char *s2) const |
bool | operator< (const GBaseString &s2) const |
bool | operator<= (const char s2) const |
bool | operator<= (const char *s2) const |
bool | operator<= (const GBaseString &s2) const |
bool | operator== (const char *s2) const |
bool | operator== (const GBaseString &s2) const |
bool | operator> (const char s2) const |
bool | operator> (const char *s2) const |
bool | operator> (const GBaseString &s2) const |
bool | operator>= (const char s2) const |
bool | operator>= (const char *s2) const |
bool | operator>= (const GBaseString &s2) const |
char | operator[] (int n) const |
int | rcontains (const char accept[], const int from=0) const |
int | rsearch (const char *str, const int from=0) const |
int | rsearch (char c, const int from=0) const |
int | search (const char *str, int from=0) const |
int | search (char c, int from=0) const |
double | toDouble (const int pos, int &endpos) const |
int | toInt (void) const |
long | toLong (const int pos, int &endpos, const int base=10) const |
unsigned long | toULong (const int pos, int &endpos, const int base=10) const |
GNativeString | UTF8ToNative (const bool currentlocale=false, const EscapeMode escape=UNKNOWN_ESCAPED) const |
Static Public Member Functions | |
static int | cmp (const char *s1, const char *s2, const int len=(-1)) |
static double | toDouble (const GNativeString &src, const int pos, int &endpos) |
static double | toDouble (const GUTF8String &src, const int pos, int &endpos) |
static long | toLong (const GNativeString &src, const int pos, int &endpos, const int base=10) |
static long | toLong (const GUTF8String &src, const int pos, int &endpos, const int base=10) |
static unsigned long | toULong (const GNativeString &src, const int pos, int &endpos, const int base=10) |
static unsigned long | toULong (const GUTF8String &src, const int pos, int &endpos, const int base=10) |
Protected Member Functions | |
int | CheckSubscript (int n) const |
GBaseString (void) | |
GBaseString & | init (const GP< GStringRep > &rep) |
void | init (void) |
~GBaseString () | |
Static Protected Member Functions | |
static void | throw_illegal_subscript () no_return |
Protected Attributes | |
const char * | gstr |
Static Protected Attributes | |
static const char * | nullstr = "" |
Friends | |
unsigned int | hash (const GBaseString &ref) |
bool | operator!= (const char *s1, const GBaseString &s2) |
bool | operator< (const char s1, const GBaseString &s2) |
bool | operator< (const char *s1, const GBaseString &s2) |
bool | operator<= (const char s1, const GBaseString &s2) |
bool | operator<= (const char *s1, const GBaseString &s2) |
bool | operator== (const char *s1, const GBaseString &s2) |
bool | operator> (const char s1, const GBaseString &s2) |
bool | operator> (const char *s1, const GBaseString &s2) |
bool | operator>= (const char s1, const GBaseString &s2) |
bool | operator>= (const char *s1, const GBaseString &s2) |
Detailed Description
General purpose character string.Each dirivied instance of class GBaseString# represents a character string. Overloaded operators provide a value semantic to GBaseString# objects. Conversion operators and constructors transparently convert between GBaseString# objects and const char*# pointers. The GBaseString# class has no public constructors, since a dirived type should always be used to specify the desired multibyte character encoding.
Functions taking strings as arguments should declare their arguments as "#const char*#". Such functions will work equally well with dirived GBaseString# objects since there is a fast conversion operator from the dirivied GBaseString# objects to "#const char*#". Functions returning strings should return GUTF8String# or GNativeString# objects because the class will automatically manage the necessary memory.
Characters in the string can be identified by their position. The first character of a string is numbered zero. Negative positions represent characters relative to the end of the string (i.e. position #-1# accesses the last character of the string, position #-2# represents the second last character, etc.)
Definition at line 464 of file GString.h.
Member Enumeration Documentation
Constructor & Destructor Documentation
GBaseString::~GBaseString | ( | ) | [protected] |
Definition at line 112 of file GString.cpp.
GBaseString::GBaseString | ( | void | ) | [inline, protected] |
Member Function Documentation
int GBaseString::CheckSubscript | ( | int | n | ) | const [inline, protected] |
int GBaseString::cmp | ( | const char * | s1, | |
const char * | s2, | |||
const int | len = (-1) | |||
) | [inline, static] |
int GBaseString::cmp | ( | const char | s2 | ) | const [inline] |
int GBaseString::cmp | ( | const char * | s2, | |
const int | len = (-1) | |||
) | const [inline] |
int GBaseString::cmp | ( | const GBaseString & | s2, | |
const int | len = (-1) | |||
) | const [inline] |
int GBaseString::contains | ( | const char | accept[], | |
const int | from = 0 | |||
) | const [inline] |
void GBaseString::empty | ( | void | ) |
int GBaseString::firstEndSpace | ( | const int | from = 0 , |
|
const int | len = (-1) | |||
) | const [inline] |
GUTF8String GBaseString::getNative2UTF8 | ( | void | ) | const |
GNativeString GBaseString::getUTF82Native | ( | EscapeMode | escape = UNKNOWN_ESCAPED |
) | const |
Converts strings between native & UTF8.
GBaseString & GBaseString::init | ( | const GP< GStringRep > & | rep | ) | [inline, protected] |
void GBaseString::init | ( | void | ) | [inline, protected] |
bool GBaseString::is_float | ( | void | ) | const |
bool GBaseString::is_int | ( | void | ) | const |
Returns TRUE# if the string contains an integer number.
Definition at line 1835 of file GString.cpp.
bool GBaseString::is_valid | ( | void | ) | const [inline] |
unsigned int GBaseString::length | ( | void | ) | const [inline] |
GUTF8String GBaseString::NativeToUTF8 | ( | void | ) | const |
int GBaseString::ncopy | ( | wchar_t *const | buf, | |
const int | buflen | |||
) | const [inline] |
int GBaseString::nextChar | ( | const int | from = 0 |
) | const [inline] |
int GBaseString::nextNonSpace | ( | const int | from = 0 , |
|
const int | len = (-1) | |||
) | const [inline] |
int GBaseString::nextSpace | ( | const int | from = 0 , |
|
const int | len = (-1) | |||
) | const [inline] |
GBaseString::operator const char * | ( | void | ) | const [inline] |
bool GBaseString::operator! | ( | void | ) | const [inline] |
Returns true if and only if the string contains zero characters.
This operator is useful for conditional expression in control structures. {verbatim} if (! str) { ... } while (!! str) { ... } -- Note the double operator! {verbatim} Class GBaseString# does not to support syntax "#if# #(str)# #{}#" because the required conversion operator introduces dangerous ambiguities with certain compilers.
Reimplemented from GP< GStringRep >.
bool GBaseString::operator!= | ( | const char * | s2 | ) | const [inline] |
bool GBaseString::operator!= | ( | const GBaseString & | s2 | ) | const [inline] |
GNativeString GBaseString::operator+ | ( | const GNativeString & | s2 | ) | const |
Reimplemented in GUTF8String.
GUTF8String GBaseString::operator+ | ( | const GUTF8String & | s2 | ) | const |
Concatenates strings.
Returns a string composed by concatenating the characters of strings s1# and s2#.
Reimplemented in GUTF8String.
Definition at line 2716 of file GString.cpp.
bool GBaseString::operator< | ( | const char | s2 | ) | const [inline] |
bool GBaseString::operator< | ( | const char * | s2 | ) | const [inline] |
bool GBaseString::operator< | ( | const GBaseString & | s2 | ) | const [inline] |
bool GBaseString::operator<= | ( | const char | s2 | ) | const [inline] |
bool GBaseString::operator<= | ( | const char * | s2 | ) | const [inline] |
bool GBaseString::operator<= | ( | const GBaseString & | s2 | ) | const [inline] |
bool GBaseString::operator== | ( | const char * | s2 | ) | const [inline] |
bool GBaseString::operator== | ( | const GBaseString & | s2 | ) | const [inline] |
Returns a boolean.
The Standard C strncmp takes two string and compares the first N characters. static bool GBaseString::ncmp will compare s1# with s2# with the len# characters starting from the beginning of the string. String comparison. Returns true if and only if character strings s1# and s2# are equal (as with strcmp#.)
bool GBaseString::operator> | ( | const char | s2 | ) | const [inline] |
bool GBaseString::operator> | ( | const char * | s2 | ) | const [inline] |
bool GBaseString::operator> | ( | const GBaseString & | s2 | ) | const [inline] |
bool GBaseString::operator>= | ( | const char | s2 | ) | const [inline] |
bool GBaseString::operator>= | ( | const char * | s2 | ) | const [inline] |
bool GBaseString::operator>= | ( | const GBaseString & | s2 | ) | const [inline] |
char GBaseString::operator[] | ( | int | n | ) | const [inline] |
Returns the character at position n#.
An exception {GException} is thrown if number n# is not in range #-len# to len-1#, where len# is the length of the string. The first character of a string is numbered zero. Negative positions represent characters relative to the end of the string.
int GBaseString::rcontains | ( | const char | accept[], | |
const int | from = 0 | |||
) | const [inline] |
int GBaseString::rsearch | ( | const char * | str, | |
const int | from = 0 | |||
) | const [inline] |
Searches sub-string str# in the string, starting at position from# and scanning backwards until reaching the beginning of the string.
This function returns the position of the first matching character of the sub-string. It returns #-1# if string str# cannot be found.
int GBaseString::rsearch | ( | char | c, | |
const int | from = 0 | |||
) | const [inline] |
int GBaseString::search | ( | const char * | str, | |
int | from = 0 | |||
) | const [inline] |
Searches sub-string str# in the string, starting at position from# and scanning forward until reaching the end of the string.
This function returns the position of the first matching character of the sub-string. It returns #-1# if string str# cannot be found.
int GBaseString::search | ( | char | c, | |
int | from = 0 | |||
) | const [inline] |
void GBaseString::throw_illegal_subscript | ( | ) | [static, protected] |
Definition at line 1877 of file GString.cpp.
double GBaseString::toDouble | ( | const GNativeString & | src, | |
const int | pos, | |||
int & | endpos | |||
) | [inline, static] |
double GBaseString::toDouble | ( | const GUTF8String & | src, | |
const int | pos, | |||
int & | endpos | |||
) | [inline, static] |
double GBaseString::toDouble | ( | const int | pos, | |
int & | endpos | |||
) | const [inline] |
int GBaseString::toInt | ( | void | ) | const [inline] |
long GBaseString::toLong | ( | const GNativeString & | src, | |
const int | pos, | |||
int & | endpos, | |||
const int | base = 10 | |||
) | [inline, static] |
long GBaseString::toLong | ( | const GUTF8String & | src, | |
const int | pos, | |||
int & | endpos, | |||
const int | base = 10 | |||
) | [inline, static] |
long GBaseString::toLong | ( | const int | pos, | |
int & | endpos, | |||
const int | base = 10 | |||
) | const [inline] |
unsigned long GBaseString::toULong | ( | const GNativeString & | src, | |
const int | pos, | |||
int & | endpos, | |||
const int | base = 10 | |||
) | [inline, static] |
unsigned long GBaseString::toULong | ( | const GUTF8String & | src, | |
const int | pos, | |||
int & | endpos, | |||
const int | base = 10 | |||
) | [inline, static] |
unsigned long GBaseString::toULong | ( | const int | pos, | |
int & | endpos, | |||
const int | base = 10 | |||
) | const [inline] |
GNativeString GBaseString::UTF8ToNative | ( | const bool | currentlocale = false , |
|
const EscapeMode | escape = UNKNOWN_ESCAPED | |||
) | const |
Friends And Related Function Documentation
unsigned int hash | ( | const GBaseString & | ref | ) | [friend] |
Returns an integer.
Implements a functional i18n atoi. Note that if you pass a GBaseString that is not in Native format the results may be disparaging. Returns a hash code for the string. This hashing function helps when creating associative maps with string keys (see {GMap}). This hash code may be reduced to an arbitrary range by computing its remainder modulo the upper bound of the range.
Definition at line 1867 of file GString.cpp.
bool operator!= | ( | const char * | s1, | |
const GBaseString & | s2 | |||
) | [friend] |
bool operator< | ( | const char | s1, | |
const GBaseString & | s2 | |||
) | [friend] |
bool operator< | ( | const char * | s1, | |
const GBaseString & | s2 | |||
) | [friend] |
bool operator<= | ( | const char | s1, | |
const GBaseString & | s2 | |||
) | [friend] |
bool operator<= | ( | const char * | s1, | |
const GBaseString & | s2 | |||
) | [friend] |
bool operator== | ( | const char * | s1, | |
const GBaseString & | s2 | |||
) | [friend] |
bool operator> | ( | const char | s1, | |
const GBaseString & | s2 | |||
) | [friend] |
bool operator> | ( | const char * | s1, | |
const GBaseString & | s2 | |||
) | [friend] |
bool operator>= | ( | const char | s1, | |
const GBaseString & | s2 | |||
) | [friend] |
bool operator>= | ( | const char * | s1, | |
const GBaseString & | s2 | |||
) | [friend] |
Member Data Documentation
const char* GBaseString::gstr [protected] |
const char * GBaseString::nullstr = "" [static, protected] |
The documentation for this class was generated from the following files: