KDECore
KConfigBackEnd Class Reference
Abstract base class for KDE configuration file loading/saving. More...
#include <kconfigbackend.h>
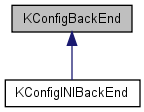
Public Member Functions | |
void | changeFileName (const QString &_fileName, const char *_resType, bool _useKDEGlobals) |
bool | checkConfigFilesWritable (bool warnUser) |
KDE_DEPRECATED QString | filename () const |
QString | fileName () const |
virtual KConfigBase::ConfigState | getConfigState () const |
KConfigBackEnd (KConfigBase *_config, const QString &_fileName, const char *_resType, bool _useKDEGlobals) | |
KLockFile::Ptr | lockFile (bool bGlobal=false) |
virtual bool | parseConfigFiles ()=0 |
const char * | resource () const |
void | setFileWriteMode (int mode) |
void | setLocaleString (const QCString &_localeString) |
virtual void | sync (bool bMerge=true)=0 |
virtual | ~KConfigBackEnd () |
Protected Member Functions | |
virtual void | virtual_hook (int id, void *data) |
Protected Attributes | |
bool | bFileImmutable: 1 |
KConfigBackEndPrivate * | d |
QCString | localeString |
KConfigBase::ConfigState | mConfigState |
int | mFileMode |
QString | mfileName |
QString | mGlobalFileName |
QString | mLocalFileName |
KConfigBase * | pConfig |
QCString | resType |
bool | useKDEGlobals: 1 |
Detailed Description
Abstract base class for KDE configuration file loading/saving.This class forms the base for all classes that implement some manner of loading/saving to configuration files. It is an abstract base class, meaning that you cannot directly instantiate objects of this class. As of right now, the only back end available is one to read/write to INI-style files, but in the future, other formats may be available, such as XML or a database.
Definition at line 47 of file kconfigbackend.h.
Constructor & Destructor Documentation
KConfigBackEnd::KConfigBackEnd | ( | KConfigBase * | _config, | |
const QString & | _fileName, | |||
const char * | _resType, | |||
bool | _useKDEGlobals | |||
) |
Constructs a configuration back end.
- Parameters:
-
_config Specifies the configuration object which values will be passed to as they are read, or from where values to be written to will be obtained from. _fileName The name of the file in which config data is stored. All registered configuration directories will be looked in in order of decreasing relevance. _resType the resource type of the fileName specified, _if_ it is not an absolute path (otherwise this parameter is ignored). _useKDEGlobals If true, the user's system-wide kdeglobals file will be imported into the config object. If false, only the filename specified will be dealt with.
Definition at line 296 of file kconfigbackend.cpp.
KConfigBackEnd::~KConfigBackEnd | ( | ) | [virtual] |
Member Function Documentation
void KConfigBackEnd::changeFileName | ( | const QString & | _fileName, | |
const char * | _resType, | |||
bool | _useKDEGlobals | |||
) |
Changes the filenames associated with this back end.
You should probably reparse your config info after doing this.
- Parameters:
-
_fileName the new filename to use _resType the resource type of the fileName specified, _if_ it is not an absolute path (otherwise this parameter is ignored). _useKDEGlobals specifies whether or not to also parse the global KDE configuration files.
Definition at line 243 of file kconfigbackend.cpp.
Check whether the config files are writable.
- Parameters:
-
warnUser Warn the user if the configuration files are not writable.
- Returns:
- Indicates that all of the configuration files used are writable.
- Since:
- 3.2
Definition at line 1100 of file kconfigbackend.cpp.
KDE_DEPRECATED QString KConfigBackEnd::filename | ( | ) | const [inline] |
QString KConfigBackEnd::fileName | ( | ) | const [inline] |
Returns the filename as passed to the constructor.
- Returns:
- the filename as passed to the constructor.
Definition at line 119 of file kconfigbackend.h.
virtual KConfigBase::ConfigState KConfigBackEnd::getConfigState | ( | ) | const [inline, virtual] |
Returns the state of the app-config object.
- See also:
- KConfig::getConfigState
Definition at line 112 of file kconfigbackend.h.
KLockFile::Ptr KConfigBackEnd::lockFile | ( | bool | bGlobal = false |
) |
Returns a lock file object for the configuration file.
- Parameters:
-
bGlobal If true, returns a lock file object for kdeglobals
- Since:
- 3.3
Definition at line 269 of file kconfigbackend.cpp.
virtual bool KConfigBackEnd::parseConfigFiles | ( | ) | [pure virtual] |
Parses all configuration files for a configuration object.
This method must be reimplemented by the derived classes.
- Returns:
- Whether or not parsing was successful.
Implemented in KConfigINIBackEnd.
const char* KConfigBackEnd::resource | ( | ) | const [inline] |
Returns the resource type as passed to the constructor.
- Returns:
- the resource type as passed to the constructor.
Definition at line 125 of file kconfigbackend.h.
void KConfigBackEnd::setFileWriteMode | ( | int | mode | ) |
Set the file mode for newly created files.
- Parameters:
-
mode the filemode (as in chmod)
Definition at line 311 of file kconfigbackend.cpp.
void KConfigBackEnd::setLocaleString | ( | const QCString & | _localeString | ) | [inline] |
Set the locale string that defines the current language.
- Parameters:
-
_localeString the identifier of the language
- See also:
- KLocale
Definition at line 132 of file kconfigbackend.h.
virtual void KConfigBackEnd::sync | ( | bool | bMerge = true |
) | [pure virtual] |
Writes configuration data to file(s).
This method must be reimplemented by the derived classes.
- Parameters:
-
bMerge Specifies whether the old config file already on disk should be merged in with the data in memory. If true, data is read off the disk and merged. If false, the on-disk file is removed and only in-memory data is written out.
Implemented in KConfigINIBackEnd.
void KConfigBackEnd::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Member Data Documentation
bool KConfigBackEnd::bFileImmutable [protected] |
Definition at line 169 of file kconfigbackend.h.
KConfigBackEndPrivate* KConfigBackEnd::d [protected] |
Definition at line 179 of file kconfigbackend.h.
QCString KConfigBackEnd::localeString [protected] |
Definition at line 170 of file kconfigbackend.h.
KConfigBase::ConfigState KConfigBackEnd::mConfigState [protected] |
Definition at line 173 of file kconfigbackend.h.
int KConfigBackEnd::mFileMode [protected] |
Definition at line 174 of file kconfigbackend.h.
QString KConfigBackEnd::mfileName [protected] |
Definition at line 166 of file kconfigbackend.h.
QString KConfigBackEnd::mGlobalFileName [protected] |
Definition at line 172 of file kconfigbackend.h.
QString KConfigBackEnd::mLocalFileName [protected] |
Definition at line 171 of file kconfigbackend.h.
KConfigBase* KConfigBackEnd::pConfig [protected] |
Definition at line 164 of file kconfigbackend.h.
QCString KConfigBackEnd::resType [protected] |
Definition at line 167 of file kconfigbackend.h.
bool KConfigBackEnd::useKDEGlobals [protected] |
Definition at line 168 of file kconfigbackend.h.
The documentation for this class was generated from the following files: