KDECore
KConfigBase Class Reference
KDE Configuration Management abstract base class. More...
#include <kconfigbase.h>
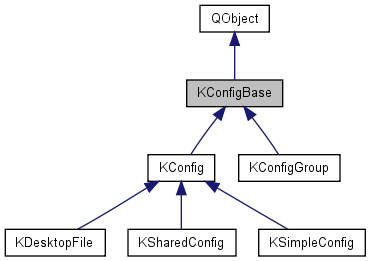
Public Types | |
enum | ConfigState { NoAccess, ReadOnly, ReadWrite } |
Public Member Functions | |
bool | checkConfigFilesWritable (bool warnUser) |
void | deleteEntry (const char *pKey, bool bNLS=false, bool bGlobal=false) |
void | deleteEntry (const QString &pKey, bool bNLS=false, bool bGlobal=false) |
bool | deleteGroup (const QString &group, bool bDeep=true, bool bGlobal=false) |
bool | entryIsImmutable (const QString &key) const |
virtual QMap< QString, QString > | entryMap (const QString &group) const =0 |
ConfigState | getConfigState () const |
QString | group () const |
bool | groupIsImmutable (const QString &group) const |
virtual QStringList | groupList () const =0 |
bool | hasDefault (const QString &key) const |
bool | hasGroup (const char *_pGroup) const |
bool | hasGroup (const QCString &_pGroup) const |
bool | hasGroup (const QString &group) const |
bool | hasKey (const char *pKey) const |
bool | hasKey (const QString &key) const |
bool | isDirty () const |
bool | isDollarExpansion () const |
bool | isImmutable () const |
bool | isReadOnly () const |
KConfigBase () | |
QString | locale () const |
bool | readBoolEntry (const char *pKey, bool bDefault=false) const |
bool | readBoolEntry (const QString &pKey, bool bDefault=false) const |
QColor | readColorEntry (const char *pKey, const QColor *pDefault=0L) const |
QColor | readColorEntry (const QString &pKey, const QColor *pDefault=0L) const |
QDateTime | readDateTimeEntry (const char *pKey, const QDateTime *pDefault=0L) const |
QDateTime | readDateTimeEntry (const QString &pKey, const QDateTime *pDefault=0L) const |
bool | readDefaults () const |
double | readDoubleNumEntry (const char *pKey, double nDefault=0.0) const |
double | readDoubleNumEntry (const QString &pKey, double nDefault=0.0) const |
QString | readEntry (const char *pKey, const QString &aDefault=QString::null) const |
QString | readEntry (const QString &pKey, const QString &aDefault=QString::null) const |
QString | readEntryUntranslated (const char *pKey, const QString &aDefault=QString::null) const |
QString | readEntryUntranslated (const QString &pKey, const QString &aDefault=QString::null) const |
QFont | readFontEntry (const char *pKey, const QFont *pDefault=0L) const |
QFont | readFontEntry (const QString &pKey, const QFont *pDefault=0L) const |
QValueList< int > | readIntListEntry (const char *pKey) const |
QValueList< int > | readIntListEntry (const QString &pKey) const |
QStringList | readListEntry (const char *pKey, const QStringList &aDefault, char sep= ',') const |
QStringList | readListEntry (const char *pKey, char sep= ',') const |
QStringList | readListEntry (const QString &pKey, char sep= ',') const |
int | readListEntry (const char *pKey, QStrList &list, char sep= ',') const |
int | readListEntry (const QString &pKey, QStrList &list, char sep= ',') const |
long | readLongNumEntry (const char *pKey, long nDefault=0) const |
long | readLongNumEntry (const QString &pKey, long nDefault=0) const |
Q_INT64 | readNum64Entry (const char *pKey, Q_INT64 nDefault=0) const |
Q_INT64 | readNum64Entry (const QString &pKey, Q_INT64 nDefault=0) const |
int | readNumEntry (const char *pKey, int nDefault=0) const |
int | readNumEntry (const QString &pKey, int nDefault=0) const |
QString | readPathEntry (const char *pKey, const QString &aDefault=QString::null) const |
QString | readPathEntry (const QString &pKey, const QString &aDefault=QString::null) const |
QStringList | readPathListEntry (const char *pKey, char sep= ',') const |
QStringList | readPathListEntry (const QString &pKey, char sep= ',') const |
QPoint | readPointEntry (const char *pKey, const QPoint *pDefault=0L) const |
QPoint | readPointEntry (const QString &pKey, const QPoint *pDefault=0L) const |
QVariant | readPropertyEntry (const char *pKey, const QVariant &aDefault) const |
QVariant | readPropertyEntry (const QString &pKey, const QVariant &aDefault) const |
QVariant | readPropertyEntry (const char *pKey, QVariant::Type) const |
QVariant | readPropertyEntry (const QString &pKey, QVariant::Type) const |
QRect | readRectEntry (const char *pKey, const QRect *pDefault=0L) const |
QRect | readRectEntry (const QString &pKey, const QRect *pDefault=0L) const |
QSize | readSizeEntry (const char *pKey, const QSize *pDefault=0L) const |
QSize | readSizeEntry (const QString &pKey, const QSize *pDefault=0L) const |
unsigned long | readUnsignedLongNumEntry (const char *pKey, unsigned long nDefault=0) const |
unsigned long | readUnsignedLongNumEntry (const QString &pKey, unsigned long nDefault=0) const |
Q_UINT64 | readUnsignedNum64Entry (const char *pKey, Q_UINT64 nDefault=0) const |
Q_UINT64 | readUnsignedNum64Entry (const QString &pKey, Q_UINT64 nDefault=0) const |
unsigned int | readUnsignedNumEntry (const char *pKey, unsigned int nDefault=0) const |
unsigned int | readUnsignedNumEntry (const QString &pKey, unsigned int nDefault=0) const |
virtual void | reparseConfiguration ()=0 |
void | revertToDefault (const QString &key) |
virtual void | rollback (bool bDeep=true) |
void | setDesktopGroup () |
void | setDollarExpansion (bool _bExpand=true) |
void | setGroup (const char *pGroup) |
void | setGroup (const QCString &pGroup) |
void | setGroup (const QString &group) |
void | setReadDefaults (bool b) |
virtual void | setReadOnly (bool _ro) |
virtual void | sync () |
void | writeEntry (const char *pKey, const QSize &rValue, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const QString &pKey, const QSize &rValue, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const char *pKey, const QPoint &rValue, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const QString &pKey, const QPoint &rValue, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const char *pKey, const QRect &rValue, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const QString &pKey, const QRect &rValue, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const char *pKey, const QDateTime &rDateTime, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const QString &pKey, const QDateTime &rDateTime, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const char *pKey, const QColor &rColor, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const QString &pKey, const QColor &rColor, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const char *pKey, const QFont &rFont, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const QString &pKey, const QFont &rFont, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const char *pKey, bool bValue, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const QString &pKey, bool bValue, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const char *pKey, double nValue, bool bPersistent=true, bool bGlobal=false, char format= 'g', int precision=6, bool bNLS=false) |
void | writeEntry (const QString &pKey, double nValue, bool bPersistent=true, bool bGlobal=false, char format= 'g', int precision=6, bool bNLS=false) |
void | writeEntry (const char *pKey, Q_UINT64 nValue, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const QString &pKey, Q_UINT64 nValue, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const char *pKey, Q_INT64 nValue, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const QString &pKey, Q_INT64 nValue, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const char *pKey, unsigned long nValue, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const QString &pKey, unsigned long nValue, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const char *pKey, long nValue, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const QString &pKey, long nValue, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const char *pKey, unsigned int nValue, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const QString &pKey, unsigned int nValue, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const char *pKey, int nValue, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const QString &pKey, int nValue, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const char *pKey, const char *pValue, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const QString &pKey, const char *pValue, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const char *pKey, const QValueList< int > &rValue, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const QString &pKey, const QValueList< int > &rValue, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const char *pKey, const QStringList &rValue, char sep= ',', bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const QString &pKey, const QStringList &rValue, char sep= ',', bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const char *pKey, const QStrList &rValue, char sep= ',', bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const QString &pKey, const QStrList &rValue, char sep= ',', bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const char *pKey, const QVariant &rValue, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const QString &pKey, const QVariant &rValue, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const char *pKey, const QString &pValue, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writeEntry (const QString &pKey, const QString &pValue, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writePathEntry (const char *pKey, const QStringList &rValue, char sep= ',', bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writePathEntry (const QString &pKey, const QStringList &rValue, char sep= ',', bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writePathEntry (const char *pKey, const QString &path, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
void | writePathEntry (const QString &pKey, const QString &path, bool bPersistent=true, bool bGlobal=false, bool bNLS=false) |
virtual | ~KConfigBase () |
Protected Member Functions | |
virtual KEntryMap | internalEntryMap () const =0 |
virtual KEntryMap | internalEntryMap (const QString &pGroup) const =0 |
virtual bool | internalHasGroup (const QCString &group) const =0 |
virtual KEntry | lookupData (const KEntryKey &_key) const =0 |
virtual void | parseConfigFiles () |
virtual void | putData (const KEntryKey &_key, const KEntry &_data, bool _checkGroup=true)=0 |
QCString | readEntryUtf8 (const char *pKey) const |
virtual void | setDirty (bool _bDirty=true) |
void | setLocale () |
virtual void | virtual_hook (int id, void *data) |
Protected Attributes | |
QCString | aLocaleString |
KConfigBackEnd * | backEnd |
bool | bDirty |
bool | bExpand |
bool | bLocaleInitialized |
bool | bReadOnly |
QCString | mGroup |
Detailed Description
KDE Configuration Management abstract base class.This class forms the base for all KDE configuration. It is an abstract base class, meaning that you cannot directly instantiate objects of this class. Either use KConfig (for usual KDE configuration) or KSimpleConfig (for special needs as in ksamba), or even KSharedConfig (stores values in shared memory).
All configuration entries are key, value pairs. Each entry also belongs to a specific group of related entries. All configuration entries that do not explicitly specify which group they are in are in a special group called the default group.
If there is a $ character in an entry, KConfigBase tries to expand environment variable and uses its value instead of its name. You can avoid this feature by having two consecutive $ characters in your config file which get expanded to one.
- Note:
- the '=' char is not allowed in keys and the ']' char is not allowed in a group name.
Definition at line 70 of file kconfigbase.h.
Member Enumeration Documentation
Possible return values for getConfigState().
- See also:
- getConfigState()
Definition at line 1804 of file kconfigbase.h.
Constructor & Destructor Documentation
KConfigBase::KConfigBase | ( | ) |
KConfigBase::~KConfigBase | ( | ) | [virtual] |
Member Function Documentation
Check whether the config files are writable.
- Parameters:
-
warnUser Warn the user if the configuration files are not writable.
- Returns:
- Indicates that all of the configuration files used are writable.
- Since:
- 3.2
Definition at line 1861 of file kconfigbase.cpp.
Deletes the entry specified by pKey
in the current group.
- Parameters:
-
pKey The key to delete. bGlobal If bGlobal
is true, the pair is not removed from the application specific config file, but from the global KDE config file.bNLS If bNLS
is true, the key with the locale tag is removed.
Definition at line 1170 of file kconfigbase.cpp.
Deletes the entry specified by pKey
in the current group.
- Parameters:
-
pKey The key to delete. bGlobal If bGlobal
is true, the pair is not removed from the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the key with the locale tag is removed.
Definition at line 1163 of file kconfigbase.cpp.
Deletes a configuration entry group.
If the group is not empty and bDeep is false, nothing gets deleted and false is returned. If this group is the current group and it is deleted, the current group is undefined and should be set with setGroup() before the next operation on the configuration object.
- Parameters:
-
group The name of the group bDeep Specify whether non-empty groups should be completely deleted (including their entries). bGlobal If bGlobal
is true, the group is not removed from the application specific config file, but from the global KDE config file.
- Returns:
- If the group is not empty and bDeep is false, deleteGroup returns false.
Definition at line 1196 of file kconfigbase.cpp.
Checks whether it is possible to change the given entry.
- Parameters:
-
key the key to check
- Returns:
- whether the entry
key
may be changed in the current group in this configuration file.
Definition at line 164 of file kconfigbase.cpp.
Returns a map (tree) of entries for all entries in a particular group.
Only the actual entry string is returned, none of the other internal data should be included.
- Parameters:
-
group A group to get keys from.
- Returns:
- A map of entries in the group specified, indexed by key. The returned map may be empty if the group is not found.
- See also:
- QMap
Implemented in KConfig.
KConfigBase::ConfigState KConfigBase::getConfigState | ( | ) | const |
Returns the state of the app-config object.
Possible return values are NoAccess (the application-specific config file could not be opened neither read-write nor read-only), ReadOnly (the application-specific config file is opened read-only, but not read-write) and ReadWrite (the application-specific config file is opened read-write).
- See also:
- ConfigState()
- Returns:
- the state of the app-config object
Definition at line 1706 of file kconfigbase.cpp.
QString KConfigBase::group | ( | ) | const |
Returns the name of the group in which we are searching for keys and from which we are retrieving entries.
- Returns:
- The current group.
Definition at line 100 of file kconfigbase.cpp.
Checks whether it is possible to change the given group.
- Parameters:
-
group the group to check
- Returns:
- whether changes may be made to
group
in this configuration file.
Definition at line 154 of file kconfigbase.cpp.
virtual QStringList KConfigBase::groupList | ( | ) | const [pure virtual] |
Returns whether a default is specified for an entry in either the system wide configuration file or the global KDE config file.
If an application computes a default value at runtime for a certain entry, e.g. like:
QColor computedDefault = kapp->palette().color(QPalette::Active, QColorGroup::Text) QColor color = config->readEntry(key, computedDefault); \encode Then it may wish to make the following check before writing back changes: \code if ( (value == computedDefault) && !config->hasDefault(key) ) config->revertToDefault(key) else config->writeEntry(key, value)
This ensures that as long as the entry is not modified to differ from the computed default, the application will keep using the computed default and will follow changes the computed default makes over time.
- Parameters:
-
key The key of the entry to check.
- Since:
- 3.2
Definition at line 1761 of file kconfigbase.cpp.
bool KConfigBase::hasGroup | ( | const char * | _pGroup | ) | const |
Definition at line 139 of file kconfigbase.cpp.
Definition at line 144 of file kconfigbase.cpp.
Returns true if the specified group is known about.
- Parameters:
-
group The group to search for.
- Returns:
- true if the group exists.
Definition at line 134 of file kconfigbase.cpp.
bool KConfigBase::hasKey | ( | const char * | pKey | ) | const |
Definition at line 114 of file kconfigbase.cpp.
Checks whether the key has an entry in the currently active group.
Use this to determine whether a key is not specified for the current group (hasKey() returns false). Keys with null data are considered nonexistent.
- Parameters:
-
key The key to search for.
- Returns:
- If true, the key is available.
Definition at line 109 of file kconfigbase.cpp.
virtual KEntryMap KConfigBase::internalEntryMap | ( | ) | const [protected, pure virtual] |
Returns a map (tree) of the entries in the tree.
Do not use this function, the implementation / return type are subject to change.
- Returns:
- A map of the entries in the tree.
For internal use only.
Implemented in KConfig.
virtual KEntryMap KConfigBase::internalEntryMap | ( | const QString & | pGroup | ) | const [protected, pure virtual] |
Returns a map (tree) of the entries in the specified group.
This may or may not return all entries that belong to the config object. The only guarantee that you are given is that any entries that are dirty (i.e. modified and not yet written back to the disk) will be contained in the map. Some derivative classes may choose to return everything.
Do not use this function, the implementation / return type are subject to change.
- Parameters:
-
pGroup The group to provide a KEntryMap for.
- Returns:
- The map of the entries in the group.
For internal use only.
Implemented in KConfig.
virtual bool KConfigBase::internalHasGroup | ( | const QCString & | group | ) | const [protected, pure virtual] |
Implemented in KConfig.
bool KConfigBase::isDirty | ( | ) | const [inline] |
Checks whether the config file has any dirty (modified) entries.
- Returns:
- true if the config file has any dirty (modified) entries.
Definition at line 1722 of file kconfigbase.h.
bool KConfigBase::isDollarExpansion | ( | ) | const [inline] |
Returns whether dollar expansion is on or off.
It is initially OFF.
- Returns:
- true if dollar expansion is on.
Definition at line 1685 of file kconfigbase.h.
bool KConfigBase::isImmutable | ( | ) | const |
Checks whether this configuration file can be modified.
- Returns:
- whether changes may be made to this configuration file.
Definition at line 149 of file kconfigbase.cpp.
bool KConfigBase::isReadOnly | ( | ) | const [inline] |
Returns the read-only status of the config object.
- Returns:
- The read-only status.
Definition at line 1738 of file kconfigbase.h.
QString KConfigBase::locale | ( | ) | const |
Returns a the current locale.
- Returns:
- A string representing the current locale.
Definition at line 74 of file kconfigbase.cpp.
Looks up an entry in the config object's internal structure.
Classes that derive from KConfigBase will need to implement this method in a storage-specific manner.
Do not use this function, the implementation and return type are subject to change.
- Parameters:
-
_key The key to look up It contains information both on the group of the key and the entry's key itself.
- Returns:
- The KEntry value (data) found for the key.
KEntry.aValue
will be the null string if nothing was located.
For internal use only.
Implemented in KConfig, and KConfigGroup.
void KConfigBase::parseConfigFiles | ( | ) | [protected, virtual] |
Parses all configuration files for a configuration object.
The actual parsing is done by the associated KConfigBackEnd.
Definition at line 1683 of file kconfigbase.cpp.
virtual void KConfigBase::putData | ( | const KEntryKey & | _key, | |
const KEntry & | _data, | |||
bool | _checkGroup = true | |||
) | [protected, pure virtual] |
Inserts a (key/value) pair into the internal storage mechanism of the configuration object.
Classes that derive from KConfigBase will need to implement this method in a storage-specific manner.
Do not use this function, the implementation / return type are subject to change.
- Parameters:
-
_key The key to insert. It contains information both on the group of the key and the key itself. If the key already exists, the old value will be replaced. _data the KEntry that is to be stored. _checkGroup When false, assume that the group already exists.
For internal use only.
Implemented in KConfig, and KConfigGroup.
Reads a boolean entry.
Read the value of an entry specified by pKey
in the current group and interpret it as a boolean value. Currently "on", "yes", "1" and "true" are accepted as true, everything else if false.
- Parameters:
-
pKey The key to search for bDefault A default value returned if the key was not found.
- Returns:
- The value for this key.
Definition at line 721 of file kconfigbase.cpp.
Reads a boolean entry.
Read the value of an entry specified by pKey
in the current group and interpret it as a boolean value. Currently "on", "yes", "1" and "true" are accepted as true, everything else if false.
- Parameters:
-
pKey The key to search for bDefault A default value returned if the key was not found.
- Returns:
- The value for this key.
Definition at line 716 of file kconfigbase.cpp.
Reads a QColor entry.
Read the value of an entry specified by pKey
in the current group and interpret it as a color.
- Parameters:
-
pKey The key to search for. pDefault A default value (null QColor by default) returned if the key was not found or if the value cannot be interpreted.
- Returns:
- The value for this key.
Definition at line 921 of file kconfigbase.cpp.
Reads a QColor entry.
Read the value of an entry specified by pKey
in the current group and interpret it as a color.
- Parameters:
-
pKey The key to search for. pDefault A default value (null QColor by default) returned if the key was not found or if the value cannot be interpreted.
- Returns:
- The value for this key.
Definition at line 915 of file kconfigbase.cpp.
QDateTime KConfigBase::readDateTimeEntry | ( | const char * | pKey, | |
const QDateTime * | pDefault = 0L | |||
) | const |
Reads a QDateTime entry.
Read the value of an entry specified by pKey
in the current group and interpret it as a date and time.
- Parameters:
-
pKey The key to search for. pDefault A default value ( currentDateTime() by default) returned if the key was not found or if the read value cannot be interpreted.
- Returns:
- The value for this key.
Definition at line 987 of file kconfigbase.cpp.
QDateTime KConfigBase::readDateTimeEntry | ( | const QString & | pKey, | |
const QDateTime * | pDefault = 0L | |||
) | const |
Reads a QDateTime entry.
Read the value of an entry specified by pKey
in the current group and interpret it as a date and time.
- Parameters:
-
pKey The key to search for. pDefault A default value ( currentDateTime() by default) returned if the key was not found or if the read value cannot be interpreted.
- Returns:
- The value for this key.
Definition at line 980 of file kconfigbase.cpp.
bool KConfigBase::readDefaults | ( | ) | const |
- Returns:
- true if all readEntry and readXXXEntry calls return the system wide (default) values instead of the user's preference.
- Since:
- 3.2
Definition at line 1729 of file kconfigbase.cpp.
Reads a floating point value.
Read the value of an entry specified by pKey
in the current group and interpret it numerically.
- Parameters:
-
pKey The key to search for. nDefault A default value returned if the key was not found or if the read value cannot be interpreted.
- Returns:
- The value for this key.
Definition at line 702 of file kconfigbase.cpp.
Reads a floating point value.
Read the value of an entry specified by pKey
in the current group and interpret it numerically.
- Parameters:
-
pKey The key to search for. nDefault A default value returned if the key was not found or if the read value cannot be interpreted.
- Returns:
- The value for this key.
Definition at line 697 of file kconfigbase.cpp.
QString KConfigBase::readEntry | ( | const char * | pKey, | |
const QString & | aDefault = QString::null | |||
) | const |
Reads the value of an entry specified by pKey
in the current group.
- Parameters:
-
pKey The key to search for. aDefault A default value returned if the key was not found.
- Returns:
- The value for this key. Can be QString::null if aDefault is null.
Definition at line 209 of file kconfigbase.cpp.
QString KConfigBase::readEntry | ( | const QString & | pKey, | |
const QString & | aDefault = QString::null | |||
) | const |
Reads the value of an entry specified by pKey
in the current group.
If you want to read a path, please use readPathEntry().
- Parameters:
-
pKey The key to search for. aDefault A default value returned if the key was not found.
- Returns:
- The value for this key. Can be QString::null if aDefault is null.
Definition at line 203 of file kconfigbase.cpp.
QString KConfigBase::readEntryUntranslated | ( | const char * | pKey, | |
const QString & | aDefault = QString::null | |||
) | const |
Reads the value of an entry specified by pKey
in the current group.
The untranslated entry is returned, you normally do not need this.
- Parameters:
-
pKey The key to search for. aDefault A default value returned if the key was not found.
- Returns:
- The value for this key.
Definition at line 193 of file kconfigbase.cpp.
QString KConfigBase::readEntryUntranslated | ( | const QString & | pKey, | |
const QString & | aDefault = QString::null | |||
) | const |
Reads the value of an entry specified by pKey
in the current group.
The untranslated entry is returned, you normally do not need this.
- Parameters:
-
pKey The key to search for. aDefault A default value returned if the key was not found.
- Returns:
- The value for this key.
Definition at line 186 of file kconfigbase.cpp.
QCString KConfigBase::readEntryUtf8 | ( | const char * | pKey | ) | const [protected] |
Definition at line 320 of file kconfigbase.cpp.
Reads a QFont value.
Read the value of an entry specified by pKey
in the current group and interpret it as a font object.
- Parameters:
-
pKey The key to search for. pDefault A default value (null QFont by default) returned if the key was not found or if the read value cannot be interpreted.
- Returns:
- The value for this key.
Definition at line 748 of file kconfigbase.cpp.
Reads a QFont value.
Read the value of an entry specified by pKey
in the current group and interpret it as a font object.
- Parameters:
-
pKey The key to search for. pDefault A default value (null QFont by default) returned if the key was not found or if the read value cannot be interpreted.
- Returns:
- The value for this key.
Definition at line 743 of file kconfigbase.cpp.
QValueList< int > KConfigBase::readIntListEntry | ( | const char * | pKey | ) | const |
Reads a list of Integers.
- Parameters:
-
pKey The key to search for.
- Returns:
- The list. Empty if the entry does not exist.
Definition at line 540 of file kconfigbase.cpp.
QValueList< int > KConfigBase::readIntListEntry | ( | const QString & | pKey | ) | const |
Reads a list of Integers.
- Parameters:
-
pKey The key to search for.
- Returns:
- The list. Empty if the entry does not exist.
Definition at line 535 of file kconfigbase.cpp.
QStringList KConfigBase::readListEntry | ( | const char * | pKey, | |
const QStringList & | aDefault, | |||
char | sep = ',' | |||
) | const |
Reads a list of strings, but returns a default if the key did not exist.
- Parameters:
-
pKey The key to search for. aDefault The default value to use if the key does not exist. sep The list separator (default is ",").
- Returns:
- The list. Contains
aDefault
if the Key does not exist.
- Since:
- 3.3
Definition at line 526 of file kconfigbase.cpp.
QStringList KConfigBase::readListEntry | ( | const char * | pKey, | |
char | sep = ',' | |||
) | const |
Reads a list of strings.
- Parameters:
-
pKey The key to search for. sep The list separator (default is ",").
- Returns:
- The list. Empty if the entry does not exist.
Definition at line 485 of file kconfigbase.cpp.
QStringList KConfigBase::readListEntry | ( | const QString & | pKey, | |
char | sep = ',' | |||
) | const |
Reads a list of strings.
- Parameters:
-
pKey The key to search for. sep The list separator (default is ",").
- Returns:
- The list. Empty if the entry does not exist.
Definition at line 480 of file kconfigbase.cpp.
int KConfigBase::readListEntry | ( | const char * | pKey, | |
QStrList & | list, | |||
char | sep = ',' | |||
) | const |
Reads a list of strings.
- Parameters:
-
pKey The key to search for list In this object, the read list will be returned. sep The list separator (default ",")
- Returns:
- The number of entries in the list.
Definition at line 441 of file kconfigbase.cpp.
Reads a list of strings.
- Parameters:
-
pKey The key to search for list In this object, the read list will be returned. sep The list separator (default ",")
- Returns:
- The number of entries in the list.
Definition at line 435 of file kconfigbase.cpp.
Reads a numerical value.
Read the value of an entry specified by pKey
in the current group and interpret it numerically.
- Parameters:
-
pKey The key to search for. nDefault A default value returned if the key was not found or if the read value cannot be interpreted.
- Returns:
- The value for this key.
Definition at line 626 of file kconfigbase.cpp.
Reads a numerical value.
Read the value of an entry specified by pKey
in the current group and interpret it numerically.
- Parameters:
-
pKey The key to search for. nDefault A default value returned if the key was not found or if the read value cannot be interpreted.
- Returns:
- The value for this key.
Definition at line 621 of file kconfigbase.cpp.
Reads a 64-bit numerical value.
Read the value of an entry specified by pKey
in the current group and interpret it numerically.
- Parameters:
-
pKey The key to search for. nDefault A default value returned if the key was not found or if the read value cannot be interpreted.
- Returns:
- The value for this key.
Definition at line 663 of file kconfigbase.cpp.
Reads a 64-bit numerical value.
Read the value of an entry specified by pKey
in the current group and interpret it numerically.
- Parameters:
-
pKey The key to search for. nDefault A default value returned if the key was not found or if the read value cannot be interpreted.
- Returns:
- The value for this key.
Definition at line 658 of file kconfigbase.cpp.
int KConfigBase::readNumEntry | ( | const char * | pKey, | |
int | nDefault = 0 | |||
) | const |
Reads a numerical value.
Read the value of an entry specified by pKey
in the current group and interpret it numerically.
- Parameters:
-
pKey The key to search for. nDefault A default value returned if the key was not found or if the read value cannot be interpreted.
- Returns:
- The value for this key.
Definition at line 586 of file kconfigbase.cpp.
int KConfigBase::readNumEntry | ( | const QString & | pKey, | |
int | nDefault = 0 | |||
) | const |
Reads a numerical value.
Read the value of an entry specified by pKey
in the current group and interpret it numerically.
- Parameters:
-
pKey The key to search for. nDefault A default value returned if the key was not found or if the read value cannot be interpreted.
- Returns:
- The value for this key.
Definition at line 581 of file kconfigbase.cpp.
QString KConfigBase::readPathEntry | ( | const char * | pKey, | |
const QString & | aDefault = QString::null | |||
) | const |
Reads a path.
Read the value of an entry specified by pKey
in the current group and interpret it as a path. This means, dollar expansion is activated for this value, so that e.g. $HOME gets expanded.
- Parameters:
-
pKey The key to search for. aDefault A default value returned if the key was not found.
- Returns:
- The value for this key. Can be QString::null if aDefault is null.
Definition at line 558 of file kconfigbase.cpp.
QString KConfigBase::readPathEntry | ( | const QString & | pKey, | |
const QString & | aDefault = QString::null | |||
) | const |
Reads a path.
Read the value of an entry specified by pKey
in the current group and interpret it as a path. This means, dollar expansion is activated for this value, so that e.g. $HOME gets expanded.
- Parameters:
-
pKey The key to search for. aDefault A default value returned if the key was not found.
- Returns:
- The value for this key. Can be QString::null if aDefault is null.
Definition at line 553 of file kconfigbase.cpp.
QStringList KConfigBase::readPathListEntry | ( | const char * | pKey, | |
char | sep = ',' | |||
) | const |
Reads a list of string paths.
Read the value of an entry specified by pKey
in the current group and interpret it as a list of paths. This means, dollar expansion is activated for this value, so that e.g. $HOME gets expanded.
- Parameters:
-
pKey The key to search for. sep The list separator (default is ",").
- Returns:
- The list. Empty if the entry does not exist.
- Since:
- 3.1.3
Definition at line 572 of file kconfigbase.cpp.
QStringList KConfigBase::readPathListEntry | ( | const QString & | pKey, | |
char | sep = ',' | |||
) | const |
Reads a list of string paths.
Read the value of an entry specified by pKey
in the current group and interpret it as a list of paths. This means, dollar expansion is activated for this value, so that e.g. $HOME gets expanded.
- Parameters:
-
pKey The key to search for. sep The list separator (default is ",").
- Returns:
- The list. Empty if the entry does not exist.
- Since:
- 3.1.3
Definition at line 567 of file kconfigbase.cpp.
Reads a QPoint entry.
Read the value of an entry specified by pKey
in the current group and interpret it as a QPoint object.
- Parameters:
-
pKey The key to search for pDefault A default value (null QPoint by default) returned if the key was not found or if the read value cannot be interpreted.
- Returns:
- The value for this key.
Definition at line 870 of file kconfigbase.cpp.
Reads a QPoint entry.
Read the value of an entry specified by pKey
in the current group and interpret it as a QPoint object.
- Parameters:
-
pKey The key to search for pDefault A default value (null QPoint by default) returned if the key was not found or if the read value cannot be interpreted.
- Returns:
- The value for this key.
Definition at line 864 of file kconfigbase.cpp.
Reads the value of an entry specified by pKey
in the current group.
The value is treated as if it is of the type of the given default value.
Note that only the following QVariant types are allowed : String, StringList, List, Font, Point, Rect, Size, Color, Int, UInt, Bool, Double, DateTime and Date.
- Parameters:
-
pKey The key to search for. aDefault A default value returned if the key was not found or if the read value cannot be converted to the QVariant::Type.
- Returns:
- The value for the key or the default value if the key was not found.
Definition at line 356 of file kconfigbase.cpp.
Reads the value of an entry specified by pKey
in the current group.
The value is treated as if it is of the type of the given default value.
Note that only the following QVariant types are allowed : String, StringList, List, Font, Point, Rect, Size, Color, Int, UInt, Bool, Double, DateTime and Date.
- Parameters:
-
pKey The key to search for. aDefault A default value returned if the key was not found or if the read value cannot be converted to the QVariant::Type.
- Returns:
- The value for the key or the default value if the key was not found.
Definition at line 350 of file kconfigbase.cpp.
QVariant KConfigBase::readPropertyEntry | ( | const char * | pKey, | |
QVariant::Type | type | |||
) | const |
Reads the value of an entry specified by pKey
in the current group.
The value is treated as if it is of the given type.
Note that only the following QVariant types are allowed : String, StringList, List, Font, Point, Rect, Size, Color, Int, UInt, Bool, Double, DateTime and Date.
- Parameters:
-
pKey The key to search for.
- Returns:
- An invalid QVariant if the key was not found or if the read value cannot be converted to the given QVariant::Type.
Definition at line 341 of file kconfigbase.cpp.
Reads the value of an entry specified by pKey
in the current group.
The value is treated as if it is of the given type.
Note that only the following QVariant types are allowed : String, StringList, List, Font, Point, Rect, Size, Color, Int, UInt, Bool, Double, DateTime and Date.
- Parameters:
-
pKey The key to search for.
- Returns:
- An invalid QVariant if the key was not found or if the read value cannot be converted to the given QVariant::Type.
Definition at line 335 of file kconfigbase.cpp.
Reads a QRect entry.
Read the value of an entry specified by pKey in the current group and interpret it as a QRect object.
- Parameters:
-
pKey The key to search for pDefault A default value (null QRect by default) returned if the key was not found or if the read value cannot be interpreted.
- Returns:
- The value for this key.
Definition at line 845 of file kconfigbase.cpp.
Reads a QRect entry.
Read the value of an entry specified by pKey in the current group and interpret it as a QRect object.
- Parameters:
-
pKey The key to search for pDefault A default value (null QRect by default) returned if the key was not found or if the read value cannot be interpreted.
- Returns:
- The value for this key.
Definition at line 840 of file kconfigbase.cpp.
Reads a QSize entry.
Read the value of an entry specified by pKey
in the current group and interpret it as a QSize object.
- Parameters:
-
pKey The key to search for pDefault A default value (null QSize by default) returned if the key was not found or if the read value cannot be interpreted.
- Returns:
- The value for this key.
Definition at line 895 of file kconfigbase.cpp.
Reads a QSize entry.
Read the value of an entry specified by pKey
in the current group and interpret it as a QSize object.
- Parameters:
-
pKey The key to search for pDefault A default value (null QSize by default) returned if the key was not found or if the read value cannot be interpreted.
- Returns:
- The value for this key.
Definition at line 889 of file kconfigbase.cpp.
unsigned long KConfigBase::readUnsignedLongNumEntry | ( | const char * | pKey, | |
unsigned long | nDefault = 0 | |||
) | const |
Read an unsigned numerical value.
Read the value of an entry specified by pKey
in the current group and interpret it numerically.
- Parameters:
-
pKey The key to search for. nDefault A default value returned if the key was not found or if the read value cannot be interpreted.
- Returns:
- The value for this key.
Definition at line 645 of file kconfigbase.cpp.
unsigned long KConfigBase::readUnsignedLongNumEntry | ( | const QString & | pKey, | |
unsigned long | nDefault = 0 | |||
) | const |
Read an unsigned numerical value.
Read the value of an entry specified by pKey
in the current group and interpret it numerically.
- Parameters:
-
pKey The key to search for. nDefault A default value returned if the key was not found or if the read value cannot be interpreted.
- Returns:
- The value for this key.
Definition at line 640 of file kconfigbase.cpp.
Read an 64-bit unsigned numerical value.
Read the value of an entry specified by pKey
in the current group and interpret it numerically.
- Parameters:
-
pKey The key to search for. nDefault A default value returned if the key was not found or if the read value cannot be interpreted.
- Returns:
- The value for this key.
Definition at line 683 of file kconfigbase.cpp.
Read an 64-bit unsigned numerical value.
Read the value of an entry specified by pKey
in the current group and interpret it numerically.
- Parameters:
-
pKey The key to search for. nDefault A default value returned if the key was not found or if the read value cannot be interpreted.
- Returns:
- The value for this key.
Definition at line 678 of file kconfigbase.cpp.
unsigned int KConfigBase::readUnsignedNumEntry | ( | const char * | pKey, | |
unsigned int | nDefault = 0 | |||
) | const |
Reads an unsigned numerical value.
Read the value of an entry specified by pKey
in the current group and interpret it numerically.
- Parameters:
-
pKey The key to search for. nDefault A default value returned if the key was not found or if the read value cannot be interpreted.
- Returns:
- The value for this key.
Definition at line 607 of file kconfigbase.cpp.
unsigned int KConfigBase::readUnsignedNumEntry | ( | const QString & | pKey, | |
unsigned int | nDefault = 0 | |||
) | const |
Reads an unsigned numerical value.
Read the value of an entry specified by pKey
in the current group and interpret it numerically.
- Parameters:
-
pKey The key to search for. nDefault A default value returned if the key was not found or if the read value cannot be interpreted.
- Returns:
- The value for this key.
Definition at line 602 of file kconfigbase.cpp.
virtual void KConfigBase::reparseConfiguration | ( | ) | [pure virtual] |
Reparses all configuration files.
This is useful for programs that use stand alone graphical configuration tools. The base method implemented here only clears the group list and then appends the default group.
Derivative classes should clear any internal data structures and then simply call parseConfigFiles() when implementing this method.
- See also:
- parseConfigFiles()
Implemented in KConfig.
void KConfigBase::revertToDefault | ( | const QString & | key | ) |
Reverts the entry with key key
in the current group in the application specific config file to either the system wide (default) value or the value specified in the global KDE config file.
To revert entries in the global KDE config file, the global KDE config file should be opened explicitly in a separate config object.
- Parameters:
-
key The key of the entry to revert.
- Since:
- 3.2
Definition at line 1734 of file kconfigbase.cpp.
void KConfigBase::rollback | ( | bool | bDeep = true |
) | [virtual] |
Mark the config object as "clean," i.e.
don't write dirty entries at destruction time. If bDeep
is false, only the global dirty flag of the KConfig object gets cleared. If you then call writeEntry() again, the global dirty flag is set again and all dirty entries will be written at a subsequent sync() call.
Classes that derive from KConfigBase should override this method and implement storage-specific behavior, as well as calling the KConfigBase::rollback() explicitly in the initializer.
- Parameters:
-
bDeep If true, the dirty flags of all entries are cleared, as well as the global dirty flag.
Reimplemented in KConfig.
Definition at line 1712 of file kconfigbase.cpp.
void KConfigBase::setDesktopGroup | ( | ) |
Sets the group to the "Desktop Entry" group used for desktop configuration files for applications, mime types, etc.
Definition at line 104 of file kconfigbase.cpp.
virtual void KConfigBase::setDirty | ( | bool | _bDirty = true |
) | [inline, protected, virtual] |
Sets the global dirty flag of the config object.
- Parameters:
-
_bDirty How to mark the object's dirty status
Reimplemented in KConfigGroup.
Definition at line 1898 of file kconfigbase.h.
void KConfigBase::setDollarExpansion | ( | bool | _bExpand = true |
) | [inline] |
Turns on or off "dollar expansion" (see KConfigBase introduction) when reading config entries.
Dollar sign expansion is initially OFF.
- Parameters:
-
_bExpand Tf true, dollar expansion is turned on.
Definition at line 1678 of file kconfigbase.h.
void KConfigBase::setGroup | ( | const char * | pGroup | ) |
Definition at line 87 of file kconfigbase.cpp.
void KConfigBase::setGroup | ( | const QCString & | pGroup | ) |
void KConfigBase::setGroup | ( | const QString & | group | ) |
Specifies the group in which keys will be read and written.
Subsequent calls to readEntry() and writeEntry() will be applied only in the activated group.
Switch back to the default group by passing a null string.
- Parameters:
-
group The name of the new group.
Definition at line 79 of file kconfigbase.cpp.
void KConfigBase::setLocale | ( | ) | [protected] |
Reads the locale and put in the configuration data struct.
Note that this should be done in the constructor, but this is not possible due to some mutual dependencies in KApplication::init()
Definition at line 62 of file kconfigbase.cpp.
void KConfigBase::setReadDefaults | ( | bool | b | ) |
When set, all readEntry and readXXXEntry calls return the system wide (default) values instead of the user's preference.
This is off by default.
- Since:
- 3.2
Definition at line 1718 of file kconfigbase.cpp.
virtual void KConfigBase::setReadOnly | ( | bool | _ro | ) | [inline, virtual] |
Sets the config object's read-only status.
- Parameters:
-
_ro If true, the config object will not write out any changes to disk even if it is destroyed or sync() is called.
Definition at line 1731 of file kconfigbase.h.
void KConfigBase::sync | ( | ) | [virtual] |
Flushes all changes that currently reside only in memory back to disk / permanent storage.
Dirty configuration entries are written to the most specific file available.
Asks the back end to flush out all pending writes, and then calls rollback(). No changes are made if the object has readOnly
status.
You should call this from your destructor in derivative classes.
- See also:
- rollback(), isReadOnly()
Reimplemented in KConfigGroup, and KSimpleConfig.
Definition at line 1695 of file kconfigbase.cpp.
void KConfigBase::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Reimplemented in KConfig, KConfigGroup, KDesktopFile, and KSimpleConfig.
Definition at line 1855 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const char * | pKey, | |
const QSize & | rValue, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
Writes a (key/value) pair.
Same as above, but writes a size.
Note: Unlike the other writeEntry() functions, the old value is _not_ returned here!
- Parameters:
-
pKey The key to write. rValue The size value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1621 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const QString & | pKey, | |
const QSize & | rValue, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
Writes a (key/value) pair.
Same as above, but writes a size.
Note: Unlike the other writeEntry() functions, the old value is _not_ returned here!
- Parameters:
-
pKey The key to write. rValue The size value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1614 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const char * | pKey, | |
const QPoint & | rValue, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
Writes a (key/value) pair.
Same as above, but writes a point.
Note: Unlike the other writeEntry() functions, the old value is _not_ returned here!
- Parameters:
-
pKey The key to write. rValue The point value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1601 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const QString & | pKey, | |
const QPoint & | rValue, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
Writes a (key/value) pair.
Same as above, but writes a point.
Note: Unlike the other writeEntry() functions, the old value is _not_ returned here!
- Parameters:
-
pKey The key to write. rValue The point value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1594 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const char * | pKey, | |
const QRect & | rValue, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
Writes a (key/value) pair.
Same as above, but writes a rectangle.
Note: Unlike the other writeEntry() functions, the old value is _not_ returned here!
- Parameters:
-
pKey The key to write. rValue The rectangle value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1579 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const QString & | pKey, | |
const QRect & | rValue, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
Writes a (key/value) pair.
Same as above, but writes a rectangle.
Note: Unlike the other writeEntry() functions, the old value is _not_ returned here!
- Parameters:
-
pKey The key to write. rValue The rectangle value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1572 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const char * | pKey, | |
const QDateTime & | rDateTime, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
Writes a (key/value) pair.
Same as above, but writes a date and time entry.
Note: Unlike the other writeEntry() functions, the old value is not returned here!
- Parameters:
-
pKey The key to write. rDateTime The date and time value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1662 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const QString & | pKey, | |
const QDateTime & | rDateTime, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
Writes a (key/value) pair.
Same as above, but writes a date and time entry.
Note: Unlike the other writeEntry() functions, the old value is not returned here!
- Parameters:
-
pKey The key to write. rDateTime The date and time value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1655 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const char * | pKey, | |
const QColor & | rColor, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
Writes a (key/value) pair.
Same as above, but write a color entry.
Note: Unlike the other writeEntry() functions, the old value is _not_ returned here!
- Parameters:
-
pKey The key to write. rColor The color value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1641 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const QString & | pKey, | |
const QColor & | rColor, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
Writes a (key/value) pair.
Same as above, but write a color entry.
Note: Unlike the other writeEntry() functions, the old value is _not_ returned here!
- Parameters:
-
pKey The key to write. rColor The color value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1633 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const char * | pKey, | |
const QFont & | rFont, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
Writes a (key/value) pair.
Same as above, but writes a font value.
- Parameters:
-
pKey The key to write. rFont The font value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1564 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const QString & | pKey, | |
const QFont & | rFont, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
Writes a (key/value) pair.
Same as above, but writes a font value.
- Parameters:
-
pKey The key to write. rFont The font value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1557 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const char * | pKey, | |
bool | bValue, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
Writes a (key/value) pair.
Same as above, but writes a boolean value.
- Parameters:
-
pKey The key to write. bValue The value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1541 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const QString & | pKey, | |
bool | bValue, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
Writes a (key/value) pair.
Same as above, but writes a boolean value.
- Parameters:
-
pKey The key to write. bValue The value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1533 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const char * | pKey, | |
double | nValue, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
char | format = 'g' , |
|||
int | precision = 6 , |
|||
bool | bNLS = false | |||
) |
Writes a (key/value) pair.
Same as above, but writes a floating-point value.
- Parameters:
-
pKey The key to write. nValue The value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.format format
determines the format to which the value is converted. Default is 'g'.precision precision
sets the precision with which the value is converted. Default is 6 as in QString.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1523 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const QString & | pKey, | |
double | nValue, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
char | format = 'g' , |
|||
int | precision = 6 , |
|||
bool | bNLS = false | |||
) |
Writes a (key/value) pair.
Same as above, but writes a floating-point value.
- Parameters:
-
pKey The key to write. nValue The value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.format format
determines the format to which the value is converted. Default is 'g'.precision precision
sets the precision with which the value is converted. Default is 6 as in QString.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1514 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const char * | pKey, | |
Q_UINT64 | nValue, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
Writes a (key/value) pair.
Same as above, but writes an unsigned 64-bit numerical value.
- Parameters:
-
pKey The key to write. nValue The value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1507 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const QString & | pKey, | |
Q_UINT64 | nValue, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
Writes a (key/value) pair.
Same as above, but writes an unsigned 64-bit numerical value.
- Parameters:
-
pKey The key to write. nValue The value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1500 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const char * | pKey, | |
Q_INT64 | nValue, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
Writes a (key/value) pair.
Same as above, but write a long numerical value.
- Parameters:
-
pKey The key to write. nValue The value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1492 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const QString & | pKey, | |
Q_INT64 | nValue, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
Writes a (key/value) pair.
Same as above, but write a 64-bit numerical value.
- Parameters:
-
pKey The key to write. nValue The value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1485 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const char * | pKey, | |
unsigned long | nValue, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
Writes a (key/value) pair.
Same as above, but writes an unsigned long numerical value.
- Parameters:
-
pKey The key to write. nValue The value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1478 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const QString & | pKey, | |
unsigned long | nValue, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
Writes a (key/value) pair.
Same as above, but writes an unsigned long numerical value.
- Parameters:
-
pKey The key to write. nValue The value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1471 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const char * | pKey, | |
long | nValue, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
Writes a (key/value) pair.
Same as above, but write a long numerical value.
- Parameters:
-
pKey The key to write. nValue The value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1463 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const QString & | pKey, | |
long | nValue, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
Writes a (key/value) pair.
Same as above, but write a long numerical value.
- Parameters:
-
pKey The key to write. nValue The value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1456 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const char * | pKey, | |
unsigned int | nValue, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
Writes a (key/value) pair.
Same as above, but writes an unsigned numerical value.
- Parameters:
-
pKey The key to write. nValue The value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1448 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const QString & | pKey, | |
unsigned int | nValue, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
Writes a (key/value) pair.
Same as above, but writes an unsigned numerical value.
- Parameters:
-
pKey The key to write. nValue The value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1441 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const char * | pKey, | |
int | nValue, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
Write a (key/value) pair.
Same as above, but writes a numerical value.
- Parameters:
-
pKey The key to write. nValue The value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1433 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const QString & | pKey, | |
int | nValue, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
Write a (key/value) pair.
Same as above, but writes a numerical value.
- Parameters:
-
pKey The key to write. nValue The value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1426 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const char * | pKey, | |
const char * | pValue, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) | [inline] |
Write a (key/value) pair.
This is stored to the most specific config file when destroying the config object or when calling sync().
- Parameters:
-
pKey The key to write. pValue The value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 999 of file kconfigbase.h.
void KConfigBase::writeEntry | ( | const QString & | pKey, | |
const char * | pValue, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) | [inline] |
Write a (key/value) pair.
This is stored to the most specific config file when destroying the config object or when calling sync().
- Parameters:
-
pKey The key to write. pValue The value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 978 of file kconfigbase.h.
void KConfigBase::writeEntry | ( | const char * | pKey, | |
const QValueList< int > & | rValue, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
writeEntry() overridden to accept a list of Integers.
Note: Unlike the other writeEntry() functions, the old value is _not_ returned here!
- Parameters:
-
pKey The key to write rValue The list to write bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
- See also:
- writeEntry()
Definition at line 1416 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const QString & | pKey, | |
const QValueList< int > & | rValue, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
writeEntry() overridden to accept a list of Integers.
Note: Unlike the other writeEntry() functions, the old value is _not_ returned here!
- Parameters:
-
pKey The key to write rValue The list to write bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
- See also:
- writeEntry()
Definition at line 1410 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const char * | pKey, | |
const QStringList & | rValue, | |||
char | sep = ',' , |
|||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
writeEntry() overridden to accept a list of strings.
Note: Unlike the other writeEntry() functions, the old value is _not_ returned here!
- Parameters:
-
pKey The key to write rValue The list to write sep The list separator (default is ","). bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
- See also:
- writeEntry()
Definition at line 1373 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const QString & | pKey, | |
const QStringList & | rValue, | |||
char | sep = ',' , |
|||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
writeEntry() overridden to accept a list of strings.
Note: Unlike the other writeEntry() functions, the old value is _not_ returned here!
- Parameters:
-
pKey The key to write rValue The list to write sep The list separator (default is ","). bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
- See also:
- writeEntry()
Definition at line 1366 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const char * | pKey, | |
const QStrList & | rValue, | |||
char | sep = ',' , |
|||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
writeEntry() overridden to accept a list of strings.
Note: Unlike the other writeEntry() functions, the old value is _not_ returned here!
- Parameters:
-
pKey The key to write rValue The list to write sep The list separator (default is ","). bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
- See also:
- writeEntry()
Definition at line 1333 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const QString & | pKey, | |
const QStrList & | rValue, | |||
char | sep = ',' , |
|||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
writeEntry() overridden to accept a list of strings.
Note: Unlike the other writeEntry() functions, the old value is _not_ returned here!
- Parameters:
-
pKey The key to write rValue The list to write sep The list separator (default is ","). bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
- See also:
- writeEntry()
Definition at line 1326 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const char * | pKey, | |
const QVariant & | rValue, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
writeEntry() Overridden to accept a property.
Note: Unlike the other writeEntry() functions, the old value is _not_ returned here!
- Parameters:
-
pKey The key to write rValue The property to write bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
- See also:
- writeEntry()
Definition at line 1234 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const QString & | pKey, | |
const QVariant & | rValue, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
writeEntry() Overridden to accept a property.
Note: Unlike the other writeEntry() functions, the old value is _not_ returned here!
- Parameters:
-
pKey The key to write rValue The property to write bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
- See also:
- writeEntry()
Definition at line 1227 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const char * | pKey, | |
const QString & | pValue, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
Writes a key/value pair.
This is stored in the most specific config file when destroying the config object or when calling sync().
- Parameters:
-
pKey The key to write. pValue The value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1020 of file kconfigbase.cpp.
void KConfigBase::writeEntry | ( | const QString & | pKey, | |
const QString & | pValue, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
Writes a key/value pair.
This is stored in the most specific config file when destroying the config object or when calling sync().
If you want to write a path, please use writePathEntry().
- Parameters:
-
pKey The key to write. pValue The value to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1012 of file kconfigbase.cpp.
void KConfigBase::writePathEntry | ( | const char * | pKey, | |
const QStringList & | rValue, | |||
char | sep = ',' , |
|||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
writePathEntry() overridden to accept a list of paths (strings).
It is checked whether the paths are located under $HOME. If so each of the paths are written out with the user's home-directory replaced with $HOME. The paths should be read back with readPathListEntry()
- Parameters:
-
pKey The key to write rValue The list to write sep The list separator (default is ","). bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
- See also:
- writePathEntry()
- Since:
- 3.1.3
Definition at line 1144 of file kconfigbase.cpp.
void KConfigBase::writePathEntry | ( | const QString & | pKey, | |
const QStringList & | rValue, | |||
char | sep = ',' , |
|||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
writePathEntry() overridden to accept a list of paths (strings).
It is checked whether the paths are located under $HOME. If so each of the paths are written out with the user's home-directory replaced with $HOME. The paths should be read back with readPathListEntry()
- Parameters:
-
pKey The key to write rValue The list to write sep The list separator (default is ","). bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
- See also:
- writePathEntry()
- Since:
- 3.1.3
Definition at line 1137 of file kconfigbase.cpp.
void KConfigBase::writePathEntry | ( | const char * | pKey, | |
const QString & | path, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
Writes a file path.
It is checked whether the path is located under $HOME. If so the path is written out with the user's home-directory replaced with $HOME. The path should be read back with readPathEntry()
- Parameters:
-
pKey The key to write. path The path to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1130 of file kconfigbase.cpp.
void KConfigBase::writePathEntry | ( | const QString & | pKey, | |
const QString & | path, | |||
bool | bPersistent = true , |
|||
bool | bGlobal = false , |
|||
bool | bNLS = false | |||
) |
Writes a file path.
It is checked whether the path is located under $HOME. If so the path is written out with the user's home-directory replaced with $HOME. The path should be read back with readPathEntry()
- Parameters:
-
pKey The key to write. path The path to write. bPersistent If bPersistent
is false, the entry's dirty flag will not be set and thus the entry will not be written to disk at deletion time.bGlobal If bGlobal
is true, the pair is not saved to the application specific config file, but to the global KDE config file.bNLS If bNLS
is true, the locale tag is added to the key when writing it back.
Definition at line 1061 of file kconfigbase.cpp.
Member Data Documentation
QCString KConfigBase::aLocaleString [protected] |
The locale to retrieve keys under if possible, i.e en_US or fr.
Definition at line 1995 of file kconfigbase.h.
KConfigBackEnd* KConfigBase::backEnd [protected] |
A back end for loading/saving to disk in a particular format.
Definition at line 1975 of file kconfigbase.h.
bool KConfigBase::bDirty [protected] |
Indicates whether there are any dirty entries in the config object that need to be written back to disk.
Definition at line 2000 of file kconfigbase.h.
bool KConfigBase::bExpand [mutable, protected] |
Definition at line 2004 of file kconfigbase.h.
bool KConfigBase::bLocaleInitialized [protected] |
Definition at line 2002 of file kconfigbase.h.
bool KConfigBase::bReadOnly [protected] |
Definition at line 2003 of file kconfigbase.h.
QCString KConfigBase::mGroup [protected] |
The documentation for this class was generated from the following files: