KDECore
KAccel Class Reference
Handle shortcuts. More...
#include <kaccel.h>
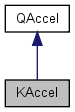
Signals | |
void | keycodeChanged () |
Public Member Functions | |
const KAccelActions & | actions () const |
KAccelActions & | actions () |
void | changeMenuAccel (QPopupMenu *menu, int id, KStdAccel::StdAccel accel) KDE_DEPRECATED |
void | changeMenuAccel (QPopupMenu *menu, int id, const QString &action) KDE_DEPRECATED |
const QString & | configGroup () const |
KDE_DEPRECATED bool | connectItem (KStdAccel::StdAccel accel, const QObject *pObjSlot, const char *psMethodSlot) |
bool | connectItem (const QString &sAction, const QObject *pObjSlot, const char *psMethodSlot, bool bActivate=true) KDE_DEPRECATED |
int | currentKey (const QString &action) const KDE_DEPRECATED |
void | emitKeycodeChanged () |
QString | findKey (int key) const KDE_DEPRECATED |
KAccelAction * | insert (KStdAccel::StdAccel id, const QObject *pObjSlot, const char *psMethodSlot, bool bConfigurable=true, bool bEnabled=true) |
KAccelAction * | insert (const char *psAction, const KShortcut &cutDef, const QObject *pObjSlot, const char *psMethodSlot, bool bConfigurable=true, bool bEnabled=true) |
KAccelAction * | insert (const QString &sAction, const QString &sLabel, const QString &sWhatsThis, const KShortcut &cutDef3, const KShortcut &cutDef4, const QObject *pObjSlot, const char *psMethodSlot, bool bConfigurable=true, bool bEnabled=true) |
KAccelAction * | insert (const QString &sAction, const QString &sLabel, const QString &sWhatsThis, const KShortcut &cutDef, const QObject *pObjSlot, const char *psMethodSlot, bool bConfigurable=true, bool bEnabled=true) |
bool | insertItem (const QString &sLabel, const QString &sAction, int key, int nIDMenu=0, QPopupMenu *pMenu=0, bool bConfigurable=true) KDE_DEPRECATED |
bool | insertItem (const QString &sLabel, const QString &sAction, const char *psKey, int nIDMenu=0, QPopupMenu *pMenu=0, bool bConfigurable=true) KDE_DEPRECATED |
bool | insertStdItem (KStdAccel::StdAccel id, const QString &descr=QString::null) KDE_DEPRECATED |
bool | isEnabled () |
KAccel (QWidget *watch, QObject *parent, const char *psName=0) | |
KAccel (QWidget *pParent, const char *psName=0) | |
bool | readSettings (KConfigBase *pConfig=0) |
bool | remove (const QString &sAction) |
bool | removeItem (const QString &sAction) KDE_DEPRECATED |
bool | setAutoUpdate (bool bAuto) |
void | setConfigGroup (const QString &name) |
bool | setEnabled (const QString &sAction, bool bEnabled) |
void | setEnabled (bool bEnabled) |
bool | setItemEnabled (const QString &sAction, bool bEnable) KDE_DEPRECATED |
bool | setShortcut (const QString &sAction, const KShortcut &shortcut) |
bool | setSlot (const QString &sAction, const QObject *pObjSlot, const char *psMethodSlot) |
const KShortcut & | shortcut (const QString &sAction) const |
bool | updateConnections () |
bool | writeSettings (KConfigBase *pConfig=0) const |
virtual | ~KAccel () |
Static Public Member Functions | |
static int | stringToKey (const QString &) KDE_DEPRECATED |
Protected Member Functions | |
virtual void | virtual_hook (int id, void *data) |
Detailed Description
Handle shortcuts.Allow a user to configure shortcuts through application configuration files or through the KKeyChooser GUI.
A KAccel contains a list of accelerator actions.
For example, CTRL+Key_P could be a shortcut for printing a document. The key codes are listed in qnamespace.h. "Print" could be the action name for printing. The action name identifies the shortcut in configuration files and the KKeyChooser GUI.
A KAccel object handles key events sent to its parent widget and to all children of this parent widget. The most recently created KAccel object has precedence over any KAccel objects created before it. When a shortcut pressed, KAccel calls the slot to which it has been connected. If you want to set global accelerators, independent of the window which has the focus, use KGlobalAccel.
Reconfiguration of a given shortcut can be prevented by specifying that an accelerator item is not configurable when it is inserted. A special group of non-configurable key bindings are known as the standard accelerators.
The standard accelerators appear repeatedly in applications for standard document actions such as printing and saving. A convenience method is available to insert and connect these accelerators which are configurable on a desktop-wide basis.
It is possible for a user to choose to have no key associated with an action.
The translated first argument for insertItem() is used only in the configuration dialog.
KAccel* pAccel = new KAccel( this ); // Insert an action "Scroll Up" which is associated with the "Up" key: pAccel->insert( "Scroll Up", i18n("Scroll up"), i18n("Scroll up the current document by one line."), Qt::Key_Up, this, SLOT(slotScrollUp()) ); // Insert an standard acclerator action. pAccel->insert( KStdAccel::Print, this, SLOT(slotPrint()) ); // Update the shortcuts by read any user-defined settings from the // application's config file. pAccel->readSettings();
Configurable shortcut support for widgets.
- See also:
- KGlobalAccel
KKeyChooser
KKeyDialog
Definition at line 93 of file kaccel.h.
Constructor & Destructor Documentation
KAccel::KAccel | ( | QWidget * | pParent, | |
const char * | psName = 0 | |||
) |
Creates a new KAccel that watches pParent
, which is also the QObject's parent.
- Parameters:
-
pParent the parent and widget to watch for key strokes psName the name of the QObject
Definition at line 414 of file kaccel.cpp.
Creates a new KAccel that watches watch
.
- Parameters:
-
watch the widget to watch for key strokes parent the parent of the QObject psName the name of the QObject
Definition at line 421 of file kaccel.cpp.
KAccel::~KAccel | ( | ) | [virtual] |
Definition at line 430 of file kaccel.cpp.
Member Function Documentation
const KAccelActions & KAccel::actions | ( | ) | const |
For internal use only.
Returns the KAccel's KAccelActions
, a list of KAccelAction
.
- Returns:
- the KAccelActions of the KAccel
Definition at line 437 of file kaccel.cpp.
KAccelActions & KAccel::actions | ( | ) |
For internal use only.
Returns the KAccel's KAccelActions
, a list of KAccelAction
.
- Returns:
- the KAccelActions of the KAccel
Definition at line 436 of file kaccel.cpp.
void KAccel::changeMenuAccel | ( | QPopupMenu * | menu, | |
int | id, | |||
KStdAccel::StdAccel | accel | |||
) |
void KAccel::changeMenuAccel | ( | QPopupMenu * | menu, | |
int | id, | |||
const QString & | action | |||
) |
const QString & KAccel::configGroup | ( | ) | const |
Returns the configuration group of the settings.
- Returns:
- the configuration group
- See also:
- KConfig
Definition at line 521 of file kaccel.cpp.
KDE_DEPRECATED bool KAccel::connectItem | ( | KStdAccel::StdAccel | accel, | |
const QObject * | pObjSlot, | |||
const char * | psMethodSlot | |||
) | [inline] |
int KAccel::currentKey | ( | const QString & | action | ) | const |
Retrieve the key code of the accelerator item with the action name
action
, or zero if either the action name cannot be found or the current key is set to no key.
Definition at line 638 of file kaccel.cpp.
void KAccel::emitKeycodeChanged | ( | ) |
QString KAccel::findKey | ( | int | key | ) | const |
- Deprecated:
- Use actions().actionPtr().
key
, or QString::null if the item cannot be found.
Definition at line 646 of file kaccel.cpp.
KAccelAction * KAccel::insert | ( | KStdAccel::StdAccel | id, | |
const QObject * | pObjSlot, | |||
const char * | psMethodSlot, | |||
bool | bConfigurable = true , |
|||
bool | bEnabled = true | |||
) |
Similar to the first insert() method, but with the action name, short description, help text, and default shortcuts all set according to one of the standard accelerators.
- See also:
- KStdAccel.
Definition at line 474 of file kaccel.cpp.
KAccelAction * KAccel::insert | ( | const char * | psAction, | |
const KShortcut & | cutDef, | |||
const QObject * | pObjSlot, | |||
const char * | psMethodSlot, | |||
bool | bConfigurable = true , |
|||
bool | bEnabled = true | |||
) |
This is an overloaded function provided for convenience.
The advantage of this is when you want to use the same text for the name of the action as for the user-visible label.
Usage:
- Parameters:
-
psAction The name AND label of the action. cutDef The default shortcut for this action. pObjSlot Pointer to the slot object. psMethodSlot Pointer to the slot method. bConfigurable Allow the user to change this shortcut if set to 'true'. bEnabled The action will be activated by the shortcut if set to 'true'.
Definition at line 464 of file kaccel.cpp.
KAccelAction * KAccel::insert | ( | const QString & | sAction, | |
const QString & | sLabel, | |||
const QString & | sWhatsThis, | |||
const KShortcut & | cutDef3, | |||
const KShortcut & | cutDef4, | |||
const QObject * | pObjSlot, | |||
const char * | psMethodSlot, | |||
bool | bConfigurable = true , |
|||
bool | bEnabled = true | |||
) |
Same as first insert(), but with separate shortcuts defined for 3- and 4- modifier defaults.
Definition at line 453 of file kaccel.cpp.
KAccelAction * KAccel::insert | ( | const QString & | sAction, | |
const QString & | sLabel, | |||
const QString & | sWhatsThis, | |||
const KShortcut & | cutDef, | |||
const QObject * | pObjSlot, | |||
const char * | psMethodSlot, | |||
bool | bConfigurable = true , |
|||
bool | bEnabled = true | |||
) |
Create an accelerator action.
Usage:
insert( "Do Something", i18n("Do Something"), i18n("This action allows you to do something really great with this program to " "the currently open document."), ALT+Key_D, this, SLOT(slotDoSomething()) ); *
- Parameters:
-
sAction The internal name of the action. sLabel An i18n'ized short description of the action displayed when using KKeyChooser to reconfigure the shortcuts. sWhatsThis An extended description of the action. cutDef The default shortcut. pObjSlot Pointer to the slot object. psMethodSlot Pointer to the slot method. bConfigurable Allow the user to change this shortcut if set to 'true'. bEnabled The action will be activated by the shortcut if set to 'true'.
Definition at line 442 of file kaccel.cpp.
bool KAccel::insertItem | ( | const QString & | sLabel, | |
const QString & | sAction, | |||
int | key, | |||
int | nIDMenu = 0 , |
|||
QPopupMenu * | pMenu = 0 , |
|||
bool | bConfigurable = true | |||
) |
bool KAccel::insertItem | ( | const QString & | sLabel, | |
const QString & | sAction, | |||
const char * | psKey, | |||
int | nIDMenu = 0 , |
|||
QPopupMenu * | pMenu = 0 , |
|||
bool | bConfigurable = true | |||
) |
bool KAccel::insertStdItem | ( | KStdAccel::StdAccel | id, | |
const QString & | descr = QString::null | |||
) |
bool KAccel::isEnabled | ( | ) |
Checks whether the KAccel is active.
- Returns:
- true if the QAccel is enabled
Definition at line 438 of file kaccel.cpp.
void KAccel::keycodeChanged | ( | ) | [signal] |
Emitted when one of the key codes has changed.
bool KAccel::readSettings | ( | KConfigBase * | pConfig = 0 |
) |
Read all shortcuts from pConfig
, or (if pConfig
is zero) from the application's configuration file KGlobal::config().
The group in which the configuration is stored can be set with setConfigGroup().
- Parameters:
-
pConfig the configuration file, or 0 for the application configuration file
- Returns:
- true if successful, false otherwise
Definition at line 527 of file kaccel.cpp.
Removes the accelerator action identified by the name.
Remember to also call updateConnections().
- Parameters:
-
sAction the name of the action to remove
- Returns:
- true if successful, false otherwise
Definition at line 492 of file kaccel.cpp.
Enable auto-update of connections.
This means that the signals are automatically disconnected when you disable an action, and re-enabled when you enable it. By default auto update is turned on. If you disable auto-update, you need to call updateConnections() after changing actions.
- Parameters:
-
bAuto true to enable, false to disable
- Returns:
- the value of the flag before this call
Definition at line 440 of file kaccel.cpp.
void KAccel::setConfigGroup | ( | const QString & | name | ) |
Returns the configuration group of the settings.
- Parameters:
-
name the new configuration group
- See also:
- KConfig
Definition at line 524 of file kaccel.cpp.
Enable or disable the action named by sAction
.
- Parameters:
-
sAction the action to en-/disable bEnabled true to enable, false to disable
- Returns:
- true if successful, false otherwise
Definition at line 506 of file kaccel.cpp.
void KAccel::setEnabled | ( | bool | bEnabled | ) |
Enables or disables the KAccel.
- Parameters:
-
bEnabled true to enable, false to disable
Reimplemented from QAccel.
Definition at line 439 of file kaccel.cpp.
Set the shortcut to be associated with the action named by sAction
.
- Parameters:
-
sAction the name of the action shortcut the shortcut to set
- Returns:
- true if successful, false otherwise
Definition at line 509 of file kaccel.cpp.
bool KAccel::setSlot | ( | const QString & | sAction, | |
const QObject * | pObjSlot, | |||
const char * | psMethodSlot | |||
) |
Set the slot to be called when the shortcut of the action named by sAction
is pressed.
- Parameters:
-
sAction the name of the action pObjSlot the owner of the slot psMethodSlot the name of the slot
- Returns:
- true if successful, false otherwise
Definition at line 503 of file kaccel.cpp.
Return the shortcut associated with the action named by sAction
.
- Parameters:
-
sAction the name of the action
- Returns:
- the action's shortcut, or a null shortcut if not found
Definition at line 497 of file kaccel.cpp.
int KAccel::stringToKey | ( | const QString & | sKey | ) | [static] |
bool KAccel::updateConnections | ( | ) |
Updates the connections of the accelerations after changing them.
This is only necessary if you have disabled auto updates which are on by default.
- Returns:
- true if successful, false otherwise
- See also:
- setAutoUpdate()
getAutoUpdate()
Definition at line 494 of file kaccel.cpp.
void KAccel::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
bool KAccel::writeSettings | ( | KConfigBase * | pConfig = 0 |
) | const |
Write the current shortcuts to pConfig
, or (if pConfig
is zero) to the application's configuration file.
- Parameters:
-
pConfig the configuration file, or 0 for the application configuration file
- Returns:
- true if successful, false otherwise
Definition at line 533 of file kaccel.cpp.
The documentation for this class was generated from the following files: