kviewshell
BSByteStream Class Reference
Performs bzz compression/decompression. More...
#include <BSByteStream.h>
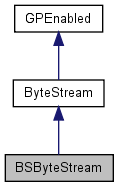
Public Types | |
enum | { MINBLOCK = 10, MAXBLOCK = 4096 } |
enum | { FREQMAX = 4, CTXIDS = 3 } |
Public Member Functions | |
virtual void | flush (void)=0 |
virtual long | tell (void) const |
~BSByteStream () | |
Static Public Member Functions | |
static GP< ByteStream > | create (GP< ByteStream > bs, const int blocksize) |
static GP< ByteStream > | create (GP< ByteStream > bs) |
Protected Member Functions | |
BSByteStream (GP< ByteStream > bs) | |
Protected Attributes | |
unsigned int | blocksize |
int | bptr |
ByteStream * | bs |
BitContext | ctx [300] |
unsigned char * | data |
GP< ByteStream > | gbs |
GPBuffer< unsigned char > | gdata |
GP< ZPCodec > | gzp |
long | offset |
int | size |
Detailed Description
Performs bzz compression/decompression.Class BSByteStream# defines a {ByteStream} which transparently performs the BZZ compression/decompression. The constructor of class {BSByteStream} takes another {ByteStream} as argument. Any data written to the BSByteStream is compressed and written to this second ByteStream. Any data read from the BSByteStream is internally generated by decompressing data read from the second ByteStream.
Program {bzz} demonstrates how to use this class. All the hard work is achieved by a simple ByteStream to ByteStream copy, as shown below. {verbatim} GP<ByteStream> in=ByteStreamcreate(infile,"rb"); GP<ByteStream> out=ByteStreamcreate(outfile,"wb"); if (encoding) { BSByteStream bsb(out, blocksize); bsb.copy(*in); } else { BSByteStream bsb(in); out->copy(bsb); } {verbatim} Due to the block oriented nature of the Burrows-Wheeler transform, there is a very significant latency between the data input and the data output. You can use function flush# to force data output at the expense of compression efficiency.
You should never directly access a ByteStream object connected to a valid BSByteStream object. The ByteStream object can be accessed again after the destruction of the BSByteStream object. Note that the encoder always flushes its internal buffers and writes a few final code bytes when the BSByteStream object is destroyed. Note also that the decoder often reads a few bytes beyond the last code byte written by the encoder. This lag means that you must reposition the ByteStream after the destruction of the BSByteStream object and before re-using the ByteStream object (see {IFFByteStream}.)
Definition at line 199 of file BSByteStream.h.
Member Enumeration Documentation
anonymous enum |
anonymous enum |
Constructor & Destructor Documentation
BSByteStream::BSByteStream | ( | GP< ByteStream > | bs | ) | [protected] |
Definition at line 108 of file BSByteStream.cpp.
BSByteStream::~BSByteStream | ( | ) |
Definition at line 116 of file BSByteStream.cpp.
Member Function Documentation
GP< ByteStream > BSByteStream::create | ( | GP< ByteStream > | bs, | |
const int | blocksize | |||
) | [static] |
Constructs a BSByteStream.
The BSByteStream will be used for compressing data. {description} [Compression] Set blocksize# to a positive number smaller than 4096 to initialize the compressor. Data written to the BSByteStream will be accumulated into an internal buffer. The buffered data will be compressed and written to ByteStream bs# whenever the buffer sizes reaches the maximum value specified by argument blocksize# (in kilobytes). Using a larger block size usually increases the compression ratio at the expense of computation time. There is no need however to specify a block size larger than the total number of bytes to compress. Setting blocksize# to #1024# is a good starting point. A minimal block size of 10 is silently enforced. {description}
Definition at line 945 of file BSEncodeByteStream.cpp.
GP< ByteStream > BSByteStream::create | ( | GP< ByteStream > | bs | ) | [static] |
Creates a BSByteStream.
The BSByteStream will be used for decompressing data. {description} [Decompression] The BSByteStream is created and the decompressor initializes. Chunks of data will be read from ByteStream bs# and decompressed into an internal buffer. Function read# can be used to access the decompressed data. {description}
Definition at line 130 of file BSByteStream.cpp.
virtual void BSByteStream::flush | ( | void | ) | [pure virtual] |
Flushes all buffers in the ByteStream.
Calling this function guarantees that pending data have been actually written (i.e. passed to the operating system). Class ByteStream# provides a default implementation which does nothing.
Reimplemented from ByteStream.
long BSByteStream::tell | ( | void | ) | const [virtual] |
Returns the offset of the current position in the ByteStream.
This function {must} be implemented by each subclass of ByteStream#.
Implements ByteStream.
Definition at line 414 of file BSByteStream.cpp.
Member Data Documentation
unsigned int BSByteStream::blocksize [protected] |
Definition at line 249 of file BSByteStream.h.
int BSByteStream::bptr [protected] |
Definition at line 248 of file BSByteStream.h.
ByteStream* BSByteStream::bs [protected] |
Definition at line 251 of file BSByteStream.h.
BitContext BSByteStream::ctx[300] [protected] |
Definition at line 257 of file BSByteStream.h.
unsigned char* BSByteStream::data [protected] |
Definition at line 253 of file BSByteStream.h.
GP<ByteStream> BSByteStream::gbs [protected] |
Definition at line 252 of file BSByteStream.h.
GPBuffer<unsigned char> BSByteStream::gdata [protected] |
Definition at line 254 of file BSByteStream.h.
GP<ZPCodec> BSByteStream::gzp [protected] |
Definition at line 256 of file BSByteStream.h.
long BSByteStream::offset [protected] |
Definition at line 247 of file BSByteStream.h.
int BSByteStream::size [protected] |
Definition at line 250 of file BSByteStream.h.
The documentation for this class was generated from the following files: