kviewshell
DPArray< TYPE > Class Template Reference
Dynamic array for {GPBase}d classes. More...
#include <Arrays.h>
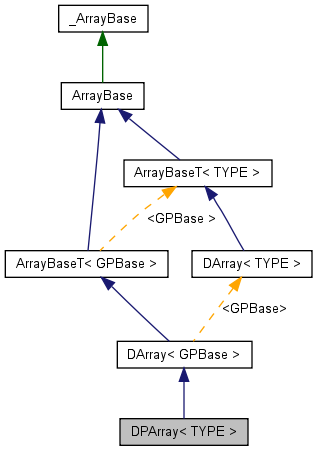
Public Member Functions | |
Arrays.h | |
Files #"Arrays.h"# and #"Arrays.cpp"# implement three array template classes. Class {TArray} implements an array of objects of trivial types such as char#, int#, float#, etc. It is faster than general implementation for any type done in {DArray} because it does not cope with element's constructors, destructors and copy operators. Although implemented as a template, which makes it possible to incorrectly use {TArray} with non-trivial classes, it should not be done. A lot of things is shared by these three arrays. That is why there are more base classes: {itemize} {ArrayBase} defines functions independent of the elements type {ArrayBaseT} template class defining functions shared by {DArray} and {TArray} {itemize} The main difference between {GArray} (now obsolete) and these ones is the copy-on-demand strategy, which allows you to copy array objects without copying the real data. It's the same thing, which has been implemented in {GString} long ago: as long as you don't try to modify the underlying data, it may be shared between several copies of array objects. As soon as you attempt to make any changes, a private copy is created automatically and transparently for you - the procedure, that we call "copy-on-demand". Also, please note that now there is no separate class, which does fast sorting. Both {TArray} (dynamic array for trivial types) and {DArray} (dynamic array for arbitrary types) can sort their elements. { Historical comments} --- Leon chose to implement his own arrays because the STL classes were not universally available and the compilers were rarely able to deal with such a template galore. Later it became clear that there is no really good reason why arrays should be derived from containers. It was also suggested to create separate arrays implementation for simple classes and do the copy-on-demand strategy, which would allow to assign array objects without immediate copying of their elements. At this point {DArray} and {TArray} should only be used when it is critical to have the copy-on-demand feature. The {GArray} implementation is a lot more efficient. Template array classes.
| |
DPArray (const DPArray< TYPE > &gc) | |
DPArray (int lobound, int hibound) | |
DPArray (int hibound) | |
DPArray () | |
void | ins (int n, const GP< TYPE > &val, unsigned int howmany=1) |
operator const GP< TYPE > * () const | |
operator const GP< TYPE > * () | |
operator GP< TYPE > * () | |
DPArray< TYPE > & | operator= (const DPArray &ga) |
const GP< TYPE > & | operator[] (int n) const |
GP< TYPE > & | operator[] (int n) |
virtual | ~DPArray () |
Detailed Description
template<class TYPE>
class DPArray< TYPE >
Dynamic array for {GPBase}d classes.
There are many situations when it's necessary to create arrays of {GP} pointers. For example, DArray<GP<Dialog> ># or DArray<GP<Button> >#. This would result in compilation of two instances of {DArray} because from the viewpoint of the compiler there are two different classes used as array elements: GP<Dialog># and GP<Button>#. In reality though, all {GP} pointers have absolutely the same binary structure because they are derived from {GPBase} class and do not add any variables or virtual functions. That's why it's possible to instantiate {DArray} only once for {GPBase} elements and then just cast types.
To implement this idea we have created this DPArray<TYPE># class, which can be used instead of DArray<GP<TYPE> >#. It behaves absolutely the same way as {DArray} but has one big advantage: overhead of using DPArray# with one more type is negligible.
Definition at line 893 of file Arrays.h.
Constructor & Destructor Documentation
Member Function Documentation
Returns a constant reference to the array element for subscript n#.
This reference can only be used for reading (as "#a[n]#") an array element. This operation will not extend the valid subscript range: an exception {GException} is thrown if argument n# is not in the valid subscript range. This variant of operator[]# is necessary when dealing with a const DArray<TYPE>#.
Reimplemented from ArrayBaseT< GPBase >.
Returns a reference to the array element for subscript n#.
This reference can be used for both reading (as "#a[n]#") and writing (as "#a[n]=v#") an array element. This operation will not extend the valid subscript range: an exception {GException} is thrown if argument n# is not in the valid subscript range.
Reimplemented from ArrayBaseT< GPBase >.
The documentation for this class was generated from the following file: