KDECore
KDesktopFile Class Reference
KDE Desktop File Management. More...
#include <kdesktopfile.h>
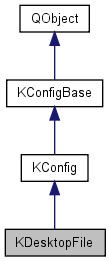
Public Member Functions | |
KDesktopFile * | copyTo (const QString &file) const |
KDE_DEPRECATED QString | filename () const |
QString | fileName () const |
bool | hasActionGroup (const QString &group) const |
bool | hasApplicationType () const |
bool | hasDeviceType () const |
bool | hasLinkType () const |
bool | hasMimeTypeType () const |
KDesktopFile (const QString &fileName, bool readOnly=false, const char *resType="apps") | |
QStringList | readActions () const |
QString | readComment () const |
QString | readDevice () const |
QString | readDocPath () const |
QString | readGenericName () const |
QString | readIcon () const |
QString | readName () const |
QString | readPath () const |
QString | readType () const |
QString | readURL () const |
QString | resource () const |
void | setActionGroup (const QString &group) |
QStringList | sortOrder () const |
bool | tryExec () const |
virtual | ~KDesktopFile () |
Static Public Member Functions | |
static bool | isAuthorizedDesktopFile (const QString &path) |
static bool | isDesktopFile (const QString &path) |
static QString | locateLocal (const QString &path) |
Protected Member Functions | |
virtual void | virtual_hook (int id, void *data) |
Detailed Description
KDE Desktop File Management.
- See also:
- KConfigBase KConfig KDE Desktop File Management class
Definition at line 34 of file kdesktopfile.h.
Constructor & Destructor Documentation
KDesktopFile::KDesktopFile | ( | const QString & | fileName, | |
bool | readOnly = false , |
|||
const char * | resType = "apps" | |||
) |
Constructs a KDesktopFile object and make it either read-write or read-only.
- Parameters:
-
fileName The name or path of the desktop file. If it is not absolute, it will be located using the resource type resType
.readOnly Whether the object should be read-only. resType Allows you to change what sort of resource to search for if fileName
is not absolute. For instance, you might want to specify "config".
Definition at line 41 of file kdesktopfile.cpp.
KDesktopFile::~KDesktopFile | ( | ) | [virtual] |
Destructs the KDesktopFile object.
Writes back any dirty configuration entries.
Definition at line 54 of file kdesktopfile.cpp.
Member Function Documentation
KDesktopFile * KDesktopFile::copyTo | ( | const QString & | file | ) | const |
Copies all entries from this config object to a new KDesktopFile object that will save itself to file
.
Actual saving to file
happens when the returned object is destructed or when sync() is called upon it.
- Parameters:
-
file the new KDesktopFile object it will save itself to.
- Since:
- 3.2
Definition at line 338 of file kdesktopfile.cpp.
KDE_DEPRECATED QString KDesktopFile::filename | ( | ) | const [inline] |
QString KDesktopFile::fileName | ( | ) | const |
Returns the file name.
- Returns:
- The filename as passed to the constructor.
the filename as passed to the constructor.
Definition at line 311 of file kdesktopfile.cpp.
Returns true if the action group exists, false otherwise.
- Parameters:
-
group the action group to test
- Returns:
- true if the action group exists
Definition at line 224 of file kdesktopfile.cpp.
bool KDesktopFile::hasApplicationType | ( | ) | const |
Checks whether there is an entry "Type=Application".
- Returns:
- true if there is a "Type=Application" entry
Definition at line 234 of file kdesktopfile.cpp.
bool KDesktopFile::hasDeviceType | ( | ) | const |
Checks whether there is an entry "Type=FSDev".
- Returns:
- true if there is a "Type=FSDev" entry
Definition at line 244 of file kdesktopfile.cpp.
bool KDesktopFile::hasLinkType | ( | ) | const |
Checks whether there is a "Type=Link" entry.
The link points to the "URL=" entry.
- Returns:
- true if there is a "Type=Link" entry
Definition at line 229 of file kdesktopfile.cpp.
bool KDesktopFile::hasMimeTypeType | ( | ) | const |
Checks whether there is an entry "Type=MimeType".
- Returns:
- true if there is a "Type=MimeType" entry
Definition at line 239 of file kdesktopfile.cpp.
Checks whether the user is authorized to run this desktop file.
By default users are authorized to run all desktop files but the KIOSK framework can be used to activate certain restrictions. See README.kiosk for more information.
- Parameters:
-
path the file to check
- Returns:
- true if the user is authorized to run the file
- Since:
- 3.1
Definition at line 123 of file kdesktopfile.cpp.
Checks whether this is really a desktop file.
The check is performed looking at the file extension (the file is not opened). Currently, valid extensions are ".kdelnk" and ".desktop".
- Parameters:
-
path the path of the file to check
- Returns:
- true if the file appears to be a desktop file.
Definition at line 111 of file kdesktopfile.cpp.
Returns the location where changes for the .desktop file path
should be written to.
- Since:
- 3.2
Definition at line 59 of file kdesktopfile.cpp.
QStringList KDesktopFile::readActions | ( | ) | const |
Returns a list of the "Actions=" entries.
- Returns:
- the list of actions
Definition at line 214 of file kdesktopfile.cpp.
QString KDesktopFile::readComment | ( | ) | const |
Returns the value of the "Comment=" entry.
- Returns:
- the comment or QString::null if not specified
Definition at line 163 of file kdesktopfile.cpp.
QString KDesktopFile::readDevice | ( | ) | const |
Returns the value of the "Dev=" entry.
- Returns:
- the device or QString::null if not specified
Definition at line 178 of file kdesktopfile.cpp.
QString KDesktopFile::readDocPath | ( | ) | const |
Returns the value of the "X-DocPath=" Or "DocPath=" entry.
X-DocPath should be used and DocPath is depreciated and will one day be not supported.
- Returns:
- The value of the "X-DocPath=" Or "DocPath=" entry.
- Since:
- 3.1
Definition at line 328 of file kdesktopfile.cpp.
QString KDesktopFile::readGenericName | ( | ) | const |
Returns the value of the "GenericName=" entry.
- Returns:
- the generic name or QString::null if not specified
Definition at line 168 of file kdesktopfile.cpp.
QString KDesktopFile::readIcon | ( | ) | const |
Returns the value of the "Icon=" entry.
- Returns:
- the icon or QString::null if not specified
Definition at line 153 of file kdesktopfile.cpp.
QString KDesktopFile::readName | ( | ) | const |
Returns the value of the "Name=" entry.
- Returns:
- the name or QString::null if not specified
Definition at line 158 of file kdesktopfile.cpp.
QString KDesktopFile::readPath | ( | ) | const |
Returns the value of the "Path=" entry.
- Returns:
- the path or QString::null if not specified
Definition at line 173 of file kdesktopfile.cpp.
QString KDesktopFile::readType | ( | ) | const |
Returns the value of the "Type=" entry.
- Returns:
- the type or QString::null if not specified
Definition at line 148 of file kdesktopfile.cpp.
QString KDesktopFile::readURL | ( | ) | const |
Returns the value of the "URL=" entry.
- Returns:
- the URL or QString::null if not specified
Definition at line 183 of file kdesktopfile.cpp.
QString KDesktopFile::resource | ( | ) | const |
Returns the resource.
- Returns:
- The resource type as passed to the constructor.
the resource type as passed to the constructor.
Definition at line 317 of file kdesktopfile.cpp.
void KDesktopFile::setActionGroup | ( | const QString & | group | ) |
Sets the desktop action group.
- Parameters:
-
group the new action group
Definition at line 219 of file kdesktopfile.cpp.
QStringList KDesktopFile::sortOrder | ( | ) | const |
Returns the entry of the "SortOrder=" entry.
- Returns:
- the value of the "SortOrder=" entry.
Definition at line 320 of file kdesktopfile.cpp.
bool KDesktopFile::tryExec | ( | ) | const |
Checks whether the TryExec field contains a binary which is found on the local system.
- Returns:
- true if TryExec contains an existing binary
Definition at line 250 of file kdesktopfile.cpp.
void KDesktopFile::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
The documentation for this class was generated from the following files: